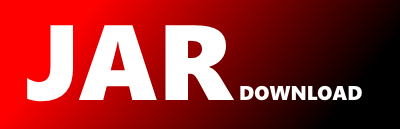
com.azure.resourcemanager.storage.models.StorageAccountUpdateParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-storage Show documentation
Show all versions of azure-resourcemanager-storage Show documentation
This package contains Microsoft Azure Storage Management SDK.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.storage.models;
import com.azure.core.annotation.Fluent;
import com.azure.resourcemanager.storage.fluent.models.StorageAccountPropertiesUpdateParameters;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.Map;
/**
* The parameters that can be provided when updating the storage account properties.
*/
@Fluent
public final class StorageAccountUpdateParameters {
/*
* Gets or sets the SKU name. Note that the SKU name cannot be updated to Standard_ZRS, Premium_LRS or Premium_ZRS,
* nor can accounts of those SKU names be updated to any other value.
*/
@JsonProperty(value = "sku")
private Sku sku;
/*
* Gets or sets a list of key value pairs that describe the resource. These tags can be used in viewing and grouping
* this resource (across resource groups). A maximum of 15 tags can be provided for a resource. Each tag must have a
* key no greater in length than 128 characters and a value no greater in length than 256 characters.
*/
@JsonProperty(value = "tags")
@JsonInclude(value = JsonInclude.Include.NON_NULL, content = JsonInclude.Include.ALWAYS)
private Map tags;
/*
* The identity of the resource.
*/
@JsonProperty(value = "identity")
private Identity identity;
/*
* The parameters used when updating a storage account.
*/
@JsonProperty(value = "properties")
private StorageAccountPropertiesUpdateParameters innerProperties;
/*
* Optional. Indicates the type of storage account. Currently only StorageV2 value supported by server.
*/
@JsonProperty(value = "kind")
private Kind kind;
/**
* Creates an instance of StorageAccountUpdateParameters class.
*/
public StorageAccountUpdateParameters() {
}
/**
* Get the sku property: Gets or sets the SKU name. Note that the SKU name cannot be updated to Standard_ZRS,
* Premium_LRS or Premium_ZRS, nor can accounts of those SKU names be updated to any other value.
*
* @return the sku value.
*/
public Sku sku() {
return this.sku;
}
/**
* Set the sku property: Gets or sets the SKU name. Note that the SKU name cannot be updated to Standard_ZRS,
* Premium_LRS or Premium_ZRS, nor can accounts of those SKU names be updated to any other value.
*
* @param sku the sku value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withSku(Sku sku) {
this.sku = sku;
return this;
}
/**
* Get the tags property: Gets or sets a list of key value pairs that describe the resource. These tags can be used
* in viewing and grouping this resource (across resource groups). A maximum of 15 tags can be provided for a
* resource. Each tag must have a key no greater in length than 128 characters and a value no greater in length than
* 256 characters.
*
* @return the tags value.
*/
public Map tags() {
return this.tags;
}
/**
* Set the tags property: Gets or sets a list of key value pairs that describe the resource. These tags can be used
* in viewing and grouping this resource (across resource groups). A maximum of 15 tags can be provided for a
* resource. Each tag must have a key no greater in length than 128 characters and a value no greater in length than
* 256 characters.
*
* @param tags the tags value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withTags(Map tags) {
this.tags = tags;
return this;
}
/**
* Get the identity property: The identity of the resource.
*
* @return the identity value.
*/
public Identity identity() {
return this.identity;
}
/**
* Set the identity property: The identity of the resource.
*
* @param identity the identity value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withIdentity(Identity identity) {
this.identity = identity;
return this;
}
/**
* Get the innerProperties property: The parameters used when updating a storage account.
*
* @return the innerProperties value.
*/
private StorageAccountPropertiesUpdateParameters innerProperties() {
return this.innerProperties;
}
/**
* Get the kind property: Optional. Indicates the type of storage account. Currently only StorageV2 value supported
* by server.
*
* @return the kind value.
*/
public Kind kind() {
return this.kind;
}
/**
* Set the kind property: Optional. Indicates the type of storage account. Currently only StorageV2 value supported
* by server.
*
* @param kind the kind value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withKind(Kind kind) {
this.kind = kind;
return this;
}
/**
* Get the customDomain property: Custom domain assigned to the storage account by the user. Name is the CNAME
* source. Only one custom domain is supported per storage account at this time. To clear the existing custom
* domain, use an empty string for the custom domain name property.
*
* @return the customDomain value.
*/
public CustomDomain customDomain() {
return this.innerProperties() == null ? null : this.innerProperties().customDomain();
}
/**
* Set the customDomain property: Custom domain assigned to the storage account by the user. Name is the CNAME
* source. Only one custom domain is supported per storage account at this time. To clear the existing custom
* domain, use an empty string for the custom domain name property.
*
* @param customDomain the customDomain value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withCustomDomain(CustomDomain customDomain) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withCustomDomain(customDomain);
return this;
}
/**
* Get the encryption property: Not applicable. Azure Storage encryption at rest is enabled by default for all
* storage accounts and cannot be disabled.
*
* @return the encryption value.
*/
public Encryption encryption() {
return this.innerProperties() == null ? null : this.innerProperties().encryption();
}
/**
* Set the encryption property: Not applicable. Azure Storage encryption at rest is enabled by default for all
* storage accounts and cannot be disabled.
*
* @param encryption the encryption value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withEncryption(Encryption encryption) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withEncryption(encryption);
return this;
}
/**
* Get the sasPolicy property: SasPolicy assigned to the storage account.
*
* @return the sasPolicy value.
*/
public SasPolicy sasPolicy() {
return this.innerProperties() == null ? null : this.innerProperties().sasPolicy();
}
/**
* Set the sasPolicy property: SasPolicy assigned to the storage account.
*
* @param sasPolicy the sasPolicy value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withSasPolicy(SasPolicy sasPolicy) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withSasPolicy(sasPolicy);
return this;
}
/**
* Get the keyPolicy property: KeyPolicy assigned to the storage account.
*
* @return the keyPolicy value.
*/
public KeyPolicy keyPolicy() {
return this.innerProperties() == null ? null : this.innerProperties().keyPolicy();
}
/**
* Set the keyPolicy property: KeyPolicy assigned to the storage account.
*
* @param keyPolicy the keyPolicy value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withKeyPolicy(KeyPolicy keyPolicy) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withKeyPolicy(keyPolicy);
return this;
}
/**
* Get the accessTier property: Required for storage accounts where kind = BlobStorage. The access tier is used for
* billing. The 'Premium' access tier is the default value for premium block blobs storage account type and it
* cannot be changed for the premium block blobs storage account type.
*
* @return the accessTier value.
*/
public AccessTier accessTier() {
return this.innerProperties() == null ? null : this.innerProperties().accessTier();
}
/**
* Set the accessTier property: Required for storage accounts where kind = BlobStorage. The access tier is used for
* billing. The 'Premium' access tier is the default value for premium block blobs storage account type and it
* cannot be changed for the premium block blobs storage account type.
*
* @param accessTier the accessTier value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withAccessTier(AccessTier accessTier) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withAccessTier(accessTier);
return this;
}
/**
* Get the azureFilesIdentityBasedAuthentication property: Provides the identity based authentication settings for
* Azure Files.
*
* @return the azureFilesIdentityBasedAuthentication value.
*/
public AzureFilesIdentityBasedAuthentication azureFilesIdentityBasedAuthentication() {
return this.innerProperties() == null ? null : this.innerProperties().azureFilesIdentityBasedAuthentication();
}
/**
* Set the azureFilesIdentityBasedAuthentication property: Provides the identity based authentication settings for
* Azure Files.
*
* @param azureFilesIdentityBasedAuthentication the azureFilesIdentityBasedAuthentication value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withAzureFilesIdentityBasedAuthentication(
AzureFilesIdentityBasedAuthentication azureFilesIdentityBasedAuthentication) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withAzureFilesIdentityBasedAuthentication(azureFilesIdentityBasedAuthentication);
return this;
}
/**
* Get the enableHttpsTrafficOnly property: Allows https traffic only to storage service if sets to true.
*
* @return the enableHttpsTrafficOnly value.
*/
public Boolean enableHttpsTrafficOnly() {
return this.innerProperties() == null ? null : this.innerProperties().enableHttpsTrafficOnly();
}
/**
* Set the enableHttpsTrafficOnly property: Allows https traffic only to storage service if sets to true.
*
* @param enableHttpsTrafficOnly the enableHttpsTrafficOnly value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withEnableHttpsTrafficOnly(Boolean enableHttpsTrafficOnly) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withEnableHttpsTrafficOnly(enableHttpsTrafficOnly);
return this;
}
/**
* Get the isSftpEnabled property: Enables Secure File Transfer Protocol, if set to true.
*
* @return the isSftpEnabled value.
*/
public Boolean isSftpEnabled() {
return this.innerProperties() == null ? null : this.innerProperties().isSftpEnabled();
}
/**
* Set the isSftpEnabled property: Enables Secure File Transfer Protocol, if set to true.
*
* @param isSftpEnabled the isSftpEnabled value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withIsSftpEnabled(Boolean isSftpEnabled) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withIsSftpEnabled(isSftpEnabled);
return this;
}
/**
* Get the isLocalUserEnabled property: Enables local users feature, if set to true.
*
* @return the isLocalUserEnabled value.
*/
public Boolean isLocalUserEnabled() {
return this.innerProperties() == null ? null : this.innerProperties().isLocalUserEnabled();
}
/**
* Set the isLocalUserEnabled property: Enables local users feature, if set to true.
*
* @param isLocalUserEnabled the isLocalUserEnabled value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withIsLocalUserEnabled(Boolean isLocalUserEnabled) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withIsLocalUserEnabled(isLocalUserEnabled);
return this;
}
/**
* Get the enableExtendedGroups property: Enables extended group support with local users feature, if set to true.
*
* @return the enableExtendedGroups value.
*/
public Boolean enableExtendedGroups() {
return this.innerProperties() == null ? null : this.innerProperties().enableExtendedGroups();
}
/**
* Set the enableExtendedGroups property: Enables extended group support with local users feature, if set to true.
*
* @param enableExtendedGroups the enableExtendedGroups value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withEnableExtendedGroups(Boolean enableExtendedGroups) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withEnableExtendedGroups(enableExtendedGroups);
return this;
}
/**
* Get the networkRuleSet property: Network rule set.
*
* @return the networkRuleSet value.
*/
public NetworkRuleSet networkRuleSet() {
return this.innerProperties() == null ? null : this.innerProperties().networkRuleSet();
}
/**
* Set the networkRuleSet property: Network rule set.
*
* @param networkRuleSet the networkRuleSet value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withNetworkRuleSet(NetworkRuleSet networkRuleSet) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withNetworkRuleSet(networkRuleSet);
return this;
}
/**
* Get the largeFileSharesState property: Allow large file shares if sets to Enabled. It cannot be disabled once it
* is enabled.
*
* @return the largeFileSharesState value.
*/
public LargeFileSharesState largeFileSharesState() {
return this.innerProperties() == null ? null : this.innerProperties().largeFileSharesState();
}
/**
* Set the largeFileSharesState property: Allow large file shares if sets to Enabled. It cannot be disabled once it
* is enabled.
*
* @param largeFileSharesState the largeFileSharesState value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withLargeFileSharesState(LargeFileSharesState largeFileSharesState) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withLargeFileSharesState(largeFileSharesState);
return this;
}
/**
* Get the routingPreference property: Maintains information about the network routing choice opted by the user for
* data transfer.
*
* @return the routingPreference value.
*/
public RoutingPreference routingPreference() {
return this.innerProperties() == null ? null : this.innerProperties().routingPreference();
}
/**
* Set the routingPreference property: Maintains information about the network routing choice opted by the user for
* data transfer.
*
* @param routingPreference the routingPreference value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withRoutingPreference(RoutingPreference routingPreference) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withRoutingPreference(routingPreference);
return this;
}
/**
* Get the allowBlobPublicAccess property: Allow or disallow public access to all blobs or containers in the storage
* account. The default interpretation is false for this property.
*
* @return the allowBlobPublicAccess value.
*/
public Boolean allowBlobPublicAccess() {
return this.innerProperties() == null ? null : this.innerProperties().allowBlobPublicAccess();
}
/**
* Set the allowBlobPublicAccess property: Allow or disallow public access to all blobs or containers in the storage
* account. The default interpretation is false for this property.
*
* @param allowBlobPublicAccess the allowBlobPublicAccess value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withAllowBlobPublicAccess(Boolean allowBlobPublicAccess) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withAllowBlobPublicAccess(allowBlobPublicAccess);
return this;
}
/**
* Get the minimumTlsVersion property: Set the minimum TLS version to be permitted on requests to storage. The
* default interpretation is TLS 1.0 for this property.
*
* @return the minimumTlsVersion value.
*/
public MinimumTlsVersion minimumTlsVersion() {
return this.innerProperties() == null ? null : this.innerProperties().minimumTlsVersion();
}
/**
* Set the minimumTlsVersion property: Set the minimum TLS version to be permitted on requests to storage. The
* default interpretation is TLS 1.0 for this property.
*
* @param minimumTlsVersion the minimumTlsVersion value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withMinimumTlsVersion(MinimumTlsVersion minimumTlsVersion) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withMinimumTlsVersion(minimumTlsVersion);
return this;
}
/**
* Get the allowSharedKeyAccess property: Indicates whether the storage account permits requests to be authorized
* with the account access key via Shared Key. If false, then all requests, including shared access signatures, must
* be authorized with Azure Active Directory (Azure AD). The default value is null, which is equivalent to true.
*
* @return the allowSharedKeyAccess value.
*/
public Boolean allowSharedKeyAccess() {
return this.innerProperties() == null ? null : this.innerProperties().allowSharedKeyAccess();
}
/**
* Set the allowSharedKeyAccess property: Indicates whether the storage account permits requests to be authorized
* with the account access key via Shared Key. If false, then all requests, including shared access signatures, must
* be authorized with Azure Active Directory (Azure AD). The default value is null, which is equivalent to true.
*
* @param allowSharedKeyAccess the allowSharedKeyAccess value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withAllowSharedKeyAccess(Boolean allowSharedKeyAccess) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withAllowSharedKeyAccess(allowSharedKeyAccess);
return this;
}
/**
* Get the allowCrossTenantReplication property: Allow or disallow cross AAD tenant object replication. Set this
* property to true for new or existing accounts only if object replication policies will involve storage accounts
* in different AAD tenants. The default interpretation is false for new accounts to follow best security practices
* by default.
*
* @return the allowCrossTenantReplication value.
*/
public Boolean allowCrossTenantReplication() {
return this.innerProperties() == null ? null : this.innerProperties().allowCrossTenantReplication();
}
/**
* Set the allowCrossTenantReplication property: Allow or disallow cross AAD tenant object replication. Set this
* property to true for new or existing accounts only if object replication policies will involve storage accounts
* in different AAD tenants. The default interpretation is false for new accounts to follow best security practices
* by default.
*
* @param allowCrossTenantReplication the allowCrossTenantReplication value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withAllowCrossTenantReplication(Boolean allowCrossTenantReplication) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withAllowCrossTenantReplication(allowCrossTenantReplication);
return this;
}
/**
* Get the defaultToOAuthAuthentication property: A boolean flag which indicates whether the default authentication
* is OAuth or not. The default interpretation is false for this property.
*
* @return the defaultToOAuthAuthentication value.
*/
public Boolean defaultToOAuthAuthentication() {
return this.innerProperties() == null ? null : this.innerProperties().defaultToOAuthAuthentication();
}
/**
* Set the defaultToOAuthAuthentication property: A boolean flag which indicates whether the default authentication
* is OAuth or not. The default interpretation is false for this property.
*
* @param defaultToOAuthAuthentication the defaultToOAuthAuthentication value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withDefaultToOAuthAuthentication(Boolean defaultToOAuthAuthentication) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withDefaultToOAuthAuthentication(defaultToOAuthAuthentication);
return this;
}
/**
* Get the publicNetworkAccess property: Allow, disallow, or let Network Security Perimeter configuration to
* evaluate public network access to Storage Account. Value is optional but if passed in, must be 'Enabled',
* 'Disabled' or 'SecuredByPerimeter'.
*
* @return the publicNetworkAccess value.
*/
public PublicNetworkAccess publicNetworkAccess() {
return this.innerProperties() == null ? null : this.innerProperties().publicNetworkAccess();
}
/**
* Set the publicNetworkAccess property: Allow, disallow, or let Network Security Perimeter configuration to
* evaluate public network access to Storage Account. Value is optional but if passed in, must be 'Enabled',
* 'Disabled' or 'SecuredByPerimeter'.
*
* @param publicNetworkAccess the publicNetworkAccess value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withPublicNetworkAccess(PublicNetworkAccess publicNetworkAccess) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withPublicNetworkAccess(publicNetworkAccess);
return this;
}
/**
* Get the immutableStorageWithVersioning property: The property is immutable and can only be set to true at the
* account creation time. When set to true, it enables object level immutability for all the containers in the
* account by default.
*
* @return the immutableStorageWithVersioning value.
*/
public ImmutableStorageAccount immutableStorageWithVersioning() {
return this.innerProperties() == null ? null : this.innerProperties().immutableStorageWithVersioning();
}
/**
* Set the immutableStorageWithVersioning property: The property is immutable and can only be set to true at the
* account creation time. When set to true, it enables object level immutability for all the containers in the
* account by default.
*
* @param immutableStorageWithVersioning the immutableStorageWithVersioning value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters
withImmutableStorageWithVersioning(ImmutableStorageAccount immutableStorageWithVersioning) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withImmutableStorageWithVersioning(immutableStorageWithVersioning);
return this;
}
/**
* Get the allowedCopyScope property: Restrict copy to and from Storage Accounts within an AAD tenant or with
* Private Links to the same VNet.
*
* @return the allowedCopyScope value.
*/
public AllowedCopyScope allowedCopyScope() {
return this.innerProperties() == null ? null : this.innerProperties().allowedCopyScope();
}
/**
* Set the allowedCopyScope property: Restrict copy to and from Storage Accounts within an AAD tenant or with
* Private Links to the same VNet.
*
* @param allowedCopyScope the allowedCopyScope value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withAllowedCopyScope(AllowedCopyScope allowedCopyScope) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withAllowedCopyScope(allowedCopyScope);
return this;
}
/**
* Get the dnsEndpointType property: Allows you to specify the type of endpoint. Set this to AzureDNSZone to create
* a large number of accounts in a single subscription, which creates accounts in an Azure DNS Zone and the endpoint
* URL will have an alphanumeric DNS Zone identifier.
*
* @return the dnsEndpointType value.
*/
public DnsEndpointType dnsEndpointType() {
return this.innerProperties() == null ? null : this.innerProperties().dnsEndpointType();
}
/**
* Set the dnsEndpointType property: Allows you to specify the type of endpoint. Set this to AzureDNSZone to create
* a large number of accounts in a single subscription, which creates accounts in an Azure DNS Zone and the endpoint
* URL will have an alphanumeric DNS Zone identifier.
*
* @param dnsEndpointType the dnsEndpointType value to set.
* @return the StorageAccountUpdateParameters object itself.
*/
public StorageAccountUpdateParameters withDnsEndpointType(DnsEndpointType dnsEndpointType) {
if (this.innerProperties() == null) {
this.innerProperties = new StorageAccountPropertiesUpdateParameters();
}
this.innerProperties().withDnsEndpointType(dnsEndpointType);
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (sku() != null) {
sku().validate();
}
if (identity() != null) {
identity().validate();
}
if (innerProperties() != null) {
innerProperties().validate();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy