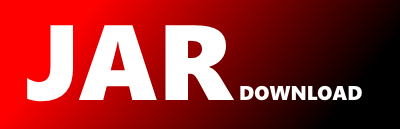
com.azure.resourcemanager.sql.fluent.models.SensitivityLabelUpdatePropertiesInner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-sql Show documentation
Show all versions of azure-resourcemanager-sql Show documentation
This package contains Microsoft Azure Sql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.sql.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.sql.models.SensitivityLabelUpdateKind;
import java.io.IOException;
/**
* Properties of an operation executed on a sensitivity label.
*/
@Fluent
public final class SensitivityLabelUpdatePropertiesInner
implements JsonSerializable {
/*
* The op property.
*/
private SensitivityLabelUpdateKind op;
/*
* Schema name of the column to update.
*/
private String schema;
/*
* Table name of the column to update.
*/
private String table;
/*
* Column name to update.
*/
private String column;
/*
* The sensitivity label information to apply on a column.
*/
private SensitivityLabelInner sensitivityLabel;
/**
* Creates an instance of SensitivityLabelUpdatePropertiesInner class.
*/
public SensitivityLabelUpdatePropertiesInner() {
}
/**
* Get the op property: The op property.
*
* @return the op value.
*/
public SensitivityLabelUpdateKind op() {
return this.op;
}
/**
* Set the op property: The op property.
*
* @param op the op value to set.
* @return the SensitivityLabelUpdatePropertiesInner object itself.
*/
public SensitivityLabelUpdatePropertiesInner withOp(SensitivityLabelUpdateKind op) {
this.op = op;
return this;
}
/**
* Get the schema property: Schema name of the column to update.
*
* @return the schema value.
*/
public String schema() {
return this.schema;
}
/**
* Set the schema property: Schema name of the column to update.
*
* @param schema the schema value to set.
* @return the SensitivityLabelUpdatePropertiesInner object itself.
*/
public SensitivityLabelUpdatePropertiesInner withSchema(String schema) {
this.schema = schema;
return this;
}
/**
* Get the table property: Table name of the column to update.
*
* @return the table value.
*/
public String table() {
return this.table;
}
/**
* Set the table property: Table name of the column to update.
*
* @param table the table value to set.
* @return the SensitivityLabelUpdatePropertiesInner object itself.
*/
public SensitivityLabelUpdatePropertiesInner withTable(String table) {
this.table = table;
return this;
}
/**
* Get the column property: Column name to update.
*
* @return the column value.
*/
public String column() {
return this.column;
}
/**
* Set the column property: Column name to update.
*
* @param column the column value to set.
* @return the SensitivityLabelUpdatePropertiesInner object itself.
*/
public SensitivityLabelUpdatePropertiesInner withColumn(String column) {
this.column = column;
return this;
}
/**
* Get the sensitivityLabel property: The sensitivity label information to apply on a column.
*
* @return the sensitivityLabel value.
*/
public SensitivityLabelInner sensitivityLabel() {
return this.sensitivityLabel;
}
/**
* Set the sensitivityLabel property: The sensitivity label information to apply on a column.
*
* @param sensitivityLabel the sensitivityLabel value to set.
* @return the SensitivityLabelUpdatePropertiesInner object itself.
*/
public SensitivityLabelUpdatePropertiesInner withSensitivityLabel(SensitivityLabelInner sensitivityLabel) {
this.sensitivityLabel = sensitivityLabel;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (op() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property op in model SensitivityLabelUpdatePropertiesInner"));
}
if (schema() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property schema in model SensitivityLabelUpdatePropertiesInner"));
}
if (table() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property table in model SensitivityLabelUpdatePropertiesInner"));
}
if (column() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property column in model SensitivityLabelUpdatePropertiesInner"));
}
if (sensitivityLabel() != null) {
sensitivityLabel().validate();
}
}
private static final ClientLogger LOGGER = new ClientLogger(SensitivityLabelUpdatePropertiesInner.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("op", this.op == null ? null : this.op.toString());
jsonWriter.writeStringField("schema", this.schema);
jsonWriter.writeStringField("table", this.table);
jsonWriter.writeStringField("column", this.column);
jsonWriter.writeJsonField("sensitivityLabel", this.sensitivityLabel);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of SensitivityLabelUpdatePropertiesInner from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of SensitivityLabelUpdatePropertiesInner if the JsonReader was pointing to an instance of it,
* or null if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the SensitivityLabelUpdatePropertiesInner.
*/
public static SensitivityLabelUpdatePropertiesInner fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
SensitivityLabelUpdatePropertiesInner deserializedSensitivityLabelUpdatePropertiesInner
= new SensitivityLabelUpdatePropertiesInner();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("op".equals(fieldName)) {
deserializedSensitivityLabelUpdatePropertiesInner.op
= SensitivityLabelUpdateKind.fromString(reader.getString());
} else if ("schema".equals(fieldName)) {
deserializedSensitivityLabelUpdatePropertiesInner.schema = reader.getString();
} else if ("table".equals(fieldName)) {
deserializedSensitivityLabelUpdatePropertiesInner.table = reader.getString();
} else if ("column".equals(fieldName)) {
deserializedSensitivityLabelUpdatePropertiesInner.column = reader.getString();
} else if ("sensitivityLabel".equals(fieldName)) {
deserializedSensitivityLabelUpdatePropertiesInner.sensitivityLabel
= SensitivityLabelInner.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedSensitivityLabelUpdatePropertiesInner;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy