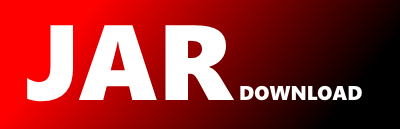
com.azure.resourcemanager.storagecache.models.CacheNetworkSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-storagecache Show documentation
Show all versions of azure-resourcemanager-storagecache Show documentation
This package contains Microsoft Azure SDK for StorageCache Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Azure Managed Lustre provides a fully managed Lustre® file system, integrated with Blob storage, for use on demand. These operations create and manage Azure Managed Lustre file systems. Package tag package-2024-03.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.storagecache.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* Cache network settings.
*/
@Fluent
public final class CacheNetworkSettings implements JsonSerializable {
/*
* The IPv4 maximum transmission unit configured for the subnet.
*/
private Integer mtu;
/*
* Array of additional IP addresses used by this cache.
*/
private List utilityAddresses;
/*
* DNS servers for the cache to use. It will be set from the network configuration if no value is provided.
*/
private List dnsServers;
/*
* DNS search domain
*/
private String dnsSearchDomain;
/*
* NTP server IP Address or FQDN for the cache to use. The default is time.windows.com.
*/
private String ntpServer;
/**
* Creates an instance of CacheNetworkSettings class.
*/
public CacheNetworkSettings() {
}
/**
* Get the mtu property: The IPv4 maximum transmission unit configured for the subnet.
*
* @return the mtu value.
*/
public Integer mtu() {
return this.mtu;
}
/**
* Set the mtu property: The IPv4 maximum transmission unit configured for the subnet.
*
* @param mtu the mtu value to set.
* @return the CacheNetworkSettings object itself.
*/
public CacheNetworkSettings withMtu(Integer mtu) {
this.mtu = mtu;
return this;
}
/**
* Get the utilityAddresses property: Array of additional IP addresses used by this cache.
*
* @return the utilityAddresses value.
*/
public List utilityAddresses() {
return this.utilityAddresses;
}
/**
* Get the dnsServers property: DNS servers for the cache to use. It will be set from the network configuration if
* no value is provided.
*
* @return the dnsServers value.
*/
public List dnsServers() {
return this.dnsServers;
}
/**
* Set the dnsServers property: DNS servers for the cache to use. It will be set from the network configuration if
* no value is provided.
*
* @param dnsServers the dnsServers value to set.
* @return the CacheNetworkSettings object itself.
*/
public CacheNetworkSettings withDnsServers(List dnsServers) {
this.dnsServers = dnsServers;
return this;
}
/**
* Get the dnsSearchDomain property: DNS search domain.
*
* @return the dnsSearchDomain value.
*/
public String dnsSearchDomain() {
return this.dnsSearchDomain;
}
/**
* Set the dnsSearchDomain property: DNS search domain.
*
* @param dnsSearchDomain the dnsSearchDomain value to set.
* @return the CacheNetworkSettings object itself.
*/
public CacheNetworkSettings withDnsSearchDomain(String dnsSearchDomain) {
this.dnsSearchDomain = dnsSearchDomain;
return this;
}
/**
* Get the ntpServer property: NTP server IP Address or FQDN for the cache to use. The default is time.windows.com.
*
* @return the ntpServer value.
*/
public String ntpServer() {
return this.ntpServer;
}
/**
* Set the ntpServer property: NTP server IP Address or FQDN for the cache to use. The default is time.windows.com.
*
* @param ntpServer the ntpServer value to set.
* @return the CacheNetworkSettings object itself.
*/
public CacheNetworkSettings withNtpServer(String ntpServer) {
this.ntpServer = ntpServer;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeNumberField("mtu", this.mtu);
jsonWriter.writeArrayField("dnsServers", this.dnsServers, (writer, element) -> writer.writeString(element));
jsonWriter.writeStringField("dnsSearchDomain", this.dnsSearchDomain);
jsonWriter.writeStringField("ntpServer", this.ntpServer);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of CacheNetworkSettings from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of CacheNetworkSettings if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IOException If an error occurs while reading the CacheNetworkSettings.
*/
public static CacheNetworkSettings fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
CacheNetworkSettings deserializedCacheNetworkSettings = new CacheNetworkSettings();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("mtu".equals(fieldName)) {
deserializedCacheNetworkSettings.mtu = reader.getNullable(JsonReader::getInt);
} else if ("utilityAddresses".equals(fieldName)) {
List utilityAddresses = reader.readArray(reader1 -> reader1.getString());
deserializedCacheNetworkSettings.utilityAddresses = utilityAddresses;
} else if ("dnsServers".equals(fieldName)) {
List dnsServers = reader.readArray(reader1 -> reader1.getString());
deserializedCacheNetworkSettings.dnsServers = dnsServers;
} else if ("dnsSearchDomain".equals(fieldName)) {
deserializedCacheNetworkSettings.dnsSearchDomain = reader.getString();
} else if ("ntpServer".equals(fieldName)) {
deserializedCacheNetworkSettings.ntpServer = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedCacheNetworkSettings;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy