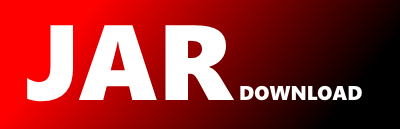
com.azure.resourcemanager.sql.implementation.DatabaseColumnsClientImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-sql Show documentation
Show all versions of azure-resourcemanager-sql Show documentation
This package contains Microsoft Azure Sql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.sql.implementation;
import com.azure.core.annotation.ExpectedResponses;
import com.azure.core.annotation.Get;
import com.azure.core.annotation.HeaderParam;
import com.azure.core.annotation.Headers;
import com.azure.core.annotation.Host;
import com.azure.core.annotation.HostParam;
import com.azure.core.annotation.PathParam;
import com.azure.core.annotation.QueryParam;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceInterface;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.annotation.UnexpectedResponseExceptionType;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.PagedResponse;
import com.azure.core.http.rest.PagedResponseBase;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.RestProxy;
import com.azure.core.management.exception.ManagementException;
import com.azure.core.util.Context;
import com.azure.core.util.FluxUtil;
import com.azure.resourcemanager.sql.fluent.DatabaseColumnsClient;
import com.azure.resourcemanager.sql.fluent.models.DatabaseColumnInner;
import com.azure.resourcemanager.sql.models.DatabaseColumnListResult;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.stream.Collectors;
import reactor.core.publisher.Mono;
/**
* An instance of this class provides access to all the operations defined in DatabaseColumnsClient.
*/
public final class DatabaseColumnsClientImpl implements DatabaseColumnsClient {
/**
* The proxy service used to perform REST calls.
*/
private final DatabaseColumnsService service;
/**
* The service client containing this operation class.
*/
private final SqlManagementClientImpl client;
/**
* Initializes an instance of DatabaseColumnsClientImpl.
*
* @param client the instance of the service client containing this operation class.
*/
DatabaseColumnsClientImpl(SqlManagementClientImpl client) {
this.service
= RestProxy.create(DatabaseColumnsService.class, client.getHttpPipeline(), client.getSerializerAdapter());
this.client = client;
}
/**
* The interface defining all the services for SqlManagementClientDatabaseColumns to be used by the proxy service to
* perform REST calls.
*/
@Host("{$host}")
@ServiceInterface(name = "SqlManagementClientD")
public interface DatabaseColumnsService {
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}/columns")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listByDatabase(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("serverName") String serverName,
@PathParam("databaseName") String databaseName,
@QueryParam(value = "schema", multipleQueryParams = true) List schema,
@QueryParam(value = "table", multipleQueryParams = true) List table,
@QueryParam(value = "column", multipleQueryParams = true) List column,
@QueryParam(value = "orderBy", multipleQueryParams = true) List orderBy,
@QueryParam("$skiptoken") String skiptoken, @PathParam("subscriptionId") String subscriptionId,
@QueryParam("api-version") String apiVersion, @HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}/schemas/{schemaName}/tables/{tableName}/columns")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listByTable(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("serverName") String serverName,
@PathParam("databaseName") String databaseName, @PathParam("schemaName") String schemaName,
@PathParam("tableName") String tableName, @QueryParam("$filter") String filter,
@PathParam("subscriptionId") String subscriptionId, @QueryParam("api-version") String apiVersion,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Sql/servers/{serverName}/databases/{databaseName}/schemas/{schemaName}/tables/{tableName}/columns/{columnName}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> get(@HostParam("$host") String endpoint,
@PathParam("resourceGroupName") String resourceGroupName, @PathParam("serverName") String serverName,
@PathParam("databaseName") String databaseName, @PathParam("schemaName") String schemaName,
@PathParam("tableName") String tableName, @PathParam("columnName") String columnName,
@PathParam("subscriptionId") String subscriptionId, @QueryParam("api-version") String apiVersion,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listByDatabaseNext(
@PathParam(value = "nextLink", encoded = true) String nextLink, @HostParam("$host") String endpoint,
@HeaderParam("Accept") String accept, Context context);
@Headers({ "Content-Type: application/json" })
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(ManagementException.class)
Mono> listByTableNext(
@PathParam(value = "nextLink", encoded = true) String nextLink, @HostParam("$host") String endpoint,
@HeaderParam("Accept") String accept, Context context);
}
/**
* List database columns.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @param schema The schema parameter.
* @param table The table parameter.
* @param column The column parameter.
* @param orderBy The orderBy parameter.
* @param skiptoken An opaque token that identifies a starting point in the collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of database columns along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByDatabaseSinglePageAsync(String resourceGroupName,
String serverName, String databaseName, List schema, List table, List column,
List orderBy, String skiptoken) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (serverName == null) {
return Mono.error(new IllegalArgumentException("Parameter serverName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String accept = "application/json";
List schemaConverted = (schema == null)
? new ArrayList<>()
: schema.stream().map(item -> Objects.toString(item, "")).collect(Collectors.toList());
List tableConverted = (table == null)
? new ArrayList<>()
: table.stream().map(item -> Objects.toString(item, "")).collect(Collectors.toList());
List columnConverted = (column == null)
? new ArrayList<>()
: column.stream().map(item -> Objects.toString(item, "")).collect(Collectors.toList());
List orderByConverted = (orderBy == null)
? new ArrayList<>()
: orderBy.stream().map(item -> Objects.toString(item, "")).collect(Collectors.toList());
return FluxUtil
.withContext(context -> service.listByDatabase(this.client.getEndpoint(), resourceGroupName, serverName,
databaseName, schemaConverted, tableConverted, columnConverted, orderByConverted, skiptoken,
this.client.getSubscriptionId(), this.client.getApiVersion(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* List database columns.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @param schema The schema parameter.
* @param table The table parameter.
* @param column The column parameter.
* @param orderBy The orderBy parameter.
* @param skiptoken An opaque token that identifies a starting point in the collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of database columns along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByDatabaseSinglePageAsync(String resourceGroupName,
String serverName, String databaseName, List schema, List table, List column,
List orderBy, String skiptoken, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (serverName == null) {
return Mono.error(new IllegalArgumentException("Parameter serverName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String accept = "application/json";
List schemaConverted = (schema == null)
? new ArrayList<>()
: schema.stream().map(item -> Objects.toString(item, "")).collect(Collectors.toList());
List tableConverted = (table == null)
? new ArrayList<>()
: table.stream().map(item -> Objects.toString(item, "")).collect(Collectors.toList());
List columnConverted = (column == null)
? new ArrayList<>()
: column.stream().map(item -> Objects.toString(item, "")).collect(Collectors.toList());
List orderByConverted = (orderBy == null)
? new ArrayList<>()
: orderBy.stream().map(item -> Objects.toString(item, "")).collect(Collectors.toList());
context = this.client.mergeContext(context);
return service
.listByDatabase(this.client.getEndpoint(), resourceGroupName, serverName, databaseName, schemaConverted,
tableConverted, columnConverted, orderByConverted, skiptoken, this.client.getSubscriptionId(),
this.client.getApiVersion(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* List database columns.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @param schema The schema parameter.
* @param table The table parameter.
* @param column The column parameter.
* @param orderBy The orderBy parameter.
* @param skiptoken An opaque token that identifies a starting point in the collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of database columns as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listByDatabaseAsync(String resourceGroupName, String serverName,
String databaseName, List schema, List table, List column, List orderBy,
String skiptoken) {
return new PagedFlux<>(() -> listByDatabaseSinglePageAsync(resourceGroupName, serverName, databaseName, schema,
table, column, orderBy, skiptoken), nextLink -> listByDatabaseNextSinglePageAsync(nextLink));
}
/**
* List database columns.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of database columns as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listByDatabaseAsync(String resourceGroupName, String serverName,
String databaseName) {
final List schema = null;
final List table = null;
final List column = null;
final List orderBy = null;
final String skiptoken = null;
return new PagedFlux<>(() -> listByDatabaseSinglePageAsync(resourceGroupName, serverName, databaseName, schema,
table, column, orderBy, skiptoken), nextLink -> listByDatabaseNextSinglePageAsync(nextLink));
}
/**
* List database columns.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @param schema The schema parameter.
* @param table The table parameter.
* @param column The column parameter.
* @param orderBy The orderBy parameter.
* @param skiptoken An opaque token that identifies a starting point in the collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of database columns as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listByDatabaseAsync(String resourceGroupName, String serverName,
String databaseName, List schema, List table, List column, List orderBy,
String skiptoken, Context context) {
return new PagedFlux<>(() -> listByDatabaseSinglePageAsync(resourceGroupName, serverName, databaseName, schema,
table, column, orderBy, skiptoken, context),
nextLink -> listByDatabaseNextSinglePageAsync(nextLink, context));
}
/**
* List database columns.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of database columns as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listByDatabase(String resourceGroupName, String serverName,
String databaseName) {
final List schema = null;
final List table = null;
final List column = null;
final List orderBy = null;
final String skiptoken = null;
return new PagedIterable<>(listByDatabaseAsync(resourceGroupName, serverName, databaseName, schema, table,
column, orderBy, skiptoken));
}
/**
* List database columns.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @param schema The schema parameter.
* @param table The table parameter.
* @param column The column parameter.
* @param orderBy The orderBy parameter.
* @param skiptoken An opaque token that identifies a starting point in the collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of database columns as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listByDatabase(String resourceGroupName, String serverName,
String databaseName, List schema, List table, List column, List orderBy,
String skiptoken, Context context) {
return new PagedIterable<>(listByDatabaseAsync(resourceGroupName, serverName, databaseName, schema, table,
column, orderBy, skiptoken, context));
}
/**
* List database columns.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @param schemaName The name of the schema.
* @param tableName The name of the table.
* @param filter An OData filter expression that filters elements in the collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of database columns along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByTableSinglePageAsync(String resourceGroupName,
String serverName, String databaseName, String schemaName, String tableName, String filter) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (serverName == null) {
return Mono.error(new IllegalArgumentException("Parameter serverName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (schemaName == null) {
return Mono.error(new IllegalArgumentException("Parameter schemaName is required and cannot be null."));
}
if (tableName == null) {
return Mono.error(new IllegalArgumentException("Parameter tableName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.listByTable(this.client.getEndpoint(), resourceGroupName, serverName,
databaseName, schemaName, tableName, filter, this.client.getSubscriptionId(),
this.client.getApiVersion(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* List database columns.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @param schemaName The name of the schema.
* @param tableName The name of the table.
* @param filter An OData filter expression that filters elements in the collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of database columns along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByTableSinglePageAsync(String resourceGroupName,
String serverName, String databaseName, String schemaName, String tableName, String filter, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (serverName == null) {
return Mono.error(new IllegalArgumentException("Parameter serverName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (schemaName == null) {
return Mono.error(new IllegalArgumentException("Parameter schemaName is required and cannot be null."));
}
if (tableName == null) {
return Mono.error(new IllegalArgumentException("Parameter tableName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service
.listByTable(this.client.getEndpoint(), resourceGroupName, serverName, databaseName, schemaName, tableName,
filter, this.client.getSubscriptionId(), this.client.getApiVersion(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* List database columns.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @param schemaName The name of the schema.
* @param tableName The name of the table.
* @param filter An OData filter expression that filters elements in the collection.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of database columns as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listByTableAsync(String resourceGroupName, String serverName,
String databaseName, String schemaName, String tableName, String filter) {
return new PagedFlux<>(() -> listByTableSinglePageAsync(resourceGroupName, serverName, databaseName, schemaName,
tableName, filter), nextLink -> listByTableNextSinglePageAsync(nextLink));
}
/**
* List database columns.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @param schemaName The name of the schema.
* @param tableName The name of the table.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of database columns as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listByTableAsync(String resourceGroupName, String serverName,
String databaseName, String schemaName, String tableName) {
final String filter = null;
return new PagedFlux<>(() -> listByTableSinglePageAsync(resourceGroupName, serverName, databaseName, schemaName,
tableName, filter), nextLink -> listByTableNextSinglePageAsync(nextLink));
}
/**
* List database columns.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @param schemaName The name of the schema.
* @param tableName The name of the table.
* @param filter An OData filter expression that filters elements in the collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of database columns as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
private PagedFlux listByTableAsync(String resourceGroupName, String serverName,
String databaseName, String schemaName, String tableName, String filter, Context context) {
return new PagedFlux<>(() -> listByTableSinglePageAsync(resourceGroupName, serverName, databaseName, schemaName,
tableName, filter, context), nextLink -> listByTableNextSinglePageAsync(nextLink, context));
}
/**
* List database columns.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @param schemaName The name of the schema.
* @param tableName The name of the table.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of database columns as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listByTable(String resourceGroupName, String serverName,
String databaseName, String schemaName, String tableName) {
final String filter = null;
return new PagedIterable<>(
listByTableAsync(resourceGroupName, serverName, databaseName, schemaName, tableName, filter));
}
/**
* List database columns.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @param schemaName The name of the schema.
* @param tableName The name of the table.
* @param filter An OData filter expression that filters elements in the collection.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of database columns as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listByTable(String resourceGroupName, String serverName,
String databaseName, String schemaName, String tableName, String filter, Context context) {
return new PagedIterable<>(
listByTableAsync(resourceGroupName, serverName, databaseName, schemaName, tableName, filter, context));
}
/**
* Get database column.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @param schemaName The name of the schema.
* @param tableName The name of the table.
* @param columnName The name of the column.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return database column along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getWithResponseAsync(String resourceGroupName, String serverName,
String databaseName, String schemaName, String tableName, String columnName) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (serverName == null) {
return Mono.error(new IllegalArgumentException("Parameter serverName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (schemaName == null) {
return Mono.error(new IllegalArgumentException("Parameter schemaName is required and cannot be null."));
}
if (tableName == null) {
return Mono.error(new IllegalArgumentException("Parameter tableName is required and cannot be null."));
}
if (columnName == null) {
return Mono.error(new IllegalArgumentException("Parameter columnName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.get(this.client.getEndpoint(), resourceGroupName, serverName, databaseName,
schemaName, tableName, columnName, this.client.getSubscriptionId(), this.client.getApiVersion(), accept,
context))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Get database column.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @param schemaName The name of the schema.
* @param tableName The name of the table.
* @param columnName The name of the column.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return database column along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> getWithResponseAsync(String resourceGroupName, String serverName,
String databaseName, String schemaName, String tableName, String columnName, Context context) {
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
if (resourceGroupName == null) {
return Mono
.error(new IllegalArgumentException("Parameter resourceGroupName is required and cannot be null."));
}
if (serverName == null) {
return Mono.error(new IllegalArgumentException("Parameter serverName is required and cannot be null."));
}
if (databaseName == null) {
return Mono.error(new IllegalArgumentException("Parameter databaseName is required and cannot be null."));
}
if (schemaName == null) {
return Mono.error(new IllegalArgumentException("Parameter schemaName is required and cannot be null."));
}
if (tableName == null) {
return Mono.error(new IllegalArgumentException("Parameter tableName is required and cannot be null."));
}
if (columnName == null) {
return Mono.error(new IllegalArgumentException("Parameter columnName is required and cannot be null."));
}
if (this.client.getSubscriptionId() == null) {
return Mono.error(new IllegalArgumentException(
"Parameter this.client.getSubscriptionId() is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.get(this.client.getEndpoint(), resourceGroupName, serverName, databaseName, schemaName,
tableName, columnName, this.client.getSubscriptionId(), this.client.getApiVersion(), accept, context);
}
/**
* Get database column.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @param schemaName The name of the schema.
* @param tableName The name of the table.
* @param columnName The name of the column.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return database column on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getAsync(String resourceGroupName, String serverName, String databaseName,
String schemaName, String tableName, String columnName) {
return getWithResponseAsync(resourceGroupName, serverName, databaseName, schemaName, tableName, columnName)
.flatMap(res -> Mono.justOrEmpty(res.getValue()));
}
/**
* Get database column.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @param schemaName The name of the schema.
* @param tableName The name of the table.
* @param columnName The name of the column.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return database column along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getWithResponse(String resourceGroupName, String serverName,
String databaseName, String schemaName, String tableName, String columnName, Context context) {
return getWithResponseAsync(resourceGroupName, serverName, databaseName, schemaName, tableName, columnName,
context).block();
}
/**
* Get database column.
*
* @param resourceGroupName The name of the resource group that contains the resource. You can obtain this value
* from the Azure Resource Manager API or the portal.
* @param serverName The name of the server.
* @param databaseName The name of the database.
* @param schemaName The name of the schema.
* @param tableName The name of the table.
* @param columnName The name of the column.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return database column.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public DatabaseColumnInner get(String resourceGroupName, String serverName, String databaseName, String schemaName,
String tableName, String columnName) {
return getWithResponse(resourceGroupName, serverName, databaseName, schemaName, tableName, columnName,
Context.NONE).getValue();
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of database columns along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByDatabaseNextSinglePageAsync(String nextLink) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.listByDatabaseNext(nextLink, this.client.getEndpoint(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of database columns along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByDatabaseNextSinglePageAsync(String nextLink,
Context context) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.listByDatabaseNext(nextLink, this.client.getEndpoint(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of database columns along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByTableNextSinglePageAsync(String nextLink) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.listByTableNext(nextLink, this.client.getEndpoint(), accept, context))
.>map(res -> new PagedResponseBase<>(res.getRequest(),
res.getStatusCode(), res.getHeaders(), res.getValue().value(), res.getValue().nextLink(), null))
.contextWrite(context -> context.putAll(FluxUtil.toReactorContext(this.client.getContext()).readOnly()));
}
/**
* Get the next page of items.
*
* @param nextLink The URL to get the next list of items.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a list of database columns along with {@link PagedResponse} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listByTableNextSinglePageAsync(String nextLink, Context context) {
if (nextLink == null) {
return Mono.error(new IllegalArgumentException("Parameter nextLink is required and cannot be null."));
}
if (this.client.getEndpoint() == null) {
return Mono.error(
new IllegalArgumentException("Parameter this.client.getEndpoint() is required and cannot be null."));
}
final String accept = "application/json";
context = this.client.mergeContext(context);
return service.listByTableNext(nextLink, this.client.getEndpoint(), accept, context)
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().value(), res.getValue().nextLink(), null));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy