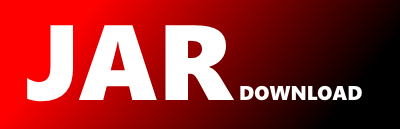
com.azure.resourcemanager.sql.implementation.SqlServerAutomaticTuningImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-sql Show documentation
Show all versions of azure-resourcemanager-sql Show documentation
This package contains Microsoft Azure Sql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.resourcemanager.sql.implementation;
import com.azure.core.util.Context;
import com.azure.core.util.FluxUtil;
import com.azure.resourcemanager.resources.fluentcore.model.implementation.RefreshableWrapperImpl;
import com.azure.resourcemanager.sql.SqlServerManager;
import com.azure.resourcemanager.sql.models.AutomaticTuningOptionModeDesired;
import com.azure.resourcemanager.sql.models.AutomaticTuningServerMode;
import com.azure.resourcemanager.sql.models.AutomaticTuningServerOptions;
import com.azure.resourcemanager.sql.models.SqlServerAutomaticTuning;
import com.azure.resourcemanager.sql.fluent.models.ServerAutomaticTuningInner;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.UUID;
import reactor.core.publisher.Mono;
/** Implementation for Azure SQL server automatic tuning. */
public class SqlServerAutomaticTuningImpl
extends RefreshableWrapperImpl
implements SqlServerAutomaticTuning, SqlServerAutomaticTuning.Update {
protected String key;
private SqlServerManager sqlServerManager;
private String resourceGroupName;
private String sqlServerName;
SqlServerAutomaticTuningImpl(SqlServerImpl server, ServerAutomaticTuningInner innerObject) {
this(server.resourceGroupName(), server.name(), innerObject, server.manager());
}
SqlServerAutomaticTuningImpl(
String resourceGroupName,
String sqlServerName,
ServerAutomaticTuningInner innerObject,
SqlServerManager sqlServerManager) {
super(innerObject);
Objects.requireNonNull(innerObject);
Objects.requireNonNull(sqlServerManager);
this.sqlServerManager = sqlServerManager;
this.resourceGroupName = resourceGroupName;
this.sqlServerName = sqlServerName;
this.key = UUID.randomUUID().toString();
}
@Override
public String key() {
return this.key;
}
@Override
public AutomaticTuningServerMode desiredState() {
return this.innerModel().desiredState();
}
@Override
public AutomaticTuningServerMode actualState() {
return this.innerModel().actualState();
}
@Override
public Map tuningOptions() {
return Collections
.unmodifiableMap(
this.innerModel().options() != null
? this.innerModel().options()
: new HashMap());
}
@Override
public SqlServerAutomaticTuningImpl withAutomaticTuningMode(AutomaticTuningServerMode desiredState) {
this.innerModel().withDesiredState(desiredState);
return this;
}
@Override
public SqlServerAutomaticTuningImpl withAutomaticTuningOption(
String tuningOptionName, AutomaticTuningOptionModeDesired desiredState) {
if (this.innerModel().options() == null) {
this.innerModel().withOptions(new HashMap());
}
AutomaticTuningServerOptions item = this.innerModel().options().get(tuningOptionName);
this
.innerModel()
.options()
.put(
tuningOptionName,
item != null
? item.withDesiredState(desiredState)
: new AutomaticTuningServerOptions().withDesiredState(desiredState));
return this;
}
@Override
public SqlServerAutomaticTuningImpl withAutomaticTuningOptions(
Map tuningOptions) {
if (tuningOptions != null) {
for (Map.Entry option : tuningOptions.entrySet()) {
this.withAutomaticTuningOption(option.getKey(), option.getValue());
}
}
return this;
}
@Override
protected Mono getInnerAsync() {
return this
.sqlServerManager
.serviceClient()
.getServerAutomaticTunings()
.getAsync(this.resourceGroupName, this.sqlServerName);
}
@Override
public SqlServerAutomaticTuning apply() {
return this.applyAsync().block();
}
@Override
public Mono applyAsync() {
return applyAsync(Context.NONE);
}
@Override
public SqlServerAutomaticTuning apply(Context context) {
return applyAsync(context).block();
}
@Override
public Mono applyAsync(Context context) {
final SqlServerAutomaticTuningImpl self = this;
return this
.sqlServerManager
.serviceClient()
.getServerAutomaticTunings()
.updateAsync(this.resourceGroupName, this.sqlServerName, this.innerModel())
.contextWrite(c -> c.putAll(FluxUtil.toReactorContext(context).readOnly()))
.map(
serverAutomaticTuningInner -> {
self.setInner(serverAutomaticTuningInner);
return self;
});
}
@Override
public Update update() {
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy