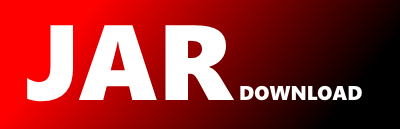
com.azure.resourcemanager.storagecache.models.AmlFilesystemRootSquashSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-storagecache Show documentation
Show all versions of azure-resourcemanager-storagecache Show documentation
This package contains Microsoft Azure SDK for StorageCache Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Azure Managed Lustre provides a fully managed Lustre® file system, integrated with Blob storage, for use on demand. These operations create and manage Azure Managed Lustre file systems. Package tag package-2024-03.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.storagecache.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* AML file system squash settings.
*/
@Fluent
public final class AmlFilesystemRootSquashSettings implements JsonSerializable {
/*
* Squash mode of the AML file system. 'All': User and Group IDs on files will be squashed to the provided values
* for all users on non-trusted systems. 'RootOnly': User and Group IDs on files will be squashed to provided values
* for solely the root user on non-trusted systems. 'None': No squashing of User and Group IDs is performed for any
* users on any systems.
*/
private AmlFilesystemSquashMode mode;
/*
* Semicolon separated NID IP Address list(s) to be added to the TrustedSystems.
*/
private String noSquashNidLists;
/*
* User ID to squash to.
*/
private Long squashUid;
/*
* Group ID to squash to.
*/
private Long squashGid;
/*
* AML file system squash status.
*/
private String status;
/**
* Creates an instance of AmlFilesystemRootSquashSettings class.
*/
public AmlFilesystemRootSquashSettings() {
}
/**
* Get the mode property: Squash mode of the AML file system. 'All': User and Group IDs on files will be squashed to
* the provided values for all users on non-trusted systems. 'RootOnly': User and Group IDs on files will be
* squashed to provided values for solely the root user on non-trusted systems. 'None': No squashing of User and
* Group IDs is performed for any users on any systems.
*
* @return the mode value.
*/
public AmlFilesystemSquashMode mode() {
return this.mode;
}
/**
* Set the mode property: Squash mode of the AML file system. 'All': User and Group IDs on files will be squashed to
* the provided values for all users on non-trusted systems. 'RootOnly': User and Group IDs on files will be
* squashed to provided values for solely the root user on non-trusted systems. 'None': No squashing of User and
* Group IDs is performed for any users on any systems.
*
* @param mode the mode value to set.
* @return the AmlFilesystemRootSquashSettings object itself.
*/
public AmlFilesystemRootSquashSettings withMode(AmlFilesystemSquashMode mode) {
this.mode = mode;
return this;
}
/**
* Get the noSquashNidLists property: Semicolon separated NID IP Address list(s) to be added to the TrustedSystems.
*
* @return the noSquashNidLists value.
*/
public String noSquashNidLists() {
return this.noSquashNidLists;
}
/**
* Set the noSquashNidLists property: Semicolon separated NID IP Address list(s) to be added to the TrustedSystems.
*
* @param noSquashNidLists the noSquashNidLists value to set.
* @return the AmlFilesystemRootSquashSettings object itself.
*/
public AmlFilesystemRootSquashSettings withNoSquashNidLists(String noSquashNidLists) {
this.noSquashNidLists = noSquashNidLists;
return this;
}
/**
* Get the squashUid property: User ID to squash to.
*
* @return the squashUid value.
*/
public Long squashUid() {
return this.squashUid;
}
/**
* Set the squashUid property: User ID to squash to.
*
* @param squashUid the squashUid value to set.
* @return the AmlFilesystemRootSquashSettings object itself.
*/
public AmlFilesystemRootSquashSettings withSquashUid(Long squashUid) {
this.squashUid = squashUid;
return this;
}
/**
* Get the squashGid property: Group ID to squash to.
*
* @return the squashGid value.
*/
public Long squashGid() {
return this.squashGid;
}
/**
* Set the squashGid property: Group ID to squash to.
*
* @param squashGid the squashGid value to set.
* @return the AmlFilesystemRootSquashSettings object itself.
*/
public AmlFilesystemRootSquashSettings withSquashGid(Long squashGid) {
this.squashGid = squashGid;
return this;
}
/**
* Get the status property: AML file system squash status.
*
* @return the status value.
*/
public String status() {
return this.status;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("mode", this.mode == null ? null : this.mode.toString());
jsonWriter.writeStringField("noSquashNidLists", this.noSquashNidLists);
jsonWriter.writeNumberField("squashUID", this.squashUid);
jsonWriter.writeNumberField("squashGID", this.squashGid);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of AmlFilesystemRootSquashSettings from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of AmlFilesystemRootSquashSettings if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the AmlFilesystemRootSquashSettings.
*/
public static AmlFilesystemRootSquashSettings fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
AmlFilesystemRootSquashSettings deserializedAmlFilesystemRootSquashSettings
= new AmlFilesystemRootSquashSettings();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("mode".equals(fieldName)) {
deserializedAmlFilesystemRootSquashSettings.mode
= AmlFilesystemSquashMode.fromString(reader.getString());
} else if ("noSquashNidLists".equals(fieldName)) {
deserializedAmlFilesystemRootSquashSettings.noSquashNidLists = reader.getString();
} else if ("squashUID".equals(fieldName)) {
deserializedAmlFilesystemRootSquashSettings.squashUid = reader.getNullable(JsonReader::getLong);
} else if ("squashGID".equals(fieldName)) {
deserializedAmlFilesystemRootSquashSettings.squashGid = reader.getNullable(JsonReader::getLong);
} else if ("status".equals(fieldName)) {
deserializedAmlFilesystemRootSquashSettings.status = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedAmlFilesystemRootSquashSettings;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy