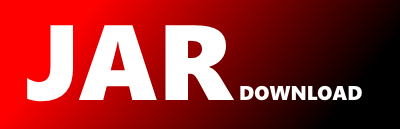
com.azure.resourcemanager.webpubsub.fluent.models.WebPubSubProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-resourcemanager-webpubsub Show documentation
Show all versions of azure-resourcemanager-webpubsub Show documentation
This package contains Microsoft Azure SDK for WebPubSub Management SDK. For documentation on how to use this package, please see https://aka.ms/azsdk/java/mgmt. REST API for Azure WebPubSub Service. Package tag package-2024-03-01.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.resourcemanager.webpubsub.fluent.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import com.azure.resourcemanager.webpubsub.models.LiveTraceConfiguration;
import com.azure.resourcemanager.webpubsub.models.ProvisioningState;
import com.azure.resourcemanager.webpubsub.models.ResourceLogConfiguration;
import com.azure.resourcemanager.webpubsub.models.WebPubSubNetworkACLs;
import com.azure.resourcemanager.webpubsub.models.WebPubSubSocketIOSettings;
import com.azure.resourcemanager.webpubsub.models.WebPubSubTlsSettings;
import java.io.IOException;
import java.util.List;
/**
* A class that describes the properties of the resource.
*/
@Fluent
public final class WebPubSubProperties implements JsonSerializable {
/*
* Provisioning state of the resource.
*/
private ProvisioningState provisioningState;
/*
* The publicly accessible IP of the resource.
*/
private String externalIp;
/*
* FQDN of the service instance.
*/
private String hostname;
/*
* The publicly accessible port of the resource which is designed for browser/client side usage.
*/
private Integer publicPort;
/*
* The publicly accessible port of the resource which is designed for customer server side usage.
*/
private Integer serverPort;
/*
* Version of the resource. Probably you need the same or higher version of client SDKs.
*/
private String version;
/*
* Private endpoint connections to the resource.
*/
private List privateEndpointConnections;
/*
* The list of shared private link resources.
*/
private List sharedPrivateLinkResources;
/*
* TLS settings for the resource
*/
private WebPubSubTlsSettings tls;
/*
* Deprecated.
*/
private String hostnamePrefix;
/*
* Live trace configuration of a Microsoft.SignalRService resource.
*/
private LiveTraceConfiguration liveTraceConfiguration;
/*
* Resource log configuration of a Microsoft.SignalRService resource.
*/
private ResourceLogConfiguration resourceLogConfiguration;
/*
* Network ACLs for the resource
*/
private WebPubSubNetworkACLs networkACLs;
/*
* Enable or disable public network access. Default to "Enabled".
* When it's Enabled, network ACLs still apply.
* When it's Disabled, public network access is always disabled no matter what you set in network ACLs.
*/
private String publicNetworkAccess;
/*
* DisableLocalAuth
* Enable or disable local auth with AccessKey
* When set as true, connection with AccessKey=xxx won't work.
*/
private Boolean disableLocalAuth;
/*
* DisableLocalAuth
* Enable or disable aad auth
* When set as true, connection with AuthType=aad won't work.
*/
private Boolean disableAadAuth;
/*
* Enable or disable the regional endpoint. Default to "Enabled".
* When it's Disabled, new connections will not be routed to this endpoint, however existing connections will not be
* affected.
* This property is replica specific. Disable the regional endpoint without replica is not allowed.
*/
private String regionEndpointEnabled;
/*
* Stop or start the resource. Default to "False".
* When it's true, the data plane of the resource is shutdown.
* When it's false, the data plane of the resource is started.
*/
private String resourceStopped;
/*
* SocketIO settings for the resource
*/
private WebPubSubSocketIOSettings socketIO;
/**
* Creates an instance of WebPubSubProperties class.
*/
public WebPubSubProperties() {
}
/**
* Get the provisioningState property: Provisioning state of the resource.
*
* @return the provisioningState value.
*/
public ProvisioningState provisioningState() {
return this.provisioningState;
}
/**
* Get the externalIp property: The publicly accessible IP of the resource.
*
* @return the externalIp value.
*/
public String externalIp() {
return this.externalIp;
}
/**
* Get the hostname property: FQDN of the service instance.
*
* @return the hostname value.
*/
public String hostname() {
return this.hostname;
}
/**
* Get the publicPort property: The publicly accessible port of the resource which is designed for browser/client
* side usage.
*
* @return the publicPort value.
*/
public Integer publicPort() {
return this.publicPort;
}
/**
* Get the serverPort property: The publicly accessible port of the resource which is designed for customer server
* side usage.
*
* @return the serverPort value.
*/
public Integer serverPort() {
return this.serverPort;
}
/**
* Get the version property: Version of the resource. Probably you need the same or higher version of client SDKs.
*
* @return the version value.
*/
public String version() {
return this.version;
}
/**
* Get the privateEndpointConnections property: Private endpoint connections to the resource.
*
* @return the privateEndpointConnections value.
*/
public List privateEndpointConnections() {
return this.privateEndpointConnections;
}
/**
* Get the sharedPrivateLinkResources property: The list of shared private link resources.
*
* @return the sharedPrivateLinkResources value.
*/
public List sharedPrivateLinkResources() {
return this.sharedPrivateLinkResources;
}
/**
* Get the tls property: TLS settings for the resource.
*
* @return the tls value.
*/
public WebPubSubTlsSettings tls() {
return this.tls;
}
/**
* Set the tls property: TLS settings for the resource.
*
* @param tls the tls value to set.
* @return the WebPubSubProperties object itself.
*/
public WebPubSubProperties withTls(WebPubSubTlsSettings tls) {
this.tls = tls;
return this;
}
/**
* Get the hostnamePrefix property: Deprecated.
*
* @return the hostnamePrefix value.
*/
public String hostnamePrefix() {
return this.hostnamePrefix;
}
/**
* Get the liveTraceConfiguration property: Live trace configuration of a Microsoft.SignalRService resource.
*
* @return the liveTraceConfiguration value.
*/
public LiveTraceConfiguration liveTraceConfiguration() {
return this.liveTraceConfiguration;
}
/**
* Set the liveTraceConfiguration property: Live trace configuration of a Microsoft.SignalRService resource.
*
* @param liveTraceConfiguration the liveTraceConfiguration value to set.
* @return the WebPubSubProperties object itself.
*/
public WebPubSubProperties withLiveTraceConfiguration(LiveTraceConfiguration liveTraceConfiguration) {
this.liveTraceConfiguration = liveTraceConfiguration;
return this;
}
/**
* Get the resourceLogConfiguration property: Resource log configuration of a Microsoft.SignalRService resource.
*
* @return the resourceLogConfiguration value.
*/
public ResourceLogConfiguration resourceLogConfiguration() {
return this.resourceLogConfiguration;
}
/**
* Set the resourceLogConfiguration property: Resource log configuration of a Microsoft.SignalRService resource.
*
* @param resourceLogConfiguration the resourceLogConfiguration value to set.
* @return the WebPubSubProperties object itself.
*/
public WebPubSubProperties withResourceLogConfiguration(ResourceLogConfiguration resourceLogConfiguration) {
this.resourceLogConfiguration = resourceLogConfiguration;
return this;
}
/**
* Get the networkACLs property: Network ACLs for the resource.
*
* @return the networkACLs value.
*/
public WebPubSubNetworkACLs networkACLs() {
return this.networkACLs;
}
/**
* Set the networkACLs property: Network ACLs for the resource.
*
* @param networkACLs the networkACLs value to set.
* @return the WebPubSubProperties object itself.
*/
public WebPubSubProperties withNetworkACLs(WebPubSubNetworkACLs networkACLs) {
this.networkACLs = networkACLs;
return this;
}
/**
* Get the publicNetworkAccess property: Enable or disable public network access. Default to "Enabled".
* When it's Enabled, network ACLs still apply.
* When it's Disabled, public network access is always disabled no matter what you set in network ACLs.
*
* @return the publicNetworkAccess value.
*/
public String publicNetworkAccess() {
return this.publicNetworkAccess;
}
/**
* Set the publicNetworkAccess property: Enable or disable public network access. Default to "Enabled".
* When it's Enabled, network ACLs still apply.
* When it's Disabled, public network access is always disabled no matter what you set in network ACLs.
*
* @param publicNetworkAccess the publicNetworkAccess value to set.
* @return the WebPubSubProperties object itself.
*/
public WebPubSubProperties withPublicNetworkAccess(String publicNetworkAccess) {
this.publicNetworkAccess = publicNetworkAccess;
return this;
}
/**
* Get the disableLocalAuth property: DisableLocalAuth
* Enable or disable local auth with AccessKey
* When set as true, connection with AccessKey=xxx won't work.
*
* @return the disableLocalAuth value.
*/
public Boolean disableLocalAuth() {
return this.disableLocalAuth;
}
/**
* Set the disableLocalAuth property: DisableLocalAuth
* Enable or disable local auth with AccessKey
* When set as true, connection with AccessKey=xxx won't work.
*
* @param disableLocalAuth the disableLocalAuth value to set.
* @return the WebPubSubProperties object itself.
*/
public WebPubSubProperties withDisableLocalAuth(Boolean disableLocalAuth) {
this.disableLocalAuth = disableLocalAuth;
return this;
}
/**
* Get the disableAadAuth property: DisableLocalAuth
* Enable or disable aad auth
* When set as true, connection with AuthType=aad won't work.
*
* @return the disableAadAuth value.
*/
public Boolean disableAadAuth() {
return this.disableAadAuth;
}
/**
* Set the disableAadAuth property: DisableLocalAuth
* Enable or disable aad auth
* When set as true, connection with AuthType=aad won't work.
*
* @param disableAadAuth the disableAadAuth value to set.
* @return the WebPubSubProperties object itself.
*/
public WebPubSubProperties withDisableAadAuth(Boolean disableAadAuth) {
this.disableAadAuth = disableAadAuth;
return this;
}
/**
* Get the regionEndpointEnabled property: Enable or disable the regional endpoint. Default to "Enabled".
* When it's Disabled, new connections will not be routed to this endpoint, however existing connections will not be
* affected.
* This property is replica specific. Disable the regional endpoint without replica is not allowed.
*
* @return the regionEndpointEnabled value.
*/
public String regionEndpointEnabled() {
return this.regionEndpointEnabled;
}
/**
* Set the regionEndpointEnabled property: Enable or disable the regional endpoint. Default to "Enabled".
* When it's Disabled, new connections will not be routed to this endpoint, however existing connections will not be
* affected.
* This property is replica specific. Disable the regional endpoint without replica is not allowed.
*
* @param regionEndpointEnabled the regionEndpointEnabled value to set.
* @return the WebPubSubProperties object itself.
*/
public WebPubSubProperties withRegionEndpointEnabled(String regionEndpointEnabled) {
this.regionEndpointEnabled = regionEndpointEnabled;
return this;
}
/**
* Get the resourceStopped property: Stop or start the resource. Default to "False".
* When it's true, the data plane of the resource is shutdown.
* When it's false, the data plane of the resource is started.
*
* @return the resourceStopped value.
*/
public String resourceStopped() {
return this.resourceStopped;
}
/**
* Set the resourceStopped property: Stop or start the resource. Default to "False".
* When it's true, the data plane of the resource is shutdown.
* When it's false, the data plane of the resource is started.
*
* @param resourceStopped the resourceStopped value to set.
* @return the WebPubSubProperties object itself.
*/
public WebPubSubProperties withResourceStopped(String resourceStopped) {
this.resourceStopped = resourceStopped;
return this;
}
/**
* Get the socketIO property: SocketIO settings for the resource.
*
* @return the socketIO value.
*/
public WebPubSubSocketIOSettings socketIO() {
return this.socketIO;
}
/**
* Set the socketIO property: SocketIO settings for the resource.
*
* @param socketIO the socketIO value to set.
* @return the WebPubSubProperties object itself.
*/
public WebPubSubProperties withSocketIO(WebPubSubSocketIOSettings socketIO) {
this.socketIO = socketIO;
return this;
}
/**
* Validates the instance.
*
* @throws IllegalArgumentException thrown if the instance is not valid.
*/
public void validate() {
if (privateEndpointConnections() != null) {
privateEndpointConnections().forEach(e -> e.validate());
}
if (sharedPrivateLinkResources() != null) {
sharedPrivateLinkResources().forEach(e -> e.validate());
}
if (tls() != null) {
tls().validate();
}
if (liveTraceConfiguration() != null) {
liveTraceConfiguration().validate();
}
if (resourceLogConfiguration() != null) {
resourceLogConfiguration().validate();
}
if (networkACLs() != null) {
networkACLs().validate();
}
if (socketIO() != null) {
socketIO().validate();
}
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("tls", this.tls);
jsonWriter.writeJsonField("liveTraceConfiguration", this.liveTraceConfiguration);
jsonWriter.writeJsonField("resourceLogConfiguration", this.resourceLogConfiguration);
jsonWriter.writeJsonField("networkACLs", this.networkACLs);
jsonWriter.writeStringField("publicNetworkAccess", this.publicNetworkAccess);
jsonWriter.writeBooleanField("disableLocalAuth", this.disableLocalAuth);
jsonWriter.writeBooleanField("disableAadAuth", this.disableAadAuth);
jsonWriter.writeStringField("regionEndpointEnabled", this.regionEndpointEnabled);
jsonWriter.writeStringField("resourceStopped", this.resourceStopped);
jsonWriter.writeJsonField("socketIO", this.socketIO);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of WebPubSubProperties from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of WebPubSubProperties if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IOException If an error occurs while reading the WebPubSubProperties.
*/
public static WebPubSubProperties fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
WebPubSubProperties deserializedWebPubSubProperties = new WebPubSubProperties();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("provisioningState".equals(fieldName)) {
deserializedWebPubSubProperties.provisioningState
= ProvisioningState.fromString(reader.getString());
} else if ("externalIP".equals(fieldName)) {
deserializedWebPubSubProperties.externalIp = reader.getString();
} else if ("hostName".equals(fieldName)) {
deserializedWebPubSubProperties.hostname = reader.getString();
} else if ("publicPort".equals(fieldName)) {
deserializedWebPubSubProperties.publicPort = reader.getNullable(JsonReader::getInt);
} else if ("serverPort".equals(fieldName)) {
deserializedWebPubSubProperties.serverPort = reader.getNullable(JsonReader::getInt);
} else if ("version".equals(fieldName)) {
deserializedWebPubSubProperties.version = reader.getString();
} else if ("privateEndpointConnections".equals(fieldName)) {
List privateEndpointConnections
= reader.readArray(reader1 -> PrivateEndpointConnectionInner.fromJson(reader1));
deserializedWebPubSubProperties.privateEndpointConnections = privateEndpointConnections;
} else if ("sharedPrivateLinkResources".equals(fieldName)) {
List sharedPrivateLinkResources
= reader.readArray(reader1 -> SharedPrivateLinkResourceInner.fromJson(reader1));
deserializedWebPubSubProperties.sharedPrivateLinkResources = sharedPrivateLinkResources;
} else if ("tls".equals(fieldName)) {
deserializedWebPubSubProperties.tls = WebPubSubTlsSettings.fromJson(reader);
} else if ("hostNamePrefix".equals(fieldName)) {
deserializedWebPubSubProperties.hostnamePrefix = reader.getString();
} else if ("liveTraceConfiguration".equals(fieldName)) {
deserializedWebPubSubProperties.liveTraceConfiguration = LiveTraceConfiguration.fromJson(reader);
} else if ("resourceLogConfiguration".equals(fieldName)) {
deserializedWebPubSubProperties.resourceLogConfiguration
= ResourceLogConfiguration.fromJson(reader);
} else if ("networkACLs".equals(fieldName)) {
deserializedWebPubSubProperties.networkACLs = WebPubSubNetworkACLs.fromJson(reader);
} else if ("publicNetworkAccess".equals(fieldName)) {
deserializedWebPubSubProperties.publicNetworkAccess = reader.getString();
} else if ("disableLocalAuth".equals(fieldName)) {
deserializedWebPubSubProperties.disableLocalAuth = reader.getNullable(JsonReader::getBoolean);
} else if ("disableAadAuth".equals(fieldName)) {
deserializedWebPubSubProperties.disableAadAuth = reader.getNullable(JsonReader::getBoolean);
} else if ("regionEndpointEnabled".equals(fieldName)) {
deserializedWebPubSubProperties.regionEndpointEnabled = reader.getString();
} else if ("resourceStopped".equals(fieldName)) {
deserializedWebPubSubProperties.resourceStopped = reader.getString();
} else if ("socketIO".equals(fieldName)) {
deserializedWebPubSubProperties.socketIO = WebPubSubSocketIOSettings.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedWebPubSubProperties;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy