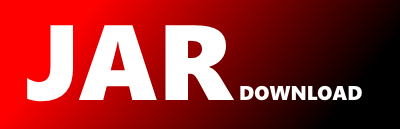
com.azure.resourcemanager.storagecache.models.CacheUsernameDownloadSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-storagecache Show documentation
Show all versions of azure-resourcemanager-storagecache Show documentation
This package contains Microsoft Azure SDK for StorageCache Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Azure Managed Lustre provides a fully managed Lustre® file system, integrated with Blob storage, for use on demand. These operations create and manage Azure Managed Lustre file systems. Package tag package-2024-03.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.storagecache.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* Settings for Extended Groups username and group download.
*/
@Fluent
public final class CacheUsernameDownloadSettings implements JsonSerializable {
/*
* Whether or not Extended Groups is enabled.
*/
private Boolean extendedGroups;
/*
* This setting determines how the cache gets username and group names for clients.
*/
private UsernameSource usernameSource;
/*
* The URI of the file containing group information (in /etc/group file format). This field must be populated when
* 'usernameSource' is set to 'File'.
*/
private String groupFileUri;
/*
* The URI of the file containing user information (in /etc/passwd file format). This field must be populated when
* 'usernameSource' is set to 'File'.
*/
private String userFileUri;
/*
* The fully qualified domain name or IP address of the LDAP server to use.
*/
private String ldapServer;
/*
* The base distinguished name for the LDAP domain.
*/
private String ldapBaseDN;
/*
* Whether or not the LDAP connection should be encrypted.
*/
private Boolean encryptLdapConnection;
/*
* Determines if the certificates must be validated by a certificate authority. When true, caCertificateURI must be
* provided.
*/
private Boolean requireValidCertificate;
/*
* Determines if the certificate should be automatically downloaded. This applies to 'caCertificateURI' only if
* 'requireValidCertificate' is true.
*/
private Boolean autoDownloadCertificate;
/*
* The URI of the CA certificate to validate the LDAP secure connection. This field must be populated when
* 'requireValidCertificate' is set to true.
*/
private String caCertificateUri;
/*
* Indicates whether or not the HPC Cache has performed the username download successfully.
*/
private UsernameDownloadedType usernameDownloaded;
/*
* When present, these are the credentials for the secure LDAP connection.
*/
private CacheUsernameDownloadSettingsCredentials credentials;
/**
* Creates an instance of CacheUsernameDownloadSettings class.
*/
public CacheUsernameDownloadSettings() {
}
/**
* Get the extendedGroups property: Whether or not Extended Groups is enabled.
*
* @return the extendedGroups value.
*/
public Boolean extendedGroups() {
return this.extendedGroups;
}
/**
* Set the extendedGroups property: Whether or not Extended Groups is enabled.
*
* @param extendedGroups the extendedGroups value to set.
* @return the CacheUsernameDownloadSettings object itself.
*/
public CacheUsernameDownloadSettings withExtendedGroups(Boolean extendedGroups) {
this.extendedGroups = extendedGroups;
return this;
}
/**
* Get the usernameSource property: This setting determines how the cache gets username and group names for clients.
*
* @return the usernameSource value.
*/
public UsernameSource usernameSource() {
return this.usernameSource;
}
/**
* Set the usernameSource property: This setting determines how the cache gets username and group names for clients.
*
* @param usernameSource the usernameSource value to set.
* @return the CacheUsernameDownloadSettings object itself.
*/
public CacheUsernameDownloadSettings withUsernameSource(UsernameSource usernameSource) {
this.usernameSource = usernameSource;
return this;
}
/**
* Get the groupFileUri property: The URI of the file containing group information (in /etc/group file format). This
* field must be populated when 'usernameSource' is set to 'File'.
*
* @return the groupFileUri value.
*/
public String groupFileUri() {
return this.groupFileUri;
}
/**
* Set the groupFileUri property: The URI of the file containing group information (in /etc/group file format). This
* field must be populated when 'usernameSource' is set to 'File'.
*
* @param groupFileUri the groupFileUri value to set.
* @return the CacheUsernameDownloadSettings object itself.
*/
public CacheUsernameDownloadSettings withGroupFileUri(String groupFileUri) {
this.groupFileUri = groupFileUri;
return this;
}
/**
* Get the userFileUri property: The URI of the file containing user information (in /etc/passwd file format). This
* field must be populated when 'usernameSource' is set to 'File'.
*
* @return the userFileUri value.
*/
public String userFileUri() {
return this.userFileUri;
}
/**
* Set the userFileUri property: The URI of the file containing user information (in /etc/passwd file format). This
* field must be populated when 'usernameSource' is set to 'File'.
*
* @param userFileUri the userFileUri value to set.
* @return the CacheUsernameDownloadSettings object itself.
*/
public CacheUsernameDownloadSettings withUserFileUri(String userFileUri) {
this.userFileUri = userFileUri;
return this;
}
/**
* Get the ldapServer property: The fully qualified domain name or IP address of the LDAP server to use.
*
* @return the ldapServer value.
*/
public String ldapServer() {
return this.ldapServer;
}
/**
* Set the ldapServer property: The fully qualified domain name or IP address of the LDAP server to use.
*
* @param ldapServer the ldapServer value to set.
* @return the CacheUsernameDownloadSettings object itself.
*/
public CacheUsernameDownloadSettings withLdapServer(String ldapServer) {
this.ldapServer = ldapServer;
return this;
}
/**
* Get the ldapBaseDN property: The base distinguished name for the LDAP domain.
*
* @return the ldapBaseDN value.
*/
public String ldapBaseDN() {
return this.ldapBaseDN;
}
/**
* Set the ldapBaseDN property: The base distinguished name for the LDAP domain.
*
* @param ldapBaseDN the ldapBaseDN value to set.
* @return the CacheUsernameDownloadSettings object itself.
*/
public CacheUsernameDownloadSettings withLdapBaseDN(String ldapBaseDN) {
this.ldapBaseDN = ldapBaseDN;
return this;
}
/**
* Get the encryptLdapConnection property: Whether or not the LDAP connection should be encrypted.
*
* @return the encryptLdapConnection value.
*/
public Boolean encryptLdapConnection() {
return this.encryptLdapConnection;
}
/**
* Set the encryptLdapConnection property: Whether or not the LDAP connection should be encrypted.
*
* @param encryptLdapConnection the encryptLdapConnection value to set.
* @return the CacheUsernameDownloadSettings object itself.
*/
public CacheUsernameDownloadSettings withEncryptLdapConnection(Boolean encryptLdapConnection) {
this.encryptLdapConnection = encryptLdapConnection;
return this;
}
/**
* Get the requireValidCertificate property: Determines if the certificates must be validated by a certificate
* authority. When true, caCertificateURI must be provided.
*
* @return the requireValidCertificate value.
*/
public Boolean requireValidCertificate() {
return this.requireValidCertificate;
}
/**
* Set the requireValidCertificate property: Determines if the certificates must be validated by a certificate
* authority. When true, caCertificateURI must be provided.
*
* @param requireValidCertificate the requireValidCertificate value to set.
* @return the CacheUsernameDownloadSettings object itself.
*/
public CacheUsernameDownloadSettings withRequireValidCertificate(Boolean requireValidCertificate) {
this.requireValidCertificate = requireValidCertificate;
return this;
}
/**
* Get the autoDownloadCertificate property: Determines if the certificate should be automatically downloaded. This
* applies to 'caCertificateURI' only if 'requireValidCertificate' is true.
*
* @return the autoDownloadCertificate value.
*/
public Boolean autoDownloadCertificate() {
return this.autoDownloadCertificate;
}
/**
* Set the autoDownloadCertificate property: Determines if the certificate should be automatically downloaded. This
* applies to 'caCertificateURI' only if 'requireValidCertificate' is true.
*
* @param autoDownloadCertificate the autoDownloadCertificate value to set.
* @return the CacheUsernameDownloadSettings object itself.
*/
public CacheUsernameDownloadSettings withAutoDownloadCertificate(Boolean autoDownloadCertificate) {
this.autoDownloadCertificate = autoDownloadCertificate;
return this;
}
/**
* Get the caCertificateUri property: The URI of the CA certificate to validate the LDAP secure connection. This
* field must be populated when 'requireValidCertificate' is set to true.
*
* @return the caCertificateUri value.
*/
public String caCertificateUri() {
return this.caCertificateUri;
}
/**
* Set the caCertificateUri property: The URI of the CA certificate to validate the LDAP secure connection. This
* field must be populated when 'requireValidCertificate' is set to true.
*
* @param caCertificateUri the caCertificateUri value to set.
* @return the CacheUsernameDownloadSettings object itself.
*/
public CacheUsernameDownloadSettings withCaCertificateUri(String caCertificateUri) {
this.caCertificateUri = caCertificateUri;
return this;
}
/**
* Get the usernameDownloaded property: Indicates whether or not the HPC Cache has performed the username download
* successfully.
*
* @return the usernameDownloaded value.
*/
public UsernameDownloadedType usernameDownloaded() {
return this.usernameDownloaded;
}
/**
* Get the credentials property: When present, these are the credentials for the secure LDAP connection.
*
* @return the credentials value.
*/
public CacheUsernameDownloadSettingsCredentials credentials() {
return this.credentials;
}
/**
* Set the credentials property: When present, these are the credentials for the secure LDAP connection.
*
* @param credentials the credentials value to set.
* @return the CacheUsernameDownloadSettings object itself.
*/
public CacheUsernameDownloadSettings withCredentials(CacheUsernameDownloadSettingsCredentials credentials) {
this.credentials = credentials;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (credentials() != null) {
credentials().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeBooleanField("extendedGroups", this.extendedGroups);
jsonWriter.writeStringField("usernameSource",
this.usernameSource == null ? null : this.usernameSource.toString());
jsonWriter.writeStringField("groupFileURI", this.groupFileUri);
jsonWriter.writeStringField("userFileURI", this.userFileUri);
jsonWriter.writeStringField("ldapServer", this.ldapServer);
jsonWriter.writeStringField("ldapBaseDN", this.ldapBaseDN);
jsonWriter.writeBooleanField("encryptLdapConnection", this.encryptLdapConnection);
jsonWriter.writeBooleanField("requireValidCertificate", this.requireValidCertificate);
jsonWriter.writeBooleanField("autoDownloadCertificate", this.autoDownloadCertificate);
jsonWriter.writeStringField("caCertificateURI", this.caCertificateUri);
jsonWriter.writeJsonField("credentials", this.credentials);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of CacheUsernameDownloadSettings from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of CacheUsernameDownloadSettings if the JsonReader was pointing to an instance of it, or null
* if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the CacheUsernameDownloadSettings.
*/
public static CacheUsernameDownloadSettings fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
CacheUsernameDownloadSettings deserializedCacheUsernameDownloadSettings
= new CacheUsernameDownloadSettings();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("extendedGroups".equals(fieldName)) {
deserializedCacheUsernameDownloadSettings.extendedGroups
= reader.getNullable(JsonReader::getBoolean);
} else if ("usernameSource".equals(fieldName)) {
deserializedCacheUsernameDownloadSettings.usernameSource
= UsernameSource.fromString(reader.getString());
} else if ("groupFileURI".equals(fieldName)) {
deserializedCacheUsernameDownloadSettings.groupFileUri = reader.getString();
} else if ("userFileURI".equals(fieldName)) {
deserializedCacheUsernameDownloadSettings.userFileUri = reader.getString();
} else if ("ldapServer".equals(fieldName)) {
deserializedCacheUsernameDownloadSettings.ldapServer = reader.getString();
} else if ("ldapBaseDN".equals(fieldName)) {
deserializedCacheUsernameDownloadSettings.ldapBaseDN = reader.getString();
} else if ("encryptLdapConnection".equals(fieldName)) {
deserializedCacheUsernameDownloadSettings.encryptLdapConnection
= reader.getNullable(JsonReader::getBoolean);
} else if ("requireValidCertificate".equals(fieldName)) {
deserializedCacheUsernameDownloadSettings.requireValidCertificate
= reader.getNullable(JsonReader::getBoolean);
} else if ("autoDownloadCertificate".equals(fieldName)) {
deserializedCacheUsernameDownloadSettings.autoDownloadCertificate
= reader.getNullable(JsonReader::getBoolean);
} else if ("caCertificateURI".equals(fieldName)) {
deserializedCacheUsernameDownloadSettings.caCertificateUri = reader.getString();
} else if ("usernameDownloaded".equals(fieldName)) {
deserializedCacheUsernameDownloadSettings.usernameDownloaded
= UsernameDownloadedType.fromString(reader.getString());
} else if ("credentials".equals(fieldName)) {
deserializedCacheUsernameDownloadSettings.credentials
= CacheUsernameDownloadSettingsCredentials.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedCacheUsernameDownloadSettings;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy