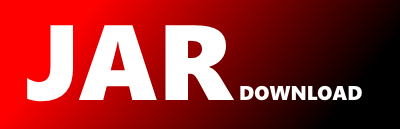
com.azure.resourcemanager.sql.models.JobTarget Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-sql Show documentation
Show all versions of azure-resourcemanager-sql Show documentation
This package contains Microsoft Azure Sql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.sql.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* A job target, for example a specific database or a container of databases that is evaluated during job execution.
*/
@Fluent
public final class JobTarget implements JsonSerializable {
/*
* Whether the target is included or excluded from the group.
*/
private JobTargetGroupMembershipType membershipType;
/*
* The target type.
*/
private JobTargetType type;
/*
* The target server name.
*/
private String serverName;
/*
* The target database name.
*/
private String databaseName;
/*
* The target elastic pool name.
*/
private String elasticPoolName;
/*
* The target shard map.
*/
private String shardMapName;
/*
* The resource ID of the credential that is used during job execution to connect to the target and determine the
* list of databases inside the target.
*/
private String refreshCredential;
/**
* Creates an instance of JobTarget class.
*/
public JobTarget() {
}
/**
* Get the membershipType property: Whether the target is included or excluded from the group.
*
* @return the membershipType value.
*/
public JobTargetGroupMembershipType membershipType() {
return this.membershipType;
}
/**
* Set the membershipType property: Whether the target is included or excluded from the group.
*
* @param membershipType the membershipType value to set.
* @return the JobTarget object itself.
*/
public JobTarget withMembershipType(JobTargetGroupMembershipType membershipType) {
this.membershipType = membershipType;
return this;
}
/**
* Get the type property: The target type.
*
* @return the type value.
*/
public JobTargetType type() {
return this.type;
}
/**
* Set the type property: The target type.
*
* @param type the type value to set.
* @return the JobTarget object itself.
*/
public JobTarget withType(JobTargetType type) {
this.type = type;
return this;
}
/**
* Get the serverName property: The target server name.
*
* @return the serverName value.
*/
public String serverName() {
return this.serverName;
}
/**
* Set the serverName property: The target server name.
*
* @param serverName the serverName value to set.
* @return the JobTarget object itself.
*/
public JobTarget withServerName(String serverName) {
this.serverName = serverName;
return this;
}
/**
* Get the databaseName property: The target database name.
*
* @return the databaseName value.
*/
public String databaseName() {
return this.databaseName;
}
/**
* Set the databaseName property: The target database name.
*
* @param databaseName the databaseName value to set.
* @return the JobTarget object itself.
*/
public JobTarget withDatabaseName(String databaseName) {
this.databaseName = databaseName;
return this;
}
/**
* Get the elasticPoolName property: The target elastic pool name.
*
* @return the elasticPoolName value.
*/
public String elasticPoolName() {
return this.elasticPoolName;
}
/**
* Set the elasticPoolName property: The target elastic pool name.
*
* @param elasticPoolName the elasticPoolName value to set.
* @return the JobTarget object itself.
*/
public JobTarget withElasticPoolName(String elasticPoolName) {
this.elasticPoolName = elasticPoolName;
return this;
}
/**
* Get the shardMapName property: The target shard map.
*
* @return the shardMapName value.
*/
public String shardMapName() {
return this.shardMapName;
}
/**
* Set the shardMapName property: The target shard map.
*
* @param shardMapName the shardMapName value to set.
* @return the JobTarget object itself.
*/
public JobTarget withShardMapName(String shardMapName) {
this.shardMapName = shardMapName;
return this;
}
/**
* Get the refreshCredential property: The resource ID of the credential that is used during job execution to
* connect to the target and determine the list of databases inside the target.
*
* @return the refreshCredential value.
*/
public String refreshCredential() {
return this.refreshCredential;
}
/**
* Set the refreshCredential property: The resource ID of the credential that is used during job execution to
* connect to the target and determine the list of databases inside the target.
*
* @param refreshCredential the refreshCredential value to set.
* @return the JobTarget object itself.
*/
public JobTarget withRefreshCredential(String refreshCredential) {
this.refreshCredential = refreshCredential;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (type() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException("Missing required property type in model JobTarget"));
}
}
private static final ClientLogger LOGGER = new ClientLogger(JobTarget.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("type", this.type == null ? null : this.type.toString());
jsonWriter.writeStringField("membershipType",
this.membershipType == null ? null : this.membershipType.toString());
jsonWriter.writeStringField("serverName", this.serverName);
jsonWriter.writeStringField("databaseName", this.databaseName);
jsonWriter.writeStringField("elasticPoolName", this.elasticPoolName);
jsonWriter.writeStringField("shardMapName", this.shardMapName);
jsonWriter.writeStringField("refreshCredential", this.refreshCredential);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of JobTarget from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of JobTarget if the JsonReader was pointing to an instance of it, or null if it was pointing
* to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the JobTarget.
*/
public static JobTarget fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
JobTarget deserializedJobTarget = new JobTarget();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("type".equals(fieldName)) {
deserializedJobTarget.type = JobTargetType.fromString(reader.getString());
} else if ("membershipType".equals(fieldName)) {
deserializedJobTarget.membershipType = JobTargetGroupMembershipType.fromString(reader.getString());
} else if ("serverName".equals(fieldName)) {
deserializedJobTarget.serverName = reader.getString();
} else if ("databaseName".equals(fieldName)) {
deserializedJobTarget.databaseName = reader.getString();
} else if ("elasticPoolName".equals(fieldName)) {
deserializedJobTarget.elasticPoolName = reader.getString();
} else if ("shardMapName".equals(fieldName)) {
deserializedJobTarget.shardMapName = reader.getString();
} else if ("refreshCredential".equals(fieldName)) {
deserializedJobTarget.refreshCredential = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedJobTarget;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy