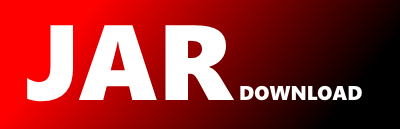
com.azure.resourcemanager.storagecache.models.ImportJob Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-storagecache Show documentation
Show all versions of azure-resourcemanager-storagecache Show documentation
This package contains Microsoft Azure SDK for StorageCache Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. Azure Managed Lustre provides a fully managed Lustre® file system, integrated with Blob storage, for use on demand. These operations create and manage Azure Managed Lustre file systems. Package tag package-2024-03.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.storagecache.models;
import com.azure.core.management.Region;
import com.azure.core.management.SystemData;
import com.azure.core.util.Context;
import com.azure.resourcemanager.storagecache.fluent.models.ImportJobInner;
import java.time.OffsetDateTime;
import java.util.List;
import java.util.Map;
/**
* An immutable client-side representation of ImportJob.
*/
public interface ImportJob {
/**
* Gets the id property: Fully qualified resource Id for the resource.
*
* @return the id value.
*/
String id();
/**
* Gets the name property: The name of the resource.
*
* @return the name value.
*/
String name();
/**
* Gets the type property: The type of the resource.
*
* @return the type value.
*/
String type();
/**
* Gets the location property: The geo-location where the resource lives.
*
* @return the location value.
*/
String location();
/**
* Gets the tags property: Resource tags.
*
* @return the tags value.
*/
Map tags();
/**
* Gets the systemData property: Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
* @return the systemData value.
*/
SystemData systemData();
/**
* Gets the provisioningState property: ARM provisioning state.
*
* @return the provisioningState value.
*/
ImportJobProvisioningStateType provisioningState();
/**
* Gets the importPrefixes property: An array of blob paths/prefixes that get imported into the cluster namespace.
* It has '/' as the default value.
*
* @return the importPrefixes value.
*/
List importPrefixes();
/**
* Gets the conflictResolutionMode property: How the import job will handle conflicts. For example, if the import
* job is trying to bring in a directory, but a file is at that path, how it handles it. Fail indicates that the
* import job should stop immediately and not do anything with the conflict. Skip indicates that it should pass over
* the conflict. OverwriteIfDirty causes the import job to delete and re-import the file or directory if it is a
* conflicting type, is dirty, or was not previously imported. OverwriteAlways extends OverwriteIfDirty to include
* releasing files that had been restored but were not dirty. Please reference
* https://learn.microsoft.com/en-us/azure/azure-managed-lustre/ for a thorough explanation of these resolution
* modes.
*
* @return the conflictResolutionMode value.
*/
ConflictResolutionMode conflictResolutionMode();
/**
* Gets the maximumErrors property: Total non-conflict oriented errors the import job will tolerate before exiting
* with failure. -1 means infinite. 0 means exit immediately and is the default.
*
* @return the maximumErrors value.
*/
Integer maximumErrors();
/**
* Gets the state property: The state of the import job. InProgress indicates the import is still running. Canceled
* indicates it has been canceled by the user. Completed indicates import finished, successfully importing all
* discovered blobs into the Lustre namespace. CompletedPartial indicates the import finished but some blobs either
* were found to be conflicting and could not be imported or other errors were encountered. Failed means the import
* was unable to complete due to a fatal error.
*
* @return the state value.
*/
ImportStatusType state();
/**
* Gets the statusMessage property: The status message of the import job.
*
* @return the statusMessage value.
*/
String statusMessage();
/**
* Gets the totalBlobsWalked property: The total blob objects walked.
*
* @return the totalBlobsWalked value.
*/
Long totalBlobsWalked();
/**
* Gets the blobsWalkedPerSecond property: A recent and frequently updated rate of blobs walked per second.
*
* @return the blobsWalkedPerSecond value.
*/
Long blobsWalkedPerSecond();
/**
* Gets the totalBlobsImported property: The total blobs that have been imported since import began.
*
* @return the totalBlobsImported value.
*/
Long totalBlobsImported();
/**
* Gets the blobsImportedPerSecond property: A recent and frequently updated rate of total files, directories, and
* symlinks imported per second.
*
* @return the blobsImportedPerSecond value.
*/
Long blobsImportedPerSecond();
/**
* Gets the lastCompletionTime property: The time of the last completed archive operation.
*
* @return the lastCompletionTime value.
*/
OffsetDateTime lastCompletionTime();
/**
* Gets the lastStartedTime property: The time the latest archive operation started.
*
* @return the lastStartedTime value.
*/
OffsetDateTime lastStartedTime();
/**
* Gets the totalErrors property: Number of errors in the import job.
*
* @return the totalErrors value.
*/
Integer totalErrors();
/**
* Gets the totalConflicts property: Number of conflicts in the import job.
*
* @return the totalConflicts value.
*/
Integer totalConflicts();
/**
* Gets the region of the resource.
*
* @return the region of the resource.
*/
Region region();
/**
* Gets the name of the resource region.
*
* @return the name of the resource region.
*/
String regionName();
/**
* Gets the name of the resource group.
*
* @return the name of the resource group.
*/
String resourceGroupName();
/**
* Gets the inner com.azure.resourcemanager.storagecache.fluent.models.ImportJobInner object.
*
* @return the inner object.
*/
ImportJobInner innerModel();
/**
* The entirety of the ImportJob definition.
*/
interface Definition extends DefinitionStages.Blank, DefinitionStages.WithLocation,
DefinitionStages.WithParentResource, DefinitionStages.WithCreate {
}
/**
* The ImportJob definition stages.
*/
interface DefinitionStages {
/**
* The first stage of the ImportJob definition.
*/
interface Blank extends WithLocation {
}
/**
* The stage of the ImportJob definition allowing to specify location.
*/
interface WithLocation {
/**
* Specifies the region for the resource.
*
* @param location The geo-location where the resource lives.
* @return the next definition stage.
*/
WithParentResource withRegion(Region location);
/**
* Specifies the region for the resource.
*
* @param location The geo-location where the resource lives.
* @return the next definition stage.
*/
WithParentResource withRegion(String location);
}
/**
* The stage of the ImportJob definition allowing to specify parent resource.
*/
interface WithParentResource {
/**
* Specifies resourceGroupName, amlFilesystemName.
*
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param amlFilesystemName Name for the AML file system. Allows alphanumerics, underscores, and hyphens.
* Start and end with alphanumeric.
* @return the next definition stage.
*/
WithCreate withExistingAmlFilesystem(String resourceGroupName, String amlFilesystemName);
}
/**
* The stage of the ImportJob definition which contains all the minimum required properties for the resource to
* be created, but also allows for any other optional properties to be specified.
*/
interface WithCreate extends DefinitionStages.WithTags, DefinitionStages.WithImportPrefixes,
DefinitionStages.WithConflictResolutionMode, DefinitionStages.WithMaximumErrors {
/**
* Executes the create request.
*
* @return the created resource.
*/
ImportJob create();
/**
* Executes the create request.
*
* @param context The context to associate with this operation.
* @return the created resource.
*/
ImportJob create(Context context);
}
/**
* The stage of the ImportJob definition allowing to specify tags.
*/
interface WithTags {
/**
* Specifies the tags property: Resource tags..
*
* @param tags Resource tags.
* @return the next definition stage.
*/
WithCreate withTags(Map tags);
}
/**
* The stage of the ImportJob definition allowing to specify importPrefixes.
*/
interface WithImportPrefixes {
/**
* Specifies the importPrefixes property: An array of blob paths/prefixes that get imported into the cluster
* namespace. It has '/' as the default value..
*
* @param importPrefixes An array of blob paths/prefixes that get imported into the cluster namespace. It
* has '/' as the default value.
* @return the next definition stage.
*/
WithCreate withImportPrefixes(List importPrefixes);
}
/**
* The stage of the ImportJob definition allowing to specify conflictResolutionMode.
*/
interface WithConflictResolutionMode {
/**
* Specifies the conflictResolutionMode property: How the import job will handle conflicts. For example, if
* the import job is trying to bring in a directory, but a file is at that path, how it handles it. Fail
* indicates that the import job should stop immediately and not do anything with the conflict. Skip
* indicates that it should pass over the conflict. OverwriteIfDirty causes the import job to delete and
* re-import the file or directory if it is a conflicting type, is dirty, or was not previously imported.
* OverwriteAlways extends OverwriteIfDirty to include releasing files that had been restored but were not
* dirty. Please reference https://learn.microsoft.com/en-us/azure/azure-managed-lustre/ for a thorough
* explanation of these resolution modes..
*
* @param conflictResolutionMode How the import job will handle conflicts. For example, if the import job is
* trying to bring in a directory, but a file is at that path, how it handles it. Fail indicates that the
* import job should stop immediately and not do anything with the conflict. Skip indicates that it should
* pass over the conflict. OverwriteIfDirty causes the import job to delete and re-import the file or
* directory if it is a conflicting type, is dirty, or was not previously imported. OverwriteAlways extends
* OverwriteIfDirty to include releasing files that had been restored but were not dirty. Please reference
* https://learn.microsoft.com/en-us/azure/azure-managed-lustre/ for a thorough explanation of these
* resolution modes.
* @return the next definition stage.
*/
WithCreate withConflictResolutionMode(ConflictResolutionMode conflictResolutionMode);
}
/**
* The stage of the ImportJob definition allowing to specify maximumErrors.
*/
interface WithMaximumErrors {
/**
* Specifies the maximumErrors property: Total non-conflict oriented errors the import job will tolerate
* before exiting with failure. -1 means infinite. 0 means exit immediately and is the default..
*
* @param maximumErrors Total non-conflict oriented errors the import job will tolerate before exiting with
* failure. -1 means infinite. 0 means exit immediately and is the default.
* @return the next definition stage.
*/
WithCreate withMaximumErrors(Integer maximumErrors);
}
}
/**
* Begins update for the ImportJob resource.
*
* @return the stage of resource update.
*/
ImportJob.Update update();
/**
* The template for ImportJob update.
*/
interface Update extends UpdateStages.WithTags {
/**
* Executes the update request.
*
* @return the updated resource.
*/
ImportJob apply();
/**
* Executes the update request.
*
* @param context The context to associate with this operation.
* @return the updated resource.
*/
ImportJob apply(Context context);
}
/**
* The ImportJob update stages.
*/
interface UpdateStages {
/**
* The stage of the ImportJob update allowing to specify tags.
*/
interface WithTags {
/**
* Specifies the tags property: Resource tags..
*
* @param tags Resource tags.
* @return the next definition stage.
*/
Update withTags(Map tags);
}
}
/**
* Refreshes the resource to sync with Azure.
*
* @return the refreshed resource.
*/
ImportJob refresh();
/**
* Refreshes the resource to sync with Azure.
*
* @param context The context to associate with this operation.
* @return the refreshed resource.
*/
ImportJob refresh(Context context);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy