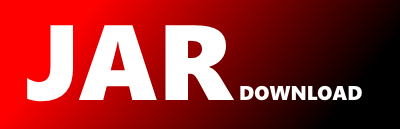
com.azure.resourcemanager.storage.models.StorageAccountMicrosoftEndpoints Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-storage Show documentation
Show all versions of azure-resourcemanager-storage Show documentation
This package contains Microsoft Azure Storage Management SDK.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.storage.models;
import com.azure.core.annotation.Immutable;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* The URIs that are used to perform a retrieval of a public blob, queue, table, web or dfs object via a microsoft
* routing endpoint.
*/
@Immutable
public final class StorageAccountMicrosoftEndpoints implements JsonSerializable {
/*
* Gets the blob endpoint.
*/
private String blob;
/*
* Gets the queue endpoint.
*/
private String queue;
/*
* Gets the table endpoint.
*/
private String table;
/*
* Gets the file endpoint.
*/
private String file;
/*
* Gets the web endpoint.
*/
private String web;
/*
* Gets the dfs endpoint.
*/
private String dfs;
/**
* Creates an instance of StorageAccountMicrosoftEndpoints class.
*/
public StorageAccountMicrosoftEndpoints() {
}
/**
* Get the blob property: Gets the blob endpoint.
*
* @return the blob value.
*/
public String blob() {
return this.blob;
}
/**
* Get the queue property: Gets the queue endpoint.
*
* @return the queue value.
*/
public String queue() {
return this.queue;
}
/**
* Get the table property: Gets the table endpoint.
*
* @return the table value.
*/
public String table() {
return this.table;
}
/**
* Get the file property: Gets the file endpoint.
*
* @return the file value.
*/
public String file() {
return this.file;
}
/**
* Get the web property: Gets the web endpoint.
*
* @return the web value.
*/
public String web() {
return this.web;
}
/**
* Get the dfs property: Gets the dfs endpoint.
*
* @return the dfs value.
*/
public String dfs() {
return this.dfs;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of StorageAccountMicrosoftEndpoints from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of StorageAccountMicrosoftEndpoints if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the StorageAccountMicrosoftEndpoints.
*/
public static StorageAccountMicrosoftEndpoints fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
StorageAccountMicrosoftEndpoints deserializedStorageAccountMicrosoftEndpoints
= new StorageAccountMicrosoftEndpoints();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("blob".equals(fieldName)) {
deserializedStorageAccountMicrosoftEndpoints.blob = reader.getString();
} else if ("queue".equals(fieldName)) {
deserializedStorageAccountMicrosoftEndpoints.queue = reader.getString();
} else if ("table".equals(fieldName)) {
deserializedStorageAccountMicrosoftEndpoints.table = reader.getString();
} else if ("file".equals(fieldName)) {
deserializedStorageAccountMicrosoftEndpoints.file = reader.getString();
} else if ("web".equals(fieldName)) {
deserializedStorageAccountMicrosoftEndpoints.web = reader.getString();
} else if ("dfs".equals(fieldName)) {
deserializedStorageAccountMicrosoftEndpoints.dfs = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedStorageAccountMicrosoftEndpoints;
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy