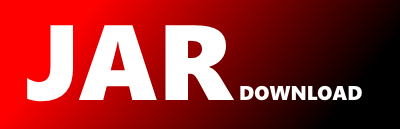
com.azure.resourcemanager.sql.fluent.models.SecurityAlertsPolicyPropertiesAutoGenerated Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-sql Show documentation
Show all versions of azure-resourcemanager-sql Show documentation
This package contains Microsoft Azure Sql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.sql.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.sql.models.SecurityAlertPolicyState;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.List;
/**
* Properties of a security alert policy.
*/
@Fluent
public final class SecurityAlertsPolicyPropertiesAutoGenerated
implements JsonSerializable {
/*
* Specifies the state of the policy, whether it is enabled or disabled or a policy has not been applied yet on the
* specific database.
*/
private SecurityAlertPolicyState state;
/*
* Specifies an array of alerts that are disabled. Allowed values are: Sql_Injection, Sql_Injection_Vulnerability,
* Access_Anomaly, Data_Exfiltration, Unsafe_Action, Brute_Force
*/
private List disabledAlerts;
/*
* Specifies an array of e-mail addresses to which the alert is sent.
*/
private List emailAddresses;
/*
* Specifies that the alert is sent to the account administrators.
*/
private Boolean emailAccountAdmins;
/*
* Specifies the blob storage endpoint (e.g. https://MyAccount.blob.core.windows.net). This blob storage will hold
* all Threat Detection audit logs.
*/
private String storageEndpoint;
/*
* Specifies the identifier key of the Threat Detection audit storage account.
*/
private String storageAccountAccessKey;
/*
* Specifies the number of days to keep in the Threat Detection audit logs.
*/
private Integer retentionDays;
/*
* Specifies the UTC creation time of the policy.
*/
private OffsetDateTime creationTime;
/**
* Creates an instance of SecurityAlertsPolicyPropertiesAutoGenerated class.
*/
public SecurityAlertsPolicyPropertiesAutoGenerated() {
}
/**
* Get the state property: Specifies the state of the policy, whether it is enabled or disabled or a policy has not
* been applied yet on the specific database.
*
* @return the state value.
*/
public SecurityAlertPolicyState state() {
return this.state;
}
/**
* Set the state property: Specifies the state of the policy, whether it is enabled or disabled or a policy has not
* been applied yet on the specific database.
*
* @param state the state value to set.
* @return the SecurityAlertsPolicyPropertiesAutoGenerated object itself.
*/
public SecurityAlertsPolicyPropertiesAutoGenerated withState(SecurityAlertPolicyState state) {
this.state = state;
return this;
}
/**
* Get the disabledAlerts property: Specifies an array of alerts that are disabled. Allowed values are:
* Sql_Injection, Sql_Injection_Vulnerability, Access_Anomaly, Data_Exfiltration, Unsafe_Action, Brute_Force.
*
* @return the disabledAlerts value.
*/
public List disabledAlerts() {
return this.disabledAlerts;
}
/**
* Set the disabledAlerts property: Specifies an array of alerts that are disabled. Allowed values are:
* Sql_Injection, Sql_Injection_Vulnerability, Access_Anomaly, Data_Exfiltration, Unsafe_Action, Brute_Force.
*
* @param disabledAlerts the disabledAlerts value to set.
* @return the SecurityAlertsPolicyPropertiesAutoGenerated object itself.
*/
public SecurityAlertsPolicyPropertiesAutoGenerated withDisabledAlerts(List disabledAlerts) {
this.disabledAlerts = disabledAlerts;
return this;
}
/**
* Get the emailAddresses property: Specifies an array of e-mail addresses to which the alert is sent.
*
* @return the emailAddresses value.
*/
public List emailAddresses() {
return this.emailAddresses;
}
/**
* Set the emailAddresses property: Specifies an array of e-mail addresses to which the alert is sent.
*
* @param emailAddresses the emailAddresses value to set.
* @return the SecurityAlertsPolicyPropertiesAutoGenerated object itself.
*/
public SecurityAlertsPolicyPropertiesAutoGenerated withEmailAddresses(List emailAddresses) {
this.emailAddresses = emailAddresses;
return this;
}
/**
* Get the emailAccountAdmins property: Specifies that the alert is sent to the account administrators.
*
* @return the emailAccountAdmins value.
*/
public Boolean emailAccountAdmins() {
return this.emailAccountAdmins;
}
/**
* Set the emailAccountAdmins property: Specifies that the alert is sent to the account administrators.
*
* @param emailAccountAdmins the emailAccountAdmins value to set.
* @return the SecurityAlertsPolicyPropertiesAutoGenerated object itself.
*/
public SecurityAlertsPolicyPropertiesAutoGenerated withEmailAccountAdmins(Boolean emailAccountAdmins) {
this.emailAccountAdmins = emailAccountAdmins;
return this;
}
/**
* Get the storageEndpoint property: Specifies the blob storage endpoint (e.g.
* https://MyAccount.blob.core.windows.net). This blob storage will hold all Threat Detection audit logs.
*
* @return the storageEndpoint value.
*/
public String storageEndpoint() {
return this.storageEndpoint;
}
/**
* Set the storageEndpoint property: Specifies the blob storage endpoint (e.g.
* https://MyAccount.blob.core.windows.net). This blob storage will hold all Threat Detection audit logs.
*
* @param storageEndpoint the storageEndpoint value to set.
* @return the SecurityAlertsPolicyPropertiesAutoGenerated object itself.
*/
public SecurityAlertsPolicyPropertiesAutoGenerated withStorageEndpoint(String storageEndpoint) {
this.storageEndpoint = storageEndpoint;
return this;
}
/**
* Get the storageAccountAccessKey property: Specifies the identifier key of the Threat Detection audit storage
* account.
*
* @return the storageAccountAccessKey value.
*/
public String storageAccountAccessKey() {
return this.storageAccountAccessKey;
}
/**
* Set the storageAccountAccessKey property: Specifies the identifier key of the Threat Detection audit storage
* account.
*
* @param storageAccountAccessKey the storageAccountAccessKey value to set.
* @return the SecurityAlertsPolicyPropertiesAutoGenerated object itself.
*/
public SecurityAlertsPolicyPropertiesAutoGenerated withStorageAccountAccessKey(String storageAccountAccessKey) {
this.storageAccountAccessKey = storageAccountAccessKey;
return this;
}
/**
* Get the retentionDays property: Specifies the number of days to keep in the Threat Detection audit logs.
*
* @return the retentionDays value.
*/
public Integer retentionDays() {
return this.retentionDays;
}
/**
* Set the retentionDays property: Specifies the number of days to keep in the Threat Detection audit logs.
*
* @param retentionDays the retentionDays value to set.
* @return the SecurityAlertsPolicyPropertiesAutoGenerated object itself.
*/
public SecurityAlertsPolicyPropertiesAutoGenerated withRetentionDays(Integer retentionDays) {
this.retentionDays = retentionDays;
return this;
}
/**
* Get the creationTime property: Specifies the UTC creation time of the policy.
*
* @return the creationTime value.
*/
public OffsetDateTime creationTime() {
return this.creationTime;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (state() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property state in model SecurityAlertsPolicyPropertiesAutoGenerated"));
}
}
private static final ClientLogger LOGGER = new ClientLogger(SecurityAlertsPolicyPropertiesAutoGenerated.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("state", this.state == null ? null : this.state.toString());
jsonWriter.writeArrayField("disabledAlerts", this.disabledAlerts,
(writer, element) -> writer.writeString(element));
jsonWriter.writeArrayField("emailAddresses", this.emailAddresses,
(writer, element) -> writer.writeString(element));
jsonWriter.writeBooleanField("emailAccountAdmins", this.emailAccountAdmins);
jsonWriter.writeStringField("storageEndpoint", this.storageEndpoint);
jsonWriter.writeStringField("storageAccountAccessKey", this.storageAccountAccessKey);
jsonWriter.writeNumberField("retentionDays", this.retentionDays);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of SecurityAlertsPolicyPropertiesAutoGenerated from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of SecurityAlertsPolicyPropertiesAutoGenerated if the JsonReader was pointing to an instance
* of it, or null if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the SecurityAlertsPolicyPropertiesAutoGenerated.
*/
public static SecurityAlertsPolicyPropertiesAutoGenerated fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
SecurityAlertsPolicyPropertiesAutoGenerated deserializedSecurityAlertsPolicyPropertiesAutoGenerated
= new SecurityAlertsPolicyPropertiesAutoGenerated();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("state".equals(fieldName)) {
deserializedSecurityAlertsPolicyPropertiesAutoGenerated.state
= SecurityAlertPolicyState.fromString(reader.getString());
} else if ("disabledAlerts".equals(fieldName)) {
List disabledAlerts = reader.readArray(reader1 -> reader1.getString());
deserializedSecurityAlertsPolicyPropertiesAutoGenerated.disabledAlerts = disabledAlerts;
} else if ("emailAddresses".equals(fieldName)) {
List emailAddresses = reader.readArray(reader1 -> reader1.getString());
deserializedSecurityAlertsPolicyPropertiesAutoGenerated.emailAddresses = emailAddresses;
} else if ("emailAccountAdmins".equals(fieldName)) {
deserializedSecurityAlertsPolicyPropertiesAutoGenerated.emailAccountAdmins
= reader.getNullable(JsonReader::getBoolean);
} else if ("storageEndpoint".equals(fieldName)) {
deserializedSecurityAlertsPolicyPropertiesAutoGenerated.storageEndpoint = reader.getString();
} else if ("storageAccountAccessKey".equals(fieldName)) {
deserializedSecurityAlertsPolicyPropertiesAutoGenerated.storageAccountAccessKey
= reader.getString();
} else if ("retentionDays".equals(fieldName)) {
deserializedSecurityAlertsPolicyPropertiesAutoGenerated.retentionDays
= reader.getNullable(JsonReader::getInt);
} else if ("creationTime".equals(fieldName)) {
deserializedSecurityAlertsPolicyPropertiesAutoGenerated.creationTime = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else {
reader.skipChildren();
}
}
return deserializedSecurityAlertsPolicyPropertiesAutoGenerated;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy