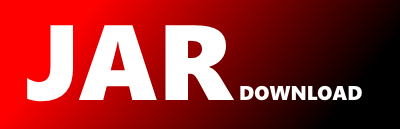
com.azure.resourcemanager.sql.models.SqlDatabase Maven / Gradle / Ivy
Show all versions of azure-resourcemanager-sql Show documentation
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.resourcemanager.sql.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.management.Region;
import com.azure.resourcemanager.resources.fluentcore.arm.models.ExternalChildResource;
import com.azure.resourcemanager.resources.fluentcore.arm.models.HasResourceGroup;
import com.azure.resourcemanager.resources.fluentcore.arm.models.Resource;
import com.azure.resourcemanager.resources.fluentcore.model.Appliable;
import com.azure.resourcemanager.resources.fluentcore.model.Attachable;
import com.azure.resourcemanager.resources.fluentcore.model.Creatable;
import com.azure.resourcemanager.resources.fluentcore.model.HasInnerModel;
import com.azure.resourcemanager.resources.fluentcore.model.Refreshable;
import com.azure.resourcemanager.resources.fluentcore.model.Updatable;
import com.azure.resourcemanager.sql.fluent.models.DatabaseInner;
import com.azure.resourcemanager.storage.models.StorageAccount;
import reactor.core.publisher.Mono;
import java.time.OffsetDateTime;
import java.util.List;
import java.util.Map;
/** An immutable client-side representation of an Azure SQL Server Database. */
@Fluent
public interface SqlDatabase
extends ExternalChildResource,
HasInnerModel,
HasResourceGroup,
Refreshable,
Updatable {
/** @return name of the SQL Server to which this database belongs */
String sqlServerName();
/** @return the collation of the Azure SQL Database */
String collation();
/** @return the creation date of the Azure SQL Database */
OffsetDateTime creationDate();
/**
* @return the current Service Level Objective Name of the Azure SQL Database, this is the Name of the Service Level
* Objective that is currently active
*/
String currentServiceObjectiveName();
/** @return the Id of the Azure SQL Database */
String databaseId();
/**
* @return the recovery period start date of the Azure SQL Database. This records the start date and time when
* recovery is available for this Azure SQL Database.
*/
OffsetDateTime earliestRestoreDate();
/** @return the edition of the Azure SQL Database */
DatabaseEdition edition();
/** @return the max size of the Azure SQL Database expressed in bytes. */
long maxSizeBytes();
/**
* @return the name of the configured Service Level Objective of the Azure SQL Database, this is the Service Level
* Objective that is being applied to the Azure SQL Database
*/
String requestedServiceObjectiveName();
/** @return the status of the Azure SQL Database */
DatabaseStatus status();
/** @return the elasticPoolId value */
String elasticPoolId();
/** @return the elasticPoolName value */
String elasticPoolName();
/** @return the defaultSecondaryLocation value */
String defaultSecondaryLocation();
/** @return the parent SQL server ID */
String parentId();
/** @return the name of the region the resource is in */
String regionName();
/** @return the region the resource is in */
Region region();
/** @return true if this Database is SqlWarehouse */
boolean isDataWarehouse();
/** @return SqlWarehouse instance for more operations */
SqlWarehouse asWarehouse();
/** @return the list of all restore points on this database */
List listRestorePoints();
/** @return the list of all restore points on this database */
PagedFlux listRestorePointsAsync();
/**
* Gets an Azure SQL Database Transparent Data Encryption for this database.
*
* @return an Azure SQL Database Transparent Data Encryption for this database
*/
TransparentDataEncryption getTransparentDataEncryption();
/**
* Gets an Azure SQL Database Transparent Data Encryption for this database.
*
* @return a representation of the deferred computation of an Azure SQL Database Transparent Data Encryption for
* this database
*/
Mono getTransparentDataEncryptionAsync();
/** @return all the replication links associated with this database */
Map listReplicationLinks();
/**
* @return a representation of the deferred computation of all the replication links associated with this database
*/
PagedFlux listReplicationLinksAsync();
/**
* Exports the current database to a specified URI path.
*
* @param storageUri the storage URI to use
* @return response object
*/
SqlDatabaseExportRequest.DefinitionStages.WithStorageTypeAndKey exportTo(String storageUri);
/**
* Exports the current database to an existing storage account and relative path.
*
* @param storageAccount an existing storage account to be used
* @param containerName the container name within the storage account to use
* @param fileName the exported database file name
* @return response object
*/
SqlDatabaseExportRequest.DefinitionStages.WithAuthenticationTypeAndLoginPassword exportTo(
StorageAccount storageAccount, String containerName, String fileName);
/**
* Exports the current database to a new storage account and relative path.
*
* @param storageAccountCreatable a storage account to be created as part of this execution flow
* @param containerName the container name within the storage account to use
* @param fileName the exported database file name
* @return response object
*/
SqlDatabaseExportRequest.DefinitionStages.WithAuthenticationTypeAndLoginPassword exportTo(
Creatable storageAccountCreatable, String containerName, String fileName);
/**
* Imports into the current database from a specified URI path; the current database must be empty.
*
* @param storageUri the storage URI to use
* @return response object
*/
SqlDatabaseImportRequest.DefinitionStages.WithStorageTypeAndKey importBacpac(String storageUri);
/**
* Imports into the current database from an existing storage account and relative path; the current database must
* be empty.
*
* @param storageAccount an existing storage account to be used
* @param containerName the container name within the storage account to use
* @param fileName the exported database file name
* @return response object
*/
SqlDatabaseImportRequest.DefinitionStages.WithAuthenticationTypeAndLoginPassword importBacpac(
StorageAccount storageAccount, String containerName, String fileName);
/**
* Begins a definition for a security alert policy.
*
* @param policyName the name of the security alert policy
* @return the first stage of the SqlDatabaseThreatDetectionPolicy definition
* @deprecated use {@link SqlDatabase#defineThreatDetectionPolicy(SecurityAlertPolicyName)}
*/
@Deprecated
SqlDatabaseThreatDetectionPolicy.DefinitionStages.Blank defineThreatDetectionPolicy(String policyName);
/**
* Begins a definition for a security alert policy.
*
* @param policyName the name of the security alert policy
* @return the first stage of the SqlDatabaseThreatDetectionPolicy definition
*/
SqlDatabaseThreatDetectionPolicy.DefinitionStages.Blank defineThreatDetectionPolicy(SecurityAlertPolicyName policyName);
/**
* Gets a SQL database threat detection policy.
*
* @return the SQL database threat detection policy for the current database
*/
SqlDatabaseThreatDetectionPolicy getThreatDetectionPolicy();
/**
* Gets a SQL database automatic tuning state and options.
*
* @return the SQL database automatic tuning state and options
*/
SqlDatabaseAutomaticTuning getDatabaseAutomaticTuning();
/**
* Lists the SQL database usage metrics.
*
* @return the SQL database usage metrics
*/
List listUsageMetrics();
/**
* Asynchronously lists the SQL database usage metrics.
*
* @return a representation of the deferred computation of this call returning the SQL database usage metrics
*/
PagedFlux listUsageMetricsAsync();
/**
* Renames the database.
*
* @param newDatabaseName the new name for the database
* @return the renamed SQL database
*/
SqlDatabase rename(String newDatabaseName);
/**
* Renames the database asynchronously.
*
* @param newDatabaseName the new name for the database
* @return a representation of the deferred computation of this call
*/
Mono renameAsync(String newDatabaseName);
/** Deletes the database from the server. */
void delete();
/**
* Deletes the database asynchronously.
*
* @return a representation of the deferred computation of this call
*/
Mono deleteAsync();
/** @return the SQL Sync Group entry point for the current database */
SqlSyncGroupOperations.SqlSyncGroupActionsDefinition syncGroups();
/**************************************************************
* Fluent interfaces to provision a SQL Database
**************************************************************/
/**
* Container interface for all the definitions that need to be implemented.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface SqlDatabaseDefinition
extends SqlDatabase.DefinitionStages.Blank,
SqlDatabase.DefinitionStages.WithAllDifferentOptions,
SqlDatabase.DefinitionStages.WithElasticPoolName,
SqlDatabase.DefinitionStages.WithRestorableDroppedDatabase,
SqlDatabase.DefinitionStages.WithImportFrom,
SqlDatabase.DefinitionStages.WithStorageKey,
SqlDatabase.DefinitionStages.WithAuthentication,
SqlDatabase.DefinitionStages.WithRestorePointDatabase,
SqlDatabase.DefinitionStages.WithSourceDatabaseId,
SqlDatabase.DefinitionStages.WithCreateMode,
SqlDatabase.DefinitionStages.WithAttachAllOptions,
SqlDatabase.DefinitionStages.WithAttachFinal {
}
/** Grouping of all the SQL Database definition stages. */
interface DefinitionStages {
/**
* The first stage of the SQL Server Firewall rule definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface Blank extends SqlDatabase.DefinitionStages.WithAllDifferentOptions {
}
/**
* The SQL database interface with all starting options for definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithAllDifferentOptions
extends SqlDatabase.DefinitionStages.WithElasticPoolName,
SqlDatabase.DefinitionStages.WithRestorableDroppedDatabase,
SqlDatabase.DefinitionStages.WithImportFrom,
SqlDatabase.DefinitionStages.WithRestorePointDatabase,
SqlDatabase.DefinitionStages.WithSampleDatabase,
SqlDatabase.DefinitionStages.WithSourceDatabaseId,
SqlDatabase.DefinitionStages.WithEditionDefaults,
SqlDatabase.DefinitionStages.WithAttachAllOptions {
}
/**
* The SQL Database definition to set the elastic pool for database.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithElasticPoolName {
/**
* Sets the existing elastic pool for the SQLDatabase.
*
* @param elasticPoolName for the SQL Database
* @return The next stage of the definition.
*/
WithExistingDatabaseAfterElasticPool withExistingElasticPool(String elasticPoolName);
/**
* Sets the existing elastic pool for the SQLDatabase.
*
* @param elasticPoolId for the SQL Database
* @return The next stage of the definition.
*/
WithExistingDatabaseAfterElasticPool withExistingElasticPoolId(String elasticPoolId);
/**
* Sets the existing elastic pool for the SQLDatabase.
*
* @param sqlElasticPool for the SQL Database
* @return The next stage of the definition.
*/
WithExistingDatabaseAfterElasticPool withExistingElasticPool(SqlElasticPool sqlElasticPool);
/**
* Sets the new elastic pool for the SQLDatabase, this will create a new elastic pool while creating
* database.
*
* @param sqlElasticPool creatable definition for new elastic pool to be created for the SQL Database
* @return The next stage of the definition.
*/
WithExistingDatabaseAfterElasticPool withNewElasticPool(Creatable sqlElasticPool);
}
/**
* The stage to decide whether using existing database or not.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithExistingDatabaseAfterElasticPool
extends SqlDatabase.DefinitionStages.WithImportFromAfterElasticPool,
SqlDatabase.DefinitionStages.WithRestorePointDatabaseAfterElasticPool,
SqlDatabase.DefinitionStages.WithSampleDatabaseAfterElasticPool,
SqlDatabase.DefinitionStages.WithSourceDatabaseId,
SqlDatabase.DefinitionStages.WithAttachAfterElasticPoolOptions {
}
/**
* The SQL Database definition to import a BACPAC file as the source database.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithImportFrom {
/**
* Creates a new database from a BACPAC file.
*
* @param storageUri the source URI for the database to be imported
* @return The next stage of the definition.
*/
SqlDatabase.DefinitionStages.WithStorageKey importFrom(String storageUri);
/**
* Creates a new database from a BACPAC file.
*
* @param storageAccount an existing storage account to be used
* @param containerName the container name within the storage account to use
* @param fileName the exported database file name
* @return The next stage of the definition.
*/
SqlDatabase.DefinitionStages.WithAuthentication importFrom(
StorageAccount storageAccount, String containerName, String fileName);
}
/**
* Sets the storage key type and value to use.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithStorageKey {
/**
* @param storageAccessKey the storage access key to use
* @return next definition stage
*/
SqlDatabase.DefinitionStages.WithAuthentication withStorageAccessKey(String storageAccessKey);
/**
* @param sharedAccessKey the shared access key to use; it must be preceded with a "?."
* @return next definition stage
*/
SqlDatabase.DefinitionStages.WithAuthentication withSharedAccessKey(String sharedAccessKey);
}
/**
* Sets the authentication type and SQL or Active Directory administrator login and password.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithAuthentication {
/**
* @param administratorLogin the SQL administrator login
* @param administratorPassword the SQL administrator password
* @return next definition stage
*/
SqlDatabase.DefinitionStages.WithAttachAllOptions withSqlAdministratorLoginAndPassword(
String administratorLogin, String administratorPassword);
/**
* @param administratorLogin the Active Directory administrator login
* @param administratorPassword the Active Directory administrator password
* @return next definition stage
*/
SqlDatabase.DefinitionStages.WithAttachAllOptions withActiveDirectoryLoginAndPassword(
String administratorLogin, String administratorPassword);
}
/**
* The SQL Database definition to import a BACPAC file as the source database within an elastic pool.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithImportFromAfterElasticPool {
/**
* Creates a new database from a BACPAC file.
*
* @param storageUri the source URI for the database to be imported
* @return The next stage of the definition.
*/
SqlDatabase.DefinitionStages.WithStorageKeyAfterElasticPool importFrom(String storageUri);
/**
* Creates a new database from a BACPAC file.
*
* @param storageAccount an existing storage account to be used
* @param containerName the container name within the storage account to use
* @param fileName the exported database file name
* @return The next stage of the definition.
*/
SqlDatabase.DefinitionStages.WithAuthenticationAfterElasticPool importFrom(
StorageAccount storageAccount, String containerName, String fileName);
}
/**
* Sets the storage key type and value to use.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithStorageKeyAfterElasticPool {
/**
* @param storageAccessKey the storage access key to use
* @return next definition stage
*/
SqlDatabase.DefinitionStages.WithAuthenticationAfterElasticPool withStorageAccessKey(
String storageAccessKey);
/**
* @param sharedAccessKey the shared access key to use; it must be preceded with a "?."
* @return next definition stage
*/
SqlDatabase.DefinitionStages.WithAuthenticationAfterElasticPool withSharedAccessKey(
String sharedAccessKey);
}
/**
* Sets the authentication type and SQL or Active Directory administrator login and password.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithAuthenticationAfterElasticPool {
/**
* @param administratorLogin the SQL administrator login
* @param administratorPassword the SQL administrator password
* @return next definition stage
*/
SqlDatabase.DefinitionStages.WithAttachFinal withSqlAdministratorLoginAndPassword(
String administratorLogin, String administratorPassword);
/**
* @param administratorLogin the Active Directory administrator login
* @param administratorPassword the Active Directory administrator password
* @return next definition stage
*/
SqlDatabase.DefinitionStages.WithAttachFinal withActiveDirectoryLoginAndPassword(
String administratorLogin, String administratorPassword);
}
/**
* The SQL Database definition to set a restorable dropped database as the source database.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithRestorableDroppedDatabase {
/**
* Creates a new database from a previously deleted database (see restorable dropped database).
*
* Collation, Edition, and MaxSizeBytes must remain the same while the link is active. Values specified
* for these parameters will be ignored.
*
* @param restorableDroppedDatabase the restorable dropped database
* @return The next stage of the definition.
*/
SqlDatabase.DefinitionStages.WithAttachFinal fromRestorableDroppedDatabase(
SqlRestorableDroppedDatabase restorableDroppedDatabase);
}
/**
* The SQL Database definition to set a restore point as the source database within an elastic pool.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithRestorePointDatabaseAfterElasticPool {
/**
* Creates a new database from a restore point.
*
* @param restorePoint the restore point
* @return The next stage of the definition.
*/
SqlDatabase.DefinitionStages.WithAttachAfterElasticPoolOptions fromRestorePoint(
RestorePoint restorePoint);
/**
* Creates a new database from a restore point.
*
* @param restorePoint the restore point
* @param restorePointDateTime date and time to restore from
* @return The next stage of the definition.
*/
SqlDatabase.DefinitionStages.WithAttachAfterElasticPoolOptions fromRestorePoint(
RestorePoint restorePoint, OffsetDateTime restorePointDateTime);
}
/**
* The SQL Database definition to set a restore point as the source database.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithRestorePointDatabase {
/**
* Creates a new database from a restore point.
*
* @param restorePoint the restore point
* @return The next stage of the definition.
*/
SqlDatabase.DefinitionStages.WithAttachAllOptions fromRestorePoint(RestorePoint restorePoint);
/**
* Creates a new database from a restore point.
*
* @param restorePoint the restore point
* @param restorePointDateTime date and time to restore from
* @return The next stage of the definition.
*/
SqlDatabase.DefinitionStages.WithAttachAllOptions fromRestorePoint(
RestorePoint restorePoint, OffsetDateTime restorePointDateTime);
}
/**
* The SQL Database definition to set a sample database as the source database within an elastic pool.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithSampleDatabaseAfterElasticPool {
/**
* Creates a new database from a restore point.
*
* @param sampleName the sample database to use as the source
* @return The next stage of the definition.
*/
SqlDatabase.DefinitionStages.WithAttachAfterElasticPoolOptions fromSample(SampleName sampleName);
}
/**
* The SQL Database definition to set a sample database as the source database.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithSampleDatabase {
/**
* Creates a new database from a restore point.
*
* @param sampleName the sample database to use as the source
* @return The next stage of the definition.
*/
SqlDatabase.DefinitionStages.WithAttachAllOptions fromSample(SampleName sampleName);
}
/**
* The SQL Database definition to set the source database id for database.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithSourceDatabaseId {
/**
* Sets the resource if of source database for the SQL Database.
*
* Collation, Edition, and MaxSizeBytes must remain the same while the link is active. Values specified
* for these parameters will be ignored.
*
* @param sourceDatabaseId id of the source database
* @return The next stage of the definition.
*/
SqlDatabase.DefinitionStages.WithCreateMode withSourceDatabase(String sourceDatabaseId);
/**
* Sets the resource if of source database for the SQL Database.
*
* Collation, Edition, and MaxSizeBytes must remain the same while the link is active. Values specified
* for these parameters will be ignored.
*
* @param sourceDatabase instance of the source database
* @return The next stage of the definition.
*/
SqlDatabase.DefinitionStages.WithCreateMode withSourceDatabase(SqlDatabase sourceDatabase);
}
/**
* The SQL Database definition to set the create mode for database.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithCreateMode {
/**
* Sets the create mode for the SQL Database.
*
* @param createMode create mode for the database, should not be default in this flow
* @return The next stage of the definition.
*/
SqlDatabase.DefinitionStages.WithAttachFinal withMode(CreateMode createMode);
}
/**
* The final stage of the SQL Database definition after the SQL Elastic Pool definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithAttachAfterElasticPoolOptions
extends SqlDatabase.DefinitionStages.WithCollationAfterElasticPoolOptions,
SqlDatabase.DefinitionStages.WithMaxSizeBytesAfterElasticPoolOptions,
SqlDatabase.DefinitionStages.WithAttachFinal {
}
/**
* The SQL Database definition to set the collation for database.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithCollationAfterElasticPoolOptions {
/**
* Sets the collation for the SQL Database.
*
* @param collation collation to be set for database
* @return The next stage of the definition
*/
SqlDatabase.DefinitionStages.WithAttachAfterElasticPoolOptions withCollation(String collation);
}
/**
* The SQL Database definition to set the Max Size in Bytes for database.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithMaxSizeBytesAfterElasticPoolOptions {
/**
* Sets the max size in bytes for SQL Database.
*
* @param maxSizeBytes max size of the Azure SQL Database expressed in bytes. Note: Only the following sizes
* are supported (in addition to limitations being placed on each edition): { 100 MB | 500 MB |1 GB | 5
* GB | 10 GB | 20 GB | 30 GB … 150 GB | 200 GB … 500 GB }
* @return The next stage of the definition.
*/
SqlDatabase.DefinitionStages.WithAttachAfterElasticPoolOptions withMaxSizeBytes(long maxSizeBytes);
}
/**
* The SQL Database definition to set the sku for database.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithSku {
/**
* Sets the sku for the SQL Database.
*
* @param sku sku to be set for database
* @return The next stage of the definition
*/
SqlDatabase.DefinitionStages.WithAttachAllOptions withSku(DatabaseSku sku);
/**
* Sets the sku for the SQL Database.
*
* @param sku sku/edition to be set for database, all possible capabilities could be found by
* {@link SqlServers#getCapabilitiesByRegion(Region)}
* @return The next stage of the definition
*/
SqlDatabase.DefinitionStages.WithAttachAllOptions withSku(Sku sku);
}
/**
* The SQL Database definition to set the edition default for database.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithEditionDefaults extends WithAttachFinal {
/**
* Sets a "Basic" edition for the SQL Database.
*
* @return The next stage of the definition
*/
SqlDatabase.DefinitionStages.WithEditionDefaults withBasicEdition();
/**
* Sets a "Basic" edition and maximum storage capacity for the SQL Database.
*
* @param maxStorageCapacity the maximum storage capacity
* @return The next stage of the definition
*/
SqlDatabase.DefinitionStages.WithEditionDefaults withBasicEdition(
SqlDatabaseBasicStorage maxStorageCapacity);
/**
* Sets a "Standard" edition for the SQL Database.
*
* @param serviceObjective edition to be set for database
* @return The next stage of the definition
*/
SqlDatabase.DefinitionStages.WithEditionDefaults withStandardEdition(
SqlDatabaseStandardServiceObjective serviceObjective);
/**
* Sets a "Standard" edition and maximum storage capacity for the SQL Database.
*
* @param serviceObjective edition to be set for database
* @param maxStorageCapacity edition to be set for database
* @return The next stage of the definition
*/
SqlDatabase.DefinitionStages.WithEditionDefaults withStandardEdition(
SqlDatabaseStandardServiceObjective serviceObjective, SqlDatabaseStandardStorage maxStorageCapacity);
/**
* Sets a "Premium" edition for the SQL Database.
*
* @param serviceObjective edition to be set for database
* @return The next stage of the definition
*/
SqlDatabase.DefinitionStages.WithEditionDefaults withPremiumEdition(
SqlDatabasePremiumServiceObjective serviceObjective);
/**
* Sets a "Premium" edition and maximum storage capacity for the SQL Database.
*
* @param serviceObjective edition to be set for database
* @param maxStorageCapacity edition to be set for database
* @return The next stage of the definition
*/
SqlDatabase.DefinitionStages.WithEditionDefaults withPremiumEdition(
SqlDatabasePremiumServiceObjective serviceObjective, SqlDatabasePremiumStorage maxStorageCapacity);
}
/**
* The SQL Database definition to set the collation for database.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithCollation {
/**
* Sets the collation for the SQL Database.
*
* @param collation collation to be set for database
* @return The next stage of the definition
*/
SqlDatabase.DefinitionStages.WithAttachAllOptions withCollation(String collation);
}
/**
* The SQL Database definition to set the Max Size in Bytes for database.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithMaxSizeBytes {
/**
* Sets the max size in bytes for SQL Database.
*
* @param maxSizeBytes max size of the Azure SQL Database expressed in bytes. Note: Only the following sizes
* are supported (in addition to limitations being placed on each edition): { 100 MB | 500 MB |1 GB | 5
* GB | 10 GB | 20 GB | 30 GB … 150 GB | 200 GB … 500 GB }
* @return The next stage of the definition.
*/
SqlDatabase.DefinitionStages.WithAttachAllOptions withMaxSizeBytes(long maxSizeBytes);
}
/**
* The final stage of the SQL Database definition with all the other options.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithAttachAllOptions
extends SqlDatabase.DefinitionStages.WithSku,
SqlDatabase.DefinitionStages.WithEditionDefaults,
SqlDatabase.DefinitionStages.WithCollation,
SqlDatabase.DefinitionStages.WithMaxSizeBytes,
SqlDatabase.DefinitionStages.WithAttachFinal {
}
/**
* The final stage of the SQL Database definition.
*
* At this stage, any remaining optional settings can be specified, or the SQL Database definition can be
* attached to the parent SQL Server definition.
*
* @param the stage of the parent definition to return to after attaching this definition
*/
interface WithAttachFinal extends Attachable.InDefinition {
}
}
/** The template for a SqlDatabase update operation, containing all the settings that can be modified. */
interface Update
extends UpdateStages.WithEdition,
UpdateStages.WithElasticPoolName,
UpdateStages.WithMaxSizeBytes,
Resource.UpdateWithTags,
Appliable {
}
/** Grouping of all the SqlDatabase update stages. */
interface UpdateStages {
/** The SQL Database definition to set the edition for database. */
interface WithEdition {
/**
* Sets the sku for the SQL Database.
*
* @param sku sku to be set for database
* @return The next stage of the update
*/
Update withSku(DatabaseSku sku);
/**
* Sets the sku for the SQL Database.
*
* @param sku sku/edition to be set for database, all possible capabilities could be found by
* {@link SqlServers#getCapabilitiesByRegion(Region)}
* @return The next stage of the update
*/
Update withSku(Sku sku);
/**
* Sets a "Basic" edition for the SQL Database.
*
* @return The next stage of the definition
*/
Update withBasicEdition();
/**
* Sets a "Basic" edition and maximum storage capacity for the SQL Database.
*
* @param maxStorageCapacity the maximum storage capacity
* @return The next stage of the definition
*/
Update withBasicEdition(SqlDatabaseBasicStorage maxStorageCapacity);
/**
* Sets a "Standard" edition for the SQL Database.
*
* @param serviceObjective edition to be set for database
* @return The next stage of the definition
*/
Update withStandardEdition(SqlDatabaseStandardServiceObjective serviceObjective);
/**
* Sets a "Standard" edition and maximum storage capacity for the SQL Database.
*
* @param serviceObjective edition to be set for database
* @param maxStorageCapacity edition to be set for database
* @return The next stage of the definition
*/
Update withStandardEdition(
SqlDatabaseStandardServiceObjective serviceObjective, SqlDatabaseStandardStorage maxStorageCapacity);
/**
* Sets a "Premium" edition for the SQL Database.
*
* @param serviceObjective edition to be set for database
* @return The next stage of the definition
*/
Update withPremiumEdition(SqlDatabasePremiumServiceObjective serviceObjective);
/**
* Sets a "Premium" edition and maximum storage capacity for the SQL Database.
*
* @param serviceObjective edition to be set for database
* @param maxStorageCapacity edition to be set for database
* @return The next stage of the definition
*/
Update withPremiumEdition(
SqlDatabasePremiumServiceObjective serviceObjective, SqlDatabasePremiumStorage maxStorageCapacity);
}
/** The SQL Database definition to set the Max Size in Bytes for database. */
interface WithMaxSizeBytes {
/**
* Sets the max size in bytes for SQL Database.
*
* @param maxSizeBytes max size of the Azure SQL Database expressed in bytes. Note: Only the following sizes
* are supported (in addition to limitations being placed on each edition): { 100 MB | 500 MB |1 GB | 5
* GB | 10 GB | 20 GB | 30 GB … 150 GB | 200 GB … 500 GB }
* @return The next stage of the update.
*/
Update withMaxSizeBytes(long maxSizeBytes);
}
/** The SQL Database definition to set the elastic pool for database. */
interface WithElasticPoolName {
/**
* Removes database from it's elastic pool.
*
* @return The next stage of the update.
*/
WithEdition withoutElasticPool();
/**
* Sets the existing elastic pool for the SQLDatabase.
*
* @param elasticPoolName for the SQL Database
* @return The next stage of the update.
*/
Update withExistingElasticPool(String elasticPoolName);
/**
* Sets the existing elastic pool for the SQLDatabase.
*
* @param elasticPoolId for the SQL Database
* @return The next stage of the definition.
*/
Update withExistingElasticPoolId(String elasticPoolId);
/**
* Sets the existing elastic pool for the SQLDatabase.
*
* @param sqlElasticPool for the SQL Database
* @return The next stage of the update.
*/
Update withExistingElasticPool(SqlElasticPool sqlElasticPool);
/**
* Sets the new elastic pool for the SQLDatabase, this will create a new elastic pool while creating
* database.
*
* @param sqlElasticPool creatable definition for new elastic pool to be created for the SQL Database
* @return The next stage of the update.
*/
Update withNewElasticPool(Creatable sqlElasticPool);
}
}
}