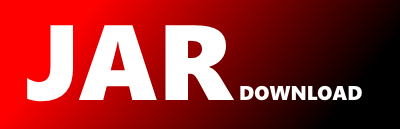
com.azure.resourcemanager.sql.fluent.models.WorkloadGroupProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-sql Show documentation
Show all versions of azure-resourcemanager-sql Show documentation
This package contains Microsoft Azure Sql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.sql.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Workload group definition. For more information look at sys.workload_management_workload_groups (DMV).
*/
@Fluent
public final class WorkloadGroupProperties implements JsonSerializable {
/*
* The workload group minimum percentage resource.
*/
private int minResourcePercent;
/*
* The workload group cap percentage resource.
*/
private int maxResourcePercent;
/*
* The workload group request minimum grant percentage.
*/
private double minResourcePercentPerRequest;
/*
* The workload group request maximum grant percentage.
*/
private Double maxResourcePercentPerRequest;
/*
* The workload group importance level.
*/
private String importance;
/*
* The workload group query execution timeout.
*/
private Integer queryExecutionTimeout;
/**
* Creates an instance of WorkloadGroupProperties class.
*/
public WorkloadGroupProperties() {
}
/**
* Get the minResourcePercent property: The workload group minimum percentage resource.
*
* @return the minResourcePercent value.
*/
public int minResourcePercent() {
return this.minResourcePercent;
}
/**
* Set the minResourcePercent property: The workload group minimum percentage resource.
*
* @param minResourcePercent the minResourcePercent value to set.
* @return the WorkloadGroupProperties object itself.
*/
public WorkloadGroupProperties withMinResourcePercent(int minResourcePercent) {
this.minResourcePercent = minResourcePercent;
return this;
}
/**
* Get the maxResourcePercent property: The workload group cap percentage resource.
*
* @return the maxResourcePercent value.
*/
public int maxResourcePercent() {
return this.maxResourcePercent;
}
/**
* Set the maxResourcePercent property: The workload group cap percentage resource.
*
* @param maxResourcePercent the maxResourcePercent value to set.
* @return the WorkloadGroupProperties object itself.
*/
public WorkloadGroupProperties withMaxResourcePercent(int maxResourcePercent) {
this.maxResourcePercent = maxResourcePercent;
return this;
}
/**
* Get the minResourcePercentPerRequest property: The workload group request minimum grant percentage.
*
* @return the minResourcePercentPerRequest value.
*/
public double minResourcePercentPerRequest() {
return this.minResourcePercentPerRequest;
}
/**
* Set the minResourcePercentPerRequest property: The workload group request minimum grant percentage.
*
* @param minResourcePercentPerRequest the minResourcePercentPerRequest value to set.
* @return the WorkloadGroupProperties object itself.
*/
public WorkloadGroupProperties withMinResourcePercentPerRequest(double minResourcePercentPerRequest) {
this.minResourcePercentPerRequest = minResourcePercentPerRequest;
return this;
}
/**
* Get the maxResourcePercentPerRequest property: The workload group request maximum grant percentage.
*
* @return the maxResourcePercentPerRequest value.
*/
public Double maxResourcePercentPerRequest() {
return this.maxResourcePercentPerRequest;
}
/**
* Set the maxResourcePercentPerRequest property: The workload group request maximum grant percentage.
*
* @param maxResourcePercentPerRequest the maxResourcePercentPerRequest value to set.
* @return the WorkloadGroupProperties object itself.
*/
public WorkloadGroupProperties withMaxResourcePercentPerRequest(Double maxResourcePercentPerRequest) {
this.maxResourcePercentPerRequest = maxResourcePercentPerRequest;
return this;
}
/**
* Get the importance property: The workload group importance level.
*
* @return the importance value.
*/
public String importance() {
return this.importance;
}
/**
* Set the importance property: The workload group importance level.
*
* @param importance the importance value to set.
* @return the WorkloadGroupProperties object itself.
*/
public WorkloadGroupProperties withImportance(String importance) {
this.importance = importance;
return this;
}
/**
* Get the queryExecutionTimeout property: The workload group query execution timeout.
*
* @return the queryExecutionTimeout value.
*/
public Integer queryExecutionTimeout() {
return this.queryExecutionTimeout;
}
/**
* Set the queryExecutionTimeout property: The workload group query execution timeout.
*
* @param queryExecutionTimeout the queryExecutionTimeout value to set.
* @return the WorkloadGroupProperties object itself.
*/
public WorkloadGroupProperties withQueryExecutionTimeout(Integer queryExecutionTimeout) {
this.queryExecutionTimeout = queryExecutionTimeout;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeIntField("minResourcePercent", this.minResourcePercent);
jsonWriter.writeIntField("maxResourcePercent", this.maxResourcePercent);
jsonWriter.writeDoubleField("minResourcePercentPerRequest", this.minResourcePercentPerRequest);
jsonWriter.writeNumberField("maxResourcePercentPerRequest", this.maxResourcePercentPerRequest);
jsonWriter.writeStringField("importance", this.importance);
jsonWriter.writeNumberField("queryExecutionTimeout", this.queryExecutionTimeout);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of WorkloadGroupProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of WorkloadGroupProperties if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the WorkloadGroupProperties.
*/
public static WorkloadGroupProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
WorkloadGroupProperties deserializedWorkloadGroupProperties = new WorkloadGroupProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("minResourcePercent".equals(fieldName)) {
deserializedWorkloadGroupProperties.minResourcePercent = reader.getInt();
} else if ("maxResourcePercent".equals(fieldName)) {
deserializedWorkloadGroupProperties.maxResourcePercent = reader.getInt();
} else if ("minResourcePercentPerRequest".equals(fieldName)) {
deserializedWorkloadGroupProperties.minResourcePercentPerRequest = reader.getDouble();
} else if ("maxResourcePercentPerRequest".equals(fieldName)) {
deserializedWorkloadGroupProperties.maxResourcePercentPerRequest
= reader.getNullable(JsonReader::getDouble);
} else if ("importance".equals(fieldName)) {
deserializedWorkloadGroupProperties.importance = reader.getString();
} else if ("queryExecutionTimeout".equals(fieldName)) {
deserializedWorkloadGroupProperties.queryExecutionTimeout = reader.getNullable(JsonReader::getInt);
} else {
reader.skipChildren();
}
}
return deserializedWorkloadGroupProperties;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy