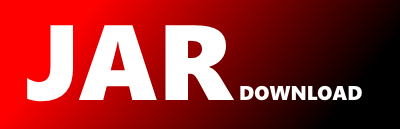
com.azure.resourcemanager.sql.fluent.models.RecommendedActionProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-sql Show documentation
Show all versions of azure-resourcemanager-sql Show documentation
This package contains Microsoft Azure Sql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.sql.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.sql.models.RecommendedActionErrorInfo;
import com.azure.resourcemanager.sql.models.RecommendedActionImpactRecord;
import com.azure.resourcemanager.sql.models.RecommendedActionImplementationInfo;
import com.azure.resourcemanager.sql.models.RecommendedActionInitiatedBy;
import com.azure.resourcemanager.sql.models.RecommendedActionMetricInfo;
import com.azure.resourcemanager.sql.models.RecommendedActionStateInfo;
import java.io.IOException;
import java.time.Duration;
import java.time.OffsetDateTime;
import java.util.List;
import java.util.Map;
/**
* Properties for a Database, Server or Elastic Pool Recommended Action.
*/
@Fluent
public final class RecommendedActionProperties implements JsonSerializable {
/*
* Gets the reason for recommending this action. e.g., DuplicateIndex
*/
private String recommendationReason;
/*
* Gets the time since when this recommended action is valid.
*/
private OffsetDateTime validSince;
/*
* Gets time when this recommended action was last refreshed.
*/
private OffsetDateTime lastRefresh;
/*
* Gets the info of the current state the recommended action is in.
*/
private RecommendedActionStateInfo state;
/*
* Gets if this recommended action is actionable by user
*/
private Boolean isExecutableAction;
/*
* Gets if changes applied by this recommended action can be reverted by user
*/
private Boolean isRevertableAction;
/*
* Gets if this recommended action was suggested some time ago but user chose to ignore this and system added a new
* recommended action again.
*/
private Boolean isArchivedAction;
/*
* Gets the time when system started applying this recommended action on the user resource. e.g., index creation
* start time
*/
private OffsetDateTime executeActionStartTime;
/*
* Gets the time taken for applying this recommended action on user resource. e.g., time taken for index creation
*/
private Duration executeActionDuration;
/*
* Gets the time when system started reverting changes of this recommended action on user resource. e.g., time when
* index drop is executed.
*/
private OffsetDateTime revertActionStartTime;
/*
* Gets the time taken for reverting changes of this recommended action on user resource. e.g., time taken for
* dropping the created index.
*/
private Duration revertActionDuration;
/*
* Gets if approval for applying this recommended action was given by user/system.
*/
private RecommendedActionInitiatedBy executeActionInitiatedBy;
/*
* Gets the time when this recommended action was approved for execution.
*/
private OffsetDateTime executeActionInitiatedTime;
/*
* Gets if approval for reverting this recommended action was given by user/system.
*/
private RecommendedActionInitiatedBy revertActionInitiatedBy;
/*
* Gets the time when this recommended action was approved for revert.
*/
private OffsetDateTime revertActionInitiatedTime;
/*
* Gets the impact of this recommended action. Possible values are 1 - Low impact, 2 - Medium Impact and 3 - High
* Impact
*/
private Integer score;
/*
* Gets the implementation details of this recommended action for user to apply it manually.
*/
private RecommendedActionImplementationInfo implementationDetails;
/*
* Gets the error details if and why this recommended action is put to error state.
*/
private RecommendedActionErrorInfo errorDetails;
/*
* Gets the estimated impact info for this recommended action e.g., Estimated CPU gain, Estimated Disk Space change
*/
private List estimatedImpact;
/*
* Gets the observed/actual impact info for this recommended action e.g., Actual CPU gain, Actual Disk Space change
*/
private List observedImpact;
/*
* Gets the time series info of metrics for this recommended action e.g., CPU consumption time series
*/
private List timeSeries;
/*
* Gets the linked objects, if any.
*/
private List linkedObjects;
/*
* Gets additional details specific to this recommended action.
*/
private Map details;
/**
* Creates an instance of RecommendedActionProperties class.
*/
public RecommendedActionProperties() {
}
/**
* Get the recommendationReason property: Gets the reason for recommending this action. e.g., DuplicateIndex.
*
* @return the recommendationReason value.
*/
public String recommendationReason() {
return this.recommendationReason;
}
/**
* Get the validSince property: Gets the time since when this recommended action is valid.
*
* @return the validSince value.
*/
public OffsetDateTime validSince() {
return this.validSince;
}
/**
* Get the lastRefresh property: Gets time when this recommended action was last refreshed.
*
* @return the lastRefresh value.
*/
public OffsetDateTime lastRefresh() {
return this.lastRefresh;
}
/**
* Get the state property: Gets the info of the current state the recommended action is in.
*
* @return the state value.
*/
public RecommendedActionStateInfo state() {
return this.state;
}
/**
* Set the state property: Gets the info of the current state the recommended action is in.
*
* @param state the state value to set.
* @return the RecommendedActionProperties object itself.
*/
public RecommendedActionProperties withState(RecommendedActionStateInfo state) {
this.state = state;
return this;
}
/**
* Get the isExecutableAction property: Gets if this recommended action is actionable by user.
*
* @return the isExecutableAction value.
*/
public Boolean isExecutableAction() {
return this.isExecutableAction;
}
/**
* Get the isRevertableAction property: Gets if changes applied by this recommended action can be reverted by user.
*
* @return the isRevertableAction value.
*/
public Boolean isRevertableAction() {
return this.isRevertableAction;
}
/**
* Get the isArchivedAction property: Gets if this recommended action was suggested some time ago but user chose to
* ignore this and system added a new recommended action again.
*
* @return the isArchivedAction value.
*/
public Boolean isArchivedAction() {
return this.isArchivedAction;
}
/**
* Get the executeActionStartTime property: Gets the time when system started applying this recommended action on
* the user resource. e.g., index creation start time.
*
* @return the executeActionStartTime value.
*/
public OffsetDateTime executeActionStartTime() {
return this.executeActionStartTime;
}
/**
* Get the executeActionDuration property: Gets the time taken for applying this recommended action on user
* resource. e.g., time taken for index creation.
*
* @return the executeActionDuration value.
*/
public Duration executeActionDuration() {
return this.executeActionDuration;
}
/**
* Get the revertActionStartTime property: Gets the time when system started reverting changes of this recommended
* action on user resource. e.g., time when index drop is executed.
*
* @return the revertActionStartTime value.
*/
public OffsetDateTime revertActionStartTime() {
return this.revertActionStartTime;
}
/**
* Get the revertActionDuration property: Gets the time taken for reverting changes of this recommended action on
* user resource. e.g., time taken for dropping the created index.
*
* @return the revertActionDuration value.
*/
public Duration revertActionDuration() {
return this.revertActionDuration;
}
/**
* Get the executeActionInitiatedBy property: Gets if approval for applying this recommended action was given by
* user/system.
*
* @return the executeActionInitiatedBy value.
*/
public RecommendedActionInitiatedBy executeActionInitiatedBy() {
return this.executeActionInitiatedBy;
}
/**
* Get the executeActionInitiatedTime property: Gets the time when this recommended action was approved for
* execution.
*
* @return the executeActionInitiatedTime value.
*/
public OffsetDateTime executeActionInitiatedTime() {
return this.executeActionInitiatedTime;
}
/**
* Get the revertActionInitiatedBy property: Gets if approval for reverting this recommended action was given by
* user/system.
*
* @return the revertActionInitiatedBy value.
*/
public RecommendedActionInitiatedBy revertActionInitiatedBy() {
return this.revertActionInitiatedBy;
}
/**
* Get the revertActionInitiatedTime property: Gets the time when this recommended action was approved for revert.
*
* @return the revertActionInitiatedTime value.
*/
public OffsetDateTime revertActionInitiatedTime() {
return this.revertActionInitiatedTime;
}
/**
* Get the score property: Gets the impact of this recommended action. Possible values are 1 - Low impact, 2 -
* Medium Impact and 3 - High Impact.
*
* @return the score value.
*/
public Integer score() {
return this.score;
}
/**
* Get the implementationDetails property: Gets the implementation details of this recommended action for user to
* apply it manually.
*
* @return the implementationDetails value.
*/
public RecommendedActionImplementationInfo implementationDetails() {
return this.implementationDetails;
}
/**
* Get the errorDetails property: Gets the error details if and why this recommended action is put to error state.
*
* @return the errorDetails value.
*/
public RecommendedActionErrorInfo errorDetails() {
return this.errorDetails;
}
/**
* Get the estimatedImpact property: Gets the estimated impact info for this recommended action e.g., Estimated CPU
* gain, Estimated Disk Space change.
*
* @return the estimatedImpact value.
*/
public List estimatedImpact() {
return this.estimatedImpact;
}
/**
* Get the observedImpact property: Gets the observed/actual impact info for this recommended action e.g., Actual
* CPU gain, Actual Disk Space change.
*
* @return the observedImpact value.
*/
public List observedImpact() {
return this.observedImpact;
}
/**
* Get the timeSeries property: Gets the time series info of metrics for this recommended action e.g., CPU
* consumption time series.
*
* @return the timeSeries value.
*/
public List timeSeries() {
return this.timeSeries;
}
/**
* Get the linkedObjects property: Gets the linked objects, if any.
*
* @return the linkedObjects value.
*/
public List linkedObjects() {
return this.linkedObjects;
}
/**
* Get the details property: Gets additional details specific to this recommended action.
*
* @return the details value.
*/
public Map details() {
return this.details;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (state() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property state in model RecommendedActionProperties"));
} else {
state().validate();
}
if (implementationDetails() != null) {
implementationDetails().validate();
}
if (errorDetails() != null) {
errorDetails().validate();
}
if (estimatedImpact() != null) {
estimatedImpact().forEach(e -> e.validate());
}
if (observedImpact() != null) {
observedImpact().forEach(e -> e.validate());
}
if (timeSeries() != null) {
timeSeries().forEach(e -> e.validate());
}
}
private static final ClientLogger LOGGER = new ClientLogger(RecommendedActionProperties.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("state", this.state);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of RecommendedActionProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of RecommendedActionProperties if the JsonReader was pointing to an instance of it, or null
* if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the RecommendedActionProperties.
*/
public static RecommendedActionProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
RecommendedActionProperties deserializedRecommendedActionProperties = new RecommendedActionProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("state".equals(fieldName)) {
deserializedRecommendedActionProperties.state = RecommendedActionStateInfo.fromJson(reader);
} else if ("recommendationReason".equals(fieldName)) {
deserializedRecommendedActionProperties.recommendationReason = reader.getString();
} else if ("validSince".equals(fieldName)) {
deserializedRecommendedActionProperties.validSince = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("lastRefresh".equals(fieldName)) {
deserializedRecommendedActionProperties.lastRefresh = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("isExecutableAction".equals(fieldName)) {
deserializedRecommendedActionProperties.isExecutableAction
= reader.getNullable(JsonReader::getBoolean);
} else if ("isRevertableAction".equals(fieldName)) {
deserializedRecommendedActionProperties.isRevertableAction
= reader.getNullable(JsonReader::getBoolean);
} else if ("isArchivedAction".equals(fieldName)) {
deserializedRecommendedActionProperties.isArchivedAction
= reader.getNullable(JsonReader::getBoolean);
} else if ("executeActionStartTime".equals(fieldName)) {
deserializedRecommendedActionProperties.executeActionStartTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("executeActionDuration".equals(fieldName)) {
deserializedRecommendedActionProperties.executeActionDuration
= reader.getNullable(nonNullReader -> Duration.parse(nonNullReader.getString()));
} else if ("revertActionStartTime".equals(fieldName)) {
deserializedRecommendedActionProperties.revertActionStartTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("revertActionDuration".equals(fieldName)) {
deserializedRecommendedActionProperties.revertActionDuration
= reader.getNullable(nonNullReader -> Duration.parse(nonNullReader.getString()));
} else if ("executeActionInitiatedBy".equals(fieldName)) {
deserializedRecommendedActionProperties.executeActionInitiatedBy
= RecommendedActionInitiatedBy.fromString(reader.getString());
} else if ("executeActionInitiatedTime".equals(fieldName)) {
deserializedRecommendedActionProperties.executeActionInitiatedTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("revertActionInitiatedBy".equals(fieldName)) {
deserializedRecommendedActionProperties.revertActionInitiatedBy
= RecommendedActionInitiatedBy.fromString(reader.getString());
} else if ("revertActionInitiatedTime".equals(fieldName)) {
deserializedRecommendedActionProperties.revertActionInitiatedTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else if ("score".equals(fieldName)) {
deserializedRecommendedActionProperties.score = reader.getNullable(JsonReader::getInt);
} else if ("implementationDetails".equals(fieldName)) {
deserializedRecommendedActionProperties.implementationDetails
= RecommendedActionImplementationInfo.fromJson(reader);
} else if ("errorDetails".equals(fieldName)) {
deserializedRecommendedActionProperties.errorDetails = RecommendedActionErrorInfo.fromJson(reader);
} else if ("estimatedImpact".equals(fieldName)) {
List estimatedImpact
= reader.readArray(reader1 -> RecommendedActionImpactRecord.fromJson(reader1));
deserializedRecommendedActionProperties.estimatedImpact = estimatedImpact;
} else if ("observedImpact".equals(fieldName)) {
List observedImpact
= reader.readArray(reader1 -> RecommendedActionImpactRecord.fromJson(reader1));
deserializedRecommendedActionProperties.observedImpact = observedImpact;
} else if ("timeSeries".equals(fieldName)) {
List timeSeries
= reader.readArray(reader1 -> RecommendedActionMetricInfo.fromJson(reader1));
deserializedRecommendedActionProperties.timeSeries = timeSeries;
} else if ("linkedObjects".equals(fieldName)) {
List linkedObjects = reader.readArray(reader1 -> reader1.getString());
deserializedRecommendedActionProperties.linkedObjects = linkedObjects;
} else if ("details".equals(fieldName)) {
Map details = reader.readMap(reader1 -> reader1.readUntyped());
deserializedRecommendedActionProperties.details = details;
} else {
reader.skipChildren();
}
}
return deserializedRecommendedActionProperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy