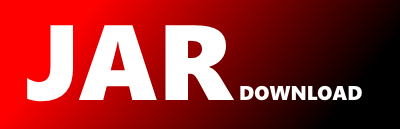
com.azure.resourcemanager.storagecache.fluent.models.CacheProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-storagecache Show documentation
Show all versions of azure-resourcemanager-storagecache Show documentation
This package contains Microsoft Azure SDK for StorageCache Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Azure Managed Lustre provides a fully managed Lustre® file system, integrated with Blob storage, for use on demand. These operations create and manage Azure Managed Lustre file systems. Package tag package-2024-03.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.storagecache.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.storagecache.models.CacheDirectorySettings;
import com.azure.resourcemanager.storagecache.models.CacheEncryptionSettings;
import com.azure.resourcemanager.storagecache.models.CacheHealth;
import com.azure.resourcemanager.storagecache.models.CacheNetworkSettings;
import com.azure.resourcemanager.storagecache.models.CacheSecuritySettings;
import com.azure.resourcemanager.storagecache.models.CacheUpgradeSettings;
import com.azure.resourcemanager.storagecache.models.CacheUpgradeStatus;
import com.azure.resourcemanager.storagecache.models.PrimingJob;
import com.azure.resourcemanager.storagecache.models.ProvisioningStateType;
import com.azure.resourcemanager.storagecache.models.StorageTargetSpaceAllocation;
import java.io.IOException;
import java.util.List;
/**
* Properties of the cache.
*/
@Fluent
public final class CacheProperties implements JsonSerializable {
/*
* The size of this Cache, in GB.
*/
private Integer cacheSizeGB;
/*
* Health of the cache.
*/
private CacheHealth health;
/*
* Array of IPv4 addresses that can be used by clients mounting this cache.
*/
private List mountAddresses;
/*
* ARM provisioning state, see
* https://github.com/Azure/azure-resource-manager-rpc/blob/master/v1.0/Addendum.md#provisioningstate-property
*/
private ProvisioningStateType provisioningState;
/*
* Subnet used for the cache.
*/
private String subnet;
/*
* Upgrade status of the cache.
*/
private CacheUpgradeStatus upgradeStatus;
/*
* Upgrade settings of the cache.
*/
private CacheUpgradeSettings upgradeSettings;
/*
* Specifies network settings of the cache.
*/
private CacheNetworkSettings networkSettings;
/*
* Specifies encryption settings of the cache.
*/
private CacheEncryptionSettings encryptionSettings;
/*
* Specifies security settings of the cache.
*/
private CacheSecuritySettings securitySettings;
/*
* Specifies Directory Services settings of the cache.
*/
private CacheDirectorySettings directoryServicesSettings;
/*
* Availability zones for resources. This field should only contain a single element in the array.
*/
private List zones;
/*
* Specifies the priming jobs defined in the cache.
*/
private List primingJobs;
/*
* Specifies the space allocation percentage for each storage target in the cache.
*/
private List spaceAllocation;
/**
* Creates an instance of CacheProperties class.
*/
public CacheProperties() {
}
/**
* Get the cacheSizeGB property: The size of this Cache, in GB.
*
* @return the cacheSizeGB value.
*/
public Integer cacheSizeGB() {
return this.cacheSizeGB;
}
/**
* Set the cacheSizeGB property: The size of this Cache, in GB.
*
* @param cacheSizeGB the cacheSizeGB value to set.
* @return the CacheProperties object itself.
*/
public CacheProperties withCacheSizeGB(Integer cacheSizeGB) {
this.cacheSizeGB = cacheSizeGB;
return this;
}
/**
* Get the health property: Health of the cache.
*
* @return the health value.
*/
public CacheHealth health() {
return this.health;
}
/**
* Get the mountAddresses property: Array of IPv4 addresses that can be used by clients mounting this cache.
*
* @return the mountAddresses value.
*/
public List mountAddresses() {
return this.mountAddresses;
}
/**
* Get the provisioningState property: ARM provisioning state, see
* https://github.com/Azure/azure-resource-manager-rpc/blob/master/v1.0/Addendum.md#provisioningstate-property.
*
* @return the provisioningState value.
*/
public ProvisioningStateType provisioningState() {
return this.provisioningState;
}
/**
* Get the subnet property: Subnet used for the cache.
*
* @return the subnet value.
*/
public String subnet() {
return this.subnet;
}
/**
* Set the subnet property: Subnet used for the cache.
*
* @param subnet the subnet value to set.
* @return the CacheProperties object itself.
*/
public CacheProperties withSubnet(String subnet) {
this.subnet = subnet;
return this;
}
/**
* Get the upgradeStatus property: Upgrade status of the cache.
*
* @return the upgradeStatus value.
*/
public CacheUpgradeStatus upgradeStatus() {
return this.upgradeStatus;
}
/**
* Get the upgradeSettings property: Upgrade settings of the cache.
*
* @return the upgradeSettings value.
*/
public CacheUpgradeSettings upgradeSettings() {
return this.upgradeSettings;
}
/**
* Set the upgradeSettings property: Upgrade settings of the cache.
*
* @param upgradeSettings the upgradeSettings value to set.
* @return the CacheProperties object itself.
*/
public CacheProperties withUpgradeSettings(CacheUpgradeSettings upgradeSettings) {
this.upgradeSettings = upgradeSettings;
return this;
}
/**
* Get the networkSettings property: Specifies network settings of the cache.
*
* @return the networkSettings value.
*/
public CacheNetworkSettings networkSettings() {
return this.networkSettings;
}
/**
* Set the networkSettings property: Specifies network settings of the cache.
*
* @param networkSettings the networkSettings value to set.
* @return the CacheProperties object itself.
*/
public CacheProperties withNetworkSettings(CacheNetworkSettings networkSettings) {
this.networkSettings = networkSettings;
return this;
}
/**
* Get the encryptionSettings property: Specifies encryption settings of the cache.
*
* @return the encryptionSettings value.
*/
public CacheEncryptionSettings encryptionSettings() {
return this.encryptionSettings;
}
/**
* Set the encryptionSettings property: Specifies encryption settings of the cache.
*
* @param encryptionSettings the encryptionSettings value to set.
* @return the CacheProperties object itself.
*/
public CacheProperties withEncryptionSettings(CacheEncryptionSettings encryptionSettings) {
this.encryptionSettings = encryptionSettings;
return this;
}
/**
* Get the securitySettings property: Specifies security settings of the cache.
*
* @return the securitySettings value.
*/
public CacheSecuritySettings securitySettings() {
return this.securitySettings;
}
/**
* Set the securitySettings property: Specifies security settings of the cache.
*
* @param securitySettings the securitySettings value to set.
* @return the CacheProperties object itself.
*/
public CacheProperties withSecuritySettings(CacheSecuritySettings securitySettings) {
this.securitySettings = securitySettings;
return this;
}
/**
* Get the directoryServicesSettings property: Specifies Directory Services settings of the cache.
*
* @return the directoryServicesSettings value.
*/
public CacheDirectorySettings directoryServicesSettings() {
return this.directoryServicesSettings;
}
/**
* Set the directoryServicesSettings property: Specifies Directory Services settings of the cache.
*
* @param directoryServicesSettings the directoryServicesSettings value to set.
* @return the CacheProperties object itself.
*/
public CacheProperties withDirectoryServicesSettings(CacheDirectorySettings directoryServicesSettings) {
this.directoryServicesSettings = directoryServicesSettings;
return this;
}
/**
* Get the zones property: Availability zones for resources. This field should only contain a single element in the
* array.
*
* @return the zones value.
*/
public List zones() {
return this.zones;
}
/**
* Set the zones property: Availability zones for resources. This field should only contain a single element in the
* array.
*
* @param zones the zones value to set.
* @return the CacheProperties object itself.
*/
public CacheProperties withZones(List zones) {
this.zones = zones;
return this;
}
/**
* Get the primingJobs property: Specifies the priming jobs defined in the cache.
*
* @return the primingJobs value.
*/
public List primingJobs() {
return this.primingJobs;
}
/**
* Get the spaceAllocation property: Specifies the space allocation percentage for each storage target in the cache.
*
* @return the spaceAllocation value.
*/
public List spaceAllocation() {
return this.spaceAllocation;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (health() != null) {
health().validate();
}
if (upgradeStatus() != null) {
upgradeStatus().validate();
}
if (upgradeSettings() != null) {
upgradeSettings().validate();
}
if (networkSettings() != null) {
networkSettings().validate();
}
if (encryptionSettings() != null) {
encryptionSettings().validate();
}
if (securitySettings() != null) {
securitySettings().validate();
}
if (directoryServicesSettings() != null) {
directoryServicesSettings().validate();
}
if (primingJobs() != null) {
primingJobs().forEach(e -> e.validate());
}
if (spaceAllocation() != null) {
spaceAllocation().forEach(e -> e.validate());
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeNumberField("cacheSizeGB", this.cacheSizeGB);
jsonWriter.writeStringField("subnet", this.subnet);
jsonWriter.writeJsonField("upgradeSettings", this.upgradeSettings);
jsonWriter.writeJsonField("networkSettings", this.networkSettings);
jsonWriter.writeJsonField("encryptionSettings", this.encryptionSettings);
jsonWriter.writeJsonField("securitySettings", this.securitySettings);
jsonWriter.writeJsonField("directoryServicesSettings", this.directoryServicesSettings);
jsonWriter.writeArrayField("zones", this.zones, (writer, element) -> writer.writeString(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of CacheProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of CacheProperties if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the CacheProperties.
*/
public static CacheProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
CacheProperties deserializedCacheProperties = new CacheProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("cacheSizeGB".equals(fieldName)) {
deserializedCacheProperties.cacheSizeGB = reader.getNullable(JsonReader::getInt);
} else if ("health".equals(fieldName)) {
deserializedCacheProperties.health = CacheHealth.fromJson(reader);
} else if ("mountAddresses".equals(fieldName)) {
List mountAddresses = reader.readArray(reader1 -> reader1.getString());
deserializedCacheProperties.mountAddresses = mountAddresses;
} else if ("provisioningState".equals(fieldName)) {
deserializedCacheProperties.provisioningState
= ProvisioningStateType.fromString(reader.getString());
} else if ("subnet".equals(fieldName)) {
deserializedCacheProperties.subnet = reader.getString();
} else if ("upgradeStatus".equals(fieldName)) {
deserializedCacheProperties.upgradeStatus = CacheUpgradeStatus.fromJson(reader);
} else if ("upgradeSettings".equals(fieldName)) {
deserializedCacheProperties.upgradeSettings = CacheUpgradeSettings.fromJson(reader);
} else if ("networkSettings".equals(fieldName)) {
deserializedCacheProperties.networkSettings = CacheNetworkSettings.fromJson(reader);
} else if ("encryptionSettings".equals(fieldName)) {
deserializedCacheProperties.encryptionSettings = CacheEncryptionSettings.fromJson(reader);
} else if ("securitySettings".equals(fieldName)) {
deserializedCacheProperties.securitySettings = CacheSecuritySettings.fromJson(reader);
} else if ("directoryServicesSettings".equals(fieldName)) {
deserializedCacheProperties.directoryServicesSettings = CacheDirectorySettings.fromJson(reader);
} else if ("zones".equals(fieldName)) {
List zones = reader.readArray(reader1 -> reader1.getString());
deserializedCacheProperties.zones = zones;
} else if ("primingJobs".equals(fieldName)) {
List primingJobs = reader.readArray(reader1 -> PrimingJob.fromJson(reader1));
deserializedCacheProperties.primingJobs = primingJobs;
} else if ("spaceAllocation".equals(fieldName)) {
List spaceAllocation
= reader.readArray(reader1 -> StorageTargetSpaceAllocation.fromJson(reader1));
deserializedCacheProperties.spaceAllocation = spaceAllocation;
} else {
reader.skipChildren();
}
}
return deserializedCacheProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy