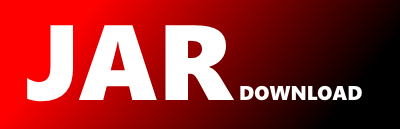
com.azure.resourcemanager.storage.fluent.BlobContainersClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-storage Show documentation
Show all versions of azure-resourcemanager-storage Show documentation
This package contains Microsoft Azure Storage Management SDK.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.storage.fluent;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.Response;
import com.azure.core.management.polling.PollResult;
import com.azure.core.util.Context;
import com.azure.core.util.polling.PollerFlux;
import com.azure.core.util.polling.SyncPoller;
import com.azure.resourcemanager.storage.fluent.models.BlobContainerInner;
import com.azure.resourcemanager.storage.fluent.models.ImmutabilityPolicyInner;
import com.azure.resourcemanager.storage.fluent.models.LeaseContainerResponseInner;
import com.azure.resourcemanager.storage.fluent.models.LegalHoldInner;
import com.azure.resourcemanager.storage.fluent.models.ListContainerItemInner;
import com.azure.resourcemanager.storage.models.BlobContainersCreateOrUpdateImmutabilityPolicyResponse;
import com.azure.resourcemanager.storage.models.BlobContainersDeleteImmutabilityPolicyResponse;
import com.azure.resourcemanager.storage.models.BlobContainersExtendImmutabilityPolicyResponse;
import com.azure.resourcemanager.storage.models.BlobContainersGetImmutabilityPolicyResponse;
import com.azure.resourcemanager.storage.models.BlobContainersLockImmutabilityPolicyResponse;
import com.azure.resourcemanager.storage.models.LeaseContainerRequest;
import com.azure.resourcemanager.storage.models.ListContainersInclude;
import java.nio.ByteBuffer;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
/**
* An instance of this class provides access to all the operations defined in BlobContainersClient.
*/
public interface BlobContainersClient {
/**
* Lists all containers and does not support a prefix like data plane. Also SRP today does not return continuation
* token.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param maxpagesize Optional. Specified maximum number of containers that can be included in the list.
* @param filter Optional. When specified, only container names starting with the filter will be listed.
* @param include Optional, used to include the properties for soft deleted blob containers.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response schema as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listAsync(String resourceGroupName, String accountName, String maxpagesize,
String filter, ListContainersInclude include);
/**
* Lists all containers and does not support a prefix like data plane. Also SRP today does not return continuation
* token.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response schema as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedFlux listAsync(String resourceGroupName, String accountName);
/**
* Lists all containers and does not support a prefix like data plane. Also SRP today does not return continuation
* token.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response schema as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable list(String resourceGroupName, String accountName);
/**
* Lists all containers and does not support a prefix like data plane. Also SRP today does not return continuation
* token.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param maxpagesize Optional. Specified maximum number of containers that can be included in the list.
* @param filter Optional. When specified, only container names starting with the filter will be listed.
* @param include Optional, used to include the properties for soft deleted blob containers.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return response schema as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
PagedIterable list(String resourceGroupName, String accountName, String maxpagesize,
String filter, ListContainersInclude include, Context context);
/**
* Creates a new container under the specified account as described by request body. The container resource includes
* metadata and properties for that container. It does not include a list of the blobs contained by the container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param blobContainer Properties of the blob container to create.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return properties of the blob container, including Id, resource name, resource type, Etag along with
* {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> createWithResponseAsync(String resourceGroupName, String accountName,
String containerName, BlobContainerInner blobContainer);
/**
* Creates a new container under the specified account as described by request body. The container resource includes
* metadata and properties for that container. It does not include a list of the blobs contained by the container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param blobContainer Properties of the blob container to create.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return properties of the blob container, including Id, resource name, resource type, Etag on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono createAsync(String resourceGroupName, String accountName, String containerName,
BlobContainerInner blobContainer);
/**
* Creates a new container under the specified account as described by request body. The container resource includes
* metadata and properties for that container. It does not include a list of the blobs contained by the container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param blobContainer Properties of the blob container to create.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return properties of the blob container, including Id, resource name, resource type, Etag along with
* {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response createWithResponse(String resourceGroupName, String accountName, String containerName,
BlobContainerInner blobContainer, Context context);
/**
* Creates a new container under the specified account as described by request body. The container resource includes
* metadata and properties for that container. It does not include a list of the blobs contained by the container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param blobContainer Properties of the blob container to create.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return properties of the blob container, including Id, resource name, resource type, Etag.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
BlobContainerInner create(String resourceGroupName, String accountName, String containerName,
BlobContainerInner blobContainer);
/**
* Updates container properties as specified in request body. Properties not mentioned in the request will be
* unchanged. Update fails if the specified container doesn't already exist.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param blobContainer Properties to update for the blob container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return properties of the blob container, including Id, resource name, resource type, Etag along with
* {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> updateWithResponseAsync(String resourceGroupName, String accountName,
String containerName, BlobContainerInner blobContainer);
/**
* Updates container properties as specified in request body. Properties not mentioned in the request will be
* unchanged. Update fails if the specified container doesn't already exist.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param blobContainer Properties to update for the blob container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return properties of the blob container, including Id, resource name, resource type, Etag on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono updateAsync(String resourceGroupName, String accountName, String containerName,
BlobContainerInner blobContainer);
/**
* Updates container properties as specified in request body. Properties not mentioned in the request will be
* unchanged. Update fails if the specified container doesn't already exist.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param blobContainer Properties to update for the blob container.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return properties of the blob container, including Id, resource name, resource type, Etag along with
* {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response updateWithResponse(String resourceGroupName, String accountName, String containerName,
BlobContainerInner blobContainer, Context context);
/**
* Updates container properties as specified in request body. Properties not mentioned in the request will be
* unchanged. Update fails if the specified container doesn't already exist.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param blobContainer Properties to update for the blob container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return properties of the blob container, including Id, resource name, resource type, Etag.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
BlobContainerInner update(String resourceGroupName, String accountName, String containerName,
BlobContainerInner blobContainer);
/**
* Gets properties of a specified container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return properties of a specified container along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> getWithResponseAsync(String resourceGroupName, String accountName,
String containerName);
/**
* Gets properties of a specified container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return properties of a specified container on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getAsync(String resourceGroupName, String accountName, String containerName);
/**
* Gets properties of a specified container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return properties of a specified container along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response getWithResponse(String resourceGroupName, String accountName, String containerName,
Context context);
/**
* Gets properties of a specified container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return properties of a specified container.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
BlobContainerInner get(String resourceGroupName, String accountName, String containerName);
/**
* Deletes specified container under its account.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> deleteWithResponseAsync(String resourceGroupName, String accountName, String containerName);
/**
* Deletes specified container under its account.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteAsync(String resourceGroupName, String accountName, String containerName);
/**
* Deletes specified container under its account.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response deleteWithResponse(String resourceGroupName, String accountName, String containerName,
Context context);
/**
* Deletes specified container under its account.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void delete(String resourceGroupName, String accountName, String containerName);
/**
* Sets legal hold tags. Setting the same tag results in an idempotent operation. SetLegalHold follows an append
* pattern and does not clear out the existing tags that are not specified in the request.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param legalHold The LegalHold property that will be set to a blob container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the LegalHold property of a blob container along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> setLegalHoldWithResponseAsync(String resourceGroupName, String accountName,
String containerName, LegalHoldInner legalHold);
/**
* Sets legal hold tags. Setting the same tag results in an idempotent operation. SetLegalHold follows an append
* pattern and does not clear out the existing tags that are not specified in the request.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param legalHold The LegalHold property that will be set to a blob container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the LegalHold property of a blob container on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono setLegalHoldAsync(String resourceGroupName, String accountName, String containerName,
LegalHoldInner legalHold);
/**
* Sets legal hold tags. Setting the same tag results in an idempotent operation. SetLegalHold follows an append
* pattern and does not clear out the existing tags that are not specified in the request.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param legalHold The LegalHold property that will be set to a blob container.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the LegalHold property of a blob container along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response setLegalHoldWithResponse(String resourceGroupName, String accountName,
String containerName, LegalHoldInner legalHold, Context context);
/**
* Sets legal hold tags. Setting the same tag results in an idempotent operation. SetLegalHold follows an append
* pattern and does not clear out the existing tags that are not specified in the request.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param legalHold The LegalHold property that will be set to a blob container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the LegalHold property of a blob container.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
LegalHoldInner setLegalHold(String resourceGroupName, String accountName, String containerName,
LegalHoldInner legalHold);
/**
* Clears legal hold tags. Clearing the same or non-existent tag results in an idempotent operation. ClearLegalHold
* clears out only the specified tags in the request.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param legalHold The LegalHold property that will be clear from a blob container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the LegalHold property of a blob container along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> clearLegalHoldWithResponseAsync(String resourceGroupName, String accountName,
String containerName, LegalHoldInner legalHold);
/**
* Clears legal hold tags. Clearing the same or non-existent tag results in an idempotent operation. ClearLegalHold
* clears out only the specified tags in the request.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param legalHold The LegalHold property that will be clear from a blob container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the LegalHold property of a blob container on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono clearLegalHoldAsync(String resourceGroupName, String accountName, String containerName,
LegalHoldInner legalHold);
/**
* Clears legal hold tags. Clearing the same or non-existent tag results in an idempotent operation. ClearLegalHold
* clears out only the specified tags in the request.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param legalHold The LegalHold property that will be clear from a blob container.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the LegalHold property of a blob container along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response clearLegalHoldWithResponse(String resourceGroupName, String accountName,
String containerName, LegalHoldInner legalHold, Context context);
/**
* Clears legal hold tags. Clearing the same or non-existent tag results in an idempotent operation. ClearLegalHold
* clears out only the specified tags in the request.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param legalHold The LegalHold property that will be clear from a blob container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the LegalHold property of a blob container.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
LegalHoldInner clearLegalHold(String resourceGroupName, String accountName, String containerName,
LegalHoldInner legalHold);
/**
* Creates or updates an unlocked immutability policy. ETag in If-Match is honored if given but not required for
* this operation.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param ifMatch The entity state (ETag) version of the immutability policy to update. A value of "*" can be used
* to apply the operation only if the immutability policy already exists. If omitted, this operation will always be
* applied.
* @param parameters The ImmutabilityPolicy Properties that will be created or updated to a blob container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ImmutabilityPolicy property of a blob container, including Id, resource name, resource type, Etag on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono createOrUpdateImmutabilityPolicyWithResponseAsync(
String resourceGroupName, String accountName, String containerName, String ifMatch,
ImmutabilityPolicyInner parameters);
/**
* Creates or updates an unlocked immutability policy. ETag in If-Match is honored if given but not required for
* this operation.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ImmutabilityPolicy property of a blob container, including Id, resource name, resource type, Etag on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono createOrUpdateImmutabilityPolicyAsync(String resourceGroupName, String accountName,
String containerName);
/**
* Creates or updates an unlocked immutability policy. ETag in If-Match is honored if given but not required for
* this operation.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param ifMatch The entity state (ETag) version of the immutability policy to update. A value of "*" can be used
* to apply the operation only if the immutability policy already exists. If omitted, this operation will always be
* applied.
* @param parameters The ImmutabilityPolicy Properties that will be created or updated to a blob container.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ImmutabilityPolicy property of a blob container, including Id, resource name, resource type, Etag.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
BlobContainersCreateOrUpdateImmutabilityPolicyResponse createOrUpdateImmutabilityPolicyWithResponse(
String resourceGroupName, String accountName, String containerName, String ifMatch,
ImmutabilityPolicyInner parameters, Context context);
/**
* Creates or updates an unlocked immutability policy. ETag in If-Match is honored if given but not required for
* this operation.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ImmutabilityPolicy property of a blob container, including Id, resource name, resource type, Etag.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ImmutabilityPolicyInner createOrUpdateImmutabilityPolicy(String resourceGroupName, String accountName,
String containerName);
/**
* Gets the existing immutability policy along with the corresponding ETag in response headers and body.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param ifMatch The entity state (ETag) version of the immutability policy to update. A value of "*" can be used
* to apply the operation only if the immutability policy already exists. If omitted, this operation will always be
* applied.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the existing immutability policy along with the corresponding ETag in response headers and body on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getImmutabilityPolicyWithResponseAsync(String resourceGroupName,
String accountName, String containerName, String ifMatch);
/**
* Gets the existing immutability policy along with the corresponding ETag in response headers and body.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the existing immutability policy along with the corresponding ETag in response headers and body on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono getImmutabilityPolicyAsync(String resourceGroupName, String accountName,
String containerName);
/**
* Gets the existing immutability policy along with the corresponding ETag in response headers and body.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param ifMatch The entity state (ETag) version of the immutability policy to update. A value of "*" can be used
* to apply the operation only if the immutability policy already exists. If omitted, this operation will always be
* applied.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the existing immutability policy along with the corresponding ETag in response headers and body.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
BlobContainersGetImmutabilityPolicyResponse getImmutabilityPolicyWithResponse(String resourceGroupName,
String accountName, String containerName, String ifMatch, Context context);
/**
* Gets the existing immutability policy along with the corresponding ETag in response headers and body.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the existing immutability policy along with the corresponding ETag in response headers and body.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ImmutabilityPolicyInner getImmutabilityPolicy(String resourceGroupName, String accountName, String containerName);
/**
* Aborts an unlocked immutability policy. The response of delete has immutabilityPeriodSinceCreationInDays set to
* 0. ETag in If-Match is required for this operation. Deleting a locked immutability policy is not allowed, the
* only way is to delete the container after deleting all expired blobs inside the policy locked container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param ifMatch The entity state (ETag) version of the immutability policy to update. A value of "*" can be used
* to apply the operation only if the immutability policy already exists. If omitted, this operation will always be
* applied.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ImmutabilityPolicy property of a blob container, including Id, resource name, resource type, Etag on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteImmutabilityPolicyWithResponseAsync(
String resourceGroupName, String accountName, String containerName, String ifMatch);
/**
* Aborts an unlocked immutability policy. The response of delete has immutabilityPeriodSinceCreationInDays set to
* 0. ETag in If-Match is required for this operation. Deleting a locked immutability policy is not allowed, the
* only way is to delete the container after deleting all expired blobs inside the policy locked container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param ifMatch The entity state (ETag) version of the immutability policy to update. A value of "*" can be used
* to apply the operation only if the immutability policy already exists. If omitted, this operation will always be
* applied.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ImmutabilityPolicy property of a blob container, including Id, resource name, resource type, Etag on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono deleteImmutabilityPolicyAsync(String resourceGroupName, String accountName,
String containerName, String ifMatch);
/**
* Aborts an unlocked immutability policy. The response of delete has immutabilityPeriodSinceCreationInDays set to
* 0. ETag in If-Match is required for this operation. Deleting a locked immutability policy is not allowed, the
* only way is to delete the container after deleting all expired blobs inside the policy locked container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param ifMatch The entity state (ETag) version of the immutability policy to update. A value of "*" can be used
* to apply the operation only if the immutability policy already exists. If omitted, this operation will always be
* applied.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ImmutabilityPolicy property of a blob container, including Id, resource name, resource type, Etag.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
BlobContainersDeleteImmutabilityPolicyResponse deleteImmutabilityPolicyWithResponse(String resourceGroupName,
String accountName, String containerName, String ifMatch, Context context);
/**
* Aborts an unlocked immutability policy. The response of delete has immutabilityPeriodSinceCreationInDays set to
* 0. ETag in If-Match is required for this operation. Deleting a locked immutability policy is not allowed, the
* only way is to delete the container after deleting all expired blobs inside the policy locked container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param ifMatch The entity state (ETag) version of the immutability policy to update. A value of "*" can be used
* to apply the operation only if the immutability policy already exists. If omitted, this operation will always be
* applied.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ImmutabilityPolicy property of a blob container, including Id, resource name, resource type, Etag.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ImmutabilityPolicyInner deleteImmutabilityPolicy(String resourceGroupName, String accountName, String containerName,
String ifMatch);
/**
* Sets the ImmutabilityPolicy to Locked state. The only action allowed on a Locked policy is
* ExtendImmutabilityPolicy action. ETag in If-Match is required for this operation.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param ifMatch The entity state (ETag) version of the immutability policy to update. A value of "*" can be used
* to apply the operation only if the immutability policy already exists. If omitted, this operation will always be
* applied.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ImmutabilityPolicy property of a blob container, including Id, resource name, resource type, Etag on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono lockImmutabilityPolicyWithResponseAsync(String resourceGroupName,
String accountName, String containerName, String ifMatch);
/**
* Sets the ImmutabilityPolicy to Locked state. The only action allowed on a Locked policy is
* ExtendImmutabilityPolicy action. ETag in If-Match is required for this operation.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param ifMatch The entity state (ETag) version of the immutability policy to update. A value of "*" can be used
* to apply the operation only if the immutability policy already exists. If omitted, this operation will always be
* applied.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ImmutabilityPolicy property of a blob container, including Id, resource name, resource type, Etag on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono lockImmutabilityPolicyAsync(String resourceGroupName, String accountName,
String containerName, String ifMatch);
/**
* Sets the ImmutabilityPolicy to Locked state. The only action allowed on a Locked policy is
* ExtendImmutabilityPolicy action. ETag in If-Match is required for this operation.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param ifMatch The entity state (ETag) version of the immutability policy to update. A value of "*" can be used
* to apply the operation only if the immutability policy already exists. If omitted, this operation will always be
* applied.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ImmutabilityPolicy property of a blob container, including Id, resource name, resource type, Etag.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
BlobContainersLockImmutabilityPolicyResponse lockImmutabilityPolicyWithResponse(String resourceGroupName,
String accountName, String containerName, String ifMatch, Context context);
/**
* Sets the ImmutabilityPolicy to Locked state. The only action allowed on a Locked policy is
* ExtendImmutabilityPolicy action. ETag in If-Match is required for this operation.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param ifMatch The entity state (ETag) version of the immutability policy to update. A value of "*" can be used
* to apply the operation only if the immutability policy already exists. If omitted, this operation will always be
* applied.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ImmutabilityPolicy property of a blob container, including Id, resource name, resource type, Etag.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ImmutabilityPolicyInner lockImmutabilityPolicy(String resourceGroupName, String accountName, String containerName,
String ifMatch);
/**
* Extends the immutabilityPeriodSinceCreationInDays of a locked immutabilityPolicy. The only action allowed on a
* Locked policy will be this action. ETag in If-Match is required for this operation.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param ifMatch The entity state (ETag) version of the immutability policy to update. A value of "*" can be used
* to apply the operation only if the immutability policy already exists. If omitted, this operation will always be
* applied.
* @param parameters The ImmutabilityPolicy Properties that will be extended for a blob container.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ImmutabilityPolicy property of a blob container, including Id, resource name, resource type, Etag on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono extendImmutabilityPolicyWithResponseAsync(
String resourceGroupName, String accountName, String containerName, String ifMatch,
ImmutabilityPolicyInner parameters);
/**
* Extends the immutabilityPeriodSinceCreationInDays of a locked immutabilityPolicy. The only action allowed on a
* Locked policy will be this action. ETag in If-Match is required for this operation.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param ifMatch The entity state (ETag) version of the immutability policy to update. A value of "*" can be used
* to apply the operation only if the immutability policy already exists. If omitted, this operation will always be
* applied.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ImmutabilityPolicy property of a blob container, including Id, resource name, resource type, Etag on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono extendImmutabilityPolicyAsync(String resourceGroupName, String accountName,
String containerName, String ifMatch);
/**
* Extends the immutabilityPeriodSinceCreationInDays of a locked immutabilityPolicy. The only action allowed on a
* Locked policy will be this action. ETag in If-Match is required for this operation.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param ifMatch The entity state (ETag) version of the immutability policy to update. A value of "*" can be used
* to apply the operation only if the immutability policy already exists. If omitted, this operation will always be
* applied.
* @param parameters The ImmutabilityPolicy Properties that will be extended for a blob container.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ImmutabilityPolicy property of a blob container, including Id, resource name, resource type, Etag.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
BlobContainersExtendImmutabilityPolicyResponse extendImmutabilityPolicyWithResponse(String resourceGroupName,
String accountName, String containerName, String ifMatch, ImmutabilityPolicyInner parameters, Context context);
/**
* Extends the immutabilityPeriodSinceCreationInDays of a locked immutabilityPolicy. The only action allowed on a
* Locked policy will be this action. ETag in If-Match is required for this operation.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param ifMatch The entity state (ETag) version of the immutability policy to update. A value of "*" can be used
* to apply the operation only if the immutability policy already exists. If omitted, this operation will always be
* applied.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the ImmutabilityPolicy property of a blob container, including Id, resource name, resource type, Etag.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
ImmutabilityPolicyInner extendImmutabilityPolicy(String resourceGroupName, String accountName, String containerName,
String ifMatch);
/**
* The Lease Container operation establishes and manages a lock on a container for delete operations. The lock
* duration can be 15 to 60 seconds, or can be infinite.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param parameters Lease Container request body.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return lease Container response schema along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> leaseWithResponseAsync(String resourceGroupName, String accountName,
String containerName, LeaseContainerRequest parameters);
/**
* The Lease Container operation establishes and manages a lock on a container for delete operations. The lock
* duration can be 15 to 60 seconds, or can be infinite.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return lease Container response schema on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono leaseAsync(String resourceGroupName, String accountName, String containerName);
/**
* The Lease Container operation establishes and manages a lock on a container for delete operations. The lock
* duration can be 15 to 60 seconds, or can be infinite.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param parameters Lease Container request body.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return lease Container response schema along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Response leaseWithResponse(String resourceGroupName, String accountName,
String containerName, LeaseContainerRequest parameters, Context context);
/**
* The Lease Container operation establishes and manages a lock on a container for delete operations. The lock
* duration can be 15 to 60 seconds, or can be infinite.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return lease Container response schema.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
LeaseContainerResponseInner lease(String resourceGroupName, String accountName, String containerName);
/**
* This operation migrates a blob container from container level WORM to object level immutability enabled
* container. Prerequisites require a container level immutability policy either in locked or unlocked state,
* Account level versioning must be enabled and there should be no Legal hold on the container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono>> objectLevelWormWithResponseAsync(String resourceGroupName, String accountName,
String containerName);
/**
* This operation migrates a blob container from container level WORM to object level immutability enabled
* container. Prerequisites require a container level immutability policy either in locked or unlocked state,
* Account level versioning must be enabled and there should be no Legal hold on the container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link PollerFlux} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
PollerFlux, Void> beginObjectLevelWormAsync(String resourceGroupName, String accountName,
String containerName);
/**
* This operation migrates a blob container from container level WORM to object level immutability enabled
* container. Prerequisites require a container level immutability policy either in locked or unlocked state,
* Account level versioning must be enabled and there should be no Legal hold on the container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginObjectLevelWorm(String resourceGroupName, String accountName,
String containerName);
/**
* This operation migrates a blob container from container level WORM to object level immutability enabled
* container. Prerequisites require a container level immutability policy either in locked or unlocked state,
* Account level versioning must be enabled and there should be no Legal hold on the container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the {@link SyncPoller} for polling of long-running operation.
*/
@ServiceMethod(returns = ReturnType.LONG_RUNNING_OPERATION)
SyncPoller, Void> beginObjectLevelWorm(String resourceGroupName, String accountName,
String containerName, Context context);
/**
* This operation migrates a blob container from container level WORM to object level immutability enabled
* container. Prerequisites require a container level immutability policy either in locked or unlocked state,
* Account level versioning must be enabled and there should be no Legal hold on the container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
Mono objectLevelWormAsync(String resourceGroupName, String accountName, String containerName);
/**
* This operation migrates a blob container from container level WORM to object level immutability enabled
* container. Prerequisites require a container level immutability policy either in locked or unlocked state,
* Account level versioning must be enabled and there should be no Legal hold on the container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void objectLevelWorm(String resourceGroupName, String accountName, String containerName);
/**
* This operation migrates a blob container from container level WORM to object level immutability enabled
* container. Prerequisites require a container level immutability policy either in locked or unlocked state,
* Account level versioning must be enabled and there should be no Legal hold on the container.
*
* @param resourceGroupName The name of the resource group within the user's subscription. The name is case
* insensitive.
* @param accountName The name of the storage account within the specified resource group. Storage account names
* must be between 3 and 24 characters in length and use numbers and lower-case letters only.
* @param containerName The name of the blob container within the specified storage account. Blob container names
* must be between 3 and 63 characters in length and use numbers, lower-case letters and dash (-) only. Every dash
* (-) character must be immediately preceded and followed by a letter or number.
* @param context The context to associate with this operation.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws com.azure.core.management.exception.ManagementException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
void objectLevelWorm(String resourceGroupName, String accountName, String containerName, Context context);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy