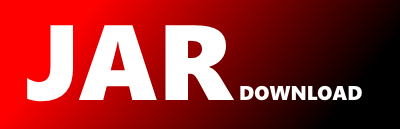
com.azure.resourcemanager.storage.models.ManagementPolicyVersion Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-storage Show documentation
Show all versions of azure-resourcemanager-storage Show documentation
This package contains Microsoft Azure Storage Management SDK.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.storage.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Management policy action for blob version.
*/
@Fluent
public final class ManagementPolicyVersion implements JsonSerializable {
/*
* The function to tier blob version to cool storage.
*/
private DateAfterCreation tierToCool;
/*
* The function to tier blob version to archive storage.
*/
private DateAfterCreation tierToArchive;
/*
* The function to tier blobs to cold storage.
*/
private DateAfterCreation tierToCold;
/*
* The function to tier blobs to hot storage. This action can only be used with Premium Block Blob Storage Accounts
*/
private DateAfterCreation tierToHot;
/*
* The function to delete the blob version
*/
private DateAfterCreation delete;
/**
* Creates an instance of ManagementPolicyVersion class.
*/
public ManagementPolicyVersion() {
}
/**
* Get the tierToCool property: The function to tier blob version to cool storage.
*
* @return the tierToCool value.
*/
public DateAfterCreation tierToCool() {
return this.tierToCool;
}
/**
* Set the tierToCool property: The function to tier blob version to cool storage.
*
* @param tierToCool the tierToCool value to set.
* @return the ManagementPolicyVersion object itself.
*/
public ManagementPolicyVersion withTierToCool(DateAfterCreation tierToCool) {
this.tierToCool = tierToCool;
return this;
}
/**
* Get the tierToArchive property: The function to tier blob version to archive storage.
*
* @return the tierToArchive value.
*/
public DateAfterCreation tierToArchive() {
return this.tierToArchive;
}
/**
* Set the tierToArchive property: The function to tier blob version to archive storage.
*
* @param tierToArchive the tierToArchive value to set.
* @return the ManagementPolicyVersion object itself.
*/
public ManagementPolicyVersion withTierToArchive(DateAfterCreation tierToArchive) {
this.tierToArchive = tierToArchive;
return this;
}
/**
* Get the tierToCold property: The function to tier blobs to cold storage.
*
* @return the tierToCold value.
*/
public DateAfterCreation tierToCold() {
return this.tierToCold;
}
/**
* Set the tierToCold property: The function to tier blobs to cold storage.
*
* @param tierToCold the tierToCold value to set.
* @return the ManagementPolicyVersion object itself.
*/
public ManagementPolicyVersion withTierToCold(DateAfterCreation tierToCold) {
this.tierToCold = tierToCold;
return this;
}
/**
* Get the tierToHot property: The function to tier blobs to hot storage. This action can only be used with Premium
* Block Blob Storage Accounts.
*
* @return the tierToHot value.
*/
public DateAfterCreation tierToHot() {
return this.tierToHot;
}
/**
* Set the tierToHot property: The function to tier blobs to hot storage. This action can only be used with Premium
* Block Blob Storage Accounts.
*
* @param tierToHot the tierToHot value to set.
* @return the ManagementPolicyVersion object itself.
*/
public ManagementPolicyVersion withTierToHot(DateAfterCreation tierToHot) {
this.tierToHot = tierToHot;
return this;
}
/**
* Get the delete property: The function to delete the blob version.
*
* @return the delete value.
*/
public DateAfterCreation delete() {
return this.delete;
}
/**
* Set the delete property: The function to delete the blob version.
*
* @param delete the delete value to set.
* @return the ManagementPolicyVersion object itself.
*/
public ManagementPolicyVersion withDelete(DateAfterCreation delete) {
this.delete = delete;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (tierToCool() != null) {
tierToCool().validate();
}
if (tierToArchive() != null) {
tierToArchive().validate();
}
if (tierToCold() != null) {
tierToCold().validate();
}
if (tierToHot() != null) {
tierToHot().validate();
}
if (delete() != null) {
delete().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("tierToCool", this.tierToCool);
jsonWriter.writeJsonField("tierToArchive", this.tierToArchive);
jsonWriter.writeJsonField("tierToCold", this.tierToCold);
jsonWriter.writeJsonField("tierToHot", this.tierToHot);
jsonWriter.writeJsonField("delete", this.delete);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ManagementPolicyVersion from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ManagementPolicyVersion if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the ManagementPolicyVersion.
*/
public static ManagementPolicyVersion fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ManagementPolicyVersion deserializedManagementPolicyVersion = new ManagementPolicyVersion();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("tierToCool".equals(fieldName)) {
deserializedManagementPolicyVersion.tierToCool = DateAfterCreation.fromJson(reader);
} else if ("tierToArchive".equals(fieldName)) {
deserializedManagementPolicyVersion.tierToArchive = DateAfterCreation.fromJson(reader);
} else if ("tierToCold".equals(fieldName)) {
deserializedManagementPolicyVersion.tierToCold = DateAfterCreation.fromJson(reader);
} else if ("tierToHot".equals(fieldName)) {
deserializedManagementPolicyVersion.tierToHot = DateAfterCreation.fromJson(reader);
} else if ("delete".equals(fieldName)) {
deserializedManagementPolicyVersion.delete = DateAfterCreation.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedManagementPolicyVersion;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy