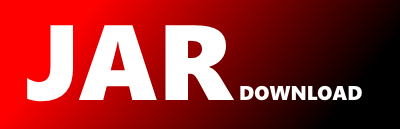
com.azure.resourcemanager.sql.models.RecommendedActionStateInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-sql Show documentation
Show all versions of azure-resourcemanager-sql Show documentation
This package contains Microsoft Azure Sql Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.sql.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.util.CoreUtils;
import com.azure.core.util.logging.ClientLogger;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
/**
* Contains information of current state for an Azure SQL Database, Server or Elastic Pool Recommended Action.
*/
@Fluent
public final class RecommendedActionStateInfo implements JsonSerializable {
/*
* Current state the recommended action is in. Some commonly used states are: Active -> recommended action is active
* and no action has been taken yet. Pending -> recommended action is approved for and is awaiting execution.
* Executing -> recommended action is being applied on the user database. Verifying -> recommended action was
* applied and is being verified of its usefulness by the system. Success -> recommended action was applied and
* improvement found during verification. Pending Revert -> verification found little or no improvement so
* recommended action is queued for revert or user has manually reverted. Reverting -> changes made while applying
* recommended action are being reverted on the user database. Reverted -> successfully reverted the changes made by
* recommended action on user database. Ignored -> user explicitly ignored/discarded the recommended action.
*/
private RecommendedActionCurrentState currentValue;
/*
* Gets who initiated the execution of this recommended action. Possible Value are: User -> When user explicity
* notified system to apply the recommended action. System -> When auto-execute status of this advisor was set to
* 'Enabled', in which case the system applied it.
*/
private RecommendedActionInitiatedBy actionInitiatedBy;
/*
* Gets the time when the state was last modified
*/
private OffsetDateTime lastModified;
/**
* Creates an instance of RecommendedActionStateInfo class.
*/
public RecommendedActionStateInfo() {
}
/**
* Get the currentValue property: Current state the recommended action is in. Some commonly used states are: Active
* -> recommended action is active and no action has been taken yet. Pending -> recommended action is approved
* for and is awaiting execution. Executing -> recommended action is being applied on the user database.
* Verifying -> recommended action was applied and is being verified of its usefulness by the system. Success
* -> recommended action was applied and improvement found during verification. Pending Revert -> verification
* found little or no improvement so recommended action is queued for revert or user has manually reverted.
* Reverting -> changes made while applying recommended action are being reverted on the user database. Reverted
* -> successfully reverted the changes made by recommended action on user database. Ignored -> user
* explicitly ignored/discarded the recommended action.
*
* @return the currentValue value.
*/
public RecommendedActionCurrentState currentValue() {
return this.currentValue;
}
/**
* Set the currentValue property: Current state the recommended action is in. Some commonly used states are: Active
* -> recommended action is active and no action has been taken yet. Pending -> recommended action is approved
* for and is awaiting execution. Executing -> recommended action is being applied on the user database.
* Verifying -> recommended action was applied and is being verified of its usefulness by the system. Success
* -> recommended action was applied and improvement found during verification. Pending Revert -> verification
* found little or no improvement so recommended action is queued for revert or user has manually reverted.
* Reverting -> changes made while applying recommended action are being reverted on the user database. Reverted
* -> successfully reverted the changes made by recommended action on user database. Ignored -> user
* explicitly ignored/discarded the recommended action.
*
* @param currentValue the currentValue value to set.
* @return the RecommendedActionStateInfo object itself.
*/
public RecommendedActionStateInfo withCurrentValue(RecommendedActionCurrentState currentValue) {
this.currentValue = currentValue;
return this;
}
/**
* Get the actionInitiatedBy property: Gets who initiated the execution of this recommended action. Possible Value
* are: User -> When user explicity notified system to apply the recommended action. System -> When
* auto-execute status of this advisor was set to 'Enabled', in which case the system applied it.
*
* @return the actionInitiatedBy value.
*/
public RecommendedActionInitiatedBy actionInitiatedBy() {
return this.actionInitiatedBy;
}
/**
* Get the lastModified property: Gets the time when the state was last modified.
*
* @return the lastModified value.
*/
public OffsetDateTime lastModified() {
return this.lastModified;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (currentValue() == null) {
throw LOGGER.atError()
.log(new IllegalArgumentException(
"Missing required property currentValue in model RecommendedActionStateInfo"));
}
}
private static final ClientLogger LOGGER = new ClientLogger(RecommendedActionStateInfo.class);
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("currentValue", this.currentValue == null ? null : this.currentValue.toString());
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of RecommendedActionStateInfo from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of RecommendedActionStateInfo if the JsonReader was pointing to an instance of it, or null if
* it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the RecommendedActionStateInfo.
*/
public static RecommendedActionStateInfo fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
RecommendedActionStateInfo deserializedRecommendedActionStateInfo = new RecommendedActionStateInfo();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("currentValue".equals(fieldName)) {
deserializedRecommendedActionStateInfo.currentValue
= RecommendedActionCurrentState.fromString(reader.getString());
} else if ("actionInitiatedBy".equals(fieldName)) {
deserializedRecommendedActionStateInfo.actionInitiatedBy
= RecommendedActionInitiatedBy.fromString(reader.getString());
} else if ("lastModified".equals(fieldName)) {
deserializedRecommendedActionStateInfo.lastModified = reader
.getNullable(nonNullReader -> CoreUtils.parseBestOffsetDateTime(nonNullReader.getString()));
} else {
reader.skipChildren();
}
}
return deserializedRecommendedActionStateInfo;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy