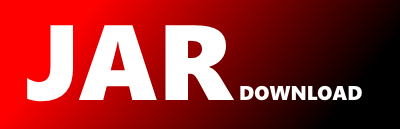
com.azure.resourcemanager.storage.models.ManagementPolicyVersion Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-storage Show documentation
Show all versions of azure-resourcemanager-storage Show documentation
This package contains Microsoft Azure Storage Management SDK.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.storage.models;
import com.azure.core.annotation.Fluent;
import com.fasterxml.jackson.annotation.JsonProperty;
/**
* Management policy action for blob version.
*/
@Fluent
public final class ManagementPolicyVersion {
/*
* The function to tier blob version to cool storage.
*/
@JsonProperty(value = "tierToCool")
private DateAfterCreation tierToCool;
/*
* The function to tier blob version to archive storage.
*/
@JsonProperty(value = "tierToArchive")
private DateAfterCreation tierToArchive;
/*
* The function to tier blobs to cold storage.
*/
@JsonProperty(value = "tierToCold")
private DateAfterCreation tierToCold;
/*
* The function to tier blobs to hot storage. This action can only be used with Premium Block Blob Storage Accounts
*/
@JsonProperty(value = "tierToHot")
private DateAfterCreation tierToHot;
/*
* The function to delete the blob version
*/
@JsonProperty(value = "delete")
private DateAfterCreation delete;
/**
* Creates an instance of ManagementPolicyVersion class.
*/
public ManagementPolicyVersion() {
}
/**
* Get the tierToCool property: The function to tier blob version to cool storage.
*
* @return the tierToCool value.
*/
public DateAfterCreation tierToCool() {
return this.tierToCool;
}
/**
* Set the tierToCool property: The function to tier blob version to cool storage.
*
* @param tierToCool the tierToCool value to set.
* @return the ManagementPolicyVersion object itself.
*/
public ManagementPolicyVersion withTierToCool(DateAfterCreation tierToCool) {
this.tierToCool = tierToCool;
return this;
}
/**
* Get the tierToArchive property: The function to tier blob version to archive storage.
*
* @return the tierToArchive value.
*/
public DateAfterCreation tierToArchive() {
return this.tierToArchive;
}
/**
* Set the tierToArchive property: The function to tier blob version to archive storage.
*
* @param tierToArchive the tierToArchive value to set.
* @return the ManagementPolicyVersion object itself.
*/
public ManagementPolicyVersion withTierToArchive(DateAfterCreation tierToArchive) {
this.tierToArchive = tierToArchive;
return this;
}
/**
* Get the tierToCold property: The function to tier blobs to cold storage.
*
* @return the tierToCold value.
*/
public DateAfterCreation tierToCold() {
return this.tierToCold;
}
/**
* Set the tierToCold property: The function to tier blobs to cold storage.
*
* @param tierToCold the tierToCold value to set.
* @return the ManagementPolicyVersion object itself.
*/
public ManagementPolicyVersion withTierToCold(DateAfterCreation tierToCold) {
this.tierToCold = tierToCold;
return this;
}
/**
* Get the tierToHot property: The function to tier blobs to hot storage. This action can only be used with Premium
* Block Blob Storage Accounts.
*
* @return the tierToHot value.
*/
public DateAfterCreation tierToHot() {
return this.tierToHot;
}
/**
* Set the tierToHot property: The function to tier blobs to hot storage. This action can only be used with Premium
* Block Blob Storage Accounts.
*
* @param tierToHot the tierToHot value to set.
* @return the ManagementPolicyVersion object itself.
*/
public ManagementPolicyVersion withTierToHot(DateAfterCreation tierToHot) {
this.tierToHot = tierToHot;
return this;
}
/**
* Get the delete property: The function to delete the blob version.
*
* @return the delete value.
*/
public DateAfterCreation delete() {
return this.delete;
}
/**
* Set the delete property: The function to delete the blob version.
*
* @param delete the delete value to set.
* @return the ManagementPolicyVersion object itself.
*/
public ManagementPolicyVersion withDelete(DateAfterCreation delete) {
this.delete = delete;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (tierToCool() != null) {
tierToCool().validate();
}
if (tierToArchive() != null) {
tierToArchive().validate();
}
if (tierToCold() != null) {
tierToCold().validate();
}
if (tierToHot() != null) {
tierToHot().validate();
}
if (delete() != null) {
delete().validate();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy