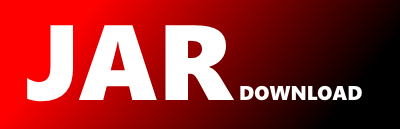
com.azure.resourcemanager.sql.models.ManagedInstanceExternalAdministrator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-sql Show documentation
Show all versions of azure-resourcemanager-sql Show documentation
This package contains Microsoft Azure Sql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.sql.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.Objects;
import java.util.UUID;
/**
* Properties of a active directory administrator.
*/
@Fluent
public final class ManagedInstanceExternalAdministrator
implements JsonSerializable {
/*
* Type of the sever administrator.
*/
private AdministratorType administratorType;
/*
* Principal Type of the sever administrator.
*/
private PrincipalType principalType;
/*
* Login name of the server administrator.
*/
private String login;
/*
* SID (object ID) of the server administrator.
*/
private UUID sid;
/*
* Tenant ID of the administrator.
*/
private UUID tenantId;
/*
* Azure Active Directory only Authentication enabled.
*/
private Boolean azureADOnlyAuthentication;
/**
* Creates an instance of ManagedInstanceExternalAdministrator class.
*/
public ManagedInstanceExternalAdministrator() {
}
/**
* Get the administratorType property: Type of the sever administrator.
*
* @return the administratorType value.
*/
public AdministratorType administratorType() {
return this.administratorType;
}
/**
* Set the administratorType property: Type of the sever administrator.
*
* @param administratorType the administratorType value to set.
* @return the ManagedInstanceExternalAdministrator object itself.
*/
public ManagedInstanceExternalAdministrator withAdministratorType(AdministratorType administratorType) {
this.administratorType = administratorType;
return this;
}
/**
* Get the principalType property: Principal Type of the sever administrator.
*
* @return the principalType value.
*/
public PrincipalType principalType() {
return this.principalType;
}
/**
* Set the principalType property: Principal Type of the sever administrator.
*
* @param principalType the principalType value to set.
* @return the ManagedInstanceExternalAdministrator object itself.
*/
public ManagedInstanceExternalAdministrator withPrincipalType(PrincipalType principalType) {
this.principalType = principalType;
return this;
}
/**
* Get the login property: Login name of the server administrator.
*
* @return the login value.
*/
public String login() {
return this.login;
}
/**
* Set the login property: Login name of the server administrator.
*
* @param login the login value to set.
* @return the ManagedInstanceExternalAdministrator object itself.
*/
public ManagedInstanceExternalAdministrator withLogin(String login) {
this.login = login;
return this;
}
/**
* Get the sid property: SID (object ID) of the server administrator.
*
* @return the sid value.
*/
public UUID sid() {
return this.sid;
}
/**
* Set the sid property: SID (object ID) of the server administrator.
*
* @param sid the sid value to set.
* @return the ManagedInstanceExternalAdministrator object itself.
*/
public ManagedInstanceExternalAdministrator withSid(UUID sid) {
this.sid = sid;
return this;
}
/**
* Get the tenantId property: Tenant ID of the administrator.
*
* @return the tenantId value.
*/
public UUID tenantId() {
return this.tenantId;
}
/**
* Set the tenantId property: Tenant ID of the administrator.
*
* @param tenantId the tenantId value to set.
* @return the ManagedInstanceExternalAdministrator object itself.
*/
public ManagedInstanceExternalAdministrator withTenantId(UUID tenantId) {
this.tenantId = tenantId;
return this;
}
/**
* Get the azureADOnlyAuthentication property: Azure Active Directory only Authentication enabled.
*
* @return the azureADOnlyAuthentication value.
*/
public Boolean azureADOnlyAuthentication() {
return this.azureADOnlyAuthentication;
}
/**
* Set the azureADOnlyAuthentication property: Azure Active Directory only Authentication enabled.
*
* @param azureADOnlyAuthentication the azureADOnlyAuthentication value to set.
* @return the ManagedInstanceExternalAdministrator object itself.
*/
public ManagedInstanceExternalAdministrator withAzureADOnlyAuthentication(Boolean azureADOnlyAuthentication) {
this.azureADOnlyAuthentication = azureADOnlyAuthentication;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("administratorType",
this.administratorType == null ? null : this.administratorType.toString());
jsonWriter.writeStringField("principalType", this.principalType == null ? null : this.principalType.toString());
jsonWriter.writeStringField("login", this.login);
jsonWriter.writeStringField("sid", Objects.toString(this.sid, null));
jsonWriter.writeStringField("tenantId", Objects.toString(this.tenantId, null));
jsonWriter.writeBooleanField("azureADOnlyAuthentication", this.azureADOnlyAuthentication);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ManagedInstanceExternalAdministrator from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ManagedInstanceExternalAdministrator if the JsonReader was pointing to an instance of it,
* or null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the ManagedInstanceExternalAdministrator.
*/
public static ManagedInstanceExternalAdministrator fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ManagedInstanceExternalAdministrator deserializedManagedInstanceExternalAdministrator
= new ManagedInstanceExternalAdministrator();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("administratorType".equals(fieldName)) {
deserializedManagedInstanceExternalAdministrator.administratorType
= AdministratorType.fromString(reader.getString());
} else if ("principalType".equals(fieldName)) {
deserializedManagedInstanceExternalAdministrator.principalType
= PrincipalType.fromString(reader.getString());
} else if ("login".equals(fieldName)) {
deserializedManagedInstanceExternalAdministrator.login = reader.getString();
} else if ("sid".equals(fieldName)) {
deserializedManagedInstanceExternalAdministrator.sid
= reader.getNullable(nonNullReader -> UUID.fromString(nonNullReader.getString()));
} else if ("tenantId".equals(fieldName)) {
deserializedManagedInstanceExternalAdministrator.tenantId
= reader.getNullable(nonNullReader -> UUID.fromString(nonNullReader.getString()));
} else if ("azureADOnlyAuthentication".equals(fieldName)) {
deserializedManagedInstanceExternalAdministrator.azureADOnlyAuthentication
= reader.getNullable(JsonReader::getBoolean);
} else {
reader.skipChildren();
}
}
return deserializedManagedInstanceExternalAdministrator;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy