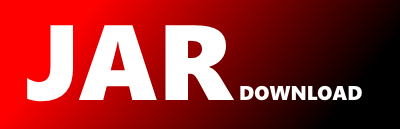
com.azure.resourcemanager.storage.fluent.StorageAccountsClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-storage Show documentation
Show all versions of azure-resourcemanager-storage Show documentation
This package contains Microsoft Azure Storage Management SDK.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.storage.fluent;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.Response;
import com.azure.core.management.polling.PollResult;
import com.azure.core.util.Context;
import com.azure.core.util.polling.PollerFlux;
import com.azure.core.util.polling.SyncPoller;
import com.azure.resourcemanager.resources.fluentcore.collection.InnerSupportsDelete;
import com.azure.resourcemanager.resources.fluentcore.collection.InnerSupportsGet;
import com.azure.resourcemanager.resources.fluentcore.collection.InnerSupportsListing;
import com.azure.resourcemanager.storage.fluent.models.BlobRestoreStatusInner;
import com.azure.resourcemanager.storage.fluent.models.CheckNameAvailabilityResultInner;
import com.azure.resourcemanager.storage.fluent.models.ListAccountSasResponseInner;
import com.azure.resourcemanager.storage.fluent.models.ListServiceSasResponseInner;
import com.azure.resourcemanager.storage.fluent.models.StorageAccountInner;
import com.azure.resourcemanager.storage.fluent.models.StorageAccountListKeysResultInner;
import com.azure.resourcemanager.storage.models.AccountSasParameters;
import com.azure.resourcemanager.storage.models.BlobRestoreParameters;
import com.azure.resourcemanager.storage.models.FailoverType;
import com.azure.resourcemanager.storage.models.ListKeyExpand;
import com.azure.resourcemanager.storage.models.ServiceSasParameters;
import com.azure.resourcemanager.storage.models.StorageAccountCheckNameAvailabilityParameters;
import com.azure.resourcemanager.storage.models.StorageAccountCreateParameters;
import com.azure.resourcemanager.storage.models.StorageAccountExpand;
import com.azure.resourcemanager.storage.models.StorageAccountRegenerateKeyParameters;
import com.azure.resourcemanager.storage.models.StorageAccountUpdateParameters;
import java.nio.ByteBuffer;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
/** An instance of this class provides access to all the operations defined in StorageAccountsClient. */
public interface StorageAccountsClient
extends InnerSupportsGet,
InnerSupportsListing,
InnerSupportsDelete {
/**
* Checks that the storage account name is valid and is not already in use.
*
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the CheckNameAvailability operation response along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> checkNameAvailabilityWithResponseAsync(
StorageAccountCheckNameAvailabilityParameters accountName);
/**
* Checks that the storage account name is valid and is not already in use.
*
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the CheckNameAvailability operation response on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono checkNameAvailabilityAsync(
StorageAccountCheckNameAvailabilityParameters accountName);
/**
* Checks that the storage account name is valid and is not already in use.
*
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the CheckNameAvailability operation response.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
CheckNameAvailabilityResultInner checkNameAvailability(StorageAccountCheckNameAvailabilityParameters accountName);
/**
* Checks that the storage account name is valid and is not already in use.
*
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the CheckNameAvailability operation response along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response checkNameAvailabilityWithResponse(
StorageAccountCheckNameAvailabilityParameters accountName, Context context);
/**
* Asynchronously creates a new storage account with the specified parameters. If an account is already created and
* a subsequent create request is issued with different properties, the account properties will be updated. If an
* account is already created and a subsequent create or update request is issued with the exact same set of
* properties, the request will succeed.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide for the created account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the storage account along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> createWithResponseAsync(
String resourceGroupName, String accountName, StorageAccountCreateParameters parameters);
/**
* Asynchronously creates a new storage account with the specified parameters. If an account is already created and
* a subsequent create request is issued with different properties, the account properties will be updated. If an
* account is already created and a subsequent create or update request is issued with the exact same set of
* properties, the request will succeed.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide for the created account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of the storage account.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, StorageAccountInner> beginCreateAsync(
String resourceGroupName, String accountName, StorageAccountCreateParameters parameters);
/**
* Asynchronously creates a new storage account with the specified parameters. If an account is already created and
* a subsequent create request is issued with different properties, the account properties will be updated. If an
* account is already created and a subsequent create or update request is issued with the exact same set of
* properties, the request will succeed.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide for the created account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of the storage account.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, StorageAccountInner> beginCreate(
String resourceGroupName, String accountName, StorageAccountCreateParameters parameters);
/**
* Asynchronously creates a new storage account with the specified parameters. If an account is already created and
* a subsequent create request is issued with different properties, the account properties will be updated. If an
* account is already created and a subsequent create or update request is issued with the exact same set of
* properties, the request will succeed.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide for the created account.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of the storage account.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, StorageAccountInner> beginCreate(
String resourceGroupName, String accountName, StorageAccountCreateParameters parameters, Context context);
/**
* Asynchronously creates a new storage account with the specified parameters. If an account is already created and
* a subsequent create request is issued with different properties, the account properties will be updated. If an
* account is already created and a subsequent create or update request is issued with the exact same set of
* properties, the request will succeed.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide for the created account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the storage account on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono createAsync(
String resourceGroupName, String accountName, StorageAccountCreateParameters parameters);
/**
* Asynchronously creates a new storage account with the specified parameters. If an account is already created and
* a subsequent create request is issued with different properties, the account properties will be updated. If an
* account is already created and a subsequent create or update request is issued with the exact same set of
* properties, the request will succeed.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide for the created account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the storage account.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
StorageAccountInner create(String resourceGroupName, String accountName, StorageAccountCreateParameters parameters);
/**
* Asynchronously creates a new storage account with the specified parameters. If an account is already created and
* a subsequent create request is issued with different properties, the account properties will be updated. If an
* account is already created and a subsequent create or update request is issued with the exact same set of
* properties, the request will succeed.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide for the created account.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the storage account.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
StorageAccountInner create(
String resourceGroupName, String accountName, StorageAccountCreateParameters parameters, Context context);
/**
* Deletes a storage account in Microsoft Azure.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> deleteWithResponseAsync(String resourceGroupName, String accountName);
/**
* Deletes a storage account in Microsoft Azure.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteAsync(String resourceGroupName, String accountName);
/**
* Deletes a storage account in Microsoft Azure.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void delete(String resourceGroupName, String accountName);
/**
* Deletes a storage account in Microsoft Azure.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response deleteWithResponse(String resourceGroupName, String accountName, Context context);
/**
* Returns the properties for the specified storage account including but not limited to name, SKU name, location,
* and account status. The ListKeys operation should be used to retrieve storage keys.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param expand May be used to expand the properties within account's properties. By default, data is not included
* when fetching properties. Currently we only support geoReplicationStats and blobRestoreStatus.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the storage account along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getByResourceGroupWithResponseAsync(
String resourceGroupName, String accountName, StorageAccountExpand expand);
/**
* Returns the properties for the specified storage account including but not limited to name, SKU name, location,
* and account status. The ListKeys operation should be used to retrieve storage keys.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param expand May be used to expand the properties within account's properties. By default, data is not included
* when fetching properties. Currently we only support geoReplicationStats and blobRestoreStatus.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the storage account on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getByResourceGroupAsync(
String resourceGroupName, String accountName, StorageAccountExpand expand);
/**
* Returns the properties for the specified storage account including but not limited to name, SKU name, location,
* and account status. The ListKeys operation should be used to retrieve storage keys.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the storage account on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getByResourceGroupAsync(String resourceGroupName, String accountName);
/**
* Returns the properties for the specified storage account including but not limited to name, SKU name, location,
* and account status. The ListKeys operation should be used to retrieve storage keys.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the storage account.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
StorageAccountInner getByResourceGroup(String resourceGroupName, String accountName);
/**
* Returns the properties for the specified storage account including but not limited to name, SKU name, location,
* and account status. The ListKeys operation should be used to retrieve storage keys.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param expand May be used to expand the properties within account's properties. By default, data is not included
* when fetching properties. Currently we only support geoReplicationStats and blobRestoreStatus.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the storage account along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getByResourceGroupWithResponse(
String resourceGroupName, String accountName, StorageAccountExpand expand, Context context);
/**
* The update operation can be used to update the SKU, encryption, access tier, or tags for a storage account. It
* can also be used to map the account to a custom domain. Only one custom domain is supported per storage account;
* the replacement/change of custom domain is not supported. In order to replace an old custom domain, the old value
* must be cleared/unregistered before a new value can be set. The update of multiple properties is supported. This
* call does not change the storage keys for the account. If you want to change the storage account keys, use the
* regenerate keys operation. The location and name of the storage account cannot be changed after creation.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide for the updated account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the storage account along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> updateWithResponseAsync(
String resourceGroupName, String accountName, StorageAccountUpdateParameters parameters);
/**
* The update operation can be used to update the SKU, encryption, access tier, or tags for a storage account. It
* can also be used to map the account to a custom domain. Only one custom domain is supported per storage account;
* the replacement/change of custom domain is not supported. In order to replace an old custom domain, the old value
* must be cleared/unregistered before a new value can be set. The update of multiple properties is supported. This
* call does not change the storage keys for the account. If you want to change the storage account keys, use the
* regenerate keys operation. The location and name of the storage account cannot be changed after creation.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide for the updated account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the storage account on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono updateAsync(
String resourceGroupName, String accountName, StorageAccountUpdateParameters parameters);
/**
* The update operation can be used to update the SKU, encryption, access tier, or tags for a storage account. It
* can also be used to map the account to a custom domain. Only one custom domain is supported per storage account;
* the replacement/change of custom domain is not supported. In order to replace an old custom domain, the old value
* must be cleared/unregistered before a new value can be set. The update of multiple properties is supported. This
* call does not change the storage keys for the account. If you want to change the storage account keys, use the
* regenerate keys operation. The location and name of the storage account cannot be changed after creation.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide for the updated account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the storage account.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
StorageAccountInner update(String resourceGroupName, String accountName, StorageAccountUpdateParameters parameters);
/**
* The update operation can be used to update the SKU, encryption, access tier, or tags for a storage account. It
* can also be used to map the account to a custom domain. Only one custom domain is supported per storage account;
* the replacement/change of custom domain is not supported. In order to replace an old custom domain, the old value
* must be cleared/unregistered before a new value can be set. The update of multiple properties is supported. This
* call does not change the storage keys for the account. If you want to change the storage account keys, use the
* regenerate keys operation. The location and name of the storage account cannot be changed after creation.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide for the updated account.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the storage account along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response updateWithResponse(
String resourceGroupName, String accountName, StorageAccountUpdateParameters parameters, Context context);
/**
* Lists all the storage accounts available under the subscription. Note that storage keys are not returned; use the
* ListKeys operation for this.
*
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response from the List Storage Accounts operation as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listAsync();
/**
* Lists all the storage accounts available under the subscription. Note that storage keys are not returned; use the
* ListKeys operation for this.
*
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response from the List Storage Accounts operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable list();
/**
* Lists all the storage accounts available under the subscription. Note that storage keys are not returned; use the
* ListKeys operation for this.
*
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response from the List Storage Accounts operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable list(Context context);
/**
* Lists all the storage accounts available under the given resource group. Note that storage keys are not returned;
* use the ListKeys operation for this.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response from the List Storage Accounts operation as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listByResourceGroupAsync(String resourceGroupName);
/**
* Lists all the storage accounts available under the given resource group. Note that storage keys are not returned;
* use the ListKeys operation for this.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response from the List Storage Accounts operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listByResourceGroup(String resourceGroupName);
/**
* Lists all the storage accounts available under the given resource group. Note that storage keys are not returned;
* use the ListKeys operation for this.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response from the List Storage Accounts operation as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable listByResourceGroup(String resourceGroupName, Context context);
/**
* Lists the access keys or Kerberos keys (if active directory enabled) for the specified storage account.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param expand Specifies type of the key to be listed. Possible value is kerb.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response from the ListKeys operation along with {@link Response} on successful completion of {@link
* Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> listKeysWithResponseAsync(
String resourceGroupName, String accountName, ListKeyExpand expand);
/**
* Lists the access keys or Kerberos keys (if active directory enabled) for the specified storage account.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param expand Specifies type of the key to be listed. Possible value is kerb.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response from the ListKeys operation on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono listKeysAsync(
String resourceGroupName, String accountName, ListKeyExpand expand);
/**
* Lists the access keys or Kerberos keys (if active directory enabled) for the specified storage account.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response from the ListKeys operation on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono listKeysAsync(String resourceGroupName, String accountName);
/**
* Lists the access keys or Kerberos keys (if active directory enabled) for the specified storage account.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response from the ListKeys operation.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
StorageAccountListKeysResultInner listKeys(String resourceGroupName, String accountName);
/**
* Lists the access keys or Kerberos keys (if active directory enabled) for the specified storage account.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param expand Specifies type of the key to be listed. Possible value is kerb.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response from the ListKeys operation along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response listKeysWithResponse(
String resourceGroupName, String accountName, ListKeyExpand expand, Context context);
/**
* Regenerates one of the access keys or Kerberos keys for the specified storage account.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param regenerateKey Specifies name of the key which should be regenerated -- key1, key2, kerb1, kerb2.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response from the ListKeys operation along with {@link Response} on successful completion of {@link
* Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> regenerateKeyWithResponseAsync(
String resourceGroupName, String accountName, StorageAccountRegenerateKeyParameters regenerateKey);
/**
* Regenerates one of the access keys or Kerberos keys for the specified storage account.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param regenerateKey Specifies name of the key which should be regenerated -- key1, key2, kerb1, kerb2.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response from the ListKeys operation on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono regenerateKeyAsync(
String resourceGroupName, String accountName, StorageAccountRegenerateKeyParameters regenerateKey);
/**
* Regenerates one of the access keys or Kerberos keys for the specified storage account.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param regenerateKey Specifies name of the key which should be regenerated -- key1, key2, kerb1, kerb2.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response from the ListKeys operation.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
StorageAccountListKeysResultInner regenerateKey(
String resourceGroupName, String accountName, StorageAccountRegenerateKeyParameters regenerateKey);
/**
* Regenerates one of the access keys or Kerberos keys for the specified storage account.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param regenerateKey Specifies name of the key which should be regenerated -- key1, key2, kerb1, kerb2.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response from the ListKeys operation along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response regenerateKeyWithResponse(
String resourceGroupName,
String accountName,
StorageAccountRegenerateKeyParameters regenerateKey,
Context context);
/**
* List SAS credentials of a storage account.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide to list SAS credentials for the storage account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List SAS credentials operation response along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> listAccountSasWithResponseAsync(
String resourceGroupName, String accountName, AccountSasParameters parameters);
/**
* List SAS credentials of a storage account.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide to list SAS credentials for the storage account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List SAS credentials operation response on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono listAccountSasAsync(
String resourceGroupName, String accountName, AccountSasParameters parameters);
/**
* List SAS credentials of a storage account.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide to list SAS credentials for the storage account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List SAS credentials operation response.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ListAccountSasResponseInner listAccountSas(
String resourceGroupName, String accountName, AccountSasParameters parameters);
/**
* List SAS credentials of a storage account.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide to list SAS credentials for the storage account.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List SAS credentials operation response along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response listAccountSasWithResponse(
String resourceGroupName, String accountName, AccountSasParameters parameters, Context context);
/**
* List service SAS credentials of a specific resource.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide to list service SAS credentials.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List service SAS credentials operation response along with {@link Response} on successful completion
* of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> listServiceSasWithResponseAsync(
String resourceGroupName, String accountName, ServiceSasParameters parameters);
/**
* List service SAS credentials of a specific resource.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide to list service SAS credentials.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List service SAS credentials operation response on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono listServiceSasAsync(
String resourceGroupName, String accountName, ServiceSasParameters parameters);
/**
* List service SAS credentials of a specific resource.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide to list service SAS credentials.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List service SAS credentials operation response.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ListServiceSasResponseInner listServiceSas(
String resourceGroupName, String accountName, ServiceSasParameters parameters);
/**
* List service SAS credentials of a specific resource.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide to list service SAS credentials.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the List service SAS credentials operation response along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response listServiceSasWithResponse(
String resourceGroupName, String accountName, ServiceSasParameters parameters, Context context);
/**
* A failover request can be triggered for a storage account in the event a primary endpoint becomes unavailable for
* any reason. The failover occurs from the storage account's primary cluster to the secondary cluster for RA-GRS
* accounts. The secondary cluster will become primary after failover and the account is converted to LRS. In the
* case of a Planned Failover, the primary and secondary clusters are swapped after failover and the account remains
* geo-replicated. Failover should continue to be used in the event of availability issues as Planned failover is
* only available while the primary and secondary endpoints are available. The primary use case of a Planned
* Failover is disaster recovery testing drills. This type of failover is invoked by setting FailoverType parameter
* to 'Planned'. Learn more about the failover options here-
* https://learn.microsoft.com/en-us/azure/storage/common/storage-disaster-recovery-guidance.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param failoverType The parameter is set to 'Planned' to indicate whether a Planned failover is requested.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> failoverWithResponseAsync(
String resourceGroupName, String accountName, FailoverType failoverType);
/**
* A failover request can be triggered for a storage account in the event a primary endpoint becomes unavailable for
* any reason. The failover occurs from the storage account's primary cluster to the secondary cluster for RA-GRS
* accounts. The secondary cluster will become primary after failover and the account is converted to LRS. In the
* case of a Planned Failover, the primary and secondary clusters are swapped after failover and the account remains
* geo-replicated. Failover should continue to be used in the event of availability issues as Planned failover is
* only available while the primary and secondary endpoints are available. The primary use case of a Planned
* Failover is disaster recovery testing drills. This type of failover is invoked by setting FailoverType parameter
* to 'Planned'. Learn more about the failover options here-
* https://learn.microsoft.com/en-us/azure/storage/common/storage-disaster-recovery-guidance.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param failoverType The parameter is set to 'Planned' to indicate whether a Planned failover is requested.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginFailoverAsync(
String resourceGroupName, String accountName, FailoverType failoverType);
/**
* A failover request can be triggered for a storage account in the event a primary endpoint becomes unavailable for
* any reason. The failover occurs from the storage account's primary cluster to the secondary cluster for RA-GRS
* accounts. The secondary cluster will become primary after failover and the account is converted to LRS. In the
* case of a Planned Failover, the primary and secondary clusters are swapped after failover and the account remains
* geo-replicated. Failover should continue to be used in the event of availability issues as Planned failover is
* only available while the primary and secondary endpoints are available. The primary use case of a Planned
* Failover is disaster recovery testing drills. This type of failover is invoked by setting FailoverType parameter
* to 'Planned'. Learn more about the failover options here-
* https://learn.microsoft.com/en-us/azure/storage/common/storage-disaster-recovery-guidance.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param failoverType The parameter is set to 'Planned' to indicate whether a Planned failover is requested.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginFailover(
String resourceGroupName, String accountName, FailoverType failoverType);
/**
* A failover request can be triggered for a storage account in the event a primary endpoint becomes unavailable for
* any reason. The failover occurs from the storage account's primary cluster to the secondary cluster for RA-GRS
* accounts. The secondary cluster will become primary after failover and the account is converted to LRS. In the
* case of a Planned Failover, the primary and secondary clusters are swapped after failover and the account remains
* geo-replicated. Failover should continue to be used in the event of availability issues as Planned failover is
* only available while the primary and secondary endpoints are available. The primary use case of a Planned
* Failover is disaster recovery testing drills. This type of failover is invoked by setting FailoverType parameter
* to 'Planned'. Learn more about the failover options here-
* https://learn.microsoft.com/en-us/azure/storage/common/storage-disaster-recovery-guidance.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param failoverType The parameter is set to 'Planned' to indicate whether a Planned failover is requested.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginFailover(
String resourceGroupName, String accountName, FailoverType failoverType, Context context);
/**
* A failover request can be triggered for a storage account in the event a primary endpoint becomes unavailable for
* any reason. The failover occurs from the storage account's primary cluster to the secondary cluster for RA-GRS
* accounts. The secondary cluster will become primary after failover and the account is converted to LRS. In the
* case of a Planned Failover, the primary and secondary clusters are swapped after failover and the account remains
* geo-replicated. Failover should continue to be used in the event of availability issues as Planned failover is
* only available while the primary and secondary endpoints are available. The primary use case of a Planned
* Failover is disaster recovery testing drills. This type of failover is invoked by setting FailoverType parameter
* to 'Planned'. Learn more about the failover options here-
* https://learn.microsoft.com/en-us/azure/storage/common/storage-disaster-recovery-guidance.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param failoverType The parameter is set to 'Planned' to indicate whether a Planned failover is requested.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono failoverAsync(String resourceGroupName, String accountName, FailoverType failoverType);
/**
* A failover request can be triggered for a storage account in the event a primary endpoint becomes unavailable for
* any reason. The failover occurs from the storage account's primary cluster to the secondary cluster for RA-GRS
* accounts. The secondary cluster will become primary after failover and the account is converted to LRS. In the
* case of a Planned Failover, the primary and secondary clusters are swapped after failover and the account remains
* geo-replicated. Failover should continue to be used in the event of availability issues as Planned failover is
* only available while the primary and secondary endpoints are available. The primary use case of a Planned
* Failover is disaster recovery testing drills. This type of failover is invoked by setting FailoverType parameter
* to 'Planned'. Learn more about the failover options here-
* https://learn.microsoft.com/en-us/azure/storage/common/storage-disaster-recovery-guidance.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono failoverAsync(String resourceGroupName, String accountName);
/**
* A failover request can be triggered for a storage account in the event a primary endpoint becomes unavailable for
* any reason. The failover occurs from the storage account's primary cluster to the secondary cluster for RA-GRS
* accounts. The secondary cluster will become primary after failover and the account is converted to LRS. In the
* case of a Planned Failover, the primary and secondary clusters are swapped after failover and the account remains
* geo-replicated. Failover should continue to be used in the event of availability issues as Planned failover is
* only available while the primary and secondary endpoints are available. The primary use case of a Planned
* Failover is disaster recovery testing drills. This type of failover is invoked by setting FailoverType parameter
* to 'Planned'. Learn more about the failover options here-
* https://learn.microsoft.com/en-us/azure/storage/common/storage-disaster-recovery-guidance.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param failoverType The parameter is set to 'Planned' to indicate whether a Planned failover is requested.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void failover(String resourceGroupName, String accountName, FailoverType failoverType);
/**
* A failover request can be triggered for a storage account in the event a primary endpoint becomes unavailable for
* any reason. The failover occurs from the storage account's primary cluster to the secondary cluster for RA-GRS
* accounts. The secondary cluster will become primary after failover and the account is converted to LRS. In the
* case of a Planned Failover, the primary and secondary clusters are swapped after failover and the account remains
* geo-replicated. Failover should continue to be used in the event of availability issues as Planned failover is
* only available while the primary and secondary endpoints are available. The primary use case of a Planned
* Failover is disaster recovery testing drills. This type of failover is invoked by setting FailoverType parameter
* to 'Planned'. Learn more about the failover options here-
* https://learn.microsoft.com/en-us/azure/storage/common/storage-disaster-recovery-guidance.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void failover(String resourceGroupName, String accountName);
/**
* A failover request can be triggered for a storage account in the event a primary endpoint becomes unavailable for
* any reason. The failover occurs from the storage account's primary cluster to the secondary cluster for RA-GRS
* accounts. The secondary cluster will become primary after failover and the account is converted to LRS. In the
* case of a Planned Failover, the primary and secondary clusters are swapped after failover and the account remains
* geo-replicated. Failover should continue to be used in the event of availability issues as Planned failover is
* only available while the primary and secondary endpoints are available. The primary use case of a Planned
* Failover is disaster recovery testing drills. This type of failover is invoked by setting FailoverType parameter
* to 'Planned'. Learn more about the failover options here-
* https://learn.microsoft.com/en-us/azure/storage/common/storage-disaster-recovery-guidance.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param failoverType The parameter is set to 'Planned' to indicate whether a Planned failover is requested.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void failover(String resourceGroupName, String accountName, FailoverType failoverType, Context context);
/**
* Live Migration of storage account to enable Hns.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param requestType Required. Hierarchical namespace migration type can either be a hierarchical namespace
* validation request 'HnsOnValidationRequest' or a hydration request 'HnsOnHydrationRequest'. The validation
* request will validate the migration whereas the hydration request will migrate the account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> hierarchicalNamespaceMigrationWithResponseAsync(
String resourceGroupName, String accountName, String requestType);
/**
* Live Migration of storage account to enable Hns.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param requestType Required. Hierarchical namespace migration type can either be a hierarchical namespace
* validation request 'HnsOnValidationRequest' or a hydration request 'HnsOnHydrationRequest'. The validation
* request will validate the migration whereas the hydration request will migrate the account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginHierarchicalNamespaceMigrationAsync(
String resourceGroupName, String accountName, String requestType);
/**
* Live Migration of storage account to enable Hns.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param requestType Required. Hierarchical namespace migration type can either be a hierarchical namespace
* validation request 'HnsOnValidationRequest' or a hydration request 'HnsOnHydrationRequest'. The validation
* request will validate the migration whereas the hydration request will migrate the account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginHierarchicalNamespaceMigration(
String resourceGroupName, String accountName, String requestType);
/**
* Live Migration of storage account to enable Hns.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param requestType Required. Hierarchical namespace migration type can either be a hierarchical namespace
* validation request 'HnsOnValidationRequest' or a hydration request 'HnsOnHydrationRequest'. The validation
* request will validate the migration whereas the hydration request will migrate the account.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginHierarchicalNamespaceMigration(
String resourceGroupName, String accountName, String requestType, Context context);
/**
* Live Migration of storage account to enable Hns.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param requestType Required. Hierarchical namespace migration type can either be a hierarchical namespace
* validation request 'HnsOnValidationRequest' or a hydration request 'HnsOnHydrationRequest'. The validation
* request will validate the migration whereas the hydration request will migrate the account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono hierarchicalNamespaceMigrationAsync(String resourceGroupName, String accountName, String requestType);
/**
* Live Migration of storage account to enable Hns.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param requestType Required. Hierarchical namespace migration type can either be a hierarchical namespace
* validation request 'HnsOnValidationRequest' or a hydration request 'HnsOnHydrationRequest'. The validation
* request will validate the migration whereas the hydration request will migrate the account.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void hierarchicalNamespaceMigration(String resourceGroupName, String accountName, String requestType);
/**
* Live Migration of storage account to enable Hns.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param requestType Required. Hierarchical namespace migration type can either be a hierarchical namespace
* validation request 'HnsOnValidationRequest' or a hydration request 'HnsOnHydrationRequest'. The validation
* request will validate the migration whereas the hydration request will migrate the account.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void hierarchicalNamespaceMigration(
String resourceGroupName, String accountName, String requestType, Context context);
/**
* Abort live Migration of storage account to enable Hns.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> abortHierarchicalNamespaceMigrationWithResponseAsync(
String resourceGroupName, String accountName);
/**
* Abort live Migration of storage account to enable Hns.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginAbortHierarchicalNamespaceMigrationAsync(
String resourceGroupName, String accountName);
/**
* Abort live Migration of storage account to enable Hns.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginAbortHierarchicalNamespaceMigration(
String resourceGroupName, String accountName);
/**
* Abort live Migration of storage account to enable Hns.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginAbortHierarchicalNamespaceMigration(
String resourceGroupName, String accountName, Context context);
/**
* Abort live Migration of storage account to enable Hns.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono abortHierarchicalNamespaceMigrationAsync(String resourceGroupName, String accountName);
/**
* Abort live Migration of storage account to enable Hns.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void abortHierarchicalNamespaceMigration(String resourceGroupName, String accountName);
/**
* Abort live Migration of storage account to enable Hns.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void abortHierarchicalNamespaceMigration(String resourceGroupName, String accountName, Context context);
/**
* Restore blobs in the specified blob ranges.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide for restore blob ranges.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return blob restore status along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> restoreBlobRangesWithResponseAsync(
String resourceGroupName, String accountName, BlobRestoreParameters parameters);
/**
* Restore blobs in the specified blob ranges.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide for restore blob ranges.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of blob restore status.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, BlobRestoreStatusInner> beginRestoreBlobRangesAsync(
String resourceGroupName, String accountName, BlobRestoreParameters parameters);
/**
* Restore blobs in the specified blob ranges.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide for restore blob ranges.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of blob restore status.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, BlobRestoreStatusInner> beginRestoreBlobRanges(
String resourceGroupName, String accountName, BlobRestoreParameters parameters);
/**
* Restore blobs in the specified blob ranges.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide for restore blob ranges.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of blob restore status.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, BlobRestoreStatusInner> beginRestoreBlobRanges(
String resourceGroupName, String accountName, BlobRestoreParameters parameters, Context context);
/**
* Restore blobs in the specified blob ranges.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide for restore blob ranges.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return blob restore status on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono restoreBlobRangesAsync(
String resourceGroupName, String accountName, BlobRestoreParameters parameters);
/**
* Restore blobs in the specified blob ranges.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide for restore blob ranges.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return blob restore status.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
BlobRestoreStatusInner restoreBlobRanges(
String resourceGroupName, String accountName, BlobRestoreParameters parameters);
/**
* Restore blobs in the specified blob ranges.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param parameters The parameters to provide for restore blob ranges.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return blob restore status.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
BlobRestoreStatusInner restoreBlobRanges(
String resourceGroupName, String accountName, BlobRestoreParameters parameters, Context context);
/**
* Revoke user delegation keys.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> revokeUserDelegationKeysWithResponseAsync(String resourceGroupName, String accountName);
/**
* Revoke user delegation keys.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono revokeUserDelegationKeysAsync(String resourceGroupName, String accountName);
/**
* Revoke user delegation keys.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void revokeUserDelegationKeys(String resourceGroupName, String accountName);
/**
* Revoke user delegation keys.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response revokeUserDelegationKeysWithResponse(String resourceGroupName, String accountName, Context context);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy