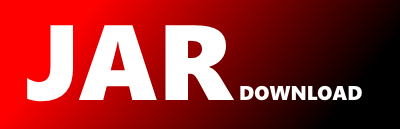
com.azure.ai.metricsadvisor.administration.MetricsAdvisorAdministrationAsyncClient Maven / Gradle / Ivy
Show all versions of azure-ai-metricsadvisor Show documentation
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.ai.metricsadvisor.administration;
import com.azure.ai.metricsadvisor.MetricsAdvisorServiceVersion;
import com.azure.ai.metricsadvisor.administration.models.AnomalyAlertConfiguration;
import com.azure.ai.metricsadvisor.administration.models.AnomalyDetectionConfiguration;
import com.azure.ai.metricsadvisor.administration.models.DataFeed;
import com.azure.ai.metricsadvisor.administration.models.DataFeedGranularity;
import com.azure.ai.metricsadvisor.administration.models.DataFeedIngestionProgress;
import com.azure.ai.metricsadvisor.administration.models.DataFeedIngestionSettings;
import com.azure.ai.metricsadvisor.administration.models.DataFeedIngestionStatus;
import com.azure.ai.metricsadvisor.administration.models.DataFeedMissingDataPointFillSettings;
import com.azure.ai.metricsadvisor.administration.models.DataFeedMissingDataPointFillType;
import com.azure.ai.metricsadvisor.administration.models.DataFeedOptions;
import com.azure.ai.metricsadvisor.administration.models.DataFeedRollupSettings;
import com.azure.ai.metricsadvisor.administration.models.DataFeedSchema;
import com.azure.ai.metricsadvisor.administration.models.DataSourceCredentialEntity;
import com.azure.ai.metricsadvisor.administration.models.ListAnomalyAlertConfigsOptions;
import com.azure.ai.metricsadvisor.administration.models.ListCredentialEntityOptions;
import com.azure.ai.metricsadvisor.administration.models.ListDataFeedFilter;
import com.azure.ai.metricsadvisor.administration.models.ListDataFeedIngestionOptions;
import com.azure.ai.metricsadvisor.administration.models.ListDataFeedOptions;
import com.azure.ai.metricsadvisor.administration.models.ListDetectionConfigsOptions;
import com.azure.ai.metricsadvisor.administration.models.ListHookOptions;
import com.azure.ai.metricsadvisor.administration.models.NotificationHook;
import com.azure.ai.metricsadvisor.implementation.MetricsAdvisorImpl;
import com.azure.ai.metricsadvisor.implementation.models.AnomalyAlertingConfiguration;
import com.azure.ai.metricsadvisor.implementation.models.AnomalyAlertingConfigurationPatch;
import com.azure.ai.metricsadvisor.implementation.models.AnomalyDetectionConfigurationPatch;
import com.azure.ai.metricsadvisor.implementation.models.DataSourceCredential;
import com.azure.ai.metricsadvisor.implementation.models.DataSourceCredentialPatch;
import com.azure.ai.metricsadvisor.implementation.models.DataSourceType;
import com.azure.ai.metricsadvisor.implementation.models.EntityStatus;
import com.azure.ai.metricsadvisor.implementation.models.FillMissingPointType;
import com.azure.ai.metricsadvisor.implementation.models.Granularity;
import com.azure.ai.metricsadvisor.implementation.models.IngestionProgressResetOptions;
import com.azure.ai.metricsadvisor.implementation.models.IngestionStatusQueryOptions;
import com.azure.ai.metricsadvisor.implementation.models.NeedRollupEnum;
import com.azure.ai.metricsadvisor.implementation.models.RollUpMethod;
import com.azure.ai.metricsadvisor.implementation.models.ViewMode;
import com.azure.ai.metricsadvisor.implementation.util.AlertConfigurationTransforms;
import com.azure.ai.metricsadvisor.implementation.util.DataFeedTransforms;
import com.azure.ai.metricsadvisor.implementation.util.DataSourceCredentialEntityTransforms;
import com.azure.ai.metricsadvisor.implementation.util.DetectionConfigurationTransforms;
import com.azure.ai.metricsadvisor.implementation.util.HookTransforms;
import com.azure.ai.metricsadvisor.implementation.util.Utility;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceClient;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.http.HttpPipeline;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.http.rest.PagedResponse;
import com.azure.core.http.rest.PagedResponseBase;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.ResponseBase;
import com.azure.core.http.rest.SimpleResponse;
import com.azure.core.util.Context;
import com.azure.core.util.CoreUtils;
import com.azure.core.util.FluxUtil;
import com.azure.core.util.logging.ClientLogger;
import reactor.core.publisher.Mono;
import java.time.OffsetDateTime;
import java.util.Objects;
import java.util.UUID;
import java.util.stream.Collectors;
import static com.azure.ai.metricsadvisor.administration.models.DataFeedGranularityType.CUSTOM;
import static com.azure.ai.metricsadvisor.implementation.util.Utility.parseOperationId;
import static com.azure.ai.metricsadvisor.implementation.util.Utility.toDataFeedIngestionProgress;
import static com.azure.ai.metricsadvisor.implementation.util.Utility.toDataFeedIngestionStatus;
import static com.azure.core.util.FluxUtil.monoError;
import static com.azure.core.util.FluxUtil.withContext;
/**
* This class provides asynchronous client to connect to the Metrics Advisor Azure Cognitive Service.
* This client provides asynchronous methods to perform:
*
* - Connect to a variety of data sources, Metrics Advisor can connect to, and ingest multi-dimensional metric data
* from many data stores, including: SQL Server, Azure Blob Storage, and MongoDB. Use
* {@link com.azure.ai.metricsadvisor.administration.MetricsAdvisorAdministrationAsyncClient#createDataFeed(DataFeed)}
* method to add your respective data source.
* - Customize anomaly detection configuration to detect anomalies for your needs using the
* {@link com.azure.ai.metricsadvisor.administration.MetricsAdvisorAdministrationAsyncClient#createDetectionConfig(String, AnomalyDetectionConfiguration)}
* method.
* - Add real-time notification through multiple channels. Configure hooks for multiple alerting and detection
* configuration using the
* {@link com.azure.ai.metricsadvisor.administration.MetricsAdvisorAdministrationAsyncClient#createHook(NotificationHook)}
* method./li>
*
*
* Service clients are the point of interaction for developers to use Azure Metrics Advisor.
* {@link com.azure.ai.metricsadvisor.administration.MetricsAdvisorAdministrationClient} is the synchronous service client and
* {@link com.azure.ai.metricsadvisor.administration.MetricsAdvisorAdministrationAsyncClient} is the asynchronous service client.
* The examples shown in this document use a credential object named DefaultAzureCredential for authentication, which is
* appropriate for most scenarios, including local development and production environments. Additionally, we
* recommend using
* managed identity
* for authentication in production environments.
* You can find more information on different ways of authenticating and their corresponding credential types in the
* Azure Identity documentation".
*
*
* Sample: Construct a MetricsAdvisorAdministrationAsyncClient with DefaultAzureCredential
*
* The following code sample demonstrates the creation of a
* {@link com.azure.ai.metricsadvisor.administration.MetricsAdvisorAdministrationAsyncClient}, using the
* `DefaultAzureCredentialBuilder` to configure it.
*
*
*
* MetricsAdvisorAdministrationAsyncClient metricsAdvisorAdminAsyncClient =
* new MetricsAdvisorAdministrationClientBuilder()
* .credential(new DefaultAzureCredentialBuilder().build())
* .endpoint("{endpoint}")
* .buildAsyncClient();
*
*
*
* Further, see the code sample below to use
* {@link com.azure.ai.metricsadvisor.models.MetricsAdvisorKeyCredential MetricsAdvisorKeyCredential} for client creation.
*
*
*
* MetricsAdvisorAdministrationAsyncClient metricsAdvisorAdminAsyncClient =
* new MetricsAdvisorAdministrationClientBuilder()
* .credential(new MetricsAdvisorKeyCredential("{subscription_key}", "{api_key}"))
* .endpoint("{endpoint}")
* .buildAsyncClient();
*
*
*
* @see com.azure.ai.metricsadvisor
* @see MetricsAdvisorAdministrationClient
* @see MetricsAdvisorAdministrationClientBuilder
*/
@ServiceClient(builder = MetricsAdvisorAdministrationClientBuilder.class, isAsync = true)
public final class MetricsAdvisorAdministrationAsyncClient {
private final ClientLogger logger = new ClientLogger(MetricsAdvisorAdministrationAsyncClient.class);
private final MetricsAdvisorImpl service;
/**
* Create a {@link MetricsAdvisorAdministrationAsyncClient} that sends requests to the Metrics Advisor
* service's endpoint. Each service call goes through the
* {@link MetricsAdvisorAdministrationClientBuilder#pipeline(HttpPipeline)} http pipeline.
*
* @param service The proxy service used to perform REST calls.
* @param serviceVersion The versions of Azure Metrics Advisor supported by this client library.
*/
MetricsAdvisorAdministrationAsyncClient(MetricsAdvisorImpl service, MetricsAdvisorServiceVersion serviceVersion) {
this.service = service;
}
/**
* Create a new data feed.
*
* Code sample
*
*
* DataFeed dataFeed = new DataFeed()
* .setName("dataFeedName")
* .setSource(new MySqlDataFeedSource("conn-string", "query"))
* .setGranularity(new DataFeedGranularity().setGranularityType(DataFeedGranularityType.DAILY))
* .setSchema(new DataFeedSchema(
* Arrays.asList(
* new DataFeedMetric("cost"),
* new DataFeedMetric("revenue")
* )).setDimensions(
* Arrays.asList(
* new DataFeedDimension("city"),
* new DataFeedDimension("category")
* ))
* )
* .setIngestionSettings(new DataFeedIngestionSettings(OffsetDateTime.parse("2020-01-01T00:00:00Z")))
* .setOptions(new DataFeedOptions()
* .setDescription("data feed description")
* .setRollupSettings(new DataFeedRollupSettings()
* .setRollupType(DataFeedRollupType.AUTO_ROLLUP)));
*
* metricsAdvisorAdminAsyncClient.createDataFeed(dataFeed)
* .subscribe(createdDataFeed -> {
* System.out.printf("Data feed Id: %s%n", createdDataFeed.getId());
* System.out.printf("Data feed description: %s%n", createdDataFeed.getOptions().getDescription());
* System.out.printf("Data feed source type: %s%n", createdDataFeed.getSourceType());
* System.out.printf("Data feed creator: %s%n", createdDataFeed.getCreator());
* });
*
*
*
* @param dataFeed The data feed to be created.
* @return A {@link Mono} containing the created data feed.
* @throws NullPointerException If {@code dataFeed}, {@code dataFeedName}, {@code dataFeedSource}, {@code metrics},
* {@code granularityType} or {@code ingestionStartTime} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono createDataFeed(DataFeed dataFeed) {
return createDataFeedWithResponse(dataFeed).flatMap(FluxUtil::toMono);
}
/**
* Create a new data feed with REST response.
*
* Code sample
*
*
* DataFeed dataFeed = new DataFeed()
* .setName("dataFeedName")
* .setSource(new MySqlDataFeedSource("conn-string", "query"))
* .setGranularity(new DataFeedGranularity().setGranularityType(DataFeedGranularityType.DAILY))
* .setSchema(new DataFeedSchema(
* Arrays.asList(
* new DataFeedMetric("metric1"),
* new DataFeedMetric("metric2")
* )
* ))
* .setIngestionSettings(new DataFeedIngestionSettings(OffsetDateTime.parse("2020-01-01T00:00:00Z")))
* .setOptions(new DataFeedOptions()
* .setDescription("data feed description")
* .setRollupSettings(new DataFeedRollupSettings()
* .setRollupType(DataFeedRollupType.AUTO_ROLLUP)));
*
* metricsAdvisorAdminAsyncClient.createDataFeedWithResponse(dataFeed)
* .subscribe(dataFeedResponse -> {
* System.out.printf("Data feed create operation status: %s%n", dataFeedResponse.getStatusCode());
* DataFeed createdDataFeed = dataFeedResponse.getValue();
* System.out.printf("Data feed Id: %s%n", createdDataFeed.getId());
* System.out.printf("Data feed description: %s%n", createdDataFeed.getOptions().getDescription());
* System.out.printf("Data feed source type: %s%n", createdDataFeed.getSourceType());
* System.out.printf("Data feed creator: %s%n", createdDataFeed.getCreator());
* });
*
*
* @param dataFeed The data feed to be created.
* @return A {@link Response} of a {@link Mono} containing the created {@link DataFeed data feed}.
* @throws NullPointerException If {@code dataFeed}, {@code dataFeedName}, {@code dataFeedSource}, {@code metrics},
* {@code granularityType} or {@code ingestionStartTime} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> createDataFeedWithResponse(DataFeed dataFeed) {
try {
return withContext(context -> createDataFeedWithResponse(dataFeed, context));
} catch (RuntimeException ex) {
return monoError(logger, ex);
}
}
Mono> createDataFeedWithResponse(DataFeed dataFeed, Context context) {
Objects.requireNonNull(dataFeed, "'dataFeed' is required and cannot be null.");
Objects.requireNonNull(dataFeed.getSource(), "'dataFeedSource' is required and cannot be null.");
Objects.requireNonNull(dataFeed.getName(), "'dataFeedName' cannot be null or empty.");
final DataFeedSchema dataFeedSchema = dataFeed.getSchema();
final DataFeedGranularity dataFeedGranularity = dataFeed.getGranularity();
final DataFeedIngestionSettings dataFeedIngestionSettings = dataFeed.getIngestionSettings();
if (dataFeedSchema == null) {
throw logger
.logExceptionAsError(new NullPointerException("'dataFeedSchema.metrics' cannot be null or empty."));
} else {
Objects.requireNonNull(dataFeedSchema.getMetrics(), "'dataFeedSchema.metrics' cannot be null or empty.");
}
if (dataFeedGranularity == null) {
throw logger.logExceptionAsError(
new NullPointerException("'dataFeedGranularity.granularityType' is required and cannot be null."));
} else {
Objects.requireNonNull(dataFeedGranularity.getGranularityType(),
"'dataFeedGranularity.granularityType' is required.");
if (CUSTOM.equals(dataFeedGranularity.getGranularityType())) {
Objects.requireNonNull(dataFeedGranularity.getCustomGranularityValue(),
"'dataFeedGranularity.customGranularityValue' is required when granularity type is CUSTOM");
}
}
if (dataFeedIngestionSettings == null) {
throw logger.logExceptionAsError(new NullPointerException(
"'dataFeedIngestionSettings.ingestionStartTime' is required and cannot be null."));
} else {
Objects.requireNonNull(dataFeedIngestionSettings.getIngestionStartTime(),
"'dataFeedIngestionSettings.ingestionStartTime' is required and cannot be null.");
}
final DataFeedOptions finalDataFeedOptions
= dataFeed.getOptions() == null ? new DataFeedOptions() : dataFeed.getOptions();
final DataFeedRollupSettings dataFeedRollupSettings = finalDataFeedOptions.getRollupSettings() == null
? new DataFeedRollupSettings()
: finalDataFeedOptions.getRollupSettings();
final DataFeedMissingDataPointFillSettings dataFeedMissingDataPointFillSettings
= finalDataFeedOptions.getMissingDataPointFillSettings() == null
? new DataFeedMissingDataPointFillSettings()
: finalDataFeedOptions.getMissingDataPointFillSettings();
return service
.createDataFeedWithResponseAsync(DataFeedTransforms.toDataFeedDetailSource(dataFeed.getSource())
.setDataFeedName(dataFeed.getName())
.setDataFeedDescription(finalDataFeedOptions.getDescription())
.setGranularityName(Granularity.fromString(dataFeedGranularity.getGranularityType() == null
? null
: dataFeedGranularity.getGranularityType().toString()))
.setGranularityAmount(dataFeedGranularity.getCustomGranularityValue())
.setDimension(DataFeedTransforms.toInnerDimensionsListForCreate(dataFeedSchema.getDimensions()))
.setMetrics(DataFeedTransforms.toInnerMetricsListForCreate(dataFeedSchema.getMetrics()))
.setTimestampColumn(dataFeedSchema.getTimestampColumn())
.setDataStartFrom(dataFeedIngestionSettings.getIngestionStartTime())
.setStartOffsetInSeconds(dataFeedIngestionSettings.getIngestionStartOffset() == null
? null
: dataFeedIngestionSettings.getIngestionStartOffset().getSeconds())
.setMaxConcurrency(dataFeedIngestionSettings.getDataSourceRequestConcurrency())
.setStopRetryAfterInSeconds(dataFeedIngestionSettings.getStopRetryAfter() == null
? null
: dataFeedIngestionSettings.getStopRetryAfter().getSeconds())
.setMinRetryIntervalInSeconds(dataFeedIngestionSettings.getIngestionRetryDelay() == null
? null
: dataFeedIngestionSettings.getIngestionRetryDelay().getSeconds())
.setRollUpColumns(dataFeedRollupSettings.getAutoRollupGroupByColumnNames())
.setRollUpMethod(RollUpMethod.fromString(dataFeedRollupSettings.getDataFeedAutoRollUpMethod() == null
? null
: dataFeedRollupSettings.getDataFeedAutoRollUpMethod().toString()))
.setNeedRollup(NeedRollupEnum.fromString(dataFeedRollupSettings.getRollupType() == null
? null
: dataFeedRollupSettings.getRollupType().toString()))
.setAllUpIdentification(dataFeedRollupSettings.getRollupIdentificationValue())
.setFillMissingPointType(
FillMissingPointType.fromString(dataFeedMissingDataPointFillSettings.getFillType() == null
? null
: dataFeedMissingDataPointFillSettings.getFillType().toString()))
.setFillMissingPointValue(dataFeedMissingDataPointFillSettings.getCustomFillValue())
.setViewMode(ViewMode.fromString(finalDataFeedOptions.getAccessMode() == null
? null
: finalDataFeedOptions.getAccessMode().toString()))
.setViewers(finalDataFeedOptions.getViewers())
.setAdmins(finalDataFeedOptions.getAdmins())
.setActionLinkTemplate(finalDataFeedOptions.getActionLinkTemplate()), context)
.flatMap(createDataFeedResponse -> {
final String dataFeedId
= parseOperationId(createDataFeedResponse.getDeserializedHeaders().getLocation());
return getDataFeedWithResponse(dataFeedId);
});
}
/**
* Get a data feed by its id.
*
* Code sample
*
*
* final String dataFeedId = "r47053f1-9080-09lo-bacf-8dccf2e86f";
* metricsAdvisorAdminAsyncClient.getDataFeed(dataFeedId)
* .subscribe(dataFeed -> {
* System.out.printf("Data feed Id: %s%n", dataFeed.getId());
* System.out.printf("Data feed description: %s%n", dataFeed.getOptions().getDescription());
* System.out.printf("Data feed source type: %s%n", dataFeed.getSourceType());
* System.out.printf("Data feed creator: %s%n", dataFeed.getCreator());
* });
*
*
*
* @param dataFeedId The data feed unique id.
*
* @return The data feed for the provided id.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code dataFeedId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getDataFeed(String dataFeedId) {
return getDataFeedWithResponse(dataFeedId).flatMap(FluxUtil::toMono);
}
/**
* Get a data feed by its id with REST response.
*
* Code sample
*
*
* final String dataFeedId = "r47053f1-9080-09lo-bacf-8dccf2e86f";
* metricsAdvisorAdminAsyncClient.getDataFeedWithResponse(dataFeedId)
* .subscribe(dataFeedResponse -> {
* System.out.printf("Data feed get operation status: %s%n", dataFeedResponse.getStatusCode());
* DataFeed dataFeed = dataFeedResponse.getValue();
* System.out.printf("Data feed Id: %s%n", dataFeed.getId());
* System.out.printf("Data feed description: %s%n", dataFeed.getOptions().getDescription());
* System.out.printf("Data feed source type: %s%n", dataFeed.getSourceType());
* System.out.printf("Data feed creator: %s%n", dataFeed.getCreator());
* });
*
*
*
* @param dataFeedId The data feed unique id.
*
* @return The data feed for the provided id.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code dataFeedId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getDataFeedWithResponse(String dataFeedId) {
try {
return withContext(context -> getDataFeedWithResponse(dataFeedId, context));
} catch (RuntimeException ex) {
return monoError(logger, ex);
}
}
Mono> getDataFeedWithResponse(String dataFeedId, Context context) {
Objects.requireNonNull(dataFeedId, "'dataFeedId' cannot be null.");
return service.getDataFeedByIdWithResponseAsync(UUID.fromString(dataFeedId), context)
.map(response -> new SimpleResponse<>(response, DataFeedTransforms.fromInner(response.getValue())));
}
/**
* Update an existing data feed.
*
* Code sample
*
*
* final String dataFeedId = "r47053f1-9080-09lo-bacf-8dccf2e86f";
* metricsAdvisorAdminAsyncClient.getDataFeed(dataFeedId)
* .flatMap(existingDataFeed -> {
* return metricsAdvisorAdminAsyncClient.updateDataFeed(
* existingDataFeed
* .setOptions(new DataFeedOptions()
* .setDescription("set updated description"))
* );
* })
* .subscribe(updatedDataFeed -> {
* System.out.printf("Data feed Id: %s%n", updatedDataFeed.getId());
* System.out.printf("Data feed updated description: %s%n", updatedDataFeed.getOptions().getDescription());
* });
*
*
*
* @param dataFeed the data feed that needs to be updated.
*
* @return the updated data feed.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono updateDataFeed(DataFeed dataFeed) {
return updateDataFeedWithResponse(dataFeed).flatMap(FluxUtil::toMono);
}
/**
* Update an existing data feed with REST response.
*
* Code sample
*
*
* final String dataFeedId = "r47053f1-9080-09lo-bacf-8dccf2e86f";
* metricsAdvisorAdminAsyncClient.getDataFeed(dataFeedId)
* .flatMap(existingDataFeed -> {
* return metricsAdvisorAdminAsyncClient.updateDataFeedWithResponse(
* existingDataFeed
* .setOptions(new DataFeedOptions()
* .setDescription("set updated description"))
* );
* })
* .subscribe(dataFeedResponse -> {
* System.out.printf("Data feed update operation status: %s%n", dataFeedResponse.getStatusCode());
* DataFeed updatedDataFeed = dataFeedResponse.getValue();
* System.out.printf("Data feed Id: %s%n", updatedDataFeed.getId());
* System.out.printf("Data feed updated description: %s%n", updatedDataFeed.getOptions().getDescription());
* });
*
*
*
* @param dataFeed the data feed that needs to be updated.
*
* @return the {@link Response} of a {@link Mono} containing the updated {@link DataFeed data feed}.
**/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> updateDataFeedWithResponse(DataFeed dataFeed) {
try {
return withContext(context -> updateDataFeedWithResponse(dataFeed, context));
} catch (RuntimeException ex) {
return monoError(logger, ex);
}
}
Mono> updateDataFeedWithResponse(DataFeed dataFeed, Context context) {
final DataFeedIngestionSettings dataFeedIngestionSettings = dataFeed.getIngestionSettings();
final DataFeedOptions dataFeedOptions
= dataFeed.getOptions() == null ? new DataFeedOptions() : dataFeed.getOptions();
final DataFeedRollupSettings dataFeedRollupSettings = dataFeedOptions.getRollupSettings() == null
? new DataFeedRollupSettings()
: dataFeedOptions.getRollupSettings();
final DataFeedMissingDataPointFillSettings dataFeedMissingDataPointFillSettings
= dataFeedOptions.getMissingDataPointFillSettings() == null
? new DataFeedMissingDataPointFillSettings()
: dataFeedOptions.getMissingDataPointFillSettings();
return service
.updateDataFeedWithResponseAsync(UUID.fromString(dataFeed.getId()), DataFeedTransforms
.toInnerForUpdate(dataFeed.getSource())
.setDataFeedName(dataFeed.getName())
.setDataFeedDescription(dataFeedOptions.getDescription())
.setTimestampColumn(dataFeed.getSchema() == null ? null : dataFeed.getSchema().getTimestampColumn())
.setDataStartFrom(dataFeed.getIngestionSettings().getIngestionStartTime())
.setStartOffsetInSeconds(dataFeedIngestionSettings.getIngestionStartOffset() == null
? null
: dataFeedIngestionSettings.getIngestionStartOffset().getSeconds())
.setMaxConcurrency(dataFeedIngestionSettings.getDataSourceRequestConcurrency())
.setStopRetryAfterInSeconds(dataFeedIngestionSettings.getStopRetryAfter() == null
? null
: dataFeedIngestionSettings.getStopRetryAfter().getSeconds())
.setMinRetryIntervalInSeconds(dataFeedIngestionSettings.getIngestionRetryDelay() == null
? null
: dataFeedIngestionSettings.getIngestionRetryDelay().getSeconds())
.setNeedRollup(dataFeedRollupSettings.getRollupType() != null
? NeedRollupEnum.fromString(dataFeedRollupSettings.getRollupType().toString())
: null)
.setRollUpColumns(dataFeedRollupSettings.getAutoRollupGroupByColumnNames())
.setRollUpMethod(dataFeedRollupSettings.getDataFeedAutoRollUpMethod() != null
? RollUpMethod.fromString(dataFeedRollupSettings.getDataFeedAutoRollUpMethod().toString())
: null)
.setAllUpIdentification(dataFeedRollupSettings.getRollupIdentificationValue())
.setFillMissingPointType(dataFeedMissingDataPointFillSettings.getFillType() != null
? FillMissingPointType.fromString(dataFeedMissingDataPointFillSettings.getFillType().toString())
: null)
.setFillMissingPointValue(
// For PATCH send 'fill-custom-value' over wire only for 'fill-custom-type'.
dataFeedMissingDataPointFillSettings.getFillType() == DataFeedMissingDataPointFillType.CUSTOM_VALUE
? dataFeedMissingDataPointFillSettings.getCustomFillValue()
: null)
.setViewMode(dataFeedOptions.getAccessMode() != null
? ViewMode.fromString(dataFeedOptions.getAccessMode().toString())
: null)
.setViewers(dataFeedOptions.getViewers())
.setAdmins(dataFeedOptions.getAdmins())
.setStatus(
dataFeed.getStatus() != null ? EntityStatus.fromString(dataFeed.getStatus().toString()) : null)
.setActionLinkTemplate(dataFeedOptions.getActionLinkTemplate()), context)
.flatMap(updatedDataFeedResponse -> getDataFeedWithResponse(dataFeed.getId()));
}
/**
* Delete a data feed.
*
* Code sample
*
*
* final String dataFeedId = "t00853f1-9080-447f-bacf-8dccf2e86f";
* metricsAdvisorAdminAsyncClient.deleteDataFeed(dataFeedId);
*
*
*
* @param dataFeedId The data feed unique id.
*
* @return An empty Mono.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code dataFeedId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono deleteDataFeed(String dataFeedId) {
return deleteDataFeedWithResponse(dataFeedId).flatMap(FluxUtil::toMono);
}
/**
* Delete a data feed with REST response.
*
* Code sample
*
*
* final String dataFeedId = "eh0854f1-8927-447f-bacf-8dccf2e86fwe";
* metricsAdvisorAdminAsyncClient.deleteDataFeedWithResponse(dataFeedId)
* .subscribe(response ->
* System.out.printf("Data feed delete operation status : %s%n", response.getStatusCode()));
*
*
*
* @param dataFeedId The data feed unique id.
*
* @return A response containing status code and headers returned after the operation.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code dataFeedId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> deleteDataFeedWithResponse(String dataFeedId) {
try {
return withContext(context -> deleteDataFeedWithResponse(dataFeedId, context));
} catch (RuntimeException ex) {
return monoError(logger, ex);
}
}
Mono> deleteDataFeedWithResponse(String dataFeedId, Context context) {
Objects.requireNonNull(dataFeedId, "'dataFeedId' cannot be null.");
return service.deleteDataFeedWithResponseAsync(UUID.fromString(dataFeedId), context)
.map(response -> new SimpleResponse<>(response, null));
}
/**
* List information of all data feeds on the metrics advisor account.
*
* Code sample
*
*
* metricsAdvisorAdminAsyncClient.listDataFeeds()
* .subscribe(dataFeed -> {
* System.out.printf("Data feed Id: %s%n", dataFeed.getId());
* System.out.printf("Data feed description: %s%n", dataFeed.getOptions().getDescription());
* System.out.printf("Data feed source type: %s%n", dataFeed.getSourceType());
* System.out.printf("Data feed creator: %s%n", dataFeed.getCreator());
* });
*
*
*
* @return A {@link PagedFlux} containing information of all the {@link DataFeed data feeds} in the account.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listDataFeeds() {
return listDataFeeds(new ListDataFeedOptions());
}
/**
* List information of all data feeds on the metrics advisor account.
*
* Code sample
*
*
* metricsAdvisorAdminAsyncClient.listDataFeeds(
* new ListDataFeedOptions()
* .setListDataFeedFilter(
* new ListDataFeedFilter()
* .setDataFeedStatus(DataFeedStatus.ACTIVE)
* .setDataFeedGranularityType(DataFeedGranularityType.DAILY))
* .setMaxPageSize(3))
* .subscribe(dataFeed -> {
* System.out.printf("Data feed Id: %s%n", dataFeed.getId());
* System.out.printf("Data feed description: %s%n", dataFeed.getOptions().getDescription());
* System.out.printf("Data feed source type: %s%n", dataFeed.getSourceType());
* System.out.printf("Data feed creator: %s%n", dataFeed.getCreator());
* System.out.printf("Data feed status: %s%n", dataFeed.getStatus());
* System.out.printf("Data feed granularity type: %s%n", dataFeed.getGranularity().getGranularityType());
* });
*
*
*
* @param listDataFeedOptions The configurable {@link ListDataFeedOptions options} to pass for filtering the output result.
*
* @return A {@link PagedFlux} containing information of all the {@link DataFeed data feeds} in the account.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listDataFeeds(ListDataFeedOptions listDataFeedOptions) {
try {
return new PagedFlux<>(
() -> withContext(context -> listDataFeedsSinglePageAsync(listDataFeedOptions, context)),
continuationToken -> withContext(context -> listDataFeedsNextPageAsync(continuationToken, context)));
} catch (RuntimeException ex) {
return new PagedFlux<>(() -> monoError(logger, ex));
}
}
private Mono> listDataFeedsSinglePageAsync(ListDataFeedOptions options, Context context) {
options = options != null ? options : new ListDataFeedOptions();
final ListDataFeedFilter dataFeedFilter
= options.getListDataFeedFilter() != null ? options.getListDataFeedFilter() : new ListDataFeedFilter();
return service.listDataFeedsSinglePageAsync(dataFeedFilter.getName(),
dataFeedFilter.getSourceType() != null
? DataSourceType.fromString(dataFeedFilter.getSourceType().toString())
: null,
dataFeedFilter.getGranularityType() != null
? Granularity.fromString(dataFeedFilter.getGranularityType().toString())
: null,
dataFeedFilter.getStatus() != null ? EntityStatus.fromString(dataFeedFilter.getStatus().toString()) : null,
dataFeedFilter.getCreator(), options.getSkip(), options.getMaxPageSize(), context)
.doOnRequest(ignoredValue -> logger.info("Listing information for all data feeds"))
.doOnSuccess(response -> logger.info("Listed data feeds {}", response))
.doOnError(error -> logger.warning("Failed to list all data feeds information - {}", error))
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().stream().map(DataFeedTransforms::fromInner).collect(Collectors.toList()),
res.getContinuationToken(), null));
}
private Mono> listDataFeedsNextPageAsync(String nextPageLink, Context context) {
if (CoreUtils.isNullOrEmpty(nextPageLink)) {
return Mono.empty();
}
return service.listDataFeedsNextSinglePageAsync(nextPageLink, context)
.doOnSubscribe(ignoredValue -> logger.info("Retrieving the next listing page - Page {}", nextPageLink))
.doOnSuccess(response -> logger.info("Retrieved the next listing page - Page {}", nextPageLink))
.doOnError(
error -> logger.warning("Failed to retrieve the next listing page - Page {}", nextPageLink, error))
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().stream().map(DataFeedTransforms::fromInner).collect(Collectors.toList()),
res.getContinuationToken(), null));
}
/**
* Fetch the ingestion status of a data feed.
*
* Code sample
*
*
* final String dataFeedId = "4957a2f7-a0f4-4fc0-b8d7-d866c1df0f4c";
* final OffsetDateTime startTime = OffsetDateTime.parse("2020-01-01T00:00:00Z");
* final OffsetDateTime endTime = OffsetDateTime.parse("2020-09-09T00:00:00Z");
* final ListDataFeedIngestionOptions options = new ListDataFeedIngestionOptions(startTime, endTime);
* metricsAdvisorAdminAsyncClient.listDataFeedIngestionStatus(dataFeedId, options)
* .subscribe(ingestionStatus -> {
* System.out.printf("Timestamp: %s%n", ingestionStatus.getTimestamp());
* System.out.printf("Status: %s%n", ingestionStatus.getStatus());
* System.out.printf("Message: %s%n", ingestionStatus.getMessage());
* });
*
*
*
* @param dataFeedId The data feed id.
* @param listDataFeedIngestionOptions The additional parameters.
*
* @return The ingestion statuses.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException If {@code dataFeedId}, {@code options}, {@code options.startTime},
* {@code options.endTime} is null.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listDataFeedIngestionStatus(String dataFeedId,
ListDataFeedIngestionOptions listDataFeedIngestionOptions) {
try {
return new PagedFlux<>(
() -> withContext(context -> listDataFeedIngestionStatusSinglePageAsync(dataFeedId,
listDataFeedIngestionOptions, context)),
continuationToken -> withContext(context -> listDataFeedIngestionStatusNextPageAsync(continuationToken,
listDataFeedIngestionOptions, context)));
} catch (RuntimeException ex) {
return new PagedFlux<>(() -> FluxUtil.monoError(logger, ex));
}
}
private Mono> listDataFeedIngestionStatusSinglePageAsync(String dataFeedId,
ListDataFeedIngestionOptions options, Context context) {
Objects.requireNonNull(dataFeedId, "'dataFeedId' is required.");
Objects.requireNonNull(options, "'options' is required.");
Objects.requireNonNull(options.getStartTime(), "'options.startTime' is required.");
Objects.requireNonNull(options.getEndTime(), "'options.endTime' is required.");
IngestionStatusQueryOptions queryOptions
= new IngestionStatusQueryOptions().setStartTime(options.getStartTime()).setEndTime(options.getEndTime());
return service
.getDataFeedIngestionStatusSinglePageAsync(UUID.fromString(dataFeedId), queryOptions, options.getSkip(),
options.getMaxPageSize(), context)
.doOnRequest(ignoredValue -> logger.info("Listing ingestion status for data feed"))
.doOnSuccess(response -> logger.info("Listed ingestion status {}", response))
.doOnError(error -> logger.warning("Failed to ingestion status for data feed", error))
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
toDataFeedIngestionStatus(res.getValue()), res.getContinuationToken(), null));
}
private Mono> listDataFeedIngestionStatusNextPageAsync(String nextPageLink,
ListDataFeedIngestionOptions options, Context context) {
if (CoreUtils.isNullOrEmpty(nextPageLink)) {
return Mono.empty();
}
IngestionStatusQueryOptions queryOptions
= new IngestionStatusQueryOptions().setStartTime(options.getStartTime()).setEndTime(options.getEndTime());
return service.getDataFeedIngestionStatusNextSinglePageAsync(nextPageLink, queryOptions, context)
.doOnSubscribe(ignoredValue -> logger.info("Retrieving the next listing page - Page {}", nextPageLink))
.doOnSuccess(
response -> logger.info("Retrieved the next listing page - Page {} {}", nextPageLink, response))
.doOnError(
error -> logger.warning("Failed to retrieve the next listing page - Page {}", nextPageLink, error))
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
toDataFeedIngestionStatus(res.getValue()), res.getContinuationToken(), null));
}
/**
* Refresh data ingestion for a period.
*
* The data in the data source for the given period will be re-ingested
* and any ingested data for the same period will be overwritten.
*
*
* Code sample
*
*
* final String dataFeedId = "4957a2f7-a0f4-4fc0-b8d7-d866c1df0f4c";
* final OffsetDateTime startTime = OffsetDateTime.parse("2020-01-01T00:00:00Z");
* final OffsetDateTime endTime = OffsetDateTime.parse("2020-03-03T00:00:00Z");
* metricsAdvisorAdminAsyncClient.refreshDataFeedIngestion(dataFeedId,
* startTime,
* endTime).subscribe();
*
*
*
* @param dataFeedId The data feed id.
* @param startTime The start point of the period.
* @param endTime The end point of the period.
*
* @return A {@link Mono} indicating ingestion reset success or failure.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException If {@code dataFeedId}, {@code startTime}, {@code endTime} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono refreshDataFeedIngestion(String dataFeedId, OffsetDateTime startTime, OffsetDateTime endTime) {
return refreshDataFeedIngestionWithResponse(dataFeedId, startTime, endTime).then();
}
/**
* Refresh data ingestion for a period.
*
* The data in the data source for the given period will be re-ingested
* and any ingested data for the same period will be overwritten.
*
*
* Code sample
*
*
* final String dataFeedId = "4957a2f7-a0f4-4fc0-b8d7-d866c1df0f4c";
* final OffsetDateTime startTime = OffsetDateTime.parse("2020-01-01T00:00:00Z");
* final OffsetDateTime endTime = OffsetDateTime.parse("2020-03-03T00:00:00Z");
* metricsAdvisorAdminAsyncClient.refreshDataFeedIngestionWithResponse(dataFeedId,
* startTime,
* endTime)
* .subscribe(response -> {
* System.out.printf("Response statusCode: %d%n", response.getStatusCode());
* });
*
*
*
* @param dataFeedId The data feed id.
* @param startTime The start point of the period.
* @param endTime The end point of the period.
*
* @return A {@link Response} of a {@link Mono} with result of reset request.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException If {@code dataFeedId}, {@code startTime}, {@code endTime} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> refreshDataFeedIngestionWithResponse(String dataFeedId, OffsetDateTime startTime,
OffsetDateTime endTime) {
try {
return withContext(
context -> refreshDataFeedIngestionWithResponse(dataFeedId, startTime, endTime, context));
} catch (RuntimeException e) {
return FluxUtil.monoError(logger, e);
}
}
Mono> refreshDataFeedIngestionWithResponse(String dataFeedId, OffsetDateTime startTime,
OffsetDateTime endTime, Context context) {
Objects.requireNonNull(dataFeedId, "'dataFeedId' is required.");
Objects.requireNonNull(startTime, "'startTime' is required.");
Objects.requireNonNull(endTime, "'endTime' is required.");
return service
.resetDataFeedIngestionStatusWithResponseAsync(UUID.fromString(dataFeedId),
new IngestionProgressResetOptions().setStartTime(startTime).setEndTime(endTime), context)
.doOnRequest(ignoredValue -> logger.info("Resetting ingestion status for the data feed"))
.doOnSuccess(response -> logger.info("Ingestion status got reset {}", response))
.doOnError(error -> logger.warning("Failed to reset ingestion status for the data feed", error));
}
/**
* Retrieve the ingestion progress of a data feed.
*
* Code sample
*
*
* final String dataFeedId = "4957a2f7-a0f4-4fc0-b8d7-d866c1df0f4c";
* metricsAdvisorAdminAsyncClient.getDataFeedIngestionProgress(dataFeedId)
* .subscribe(ingestionProgress -> {
* System.out.printf("Latest active timestamp: %s%n", ingestionProgress.getLatestActiveTimestamp());
* System.out.printf("Latest successful timestamp: %s%n", ingestionProgress.getLatestSuccessTimestamp());
* });
*
*
*
* @param dataFeedId The data feed id.
*
* @return A {@link Mono} containing {@link DataFeedIngestionProgress} of the data feed.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code dataFeedId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getDataFeedIngestionProgress(String dataFeedId) {
return getDataFeedIngestionProgressWithResponse(dataFeedId, Context.NONE).map(Response::getValue);
}
/**
* Retrieve the ingestion progress of a data feed.
*
* Code sample
*
*
* final String dataFeedId = "4957a2f7-a0f4-4fc0-b8d7-d866c1df0f4c";
* metricsAdvisorAdminAsyncClient.getDataFeedIngestionProgressWithResponse(dataFeedId, Context.NONE)
* .subscribe(response -> {
* System.out.printf("Response statusCode: %d%n", response.getStatusCode());
* DataFeedIngestionProgress ingestionProgress = response.getValue();
* System.out.printf("Latest active timestamp: %s%n", ingestionProgress.getLatestActiveTimestamp());
* System.out.printf("Latest successful timestamp: %s%n", ingestionProgress.getLatestSuccessTimestamp());
* });
*
*
*
* @param dataFeedId The data feed id.
*
* @return A {@link Response} of a {@link Mono} containing {@link DataFeedIngestionProgress} of the data feed.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code dataFeedId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getDataFeedIngestionProgressWithResponse(String dataFeedId) {
try {
return withContext(context -> getDataFeedIngestionProgressWithResponse(dataFeedId, context));
} catch (RuntimeException e) {
return FluxUtil.monoError(logger, e);
}
}
Mono> getDataFeedIngestionProgressWithResponse(String dataFeedId,
Context context) {
Objects.requireNonNull(dataFeedId, "'dataFeedId' is required.");
return service.getIngestionProgressWithResponseAsync(UUID.fromString(dataFeedId), context)
.doOnRequest(ignoredValue -> logger.info("Retrieving ingestion progress for metric"))
.doOnSuccess(response -> logger.info("Retrieved ingestion progress {}", response))
.doOnError(error -> logger.warning("Failed to retrieve ingestion progress for metric", error))
.map(response -> new SimpleResponse<>(response, toDataFeedIngestionProgress(response.getValue())));
}
/**
* Create a configuration to detect anomalies in the time series of a metric.
*
* Code sample
*
*
* final MetricWholeSeriesDetectionCondition wholeSeriesCondition = new MetricWholeSeriesDetectionCondition()
* .setConditionOperator(DetectionConditionOperator.OR)
* .setSmartDetectionCondition(new SmartDetectionCondition(
* 50,
* AnomalyDetectorDirection.BOTH,
* new SuppressCondition(50, 50)))
* .setHardThresholdCondition(new HardThresholdCondition(
* AnomalyDetectorDirection.BOTH,
* new SuppressCondition(5, 5))
* .setLowerBound(0.0)
* .setUpperBound(100.0))
* .setChangeThresholdCondition(new ChangeThresholdCondition(
* 50,
* 30,
* true,
* AnomalyDetectorDirection.BOTH,
* new SuppressCondition(2, 2)));
*
* final String detectionConfigName = "my_detection_config";
* final String detectionConfigDescription = "anomaly detection config for metric";
* final AnomalyDetectionConfiguration detectionConfig
* = new AnomalyDetectionConfiguration(detectionConfigName)
* .setDescription(detectionConfigDescription)
* .setWholeSeriesDetectionCondition(wholeSeriesCondition);
*
* final String metricId = "0b836da8-10e6-46cd-8f4f-28262e113a62";
* metricsAdvisorAdminAsyncClient
* .createDetectionConfig(metricId, detectionConfig)
* .subscribe(createdDetectionConfig -> {
* System.out.printf("Detection config Id: %s%n", createdDetectionConfig.getId());
* System.out.printf("Name: %s%n", createdDetectionConfig.getName());
* System.out.printf("Description: %s%n", createdDetectionConfig.getDescription());
* System.out.printf("MetricId: %s%n", createdDetectionConfig.getMetricId());
* });
*
*
*
* @param metricId The metric id to associate the configuration with.
* @param detectionConfiguration The anomaly detection configuration.
* @return A {@link Mono} containing the created {@link AnomalyDetectionConfiguration}.
* @throws IllegalArgumentException If {@code metricId} does not conform to the UUID
* format specification, or {@code detectionConfiguration.name} is not set.
* @throws NullPointerException thrown if the {@code metricId} is null
* or {@code detectionConfiguration} is null
* or {@code detectionConfiguration.wholeSeriesCondition} is null
* or {@code seriesKey} is missing for any {@code MetricSingleSeriesDetectionCondition} in the configuration
* or {@code seriesGroupKey} is missing for any {@code MetricSeriesGroupDetectionCondition} in the configuration
* or {@code conditionOperator} is missing when multiple nested conditions are set in a
* {@code MetricSingleSeriesDetectionCondition} or {@code MetricSeriesGroupDetectionCondition}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono createDetectionConfig(String metricId,
AnomalyDetectionConfiguration detectionConfiguration) {
return createDetectionConfigWithResponse(metricId, detectionConfiguration).map(Response::getValue);
}
/**
* Create a configuration to detect anomalies in the time series of a metric.
*
* Code sample
*
*
* final MetricWholeSeriesDetectionCondition wholeSeriesCondition = new MetricWholeSeriesDetectionCondition()
* .setConditionOperator(DetectionConditionOperator.OR)
* .setSmartDetectionCondition(new SmartDetectionCondition(
* 50,
* AnomalyDetectorDirection.BOTH,
* new SuppressCondition(50, 50)))
* .setHardThresholdCondition(new HardThresholdCondition(
* AnomalyDetectorDirection.BOTH,
* new SuppressCondition(5, 5))
* .setLowerBound(0.0)
* .setUpperBound(100.0))
* .setChangeThresholdCondition(new ChangeThresholdCondition(
* 50,
* 30,
* true,
* AnomalyDetectorDirection.BOTH,
* new SuppressCondition(2, 2)));
*
* final String detectionConfigName = "my_detection_config";
* final String detectionConfigDescription = "anomaly detection config for metric";
* final AnomalyDetectionConfiguration detectionConfig
* = new AnomalyDetectionConfiguration(detectionConfigName)
* .setDescription(detectionConfigDescription)
* .setWholeSeriesDetectionCondition(wholeSeriesCondition);
*
* final String metricId = "0b836da8-10e6-46cd-8f4f-28262e113a62";
* metricsAdvisorAdminAsyncClient
* .createDetectionConfigWithResponse(metricId, detectionConfig)
* .subscribe(response -> {
* System.out.printf("Response statusCode: %d%n", response.getStatusCode());
* AnomalyDetectionConfiguration createdDetectionConfig = response.getValue();
* System.out.printf("Detection config Id: %s%n", createdDetectionConfig.getId());
* System.out.printf("Name: %s%n", createdDetectionConfig.getName());
* System.out.printf("Description: %s%n", createdDetectionConfig.getDescription());
* System.out.printf("MetricId: %s%n", createdDetectionConfig.getMetricId());
* });
*
*
*
* @param metricId The metric id to associate the configuration with.
* @param detectionConfiguration The anomaly detection configuration.
* @return A {@link Response} of a {@link Mono} containing the created {@link AnomalyDetectionConfiguration}.
* @throws IllegalArgumentException If {@code metricId} does not conform to the UUID
* format specification, or {@code detectionConfiguration.name} is not set.
* @throws NullPointerException thrown if the {@code metricId} is null
* or {@code detectionConfiguration} is null
* or {@code detectionConfiguration.wholeSeriesCondition} is null
* or {@code seriesKey} is missing for any {@code MetricSingleSeriesDetectionCondition} in the configuration
* or {@code seriesGroupKey} is missing for any {@code MetricSeriesGroupDetectionCondition} in the configuration
* or {@code conditionOperator} is missing when multiple nested conditions are set in a
* {@code MetricSingleSeriesDetectionCondition} or {@code MetricSeriesGroupDetectionCondition}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> createDetectionConfigWithResponse(String metricId,
AnomalyDetectionConfiguration detectionConfiguration) {
try {
return withContext(context -> createDetectionConfigWithResponse(metricId, detectionConfiguration, context));
} catch (RuntimeException e) {
return FluxUtil.monoError(logger, e);
}
}
Mono> createDetectionConfigWithResponse(String metricId,
AnomalyDetectionConfiguration detectionConfiguration, Context context) {
Objects.requireNonNull(metricId, "metricId is required");
Objects.requireNonNull(detectionConfiguration, "detectionConfiguration is required");
final com.azure.ai.metricsadvisor.implementation.models.AnomalyDetectionConfiguration innerDetectionConfiguration
= DetectionConfigurationTransforms.toInnerForCreate(logger, metricId, detectionConfiguration);
return service.createAnomalyDetectionConfigurationWithResponseAsync(innerDetectionConfiguration, context)
.doOnSubscribe(ignoredValue -> logger.info("Creating AnomalyDetectionConfiguration"))
.doOnSuccess(response -> logger.info("Created AnomalyDetectionConfiguration"))
.doOnError(error -> logger.warning("Failed to create AnomalyDetectionConfiguration", error))
.flatMap(response -> {
final String configurationId
= Utility.parseOperationId(response.getDeserializedHeaders().getLocation());
return getDetectionConfigWithResponse(configurationId, context)
.map(configurationResponse -> new ResponseBase(
response.getRequest(), response.getStatusCode(), response.getHeaders(),
configurationResponse.getValue(), null));
});
}
/**
* Get the anomaly detection configuration by its id.
*
* Code sample
*
*
* final String detectionConfigId = "7b8069a1-1564-46da-9f50-b5d0dd9129ab";
* metricsAdvisorAdminAsyncClient
* .getDetectionConfig(detectionConfigId)
* .subscribe(detectionConfig -> {
* System.out.printf("Detection config Id: %s%n", detectionConfig.getId());
* System.out.printf("Name: %s%n", detectionConfig.getName());
* System.out.printf("Description: %s%n", detectionConfig.getDescription());
* System.out.printf("MetricId: %s%n", detectionConfig.getMetricId());
*
* System.out.printf("Detection conditions specified for configuration...%n");
*
* System.out.printf("Whole Series Detection Conditions:%n");
* MetricWholeSeriesDetectionCondition wholeSeriesDetectionCondition
* = detectionConfig.getWholeSeriesDetectionCondition();
*
* System.out.printf("- Use %s operator for multiple detection conditions:%n",
* wholeSeriesDetectionCondition.getConditionOperator());
*
* System.out.printf("- Smart Detection Condition:%n");
* System.out.printf(" - Sensitivity: %s%n",
* wholeSeriesDetectionCondition.getSmartDetectionCondition()
* .getSensitivity());
* System.out.printf(" - Detection direction: %s%n",
* wholeSeriesDetectionCondition.getSmartDetectionCondition()
* .getAnomalyDetectorDirection());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* wholeSeriesDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinNumber(),
* wholeSeriesDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf("- Hard Threshold Condition:%n");
* System.out.printf(" - Lower bound: %s%n",
* wholeSeriesDetectionCondition.getHardThresholdCondition()
* .getLowerBound());
* System.out.printf(" - Upper bound: %s%n",
* wholeSeriesDetectionCondition.getHardThresholdCondition()
* .getUpperBound());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* wholeSeriesDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* wholeSeriesDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf("- Change Threshold Condition:%n");
* System.out.printf(" - Change percentage: %s%n",
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .getChangePercentage());
* System.out.printf(" - Shift point: %s%n",
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .getShiftPoint());
* System.out.printf(" - Detect anomaly if within range: %s%n",
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .isWithinRange());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinRatio());
*
* List<MetricSingleSeriesDetectionCondition> seriesDetectionConditions
* = detectionConfig.getSeriesDetectionConditions();
* System.out.printf("Series Detection Conditions:%n");
* for (MetricSingleSeriesDetectionCondition seriesDetectionCondition : seriesDetectionConditions) {
* DimensionKey seriesKey = seriesDetectionCondition.getSeriesKey();
* final String seriesKeyStr
* = Arrays.toString(seriesKey.asMap().entrySet().stream().toArray());
* System.out.printf("- Series Key: %s%n", seriesKeyStr);
* System.out.printf(" - Use %s operator for multiple detection conditions:%n",
* seriesDetectionCondition.getConditionOperator());
*
* System.out.printf(" - Smart Detection Condition:%n");
* System.out.printf(" - Sensitivity: %s%n",
* seriesDetectionCondition.getSmartDetectionCondition()
* .getSensitivity());
* System.out.printf(" - Detection direction: %s%n",
* seriesDetectionCondition.getSmartDetectionCondition()
* .getAnomalyDetectorDirection());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinNumber(),
* seriesDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf(" - Hard Threshold Condition:%n");
* System.out.printf(" - Lower bound: %s%n",
* seriesDetectionCondition.getHardThresholdCondition()
* .getLowerBound());
* System.out.printf(" - Upper bound: %s%n",
* seriesDetectionCondition.getHardThresholdCondition()
* .getUpperBound());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* seriesDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf(" - Change Threshold Condition:%n");
* System.out.printf(" - Change percentage: %s%n",
* seriesDetectionCondition.getChangeThresholdCondition()
* .getChangePercentage());
* System.out.printf(" - Shift point: %s%n",
* seriesDetectionCondition.getChangeThresholdCondition()
* .getShiftPoint());
* System.out.printf(" - Detect anomaly if within range: %s%n",
* seriesDetectionCondition.getChangeThresholdCondition()
* .isWithinRange());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* seriesDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinRatio());
* }
*
* List<MetricSeriesGroupDetectionCondition> seriesGroupDetectionConditions
* = detectionConfig.getSeriesGroupDetectionConditions();
* System.out.printf("Series Group Detection Conditions:%n");
* for (MetricSeriesGroupDetectionCondition seriesGroupDetectionCondition
* : seriesGroupDetectionConditions) {
* DimensionKey seriesGroupKey = seriesGroupDetectionCondition.getSeriesGroupKey();
* final String seriesGroupKeyStr
* = Arrays.toString(seriesGroupKey.asMap().entrySet().stream().toArray());
* System.out.printf("- Series Group Key: %s%n", seriesGroupKeyStr);
* System.out.printf(" - Use %s operator for multiple detection conditions:%n",
* seriesGroupDetectionCondition.getConditionOperator());
*
* System.out.printf(" - Smart Detection Condition:%n");
* System.out.printf(" - Sensitivity: %s%n",
* seriesGroupDetectionCondition.getSmartDetectionCondition()
* .getSensitivity());
* System.out.printf(" - Detection direction: %s%n",
* seriesGroupDetectionCondition.getSmartDetectionCondition()
* .getAnomalyDetectorDirection());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesGroupDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinNumber(),
* seriesGroupDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf(" - Hard Threshold Condition:%n");
* System.out.printf(" - Lower bound: %s%n",
* seriesGroupDetectionCondition.getHardThresholdCondition()
* .getLowerBound());
* System.out.printf(" - Upper bound: %s%n",
* seriesGroupDetectionCondition.getHardThresholdCondition()
* .getUpperBound());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesGroupDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* seriesGroupDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf(" - Change Threshold Condition:%n");
* System.out.printf(" - Change percentage: %s%n",
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .getChangePercentage());
* System.out.printf(" - Shift point: %s%n",
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .getShiftPoint());
* System.out.printf(" - Detect anomaly if within range: %s%n",
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .isWithinRange());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinRatio());
* }
* });
*
*
*
* @param detectionConfigurationId The anomaly detection configuration id.
* @return A {@link Mono} containing the {@link AnomalyDetectionConfiguration} for the provided id.
* @throws IllegalArgumentException If {@code detectionConfigurationId} does not conform to the UUID
* format specification.
* @throws NullPointerException thrown if the {@code detectionConfigurationId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getDetectionConfig(String detectionConfigurationId) {
return getDetectionConfigWithResponse(detectionConfigurationId).map(Response::getValue);
}
/**
* Get the anomaly detection configuration by its id.
*
* Code sample
*
*
* final String detectionConfigId = "7b8069a1-1564-46da-9f50-b5d0dd9129ab";
* metricsAdvisorAdminAsyncClient
* .getDetectionConfigWithResponse(detectionConfigId)
* .subscribe(response -> {
* System.out.printf("Response statusCode: %d%n", response.getStatusCode());
*
* AnomalyDetectionConfiguration detectionConfig = response.getValue();
* System.out.printf("Detection config Id: %s%n", detectionConfig.getId());
* System.out.printf("Name: %s%n", detectionConfig.getName());
* System.out.printf("Description: %s%n", detectionConfig.getDescription());
* System.out.printf("MetricId: %s%n", detectionConfig.getMetricId());
*
* System.out.printf("Detection conditions specified for configuration...%n");
*
* System.out.printf("Whole Series Detection Conditions:%n");
* MetricWholeSeriesDetectionCondition wholeSeriesDetectionCondition
* = detectionConfig.getWholeSeriesDetectionCondition();
*
* System.out.printf("- Use %s operator for multiple detection conditions:%n",
* wholeSeriesDetectionCondition.getConditionOperator());
*
* System.out.printf("- Smart Detection Condition:%n");
* System.out.printf(" - Sensitivity: %s%n",
* wholeSeriesDetectionCondition.getSmartDetectionCondition()
* .getSensitivity());
* System.out.printf(" - Detection direction: %s%n",
* wholeSeriesDetectionCondition.getSmartDetectionCondition()
* .getAnomalyDetectorDirection());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* wholeSeriesDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinNumber(),
* wholeSeriesDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf("- Hard Threshold Condition:%n");
* System.out.printf(" - Lower bound: %s%n",
* wholeSeriesDetectionCondition.getHardThresholdCondition()
* .getLowerBound());
* System.out.printf(" - Upper bound: %s%n",
* wholeSeriesDetectionCondition.getHardThresholdCondition()
* .getUpperBound());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* wholeSeriesDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* wholeSeriesDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf("- Change Threshold Condition:%n");
* System.out.printf(" - Change percentage: %s%n",
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .getChangePercentage());
* System.out.printf(" - Shift point: %s%n",
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .getShiftPoint());
* System.out.printf(" - Detect anomaly if within range: %s%n",
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .isWithinRange());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinRatio());
*
* List<MetricSingleSeriesDetectionCondition> seriesDetectionConditions
* = detectionConfig.getSeriesDetectionConditions();
* System.out.printf("Series Detection Conditions:%n");
* for (MetricSingleSeriesDetectionCondition seriesDetectionCondition : seriesDetectionConditions) {
* DimensionKey seriesKey = seriesDetectionCondition.getSeriesKey();
* final String seriesKeyStr
* = Arrays.toString(seriesKey.asMap().entrySet().stream().toArray());
* System.out.printf("- Series Key: %s%n", seriesKeyStr);
* System.out.printf(" - Use %s operator for multiple detection conditions:%n",
* seriesDetectionCondition.getConditionOperator());
*
* System.out.printf(" - Smart Detection Condition:%n");
* System.out.printf(" - Sensitivity: %s%n",
* seriesDetectionCondition.getSmartDetectionCondition()
* .getSensitivity());
* System.out.printf(" - Detection direction: %s%n",
* seriesDetectionCondition.getSmartDetectionCondition()
* .getAnomalyDetectorDirection());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinNumber(),
* seriesDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf(" - Hard Threshold Condition:%n");
* System.out.printf(" - Lower bound: %s%n",
* seriesDetectionCondition.getHardThresholdCondition()
* .getLowerBound());
* System.out.printf(" - Upper bound: %s%n",
* seriesDetectionCondition.getHardThresholdCondition()
* .getUpperBound());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* seriesDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf(" - Change Threshold Condition:%n");
* System.out.printf(" - Change percentage: %s%n",
* seriesDetectionCondition.getChangeThresholdCondition()
* .getChangePercentage());
* System.out.printf(" - Shift point: %s%n",
* seriesDetectionCondition.getChangeThresholdCondition()
* .getShiftPoint());
* System.out.printf(" - Detect anomaly if within range: %s%n",
* seriesDetectionCondition.getChangeThresholdCondition()
* .isWithinRange());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* seriesDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinRatio());
* }
*
* List<MetricSeriesGroupDetectionCondition> seriesGroupDetectionConditions
* = detectionConfig.getSeriesGroupDetectionConditions();
* System.out.printf("Series Group Detection Conditions:%n");
* for (MetricSeriesGroupDetectionCondition seriesGroupDetectionCondition
* : seriesGroupDetectionConditions) {
* DimensionKey seriesGroupKey = seriesGroupDetectionCondition.getSeriesGroupKey();
* final String seriesGroupKeyStr
* = Arrays.toString(seriesGroupKey.asMap().entrySet().stream().toArray());
* System.out.printf("- Series Group Key: %s%n", seriesGroupKeyStr);
* System.out.printf(" - Use %s operator for multiple detection conditions:%n",
* seriesGroupDetectionCondition.getConditionOperator());
*
* System.out.printf(" - Smart Detection Condition:%n");
* System.out.printf(" - Sensitivity: %s%n",
* seriesGroupDetectionCondition.getSmartDetectionCondition()
* .getSensitivity());
* System.out.printf(" - Detection direction: %s%n",
* seriesGroupDetectionCondition.getSmartDetectionCondition()
* .getAnomalyDetectorDirection());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesGroupDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinNumber(),
* seriesGroupDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf(" - Hard Threshold Condition:%n");
* System.out.printf(" - Lower bound: %s%n",
* seriesGroupDetectionCondition.getHardThresholdCondition()
* .getLowerBound());
* System.out.printf(" - Upper bound: %s%n",
* seriesGroupDetectionCondition.getHardThresholdCondition()
* .getUpperBound());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesGroupDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* seriesGroupDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf(" - Change Threshold Condition:%n");
* System.out.printf(" - Change percentage: %s%n",
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .getChangePercentage());
* System.out.printf(" - Shift point: %s%n",
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .getShiftPoint());
* System.out.printf(" - Detect anomaly if within range: %s%n",
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .isWithinRange());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinRatio());
* }
* });
*
*
*
* @param detectionConfigurationId The anomaly detection configuration id.
* @return A {@link Response} of a {@link Mono} containing the {@link AnomalyDetectionConfiguration}
* for the provided id.
* @throws IllegalArgumentException If {@code detectionConfigurationId} does not conform to the UUID
* format specification.
* @throws NullPointerException thrown if the {@code detectionConfigurationId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>
getDetectionConfigWithResponse(String detectionConfigurationId) {
try {
return withContext(context -> getDetectionConfigWithResponse(detectionConfigurationId, context));
} catch (RuntimeException e) {
return FluxUtil.monoError(logger, e);
}
}
Mono> getDetectionConfigWithResponse(String detectionConfigurationId,
Context context) {
Objects.requireNonNull(detectionConfigurationId, "detectionConfigurationId is required.");
return service
.getAnomalyDetectionConfigurationWithResponseAsync(UUID.fromString(detectionConfigurationId), context)
.doOnSubscribe(
ignoredValue -> logger.info("Retrieving AnomalyDetectionConfiguration - {}", detectionConfigurationId))
.doOnSuccess(response -> logger.info("Retrieved AnomalyDetectionConfiguration - {}", response))
.doOnError(error -> logger.warning("Failed to retrieve AnomalyDetectionConfiguration - {}",
detectionConfigurationId, error))
.map(response -> {
AnomalyDetectionConfiguration configuration
= DetectionConfigurationTransforms.fromInner(response.getValue());
return new ResponseBase(response.getRequest(),
response.getStatusCode(), response.getHeaders(), configuration, null);
});
}
/**
* Update a configuration to detect anomalies in the time series of a metric.
*
* Code sample
*
*
* final String detectionConfigId = "7b8069a1-1564-46da-9f50-b5d0dd9129ab";
* metricsAdvisorAdminAsyncClient
* .getDetectionConfig(detectionConfigId)
* .flatMap(detectionConfig -> {
* detectionConfig.setName("updated config name");
* detectionConfig.setDescription("updated with more detection conditions");
*
* DimensionKey seriesGroupKey = new DimensionKey()
* .put("city", "Seoul");
* detectionConfig.addSeriesGroupDetectionCondition(
* new MetricSeriesGroupDetectionCondition(seriesGroupKey)
* .setSmartDetectionCondition(new SmartDetectionCondition(
* 10.0,
* AnomalyDetectorDirection.UP,
* new SuppressCondition(2, 2))));
* return metricsAdvisorAdminAsyncClient
* .updateDetectionConfig(detectionConfig);
* })
* .subscribe(updatedDetectionConfig -> {
* System.out.printf("Detection config Id: %s%n", updatedDetectionConfig.getId());
* System.out.printf("Name: %s%n", updatedDetectionConfig.getName());
* System.out.printf("Description: %s%n", updatedDetectionConfig.getDescription());
* System.out.printf("MetricId: %s%n", updatedDetectionConfig.getMetricId());
* });
*
*
*
* @param detectionConfiguration The anomaly detection configuration.
* @return A {@link Mono} containing the updated {@link AnomalyDetectionConfiguration}.
* @throws NullPointerException thrown if the {@code detectionConfiguration} is null
* or {@code detectionConfiguration.id} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono
updateDetectionConfig(AnomalyDetectionConfiguration detectionConfiguration) {
return updateDetectionConfigWithResponse(detectionConfiguration).map(Response::getValue);
}
/**
* Update a configuration to detect anomalies in the time series of a metric.
*
* Code sample
*
*
* final String detectionConfigId = "7b8069a1-1564-46da-9f50-b5d0dd9129ab";
* metricsAdvisorAdminAsyncClient
* .getDetectionConfigWithResponse(detectionConfigId)
* .flatMap(response -> {
* AnomalyDetectionConfiguration detectionConfig = response.getValue();
* detectionConfig.setName("updated config name");
* detectionConfig.setDescription("updated with more detection conditions");
* DimensionKey seriesGroupKey = new DimensionKey()
* .put("city", "Seoul");
* detectionConfig.addSeriesGroupDetectionCondition(
* new MetricSeriesGroupDetectionCondition(seriesGroupKey)
* .setSmartDetectionCondition(new SmartDetectionCondition(
* 10.0,
* AnomalyDetectorDirection.UP,
* new SuppressCondition(2, 2))));
* return metricsAdvisorAdminAsyncClient
* .updateDetectionConfigWithResponse(detectionConfig);
* })
* .subscribe(response -> {
* AnomalyDetectionConfiguration updatedDetectionConfig = response.getValue();
* System.out.printf("Detection config Id: %s%n", updatedDetectionConfig.getId());
* System.out.printf("Name: %s%n", updatedDetectionConfig.getName());
* System.out.printf("Description: %s%n", updatedDetectionConfig.getDescription());
* System.out.printf("MetricId: %s%n", updatedDetectionConfig.getMetricId());
* });
*
*
*
* @param detectionConfiguration The anomaly detection configuration.
* @return A {@link Response} of a {@link Mono} containing the updated {@link AnomalyDetectionConfiguration}.
* @throws NullPointerException thrown if the {@code detectionConfiguration} is null
* or {@code detectionConfiguration.id} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>
updateDetectionConfigWithResponse(AnomalyDetectionConfiguration detectionConfiguration) {
try {
return withContext(context -> updateDetectionConfigWithResponse(detectionConfiguration, context));
} catch (RuntimeException e) {
return FluxUtil.monoError(logger, e);
}
}
Mono>
updateDetectionConfigWithResponse(AnomalyDetectionConfiguration detectionConfiguration, Context context) {
Objects.requireNonNull(detectionConfiguration, "detectionConfiguration is required");
Objects.requireNonNull(detectionConfiguration.getId(), "detectionConfiguration.id is required");
final AnomalyDetectionConfigurationPatch innerDetectionConfigurationPatch
= DetectionConfigurationTransforms.toInnerForUpdate(logger, detectionConfiguration);
return service
.updateAnomalyDetectionConfigurationWithResponseAsync(UUID.fromString(detectionConfiguration.getId()),
innerDetectionConfigurationPatch, context)
.doOnSubscribe(ignoredValue -> logger.info("Updating AnomalyDetectionConfiguration"))
.doOnSuccess(response -> logger.info("Updated AnomalyDetectionConfiguration"))
.doOnError(error -> logger.warning("Failed to update AnomalyDetectionConfiguration", error))
.flatMap(response -> {
return getDetectionConfigWithResponse(detectionConfiguration.getId(), context)
.map(configurationResponse -> new ResponseBase(
response.getRequest(), response.getStatusCode(), response.getHeaders(),
configurationResponse.getValue(), null));
});
}
/**
* Delete a metric anomaly detection configuration.
*
* @param detectionConfigurationId The metric anomaly detection configuration unique id.
*
* Code sample
*
*
* final String detectionConfigId = "7b8069a1-1564-46da-9f50-b5d0dd9129ab";
* metricsAdvisorAdminAsyncClient
* .deleteDetectionConfig(detectionConfigId)
* .subscribe();
*
*
*
* @return An empty Mono.
* @throws NullPointerException thrown if the {@code detectionConfigurationId} is null.
* @throws IllegalArgumentException If {@code detectionConfigurationId} does not conform to the UUID
* format specification.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono deleteDetectionConfig(String detectionConfigurationId) {
return deleteDetectionConfigWithResponse(detectionConfigurationId).then();
}
/**
* Delete a metric anomaly detection configuration.
*
* Code sample
*
*
* final String detectionConfigId = "7b8069a1-1564-46da-9f50-b5d0dd9129ab";
* metricsAdvisorAdminAsyncClient
* .deleteDetectionConfigWithResponse(detectionConfigId)
* .subscribe(response ->
* System.out.printf("Response statusCode: %d%n", response.getStatusCode()));
*
*
*
* @param detectionConfigurationId The metric anomaly detection configuration unique id.
*
* @return A {@link Response} of a {@link Mono}.
* @throws NullPointerException thrown if the {@code detectionConfigurationId} is null.
* @throws IllegalArgumentException If {@code detectionConfigurationId} does not conform to the UUID
* format specification.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> deleteDetectionConfigWithResponse(String detectionConfigurationId) {
try {
return withContext(context -> deleteDetectionConfigWithResponse(detectionConfigurationId, context));
} catch (RuntimeException e) {
return FluxUtil.monoError(logger, e);
}
}
Mono> deleteDetectionConfigWithResponse(String detectionConfigurationId, Context context) {
Objects.requireNonNull(detectionConfigurationId, "detectionConfigurationId is required.");
return service.deleteHookWithResponseAsync(UUID.fromString(detectionConfigurationId), context)
.doOnRequest(ignoredValue -> logger.info("Deleting MetricAnomalyDetectionConfiguration"))
.doOnSuccess(response -> logger.info("Deleted MetricAnomalyDetectionConfiguration"))
.doOnError(error -> logger.warning("Failed to delete MetricAnomalyDetectionConfiguration", error));
}
/**
* Given a metric id, retrieve all anomaly detection configurations applied to it.
*
* Code sample
*
*
* final String metricId = "0b836da8-10e6-46cd-8f4f-28262e113a62";
* metricsAdvisorAdminAsyncClient.listDetectionConfigs(metricId)
* .subscribe(detectionConfig -> {
* System.out.printf("Detection config Id: %s%n", detectionConfig.getId());
* System.out.printf("Name: %s%n", detectionConfig.getName());
* System.out.printf("Description: %s%n", detectionConfig.getDescription());
* System.out.printf("MetricId: %s%n", detectionConfig.getMetricId());
* });
*
*
*
* @param metricId The metric id.
* @return The anomaly detection configurations.
* @throws NullPointerException thrown if the {@code metricId} is null.
* @throws IllegalArgumentException If {@code metricId} does not conform to the UUID format specification.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listDetectionConfigs(String metricId) {
return listDetectionConfigs(metricId, null);
}
/**
* Given a metric id, retrieve all anomaly detection configurations applied to it.
*
* Code sample
*
*
* final String metricId = "0b836da8-10e6-46cd-8f4f-28262e113a62";
* metricsAdvisorAdminAsyncClient.listDetectionConfigs(metricId,
* new ListDetectionConfigsOptions())
* .subscribe(detectionConfig -> {
* System.out.printf("Detection config Id: %s%n", detectionConfig.getId());
* System.out.printf("Name: %s%n", detectionConfig.getName());
* System.out.printf("Description: %s%n", detectionConfig.getDescription());
* System.out.printf("MetricId: %s%n", detectionConfig.getMetricId());
* });
*
*
*
* @param metricId The metric id.
* @param listDetectionConfigsOptions the additional configurable options to specify when querying the result.
* @return The anomaly detection configurations.
* @throws NullPointerException thrown if the {@code metricId} is null.
* @throws IllegalArgumentException If {@code metricId} does not conform to the UUID format specification.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listDetectionConfigs(String metricId,
ListDetectionConfigsOptions listDetectionConfigsOptions) {
try {
return new PagedFlux<>(() -> withContext(
context -> listAnomalyDetectionConfigsSinglePageAsync(metricId, listDetectionConfigsOptions, context)),
continuationToken -> withContext(
context -> listAnomalyDetectionConfigsNextPageAsync(continuationToken, context)));
} catch (RuntimeException ex) {
return new PagedFlux<>(() -> FluxUtil.monoError(logger, ex));
}
}
private Mono> listAnomalyDetectionConfigsSinglePageAsync(
String metricId, ListDetectionConfigsOptions options, Context context) {
if (options == null) {
options = new ListDetectionConfigsOptions();
}
return service
.getAnomalyDetectionConfigurationsByMetricSinglePageAsync(UUID.fromString(metricId), options.getSkip(),
options.getMaxPageSize(), context)
.doOnRequest(ignoredValue -> logger.info("Listing MetricAnomalyDetectionConfigs"))
.doOnSuccess(response -> logger.info("Listed MetricAnomalyDetectionConfigs {}", response))
.doOnError(error -> logger.warning("Failed to list the MetricAnomalyDetectionConfigs", error))
.map(DetectionConfigurationTransforms::fromInnerPagedResponse);
}
private Mono>
listAnomalyDetectionConfigsNextPageAsync(String nextPageLink, Context context) {
if (CoreUtils.isNullOrEmpty(nextPageLink)) {
return Mono.empty();
}
return service.getAnomalyDetectionConfigurationsByMetricNextSinglePageAsync(nextPageLink, context)
.doOnSubscribe(ignoredValue -> logger.info("Retrieving the next listing page - Page {}", nextPageLink))
.doOnSuccess(
response -> logger.info("Retrieved the next listing page - Page {} {}", nextPageLink, response))
.doOnError(
error -> logger.warning("Failed to retrieve the next listing page - Page {}", nextPageLink, error))
.map(DetectionConfigurationTransforms::fromInnerPagedResponse);
}
/**
* Creates a notificationHook that receives anomaly incident alerts.
*
* Code sample
*
*
* NotificationHook emailNotificationHook = new EmailNotificationHook("email hook")
* .setDescription("my email hook")
* .setEmailsToAlert(new ArrayList<String>() {{
* add("alertme@alertme.com");
* }})
* .setExternalLink("https://adwiki.azurewebsites.net/articles/howto/alerts/create-hooks.html");
*
* metricsAdvisorAdminAsyncClient.createHook(emailNotificationHook)
* .subscribe(hook -> {
* EmailNotificationHook createdEmailHook = (EmailNotificationHook) hook;
* System.out.printf("NotificationHook Id: %s%n", createdEmailHook.getId());
* System.out.printf("NotificationHook Name: %s%n", createdEmailHook.getName());
* System.out.printf("NotificationHook Description: %s%n", createdEmailHook.getDescription());
* System.out.printf("NotificationHook External Link: %s%n", createdEmailHook.getExternalLink());
* System.out.printf("NotificationHook Emails: %s%n", String.join(",",
* createdEmailHook.getEmailsToAlert()));
* });
*
*
*
* @param notificationHook The notificationHook.
*
* @return A {@link Mono} containing the created {@link NotificationHook}.
* @throws NullPointerException If {@code notificationHook}, {@code notificationHook.name},
* {@code notificationHook.endpoint} (for web notificationHook) is null.
* @throws IllegalArgumentException If at least one email not present for email notificationHook.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono createHook(NotificationHook notificationHook) {
return createHookWithResponse(notificationHook).map(Response::getValue);
}
/**
* Creates a notificationHook that receives anomaly incident alerts.
*
* Code sample
*
*
* NotificationHook emailNotificationHook = new EmailNotificationHook("email hook")
* .setDescription("my email hook")
* .setEmailsToAlert(new ArrayList<String>() {{
* add("alertme@alertme.com");
* }})
* .setExternalLink("https://adwiki.azurewebsites.net/articles/howto/alerts/create-hooks.html");
*
* metricsAdvisorAdminAsyncClient.createHookWithResponse(emailNotificationHook)
* .subscribe(response -> {
* System.out.printf("Response statusCode: %d%n", response.getStatusCode());
* EmailNotificationHook createdEmailHook = (EmailNotificationHook) response.getValue();
* System.out.printf("NotificationHook Id: %s%n", createdEmailHook.getId());
* System.out.printf("NotificationHook Name: %s%n", createdEmailHook.getName());
* System.out.printf("NotificationHook Description: %s%n", createdEmailHook.getDescription());
* System.out.printf("NotificationHook External Link: %s%n", createdEmailHook.getExternalLink());
* System.out.printf("NotificationHook Emails: %s%n", String.join(",",
* createdEmailHook.getEmailsToAlert()));
* });
*
*
*
* @param notificationHook The notificationHook.
*
* @return A {@link Response} of a {@link Mono} containing the created {@link NotificationHook}.
* @throws NullPointerException If {@code notificationHook}, {@code notificationHook.name},
* {@code notificationHook.endpoint} (for web notificationHook) is null.
* @throws IllegalArgumentException If at least one email not present for email notificationHook.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> createHookWithResponse(NotificationHook notificationHook) {
try {
return withContext(context -> createHookWithResponse(notificationHook, context));
} catch (RuntimeException e) {
return FluxUtil.monoError(logger, e);
}
}
Mono> createHookWithResponse(NotificationHook notificationHook, Context context) {
Objects.requireNonNull(notificationHook, "'notificationHook' cannot be null.");
return service.createHookWithResponseAsync(HookTransforms.toInnerForCreate(logger, notificationHook), context)
.doOnRequest(ignoredValue -> logger.info("Creating NotificationHook"))
.doOnSuccess(response -> logger.info("Created NotificationHook {}", response))
.doOnError(error -> logger.warning("Failed to create notificationHook", error))
.flatMap(response -> {
final String hookUri = response.getDeserializedHeaders().getLocation();
final String hookId = parseOperationId(hookUri);
return getHookWithResponse(hookId, context)
.map(hookResponse -> new ResponseBase(response.getRequest(),
response.getStatusCode(), response.getHeaders(), hookResponse.getValue(), null));
});
}
/**
* Get a hook by its id.
*
* Code sample
*
*
* final String hookId = "f00853f1-6627-447f-bacf-8dccf2e86fed";
* metricsAdvisorAdminAsyncClient.getHook(hookId)
* .subscribe(hook -> {
* if (hook instanceof EmailNotificationHook) {
* EmailNotificationHook emailHook = (EmailNotificationHook) hook;
* System.out.printf("Email Hook Id: %s%n", emailHook.getId());
* System.out.printf("Email Hook Name: %s%n", emailHook.getName());
* System.out.printf("Email Hook Description: %s%n", emailHook.getDescription());
* System.out.printf("Email Hook External Link: %s%n", emailHook.getExternalLink());
* System.out.printf("Email Hook Emails: %s%n", String.join(",",
* emailHook.getEmailsToAlert()));
* } else if (hook instanceof WebNotificationHook) {
* WebNotificationHook webHook = (WebNotificationHook) hook;
* System.out.printf("Web Hook Id: %s%n", webHook.getId());
* System.out.printf("Web Hook Name: %s%n", webHook.getName());
* System.out.printf("Web Hook Description: %s%n", webHook.getDescription());
* System.out.printf("Web Hook External Link: %s%n", webHook.getExternalLink());
* System.out.printf("Web Hook Endpoint: %s%n", webHook.getEndpoint());
* System.out.printf("Web Hook Headers: %s%n", webHook.getHttpHeaders());
* }
* });
*
*
*
* @param hookId The hook unique id.
*
* @return A {@link Mono} containing the {@link NotificationHook} for the provided id.
* @throws IllegalArgumentException If {@code hookId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code hookId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getHook(String hookId) {
return getHookWithResponse(hookId).map(Response::getValue);
}
/**
* Get a hook by its id.
*
* Code sample
*
*
* final String hookId = "f00853f1-6627-447f-bacf-8dccf2e86fed";
* metricsAdvisorAdminAsyncClient.getHookWithResponse(hookId)
* .subscribe(response -> {
* System.out.printf("Response status code: %d%n", response.getStatusCode());
* NotificationHook notificationHook = response.getValue();
* if (notificationHook instanceof EmailNotificationHook) {
* EmailNotificationHook emailHook = (EmailNotificationHook) notificationHook;
* System.out.printf("Email Hook Id: %s%n", emailHook.getId());
* System.out.printf("Email Hook Name: %s%n", emailHook.getName());
* System.out.printf("Email Hook Description: %s%n", emailHook.getDescription());
* System.out.printf("Email Hook External Link: %s%n", emailHook.getExternalLink());
* System.out.printf("Email Hook Emails: %s%n", String.join(",", emailHook.getEmailsToAlert()));
* } else if (notificationHook instanceof WebNotificationHook) {
* WebNotificationHook webHook = (WebNotificationHook) notificationHook;
* System.out.printf("Web Hook Id: %s%n", webHook.getId());
* System.out.printf("Web Hook Name: %s%n", webHook.getName());
* System.out.printf("Web Hook Description: %s%n", webHook.getDescription());
* System.out.printf("Web Hook External Link: %s%n", webHook.getExternalLink());
* System.out.printf("Web Hook Endpoint: %s%n", webHook.getEndpoint());
* System.out.printf("Web Hook Headers: %s%n", webHook.getHttpHeaders());
* }
* });
*
*
*
* @param hookId The hook unique id.
*
* @return A {@link Response} of a {@link Mono} containing the {@link NotificationHook} for the provided id.
* @throws IllegalArgumentException If {@code hookId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code hookId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getHookWithResponse(String hookId) {
try {
return withContext(context -> getHookWithResponse(hookId, context));
} catch (RuntimeException e) {
return FluxUtil.monoError(logger, e);
}
}
Mono> getHookWithResponse(String hookId, Context context) {
Objects.requireNonNull(hookId, "hookId is required.");
return service.getHookWithResponseAsync(UUID.fromString(hookId), context)
.doOnRequest(ignoredValue -> logger.info("Retrieving NotificationHook"))
.doOnSuccess(response -> logger.info("Retrieved NotificationHook {}", response))
.doOnError(error -> logger.warning("Failed to retrieve hook", error))
.map(innerResponse -> new ResponseBase(innerResponse.getRequest(),
innerResponse.getStatusCode(), innerResponse.getHeaders(),
HookTransforms.fromInner(logger, innerResponse.getValue()), null));
}
/**
* Update an existing notificationHook.
*
* Code sample
*
*
* final String emailHookId = "f00853f1-6627-447f-bacf-8dccf2e86fed";
* metricsAdvisorAdminAsyncClient.getHook(emailHookId)
* .flatMap(hook -> {
* EmailNotificationHook emailHook = (EmailNotificationHook) hook;
* List<String> emailsToUpdate = new ArrayList<>(emailHook.getEmailsToAlert());
* emailsToUpdate.remove("alertme@alertme.com");
* emailsToUpdate.add("alertme2@alertme.com");
* emailsToUpdate.add("alertme3@alertme.com");
* emailHook.setEmailsToAlert(emailsToUpdate);
* return metricsAdvisorAdminAsyncClient.updateHook(emailHook);
* })
* .subscribe(hook -> {
* EmailNotificationHook emailHook = (EmailNotificationHook) hook;
* System.out.printf("Email Hook Id: %s%n", emailHook.getId());
* System.out.printf("Email Hook Name: %s%n", emailHook.getName());
* System.out.printf("Email Hook Description: %s%n", emailHook.getDescription());
* System.out.printf("Email Hook External Link: %s%n", emailHook.getExternalLink());
* System.out.printf("Email Hook Emails: %s%n", String.join(",", emailHook.getEmailsToAlert()));
* });
*
*
*
* @param notificationHook The notificationHook to update.
*
* @return A {@link Mono} containing the updated {@link NotificationHook}.
* @throws NullPointerException If {@code notificationHook.id} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono updateHook(NotificationHook notificationHook) {
return updateHookWithResponse(notificationHook).map(Response::getValue);
}
/**
* Update an existing notification hook.
*
* Code sample
*
*
* final String emailHookId = "f00853f1-6627-447f-bacf-8dccf2e86fed";
* metricsAdvisorAdminAsyncClient.getHookWithResponse(emailHookId)
* .flatMap(response -> {
* EmailNotificationHook emailHook = (EmailNotificationHook) response.getValue();
* List<String> emailsToUpdate = new ArrayList<>(emailHook.getEmailsToAlert());
* emailsToUpdate.remove("alertme@alertme.com");
* emailsToUpdate.add("alertme2@alertme.com");
* emailsToUpdate.add("alertme3@alertme.com");
* emailHook.setEmailsToAlert(emailsToUpdate);
* return metricsAdvisorAdminAsyncClient.updateHookWithResponse(emailHook);
* })
* .subscribe(response -> {
* System.out.printf("Response statusCode: %d%n", response.getStatusCode());
* EmailNotificationHook emailHook = (EmailNotificationHook) response.getValue();
* System.out.printf("Email Hook Id: %s%n", emailHook.getId());
* System.out.printf("Email Hook Name: %s%n", emailHook.getName());
* System.out.printf("Email Hook Description: %s%n", emailHook.getDescription());
* System.out.printf("Email Hook External Link: %s%n", emailHook.getExternalLink());
* System.out.printf("Email Hook Emails: %s%n", String.join(",", emailHook.getEmailsToAlert()));
* });
*
*
*
* @param notificationHook The notificationHook to update.
*
* @return A {@link Response} of a {@link Mono} containing the updated {@link NotificationHook}.
* @throws NullPointerException If {@code notificationHook.id} is null.
* @throws IllegalArgumentException If {@code notificationHook.Id} does not conform to the UUID format
* specification.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> updateHookWithResponse(NotificationHook notificationHook) {
try {
return withContext(context -> updateHookWithResponse(notificationHook, context));
} catch (RuntimeException e) {
return FluxUtil.monoError(logger, e);
}
}
Mono> updateHookWithResponse(NotificationHook notificationHook, Context context) {
Objects.requireNonNull(notificationHook, "'notificationHook' cannot be null.");
Objects.requireNonNull(notificationHook.getId(), "'notificationHook.id' cannot be null.");
return service
.updateHookWithResponseAsync(UUID.fromString(notificationHook.getId()),
HookTransforms.toInnerForUpdate(logger, notificationHook), context)
.doOnRequest(ignoredValue -> logger.info("Updating NotificationHook"))
.doOnSuccess(response -> logger.info("Updated NotificationHook {}", response))
.doOnError(error -> logger.warning("Failed to update notificationHook", error))
.flatMap(response -> getHookWithResponse(notificationHook.getId(), context)
.map(hookResponse -> new ResponseBase(response.getRequest(),
response.getStatusCode(), response.getHeaders(), hookResponse.getValue(), null)));
}
/**
* Delete a hook.
*
* Code sample
*
*
* final String emailHookId = "f00853f1-6627-447f-bacf-8dccf2e86fed";
* metricsAdvisorAdminAsyncClient.deleteHook(emailHookId);
*
*
*
* @param hookId The hook unique id.
*
* @return An empty Mono.
* @throws NullPointerException thrown if the {@code hookId} is null.
* @throws IllegalArgumentException If {@code hookId} does not conform to the UUID format specification.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono deleteHook(String hookId) {
return deleteHookWithResponse(hookId).then();
}
/**
* Delete a hook.
*
* Code sample
*
*
* final String emailHookId = "f00853f1-6627-447f-bacf-8dccf2e86fed";
* metricsAdvisorAdminAsyncClient.deleteHookWithResponse(emailHookId)
* .subscribe(response -> {
* System.out.printf("Response statusCode: %d%n", response.getStatusCode());
* });
*
*
*
* @param hookId The hook unique id.
*
* @return A {@link Response} of a {@link Mono}.
* @throws NullPointerException thrown if the {@code hookId} is null.
* @throws IllegalArgumentException If {@code hookId} does not conform to the UUID format specification.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> deleteHookWithResponse(String hookId) {
try {
return withContext(context -> deleteHookWithResponse(hookId, context));
} catch (RuntimeException e) {
return FluxUtil.monoError(logger, e);
}
}
Mono> deleteHookWithResponse(String hookId, Context context) {
Objects.requireNonNull(hookId, "hookId is required.");
return service.deleteHookWithResponseAsync(UUID.fromString(hookId), context)
.doOnRequest(ignoredValue -> logger.info("Deleting NotificationHook"))
.doOnSuccess(response -> logger.info("Deleted NotificationHook"))
.doOnError(error -> logger.warning("Failed to delete hook", error));
}
/**
* List information of hooks on the metrics advisor account.
*
* Code sample
*
*
* metricsAdvisorAdminAsyncClient.listHooks()
* .subscribe(hook -> {
* if (hook instanceof EmailNotificationHook) {
* EmailNotificationHook emailHook = (EmailNotificationHook) hook;
* System.out.printf("Email Hook Id: %s%n", emailHook.getId());
* System.out.printf("Email Hook Name: %s%n", emailHook.getName());
* System.out.printf("Email Hook Description: %s%n", emailHook.getDescription());
* System.out.printf("Email Hook External Link: %s%n", emailHook.getExternalLink());
* System.out.printf("Email Hook Emails: %s%n", String.join(",", emailHook.getEmailsToAlert()));
* } else if (hook instanceof WebNotificationHook) {
* WebNotificationHook webHook = (WebNotificationHook) hook;
* System.out.printf("Web Hook Id: %s%n", webHook.getId());
* System.out.printf("Web Hook Name: %s%n", webHook.getName());
* System.out.printf("Web Hook Description: %s%n", webHook.getDescription());
* System.out.printf("Web Hook External Link: %s%n", webHook.getExternalLink());
* System.out.printf("Web Hook Endpoint: %s%n", webHook.getEndpoint());
* System.out.printf("Web Hook Headers: %s%n", webHook.getHttpHeaders());
* }
* });
*
*
*
* @return A {@link PagedFlux} containing information of all the {@link NotificationHook} in the account.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listHooks() {
return listHooks(new ListHookOptions());
}
/**
* List information of hooks.
*
* Code sample
*
*
* ListHookOptions options = new ListHookOptions()
* .setSkip(100)
* .setMaxPageSize(20);
* int[] pageCount = new int[1];
* metricsAdvisorAdminAsyncClient.listHooks(options).byPage()
* .subscribe(hookPage -> {
* System.out.printf("Page: %d%n", pageCount[0]++);
* for (NotificationHook notificationHook : hookPage.getElements()) {
* if (notificationHook instanceof EmailNotificationHook) {
* EmailNotificationHook emailHook = (EmailNotificationHook) notificationHook;
* System.out.printf("Email Hook Id: %s%n", emailHook.getId());
* System.out.printf("Email Hook Name: %s%n", emailHook.getName());
* System.out.printf("Email Hook Description: %s%n", emailHook.getDescription());
* System.out.printf("Email Hook External Link: %s%n", emailHook.getExternalLink());
* System.out.printf("Email Hook Emails: %s%n", String.join(",", emailHook.getEmailsToAlert()));
* System.out.printf("Email Hook Admins: %s%n", String.join(",", emailHook.getAdmins()));
* } else if (notificationHook instanceof WebNotificationHook) {
* WebNotificationHook webHook = (WebNotificationHook) notificationHook;
* System.out.printf("Web Hook Id: %s%n", webHook.getId());
* System.out.printf("Web Hook Name: %s%n", webHook.getName());
* System.out.printf("Web Hook Description: %s%n", webHook.getDescription());
* System.out.printf("Web Hook External Link: %s%n", webHook.getExternalLink());
* System.out.printf("Web Hook Endpoint: %s%n", webHook.getEndpoint());
* System.out.printf("Web Hook Headers: %s%n", webHook.getHttpHeaders());
* System.out.printf("Web Hook Admins: %s%n", String.join(",", webHook.getAdmins()));
* }
* }
* });
*
*
*
* @param listHookOptions the additional configurable options to specify when listing hooks.
*
* @return A {@link PagedFlux} containing information of the {@link NotificationHook} resources.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listHooks(ListHookOptions listHookOptions) {
try {
return new PagedFlux<>(() -> withContext(context -> listHooksSinglePageAsync(listHookOptions, context)),
continuationToken -> withContext(context -> listHooksNextPageAsync(continuationToken, context)));
} catch (RuntimeException ex) {
return new PagedFlux<>(() -> FluxUtil.monoError(logger, ex));
}
}
private Mono> listHooksSinglePageAsync(ListHookOptions options, Context context) {
return service
.listHooksSinglePageAsync(options != null ? options.getHookNameFilter() : null,
options != null ? options.getSkip() : null, options != null ? options.getMaxPageSize() : null, context)
.doOnRequest(ignoredValue -> logger.info("Listing hooks"))
.doOnSuccess(response -> logger.info("Listed hooks {}", response))
.doOnError(error -> logger.warning("Failed to list the hooks", error))
.map(response -> HookTransforms.fromInnerPagedResponse(logger, response));
}
private Mono> listHooksNextPageAsync(String nextPageLink, Context context) {
if (CoreUtils.isNullOrEmpty(nextPageLink)) {
return Mono.empty();
}
return service.listHooksNextSinglePageAsync(nextPageLink, context)
.doOnSubscribe(ignoredValue -> logger.info("Retrieving the next listing page - Page {}", nextPageLink))
.doOnSuccess(
response -> logger.info("Retrieved the next listing page - Page {} {}", nextPageLink, response))
.doOnError(
error -> logger.warning("Failed to retrieve the next listing page - Page {}", nextPageLink, error))
.map(response -> HookTransforms.fromInnerPagedResponse(logger, response));
}
/**
* Create a configuration to trigger alert when anomalies are detected.
*
* Code sample
*
*
* String detectionConfigurationId1 = "9ol48er30-6e6e-4391-b78f-b00dfee1e6f5";
* String detectionConfigurationId2 = "3e58er30-6e6e-4391-b78f-b00dfee1e6f5";
* String hookId1 = "5f48er30-6e6e-4391-b78f-b00dfee1e6f5";
* String hookId2 = "8i48er30-6e6e-4391-b78f-b00dfee1e6f5";
*
* metricsAdvisorAdminAsyncClient.createAlertConfig(
* new AnomalyAlertConfiguration("My AnomalyAlert config name")
* .setDescription("alert config description")
* .setMetricAlertConfigurations(Arrays.asList(
* new MetricAlertConfiguration(detectionConfigurationId1,
* MetricAnomalyAlertScope.forWholeSeries()),
* new MetricAlertConfiguration(detectionConfigurationId2,
* MetricAnomalyAlertScope.forWholeSeries())
* .setAlertConditions(new MetricAnomalyAlertConditions()
* .setSeverityRangeCondition(new SeverityCondition(AnomalySeverity.HIGH, AnomalySeverity.HIGH)))))
* .setCrossMetricsOperator(MetricAlertConfigurationsOperator.AND)
* .setHookIdsToAlert(Arrays.asList(hookId1, hookId2)))
* .subscribe(anomalyAlertConfiguration -> {
* System.out.printf("DataPoint Anomaly alert configuration Id: %s%n", anomalyAlertConfiguration.getId());
* System.out.printf("DataPoint Anomaly alert configuration description: %s%n",
* anomalyAlertConfiguration.getDescription());
* System.out.printf("DataPoint Anomaly alert configuration hook ids: %s%n",
* anomalyAlertConfiguration.getHookIdsToAlert());
* System.out.printf("DataPoint Anomaly alert configuration cross metrics operator: %s%n",
* anomalyAlertConfiguration.getCrossMetricsOperator().toString());
* });
*
*
*
* @param alertConfiguration The anomaly alerting configuration.
*
* @return A {@link Mono} containing the created {@link AnomalyAlertConfiguration}.
* @throws NullPointerException thrown if the {@code alertConfiguration} or
* {@code alertConfiguration.metricAnomalyAlertConfigurations} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono createAlertConfig(AnomalyAlertConfiguration alertConfiguration) {
return createAlertConfigWithResponse(alertConfiguration).flatMap(FluxUtil::toMono);
}
/**
*
* Code sample
*
*
*
* String detectionConfigurationId1 = "9ol48er30-6e6e-4391-b78f-b00dfee1e6f5";
* String detectionConfigurationId2 = "3e58er30-6e6e-4391-b78f-b00dfee1e6f5";
* String hookId1 = "5f48er30-6e6e-4391-b78f-b00dfee1e6f5";
* String hookId2 = "8i48er30-6e6e-4391-b78f-b00dfee1e6f5";
*
* metricsAdvisorAdminAsyncClient.createAlertConfigWithResponse(
* new AnomalyAlertConfiguration("My AnomalyAlert config name")
* .setDescription("alert config description")
* .setMetricAlertConfigurations(Arrays.asList(
* new MetricAlertConfiguration(detectionConfigurationId1,
* MetricAnomalyAlertScope.forWholeSeries()),
* new MetricAlertConfiguration(detectionConfigurationId2,
* MetricAnomalyAlertScope.forWholeSeries())
* .setAlertConditions(new MetricAnomalyAlertConditions()
* .setSeverityRangeCondition(new SeverityCondition(AnomalySeverity.HIGH, AnomalySeverity.HIGH)))))
* .setCrossMetricsOperator(MetricAlertConfigurationsOperator.AND)
* .setHookIdsToAlert(Arrays.asList(hookId1, hookId2)))
* .subscribe(alertConfigurationResponse -> {
* System.out.printf("DataPoint Anomaly alert creation operation status: %s%n",
* alertConfigurationResponse.getStatusCode());
* final AnomalyAlertConfiguration anomalyAlertConfiguration = alertConfigurationResponse.getValue();
* System.out.printf("DataPoint Anomaly alert configuration Id: %s%n", anomalyAlertConfiguration.getId());
* System.out.printf("DataPoint Anomaly alert configuration description: %s%n",
* anomalyAlertConfiguration.getDescription());
* System.out.printf("DataPoint Anomaly alert configuration hook ids: %s%n",
* anomalyAlertConfiguration.getHookIdsToAlert());
* System.out.printf("DataPoint Anomaly alert configuration cross metrics operator: %s%n",
* anomalyAlertConfiguration.getCrossMetricsOperator().toString());
* });
*
*
*
* @param alertConfiguration The anomaly alerting configuration.
*
* @return A {@link Response} of a {@link Mono} containing the created {@link AnomalyAlertConfiguration}.
* @throws NullPointerException thrown if the {@code alertConfiguration} or
* {@code alertConfiguration.metricAnomalyAlertConfigurations} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>
createAlertConfigWithResponse(AnomalyAlertConfiguration alertConfiguration) {
try {
return withContext(context -> createAlertConfigWithResponse(alertConfiguration, context));
} catch (RuntimeException ex) {
return monoError(logger, ex);
}
}
Mono>
createAlertConfigWithResponse(AnomalyAlertConfiguration alertConfiguration, Context context) {
Objects.requireNonNull(alertConfiguration, "'alertConfiguration' is required.");
if (CoreUtils.isNullOrEmpty(alertConfiguration.getMetricAlertConfigurations())) {
throw logger.logExceptionAsError(
new NullPointerException("'alertConfiguration.metricAnomalyAlertConfigurations' is required"));
}
if (alertConfiguration.getCrossMetricsOperator() == null
&& alertConfiguration.getMetricAlertConfigurations().size() > 1) {
throw logger.logExceptionAsError(new IllegalArgumentException("crossMetricsOperator is required"
+ " when there are more than one metric level alert configuration."));
}
final AnomalyAlertingConfiguration innerAlertConfiguration
= AlertConfigurationTransforms.toInnerForCreate(alertConfiguration);
return service.createAnomalyAlertingConfigurationWithResponseAsync(innerAlertConfiguration, context)
.doOnSubscribe(
ignoredValue -> logger.info("Creating AnomalyAlertConfiguration - {}", innerAlertConfiguration))
.doOnSuccess(response -> logger.info("Created AnomalyAlertConfiguration - {}", response))
.doOnError(error -> logger.warning("Failed to create AnomalyAlertConfiguration - {}",
innerAlertConfiguration, error))
.flatMap(response -> {
final String configurationId = parseOperationId(response.getDeserializedHeaders().getLocation());
return getAlertConfigWithResponse(configurationId, context)
.map(getResponse -> new ResponseBase(response.getRequest(),
response.getStatusCode(), response.getHeaders(), getResponse.getValue(), null));
});
}
/**
* Get the anomaly alert configuration identified by {@code alertConfigurationId}.
*
* Code sample
*
*
* String alertConfigId = "1p0f8er30-6e6e-4391-b78f-bpfdfee1e6f5";
*
* metricsAdvisorAdminAsyncClient.getAlertConfig(alertConfigId)
* .subscribe(anomalyAlertConfiguration -> {
* System.out.printf("DataPoint Anomaly alert configuration Id: %s%n", anomalyAlertConfiguration.getId());
* System.out.printf("DataPoint Anomaly alert configuration description: %s%n",
* anomalyAlertConfiguration.getDescription());
* System.out.printf("DataPoint Anomaly alert configuration hook ids: %s%n",
* anomalyAlertConfiguration.getHookIdsToAlert());
* System.out.printf("DataPoint Anomaly alert configuration cross metrics operator: %s%n",
* anomalyAlertConfiguration.getCrossMetricsOperator().toString());
* });
*
*
*
* @param alertConfigurationId The anomaly alert configuration id.
*
* @return A {@link Mono} containing the {@link AnomalyAlertConfiguration} identified by the given id.
* @throws IllegalArgumentException If {@code alertConfigurationId} does not conform to the
* UUID format specification.
* @throws NullPointerException thrown if the {@code alertConfigurationId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getAlertConfig(String alertConfigurationId) {
return getAlertConfigWithResponse(alertConfigurationId).flatMap(FluxUtil::toMono);
}
/**
* Get the anomaly alert configuration identified by {@code alertConfigurationId}.
*
* Code sample
*
*
* String alertConfigId = "1p0f8er30-6e6e-4391-b78f-bpfdfee1e6f5";
*
* metricsAdvisorAdminAsyncClient.getAlertConfigWithResponse(alertConfigId)
* .subscribe(alertConfigurationResponse -> {
* System.out.printf("DataPointAnomaly alert creation operation status: %s%n",
* alertConfigurationResponse.getStatusCode());
* final AnomalyAlertConfiguration anomalyAlertConfiguration = alertConfigurationResponse.getValue();
* System.out.printf("DataPoint Anomaly alert configuration Id: %s%n", anomalyAlertConfiguration.getId());
* System.out.printf("DataPoint Anomaly alert configuration description: %s%n",
* anomalyAlertConfiguration.getDescription());
* System.out.printf("DataPoint Anomaly alert configuration hook ids: %s%n",
* anomalyAlertConfiguration.getHookIdsToAlert());
* System.out.printf("DataPoint Anomaly alert configuration cross metrics operator: %s%n",
* anomalyAlertConfiguration.getCrossMetricsOperator().toString());
* });
*
*
*
* @param alertConfigurationId The anomaly alert configuration id.
*
* @return A {@link Response response} of a {@link Mono}
* containing the {@link AnomalyAlertConfiguration} identified by the given id.
* @throws IllegalArgumentException If {@code alertConfigurationId} does not conform to the
* UUID format specification.
* @throws NullPointerException thrown if the {@code alertConfigurationId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getAlertConfigWithResponse(String alertConfigurationId) {
try {
return withContext(context -> getAlertConfigWithResponse(alertConfigurationId, context));
} catch (RuntimeException ex) {
return monoError(logger, ex);
}
}
Mono> getAlertConfigWithResponse(String alertConfigurationId, Context context) {
Objects.requireNonNull(alertConfigurationId, "'alertConfigurationId' is required.");
return service.getAnomalyAlertingConfigurationWithResponseAsync(UUID.fromString(alertConfigurationId), context)
.doOnSubscribe(
ignoredValue -> logger.info("Retrieving AnomalyDetectionConfiguration - {}", alertConfigurationId))
.doOnSuccess(response -> logger.info("Retrieved AnomalyDetectionConfiguration - {}", response))
.doOnError(error -> logger.warning("Failed to retrieve AnomalyDetectionConfiguration - {}",
alertConfigurationId, error))
.map(response -> new ResponseBase(response.getRequest(),
response.getStatusCode(), response.getHeaders(),
AlertConfigurationTransforms.fromInner(response.getValue()), null));
}
/**
* Update anomaly alert configuration.
*
* Code sample
*
*
*
* String alertConfigId = "1p0f8er30-6e6e-4391-b78f-bpfdfee1e6f5";
* String additionalHookId = "2gh8er30-6e6e-4391-b78f-bpfdfee1e6f5";
*
* metricsAdvisorAdminAsyncClient.getAlertConfig(alertConfigId)
* .flatMap(existingAnomalyConfig -> {
* List<String> hookIds = new ArrayList<>(existingAnomalyConfig.getHookIdsToAlert());
* hookIds.add(additionalHookId);
* return metricsAdvisorAdminAsyncClient.updateAlertConfig(
* existingAnomalyConfig
* .setHookIdsToAlert(hookIds)
* .setDescription("updated to add more hook ids"));
* }).subscribe(updateAnomalyAlertConfiguration -> {
* System.out.printf("Updated anomaly alert configuration Id: %s%n",
* updateAnomalyAlertConfiguration.getId());
* System.out.printf("Updated anomaly alert configuration description: %s%n",
* updateAnomalyAlertConfiguration.getDescription());
* System.out.printf("Updated anomaly alert configuration hook ids: %s%n",
* updateAnomalyAlertConfiguration.getHookIdsToAlert());
* });
*
*
*
* @param alertConfiguration The anomaly alert configuration to update.
*
* @return A {@link Mono} containing the {@link AnomalyAlertConfiguration} that was updated.
* @throws NullPointerException thrown if {@code alertConfiguration} or
* {@code alertConfiguration.metricAnomalyAlertConfigurations} is null or empty.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono updateAlertConfig(AnomalyAlertConfiguration alertConfiguration) {
return updateAlertConfigWithResponse(alertConfiguration).flatMap(FluxUtil::toMono);
}
/**
* Update anomaly alert configuration.
*
* Code sample
*
*
*
* String alertConfigId = "1p0f8er30-6e6e-4391-b78f-bpfdfee1e6f5";
* String additionalHookId = "2gh8er30-6e6e-4391-b78f-bpfdfee1e6f5";
*
* metricsAdvisorAdminAsyncClient.getAlertConfig(alertConfigId)
* .flatMap(existingAnomalyConfig -> {
* List<String> hookIds = new ArrayList<>(existingAnomalyConfig.getHookIdsToAlert());
* hookIds.add(additionalHookId);
* return metricsAdvisorAdminAsyncClient.updateAlertConfigWithResponse(
* existingAnomalyConfig
* .setHookIdsToAlert(hookIds)
* .setDescription("updated to add more hook ids"));
* }).subscribe(alertConfigurationResponse -> {
* System.out.printf("Update anomaly alert operation status: %s%n",
* alertConfigurationResponse.getStatusCode());
* final AnomalyAlertConfiguration updatedAnomalyAlertConfiguration
* = alertConfigurationResponse.getValue();
* System.out.printf("Updated anomaly alert configuration Id: %s%n",
* updatedAnomalyAlertConfiguration.getId());
* System.out.printf("Updated anomaly alert configuration description: %s%n",
* updatedAnomalyAlertConfiguration.getDescription());
* System.out.printf("Updated anomaly alert configuration hook ids: %s%n",
* updatedAnomalyAlertConfiguration.getHookIdsToAlert());
* });
*
*
*
* @param alertConfiguration The anomaly alert configuration to update.
*
* @return A {@link Response} of a {@link Mono} containing the {@link AnomalyAlertConfiguration} that was updated.
* @throws NullPointerException thrown if {@code alertConfiguration} or
* {@code alertConfiguration.metricAnomalyAlertConfigurations} is null or empty.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>
updateAlertConfigWithResponse(AnomalyAlertConfiguration alertConfiguration) {
try {
return withContext(context -> updateAlertConfigWithResponse(alertConfiguration, context));
} catch (RuntimeException ex) {
return monoError(logger, ex);
}
}
Mono>
updateAlertConfigWithResponse(AnomalyAlertConfiguration alertConfiguration, Context context) {
Objects.requireNonNull(alertConfiguration, "'alertConfiguration' is required");
if (CoreUtils.isNullOrEmpty(alertConfiguration.getMetricAlertConfigurations())) {
throw logger.logExceptionAsError(new NullPointerException(
"'alertConfiguration.metricAnomalyAlertConfigurations' is required and cannot be empty"));
}
final AnomalyAlertingConfigurationPatch innerAlertConfiguration
= AlertConfigurationTransforms.toInnerForUpdate(alertConfiguration);
return service
.updateAnomalyAlertingConfigurationWithResponseAsync(UUID.fromString(alertConfiguration.getId()),
innerAlertConfiguration, context)
.doOnSubscribe(
ignoredValue -> logger.info("Updating AnomalyAlertConfiguration - {}", innerAlertConfiguration))
.doOnSuccess(response -> logger.info("Updated AnomalyAlertConfiguration - {}", response))
.doOnError(error -> logger.warning("Failed to update AnomalyAlertConfiguration - {}",
innerAlertConfiguration, error))
.flatMap(response -> getAlertConfigWithResponse(alertConfiguration.getId(), context)
.map(getResponse -> new ResponseBase(response.getRequest(),
response.getStatusCode(), response.getHeaders(), getResponse.getValue(), null)));
}
/**
* Deletes the anomaly alert configuration identified by {@code alertConfigurationId}.
*
* Code sample
*
*
* String alertConfigId = "1p0f8er30-6e6e-4391-b78f-bpfdfee1e6f5";
* metricsAdvisorAdminAsyncClient.deleteAlertConfig(alertConfigId);
*
*
*
* @param alertConfigurationId The anomaly alert configuration id.
*
* @return An empty Mono.
* @throws IllegalArgumentException If {@code alertConfigurationId} does not conform to the
* UUID format specification.
* @throws NullPointerException thrown if the {@code alertConfigurationId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono deleteAlertConfig(String alertConfigurationId) {
return deleteAlertConfigWithResponse(alertConfigurationId).flatMap(FluxUtil::toMono);
}
/**
* Deletes the anomaly alert configuration identified by {@code alertConfigurationId}.
*
* Code sample
*
*
* String alertConfigId = "1p0f8er30-6e6e-4391-b78f-bpfdfee1e6f5";
*
* metricsAdvisorAdminAsyncClient.deleteAlertConfigWithResponse(alertConfigId)
* .subscribe(response -> {
* System.out.printf("DataPoint Anomaly alert config delete operation status : %s%n",
* response.getStatusCode());
* });
*
*
*
* @param alertConfigurationId The anomaly alert configuration id.
*
* @return A response containing status code and headers returned after the operation.
* @throws IllegalArgumentException If {@code alertConfigurationId} does not conform to the
* UUID format specification.
* @throws NullPointerException thrown if the {@code alertConfigurationId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> deleteAlertConfigWithResponse(String alertConfigurationId) {
try {
return withContext(context -> deleteAlertConfigWithResponse(alertConfigurationId, context));
} catch (RuntimeException ex) {
return monoError(logger, ex);
}
}
Mono> deleteAlertConfigWithResponse(String alertConfigurationId, Context context) {
Objects.requireNonNull(alertConfigurationId, "'alertConfigurationId' is required.");
return service
.deleteAnomalyAlertingConfigurationWithResponseAsync(UUID.fromString(alertConfigurationId), context)
.doOnSubscribe(ignoredValue -> logger.info("Deleting AnomalyAlertConfiguration - {}", alertConfigurationId))
.doOnSuccess(response -> logger.info("Deleted AnomalyAlertConfiguration - {}", response))
.doOnError(error -> logger.warning("Failed to delete AnomalyAlertConfiguration - {}", alertConfigurationId,
error));
}
/**
* Fetch the anomaly alert configurations associated with a detection configuration.
*
* Code sample
*
*
* String detectionConfigId = "3rt98er30-6e6e-4391-b78f-bpfdfee1e6f5";
* metricsAdvisorAdminAsyncClient.listAlertConfigs(detectionConfigId, new ListAnomalyAlertConfigsOptions())
* .subscribe(anomalyAlertConfiguration -> {
* System.out.printf("DataPoint Anomaly alert configuration Id: %s%n", anomalyAlertConfiguration.getId());
* System.out.printf("DataPoint Anomaly alert configuration description: %s%n",
* anomalyAlertConfiguration.getDescription());
* System.out.printf("DataPoint Anomaly alert configuration hook ids: %s%n",
* anomalyAlertConfiguration.getHookIdsToAlert());
* System.out.printf("DataPoint Anomaly alert configuration cross metrics operator: %s%n",
* anomalyAlertConfiguration.getCrossMetricsOperator().toString());
* });
*
*
*
* @param detectionConfigurationId The id of the detection configuration.
* @param listAnomalyAlertConfigsOptions th e additional configurable options to specify when querying the result.
*
* @return A {@link PagedFlux} containing information of all the
* {@link AnomalyAlertConfiguration anomaly alert configurations} for the specified detection configuration.
* @throws NullPointerException thrown if the {@code detectionConfigurationId} is null.
* @throws IllegalArgumentException If {@code detectionConfigurationId} does not conform to the
* UUID format specification.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listAlertConfigs(String detectionConfigurationId,
ListAnomalyAlertConfigsOptions listAnomalyAlertConfigsOptions) {
try {
return new PagedFlux<>(
() -> withContext(context -> listAnomalyAlertConfigsSinglePageAsync(detectionConfigurationId,
listAnomalyAlertConfigsOptions, context)),
continuationToken -> withContext(
context -> listAnomalyAlertConfigsNextPageAsync(continuationToken, context)));
} catch (RuntimeException ex) {
return new PagedFlux<>(() -> FluxUtil.monoError(logger, ex));
}
}
private Mono> listAnomalyAlertConfigsSinglePageAsync(
String detectionConfigurationId, ListAnomalyAlertConfigsOptions options, Context context) {
Objects.requireNonNull(detectionConfigurationId, "'detectionConfigurationId' is required.");
if (options == null) {
options = new ListAnomalyAlertConfigsOptions();
}
return service
.getAnomalyAlertingConfigurationsByAnomalyDetectionConfigurationSinglePageAsync(
UUID.fromString(detectionConfigurationId), options.getSkip(), options.getMaxPageSize(), context)
.doOnRequest(ignoredValue -> logger.info("Listing AnomalyAlertConfigs"))
.doOnSuccess(response -> logger.info("Listed AnomalyAlertConfigs {}", response))
.doOnError(error -> logger.warning("Failed to list the AnomalyAlertConfigs", error))
.map(AlertConfigurationTransforms::fromInnerPagedResponse);
}
private Mono> listAnomalyAlertConfigsNextPageAsync(String nextPageLink,
Context context) {
if (CoreUtils.isNullOrEmpty(nextPageLink)) {
return Mono.empty();
}
return service
.getAnomalyAlertingConfigurationsByAnomalyDetectionConfigurationNextSinglePageAsync(nextPageLink, context)
.doOnSubscribe(ignoredValue -> logger.info("Retrieving the next listing page - Page {}", nextPageLink))
.doOnSuccess(
response -> logger.info("Retrieved the next listing page - Page {} {}", nextPageLink, response))
.doOnError(
error -> logger.warning("Failed to retrieve the next listing page - Page {}", nextPageLink, error))
.map(AlertConfigurationTransforms::fromInnerPagedResponse);
}
/**
* Create a data source credential entity.
*
* Code sample
*
*
* DataSourceCredentialEntity datasourceCredential;
* final String name = "sample_name" + UUID.randomUUID();
* final String cId = "f45668b2-bffa-11eb-8529-0246ac130003";
* final String tId = "67890ded-5e07-4e52-b225-4ae8f905afb5";
* final String mockSecret = "890hy69-5e07-4e52-b225-4ae8f905afb5";
*
* datasourceCredential = new DataSourceServicePrincipalInKeyVault()
* .setName(name)
* .setKeyVaultForDataSourceSecrets("kv", cId, mockSecret)
* .setTenantId(tId)
* .setSecretNameForDataSourceClientId("DSClientID_1")
* .setSecretNameForDataSourceClientSecret("DSClientSer_1");
*
* metricsAdvisorAdminAsyncClient.createDataSourceCredential(datasourceCredential)
* .subscribe(credentialEntity -> {
* if (credentialEntity instanceof DataSourceServicePrincipalInKeyVault) {
* DataSourceServicePrincipalInKeyVault actualCredentialSPInKV
* = (DataSourceServicePrincipalInKeyVault) credentialEntity;
* System.out
* .printf("Actual credential entity key vault endpoint: %s%n",
* actualCredentialSPInKV.getKeyVaultEndpoint());
* System.out.printf("Actual credential entity key vault client Id: %s%n",
* actualCredentialSPInKV.getKeyVaultClientId());
* System.out.printf("Actual credential entity key vault secret name for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientId());
* System.out.printf("Actual credential entity key vault secret for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientSecret());
* }
* });
*
*
*
* @param dataSourceCredential The credential entity.
* @return A {@link Mono} containing the created {@link DataSourceCredentialEntity}.
* @throws NullPointerException thrown if the {@code credentialEntity} is null
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono
createDataSourceCredential(DataSourceCredentialEntity dataSourceCredential) {
return createDataSourceCredentialWithResponse(dataSourceCredential).map(Response::getValue);
}
/**
* Create a data source credential entity with REST response.
*
* Code sample
*
*
* DataSourceCredentialEntity datasourceCredential;
* final String name = "sample_name" + UUID.randomUUID();
* final String cId = "f45668b2-bffa-11eb-8529-0246ac130003";
* final String tId = "67890ded-5e07-4e52-b225-4ae8f905afb5";
* final String mockSecret = "890hy69-5e07-4e52-b225-4ae8f905afb5";
*
* datasourceCredential = new DataSourceServicePrincipalInKeyVault()
* .setName(name)
* .setKeyVaultForDataSourceSecrets("kv", cId, mockSecret)
* .setTenantId(tId)
* .setSecretNameForDataSourceClientId("DSClientID_1")
* .setSecretNameForDataSourceClientSecret("DSClientSer_1");
*
* metricsAdvisorAdminAsyncClient.createDataSourceCredentialWithResponse(datasourceCredential)
* .subscribe(credentialEntityWithResponse -> {
* System.out.printf("Credential Entity creation operation status: %s%n",
* credentialEntityWithResponse.getStatusCode());
* if (credentialEntityWithResponse.getValue() instanceof DataSourceServicePrincipalInKeyVault) {
* DataSourceServicePrincipalInKeyVault actualCredentialSPInKV
* = (DataSourceServicePrincipalInKeyVault) credentialEntityWithResponse.getValue();
* System.out
* .printf("Actual credential entity key vault endpoint: %s%n",
* actualCredentialSPInKV.getKeyVaultEndpoint());
* System.out.printf("Actual credential entity key vault client Id: %s%n",
* actualCredentialSPInKV.getKeyVaultClientId());
* System.out.printf("Actual credential entity key vault secret name for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientId());
* System.out.printf("Actual credential entity key vault secret for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientSecret());
* }
* });
*
*
*
* @param dataSourceCredential The credential entity.
* @return A {@link Mono} containing the created {@link DataSourceCredentialEntity}.
* @throws NullPointerException thrown if the {@code credentialEntity} is null
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>
createDataSourceCredentialWithResponse(DataSourceCredentialEntity dataSourceCredential) {
try {
return withContext(context -> createDataSourceCredentialWithResponse(dataSourceCredential, context));
} catch (RuntimeException e) {
return FluxUtil.monoError(logger, e);
}
}
Mono>
createDataSourceCredentialWithResponse(DataSourceCredentialEntity dataSourceCredential, Context context) {
Objects.requireNonNull(dataSourceCredential, "dataSourceCredential is required");
final DataSourceCredential innerDataSourceCredential
= DataSourceCredentialEntityTransforms.toInnerForCreate(dataSourceCredential);
return service.createCredentialWithResponseAsync(innerDataSourceCredential, context)
.doOnSubscribe(ignoredValue -> logger.info("Creating DataSourceCredentialEntity"))
.doOnSuccess(response -> logger.info("Created DataSourceCredentialEntity"))
.doOnError(error -> logger.warning("Failed to create DataSourceCredentialEntity", error))
.flatMap(response -> {
final String credentialId = Utility.parseOperationId(response.getDeserializedHeaders().getLocation());
return this.getDataSourceCredentialWithResponse(credentialId, context)
.map(configurationResponse -> new ResponseBase(
response.getRequest(), response.getStatusCode(), response.getHeaders(),
configurationResponse.getValue(), null));
});
}
/**
* Update a data source credential entity.
*
* Code sample
*
*
* String credentialId = "";
* metricsAdvisorAdminAsyncClient.getDataSourceCredential(credentialId)
* .flatMap(existingDatasourceCredential -> {
* DataSourceServicePrincipalInKeyVault actualCredentialSPInKV = null;
* if (existingDatasourceCredential instanceof DataSourceServicePrincipalInKeyVault) {
* actualCredentialSPInKV = (DataSourceServicePrincipalInKeyVault) existingDatasourceCredential;
* }
* return metricsAdvisorAdminAsyncClient.updateDataSourceCredential(
* actualCredentialSPInKV.setDescription("set updated description"));
* })
* .subscribe(credentialEntity -> {
* if (credentialEntity instanceof DataSourceServicePrincipalInKeyVault) {
* DataSourceServicePrincipalInKeyVault actualCredentialSPInKV
* = (DataSourceServicePrincipalInKeyVault) credentialEntity;
* System.out.printf("Actual credential entity key vault endpoint: %s%n",
* actualCredentialSPInKV.getKeyVaultEndpoint());
* System.out.printf("Actual credential entity key vault updated description: %s%n",
* actualCredentialSPInKV.getDescription());
* }
* });
*
*
*
* @param dataSourceCredential The credential entity.
* @return A {@link Mono} containing the updated {@link DataSourceCredentialEntity}.
* @throws NullPointerException thrown if the {@code credentialEntity} is null
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono
updateDataSourceCredential(DataSourceCredentialEntity dataSourceCredential) {
return updateDataSourceCredentialWithResponse(dataSourceCredential).map(Response::getValue);
}
/**
* Update a data source credential entity with REST response.
*
* Code sample
*
*
* String credentialId = "";
* metricsAdvisorAdminAsyncClient.getDataSourceCredential(credentialId)
* .flatMap(existingDatasourceCredential -> {
* DataSourceServicePrincipalInKeyVault actualCredentialSPInKV = null;
* if (existingDatasourceCredential instanceof DataSourceServicePrincipalInKeyVault) {
* actualCredentialSPInKV = (DataSourceServicePrincipalInKeyVault) existingDatasourceCredential;
* }
* return metricsAdvisorAdminAsyncClient.updateDataSourceCredentialWithResponse(
* actualCredentialSPInKV.setDescription("set updated description"));
* })
* .subscribe(credentialEntityWithResponse -> {
* System.out.printf("Credential Entity creation operation status: %s%n",
* credentialEntityWithResponse.getStatusCode());
* if (credentialEntityWithResponse.getValue() instanceof DataSourceServicePrincipalInKeyVault) {
* DataSourceServicePrincipalInKeyVault actualCredentialSPInKV
* = (DataSourceServicePrincipalInKeyVault) credentialEntityWithResponse.getValue();
* System.out.printf("Actual credential entity key vault endpoint: %s%n",
* actualCredentialSPInKV.getKeyVaultEndpoint());
* System.out.printf("Actual credential entity key vault updated description: %s%n",
* actualCredentialSPInKV.getDescription());
* }
* });
*
*
*
* @param dataSourceCredential The credential entity.
* @return A {@link Mono} containing the updated {@link DataSourceCredentialEntity}.
* @throws NullPointerException thrown if the {@code credentialEntity} is null
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>
updateDataSourceCredentialWithResponse(DataSourceCredentialEntity dataSourceCredential) {
try {
return withContext(context -> updateDataSourceCredentialWithResponse(dataSourceCredential, context));
} catch (RuntimeException e) {
return FluxUtil.monoError(logger, e);
}
}
Mono>
updateDataSourceCredentialWithResponse(DataSourceCredentialEntity dataSourceCredential, Context context) {
Objects.requireNonNull(dataSourceCredential, "dataSourceCredential is required");
final DataSourceCredentialPatch innerDataSourceCredential
= DataSourceCredentialEntityTransforms.toInnerForUpdate(dataSourceCredential);
return service
.updateCredentialWithResponseAsync(UUID.fromString(dataSourceCredential.getId()), innerDataSourceCredential,
context)
.doOnSubscribe(ignoredValue -> logger.info("Updating DataSourceCredentialEntity"))
.doOnSuccess(response -> logger.info("Updated DataSourceCredentialEntity"))
.doOnError(error -> logger.warning("Failed to update DataSourceCredentialEntity", error))
.flatMap(response -> {
return this.getDataSourceCredentialWithResponse(dataSourceCredential.getId(), context)
.map(configurationResponse -> new ResponseBase(
response.getRequest(), response.getStatusCode(), response.getHeaders(),
configurationResponse.getValue(), null));
});
}
/**
* Get a data source credential entity by its id.
*
* Code sample
*
*
* final String datasourceCredentialId = "f45668b2-bffa-11eb-8529-0246ac130003";
*
* metricsAdvisorAdminAsyncClient.getDataSourceCredential(datasourceCredentialId)
* .subscribe(credentialEntity -> {
* if (credentialEntity instanceof DataSourceServicePrincipalInKeyVault) {
* DataSourceServicePrincipalInKeyVault actualCredentialSPInKV
* = (DataSourceServicePrincipalInKeyVault) credentialEntity;
* System.out
* .printf("Actual credential entity key vault endpoint: %s%n",
* actualCredentialSPInKV.getKeyVaultEndpoint());
* System.out.printf("Actual credential entity key vault client Id: %s%n",
* actualCredentialSPInKV.getKeyVaultClientId());
* System.out.printf("Actual credential entity key vault secret name for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientId());
* System.out.printf("Actual credential entity key vault secret for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientSecret());
* }
* });
*
*
*
* @param credentialId The data source credential entity unique id.
*
* @return The data source credential entity for the provided id.
* @throws IllegalArgumentException If {@code credentialId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code credentialId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getDataSourceCredential(String credentialId) {
return getDataSourceCredentialWithResponse(credentialId).flatMap(FluxUtil::toMono);
}
/**
* Get a data source credential entity by its id with REST response.
*
* Code sample
*
*
* final String datasourceCredentialId = "f45668b2-bffa-11eb-8529-0246ac130003";
*
* metricsAdvisorAdminAsyncClient.getDataSourceCredentialWithResponse(datasourceCredentialId)
* .subscribe(credentialEntityWithResponse -> {
* System.out.printf("Credential Entity creation operation status: %s%n",
* credentialEntityWithResponse.getStatusCode());
* if (credentialEntityWithResponse.getValue() instanceof DataSourceServicePrincipalInKeyVault) {
* DataSourceServicePrincipalInKeyVault actualCredentialSPInKV
* = (DataSourceServicePrincipalInKeyVault) credentialEntityWithResponse.getValue();
* System.out
* .printf("Actual credential entity key vault endpoint: %s%n",
* actualCredentialSPInKV.getKeyVaultEndpoint());
* System.out.printf("Actual credential entity key vault client Id: %s%n",
* actualCredentialSPInKV.getKeyVaultClientId());
* System.out.printf("Actual credential entity key vault secret name for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientId());
* System.out.printf("Actual credential entity key vault secret for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientSecret());
* }
* });
*
*
*
* @param credentialId The data source credential entity unique id.
*
* @return The data source credential entity for the provided id.
* @throws IllegalArgumentException If {@code credentialId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code credentialId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getDataSourceCredentialWithResponse(String credentialId) {
try {
return withContext(context -> getDataSourceCredentialWithResponse(credentialId, context));
} catch (RuntimeException ex) {
return monoError(logger, ex);
}
}
Mono> getDataSourceCredentialWithResponse(String credentialId,
Context context) {
Objects.requireNonNull(credentialId, "'credentialId' cannot be null.");
return service.getCredentialWithResponseAsync(UUID.fromString(credentialId), context)
.map(response -> new SimpleResponse<>(response,
DataSourceCredentialEntityTransforms.fromInner(response.getValue())));
}
/**
* Deletes the data source credential entity identified by {@code credentialId}.
*
* Code sample
*
*
* final String datasourceCredentialId = "t00853f1-9080-447f-bacf-8dccf2e86f";
* metricsAdvisorAdminAsyncClient.deleteDataFeed(datasourceCredentialId);
*
*
*
* @param credentialId The data source credential entity id.
*
* @return An empty Mono.
* @throws IllegalArgumentException If {@code credentialId} does not conform to the
* UUID format specification.
* @throws NullPointerException thrown if the {@code credentialId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono deleteDataSourceCredential(String credentialId) {
return deleteDataSourceCredentialWithResponse(credentialId).flatMap(FluxUtil::toMono);
}
/**
* Deletes the data source credential entity identified by {@code credentialId}.
*
* Code sample
*
*
* final String datasourceCredentialId = "eh0854f1-8927-447f-bacf-8dccf2e86fwe";
* metricsAdvisorAdminAsyncClient.deleteDataSourceCredentialWithResponse(datasourceCredentialId)
* .subscribe(response ->
* System.out.printf("Datasource credential delete operation status : %s%n", response.getStatusCode()));
*
*
*
* @param credentialId The data source credential entity id.
*
* @return A response containing status code and headers returned after the operation.
* @throws IllegalArgumentException If {@code credentialId} does not conform to the
* UUID format specification.
* @throws NullPointerException thrown if the {@code credentialId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> deleteDataSourceCredentialWithResponse(String credentialId) {
try {
return withContext(context -> deleteDataSourceCredentialWithResponse(credentialId, context));
} catch (RuntimeException ex) {
return monoError(logger, ex);
}
}
Mono> deleteDataSourceCredentialWithResponse(String credentialId, Context context) {
Objects.requireNonNull(credentialId, "'credentialId' is required.");
return service.deleteCredentialWithResponseAsync(UUID.fromString(credentialId), context)
.doOnSubscribe(ignoredValue -> logger.info("Deleting deleteDataSourceCredentialEntity - {}", credentialId))
.doOnSuccess(response -> logger.info("Deleted deleteDataSourceCredentialEntity - {}", response))
.doOnError(
error -> logger.warning("Failed to delete deleteDataSourceCredentialEntity - {}", credentialId, error));
}
/**
* List information of all data source credential entities on the metrics advisor account.
*
* Code sample
*
*
* metricsAdvisorAdminAsyncClient.listDataSourceCredentials()
* .subscribe(datasourceCredentialEntity -> {
* if (datasourceCredentialEntity instanceof DataSourceServicePrincipalInKeyVault) {
* DataSourceServicePrincipalInKeyVault actualCredentialSPInKV
* = (DataSourceServicePrincipalInKeyVault) datasourceCredentialEntity;
* System.out
* .printf("Actual credential entity key vault endpoint: %s%n",
* actualCredentialSPInKV.getKeyVaultEndpoint());
* System.out.printf("Actual credential entity key vault client Id: %s%n",
* actualCredentialSPInKV.getKeyVaultClientId());
* System.out.printf("Actual credential entity key vault secret name for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientId());
* System.out.printf("Actual credential entity key vault secret for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientSecret());
* }
* });
*
*
*
* @return A {@link PagedFlux} containing information of all the {@link DataSourceCredentialEntity data feeds}
* in the account.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listDataSourceCredentials() {
return listDataSourceCredentials(new ListCredentialEntityOptions());
}
/**
* List information of all data source credential entities on the metrics advisor account.
*
* Code sample
*
*
* metricsAdvisorAdminAsyncClient.listDataSourceCredentials(
* new ListCredentialEntityOptions()
* .setMaxPageSize(3))
* .subscribe(datasourceCredentialEntity -> {
* if (datasourceCredentialEntity instanceof DataSourceServicePrincipalInKeyVault) {
* DataSourceServicePrincipalInKeyVault actualCredentialSPInKV
* = (DataSourceServicePrincipalInKeyVault) datasourceCredentialEntity;
* System.out
* .printf("Actual credential entity key vault endpoint: %s%n",
* actualCredentialSPInKV.getKeyVaultEndpoint());
* System.out.printf("Actual credential entity key vault client Id: %s%n",
* actualCredentialSPInKV.getKeyVaultClientId());
* System.out.printf("Actual credential entity key vault secret name for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientId());
* System.out.printf("Actual credential entity key vault secret for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientSecret());
* }
* });
*
*
*
* @param listCredentialEntityOptions The configurable {@link ListCredentialEntityOptions options} to pass for filtering
* the output result.
*
* @return A {@link PagedFlux} containing information of all the {@link DataSourceCredentialEntity data feeds}
* in the account.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux
listDataSourceCredentials(ListCredentialEntityOptions listCredentialEntityOptions) {
try {
return new PagedFlux<>(
() -> withContext(
context -> listCredentialEntitiesSinglePageAsync(listCredentialEntityOptions, context)),
continuationToken -> withContext(
context -> listCredentialEntitiesSNextPageAsync(continuationToken, context)));
} catch (RuntimeException ex) {
return new PagedFlux<>(() -> monoError(logger, ex));
}
}
private Mono>
listCredentialEntitiesSinglePageAsync(ListCredentialEntityOptions options, Context context) {
options = options != null ? options : new ListCredentialEntityOptions();
return service.listCredentialsSinglePageAsync(options.getSkip(), options.getMaxPageSize(), context)
.doOnRequest(ignoredValue -> logger.info("Listing information for all data source credentials"))
.doOnSuccess(response -> logger.info("Listed data source credentials {}", response))
.doOnError(error -> logger.warning("Failed to list all data source credential information - {}", error))
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue()
.stream()
.map(DataSourceCredentialEntityTransforms::fromInner)
.collect(Collectors.toList()),
res.getContinuationToken(), null));
}
private Mono> listCredentialEntitiesSNextPageAsync(String nextPageLink,
Context context) {
if (CoreUtils.isNullOrEmpty(nextPageLink)) {
return Mono.empty();
}
return service.listCredentialsNextSinglePageAsync(nextPageLink, context)
.doOnSubscribe(ignoredValue -> logger.info("Retrieving the next listing page - Page {}", nextPageLink))
.doOnSuccess(response -> logger.info("Retrieved the next listing page - Page {}", nextPageLink))
.doOnError(
error -> logger.warning("Failed to retrieve the next listing page - Page {}", nextPageLink, error))
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue()
.stream()
.map(DataSourceCredentialEntityTransforms::fromInner)
.collect(Collectors.toList()),
res.getContinuationToken(), null));
}
}