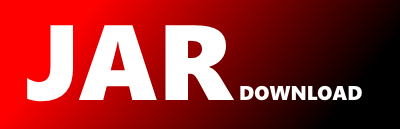
com.azure.ai.metricsadvisor.administration.MetricsAdvisorAdministrationClient Maven / Gradle / Ivy
Show all versions of azure-ai-metricsadvisor Show documentation
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.ai.metricsadvisor.administration;
import com.azure.ai.metricsadvisor.administration.models.AnomalyAlertConfiguration;
import com.azure.ai.metricsadvisor.administration.models.AnomalyDetectionConfiguration;
import com.azure.ai.metricsadvisor.administration.models.DataFeed;
import com.azure.ai.metricsadvisor.administration.models.DataFeedGranularity;
import com.azure.ai.metricsadvisor.administration.models.DataFeedIngestionProgress;
import com.azure.ai.metricsadvisor.administration.models.DataFeedIngestionSettings;
import com.azure.ai.metricsadvisor.administration.models.DataFeedIngestionStatus;
import com.azure.ai.metricsadvisor.administration.models.DataFeedMissingDataPointFillSettings;
import com.azure.ai.metricsadvisor.administration.models.DataFeedMissingDataPointFillType;
import com.azure.ai.metricsadvisor.administration.models.DataFeedOptions;
import com.azure.ai.metricsadvisor.administration.models.DataFeedRollupSettings;
import com.azure.ai.metricsadvisor.administration.models.DataFeedSchema;
import com.azure.ai.metricsadvisor.administration.models.DataSourceCredentialEntity;
import com.azure.ai.metricsadvisor.administration.models.ListAnomalyAlertConfigsOptions;
import com.azure.ai.metricsadvisor.administration.models.ListCredentialEntityOptions;
import com.azure.ai.metricsadvisor.administration.models.ListDataFeedFilter;
import com.azure.ai.metricsadvisor.administration.models.ListDataFeedIngestionOptions;
import com.azure.ai.metricsadvisor.administration.models.ListDataFeedOptions;
import com.azure.ai.metricsadvisor.administration.models.ListDetectionConfigsOptions;
import com.azure.ai.metricsadvisor.administration.models.ListHookOptions;
import com.azure.ai.metricsadvisor.administration.models.NotificationHook;
import com.azure.ai.metricsadvisor.implementation.MetricsAdvisorImpl;
import com.azure.ai.metricsadvisor.implementation.models.AnomalyAlertingConfiguration;
import com.azure.ai.metricsadvisor.implementation.models.AnomalyAlertingConfigurationPatch;
import com.azure.ai.metricsadvisor.implementation.models.AnomalyDetectionConfigurationPatch;
import com.azure.ai.metricsadvisor.implementation.models.CreateAnomalyAlertingConfigurationResponse;
import com.azure.ai.metricsadvisor.implementation.models.CreateAnomalyDetectionConfigurationResponse;
import com.azure.ai.metricsadvisor.implementation.models.CreateCredentialResponse;
import com.azure.ai.metricsadvisor.implementation.models.CreateDataFeedResponse;
import com.azure.ai.metricsadvisor.implementation.models.CreateHookResponse;
import com.azure.ai.metricsadvisor.implementation.models.DataFeedDetail;
import com.azure.ai.metricsadvisor.implementation.models.DataSourceCredential;
import com.azure.ai.metricsadvisor.implementation.models.DataSourceCredentialPatch;
import com.azure.ai.metricsadvisor.implementation.models.DataSourceType;
import com.azure.ai.metricsadvisor.implementation.models.EntityStatus;
import com.azure.ai.metricsadvisor.implementation.models.FillMissingPointType;
import com.azure.ai.metricsadvisor.implementation.models.Granularity;
import com.azure.ai.metricsadvisor.implementation.models.HookInfo;
import com.azure.ai.metricsadvisor.implementation.models.IngestionProgressResetOptions;
import com.azure.ai.metricsadvisor.implementation.models.IngestionStatusQueryOptions;
import com.azure.ai.metricsadvisor.implementation.models.NeedRollupEnum;
import com.azure.ai.metricsadvisor.implementation.models.RollUpMethod;
import com.azure.ai.metricsadvisor.implementation.models.ViewMode;
import com.azure.ai.metricsadvisor.implementation.util.AlertConfigurationTransforms;
import com.azure.ai.metricsadvisor.implementation.util.DataFeedTransforms;
import com.azure.ai.metricsadvisor.implementation.util.DataSourceCredentialEntityTransforms;
import com.azure.ai.metricsadvisor.implementation.util.DetectionConfigurationTransforms;
import com.azure.ai.metricsadvisor.implementation.util.HookTransforms;
import com.azure.ai.metricsadvisor.implementation.util.Utility;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceClient;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.PagedResponse;
import com.azure.core.http.rest.PagedResponseBase;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.ResponseBase;
import com.azure.core.http.rest.SimpleResponse;
import com.azure.core.util.Context;
import com.azure.core.util.CoreUtils;
import com.azure.core.util.logging.ClientLogger;
import java.time.OffsetDateTime;
import java.util.Objects;
import java.util.UUID;
import java.util.stream.Collectors;
import static com.azure.ai.metricsadvisor.administration.models.DataFeedGranularityType.CUSTOM;
import static com.azure.ai.metricsadvisor.implementation.util.Utility.parseOperationId;
import static com.azure.ai.metricsadvisor.implementation.util.Utility.toDataFeedIngestionProgress;
import static com.azure.ai.metricsadvisor.implementation.util.Utility.toDataFeedIngestionStatus;
/**
* This class provides a synchronous client to connect to the Metrics Advisor Azure Cognitive Service.
* This client provides synchronous methods to perform:
*
* - Connect to a variety of data sources, Metrics Advisor can connect to, and ingest multi-dimensional metric data
* from many data stores, including: SQL Server, Azure Blob Storage, and MongoDB. Use
* {@link com.azure.ai.metricsadvisor.administration.MetricsAdvisorAdministrationClient#createDataFeed(DataFeed)}
* method to add your respective data source.
* - Customize anomaly detection configuration to detect anomalies for your needs using the
* {@link com.azure.ai.metricsadvisor.administration.MetricsAdvisorAdministrationClient#createDetectionConfig(String, AnomalyDetectionConfiguration)}
* method.
* - Add real-time notification through multiple channels. Configure hooks for multiple alerting and detection
* configuration using the
* {@link com.azure.ai.metricsadvisor.administration.MetricsAdvisorAdministrationClient#createHook(NotificationHook)}
* method./li>
*
*
* Service clients are the point of interaction for developers to use Azure Metrics Advisor.
* {@link com.azure.ai.metricsadvisor.administration.MetricsAdvisorAdministrationClient} is the synchronous service client and
* {@link com.azure.ai.metricsadvisor.administration.MetricsAdvisorAdministrationAsyncClient} is the asynchronous service client.
* The examples shown in this document use a credential object named DefaultAzureCredential for authentication, which is
* appropriate for most scenarios, including local development and production environments. Additionally, we
* recommend using
* managed identity
* for authentication in production environments.
* You can find more information on different ways of authenticating and their corresponding credential types in the
* Azure Identity documentation".
*
*
* Sample: Construct a MetricsAdvisorAdministrationClient with DefaultAzureCredential
*
* The following code sample demonstrates the creation of a
* {@link com.azure.ai.metricsadvisor.administration.MetricsAdvisorAdministrationClient}, using the `DefaultAzureCredentialBuilder` to configure it.
*
*
*
* MetricsAdvisorAdministrationClient metricsAdvisorAdminClient =
* new MetricsAdvisorAdministrationClientBuilder()
* .credential(new DefaultAzureCredentialBuilder().build())
* .endpoint("{endpoint}")
* .buildClient();
*
*
*
* Further, see the code sample below to use
* {@link com.azure.ai.metricsadvisor.models.MetricsAdvisorKeyCredential MetricsAdvisorKeyCredential} for client creation.
*
*
*
* MetricsAdvisorAdministrationClient metricsAdvisorAdminClient =
* new MetricsAdvisorAdministrationClientBuilder()
* .credential(new MetricsAdvisorKeyCredential("{subscription_key}", "{api_key}"))
* .endpoint("{endpoint}")
* .buildClient();
*
*
*
* @see com.azure.ai.metricsadvisor
* @see MetricsAdvisorAdministrationAsyncClient
* @see MetricsAdvisorAdministrationClientBuilder
*/
@ServiceClient(builder = MetricsAdvisorAdministrationClientBuilder.class)
public final class MetricsAdvisorAdministrationClient {
private final ClientLogger logger = new ClientLogger(MetricsAdvisorAdministrationClient.class);
private final MetricsAdvisorImpl service;
/**
* Create a {@link MetricsAdvisorAdministrationClient client} that sends requests to the Metrics Advisor service's
* endpoint.
* Each service call goes through the {@link MetricsAdvisorAdministrationClientBuilder#pipeline http pipeline}.
*
* @param service The proxy service used to perform REST calls.
*/
MetricsAdvisorAdministrationClient(MetricsAdvisorImpl service) {
this.service = service;
}
/**
* Create a new data feed.
*
* Code sample
*
*
* DataFeed dataFeed = new DataFeed()
* .setName("dataFeedName")
* .setSource(new MySqlDataFeedSource("conn-string", "query"))
* .setGranularity(new DataFeedGranularity().setGranularityType(DataFeedGranularityType.DAILY))
* .setSchema(new DataFeedSchema(
* Arrays.asList(
* new DataFeedMetric("cost"),
* new DataFeedMetric("revenue")
* )).setDimensions(
* Arrays.asList(
* new DataFeedDimension("city"),
* new DataFeedDimension("category")
* ))
* )
* .setIngestionSettings(new DataFeedIngestionSettings(OffsetDateTime.parse("2020-01-01T00:00:00Z")))
* .setOptions(new DataFeedOptions()
* .setDescription("data feed description")
* .setRollupSettings(new DataFeedRollupSettings()
* .setRollupType(DataFeedRollupType.AUTO_ROLLUP)));
*
* DataFeed createdDataFeed = metricsAdvisorAdminClient.createDataFeed(dataFeed);
*
* System.out.printf("Data feed Id: %s%n", createdDataFeed.getId());
* System.out.printf("Data feed description: %s%n", createdDataFeed.getOptions().getDescription());
* System.out.printf("Data feed source type: %s%n", createdDataFeed.getSourceType());
* System.out.printf("Data feed creator: %s%n", createdDataFeed.getCreator());
*
*
*
* @param dataFeed The data feed to be created.
* @return The created data feed.
* @throws NullPointerException If {@code dataFeed}, {@code dataFeedName}, {@code dataFeedSource}, {@code metrics},
* {@code granularityType} or {@code ingestionStartTime} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public DataFeed createDataFeed(DataFeed dataFeed) {
return createDataFeedWithResponse(dataFeed, Context.NONE).getValue();
}
/**
* Create a new data feed with REST response.
*
* Code sample
*
*
* DataFeed dataFeed = new DataFeed()
* .setName("dataFeedName")
* .setSource(new MySqlDataFeedSource("conn-string", "query"))
* .setGranularity(new DataFeedGranularity().setGranularityType(DataFeedGranularityType.DAILY))
* .setSchema(new DataFeedSchema(
* Arrays.asList(
* new DataFeedMetric("cost"),
* new DataFeedMetric("revenue")
* )).setDimensions(
* Arrays.asList(
* new DataFeedDimension("city"),
* new DataFeedDimension("category")
* ))
* )
* .setIngestionSettings(new DataFeedIngestionSettings(OffsetDateTime.parse("2020-01-01T00:00:00Z")))
* .setOptions(new DataFeedOptions()
* .setDescription("data feed description")
* .setRollupSettings(new DataFeedRollupSettings()
* .setRollupType(DataFeedRollupType.AUTO_ROLLUP)));
*
* final Response<DataFeed> createdDataFeedResponse =
* metricsAdvisorAdminClient.createDataFeedWithResponse(dataFeed, Context.NONE);
*
* System.out.printf("Data feed create operation status: %s%n", createdDataFeedResponse.getStatusCode());
* DataFeed createdDataFeed = createdDataFeedResponse.getValue();
* System.out.printf("Data feed Id: %s%n", createdDataFeed.getId());
* System.out.printf("Data feed description: %s%n", createdDataFeed.getOptions().getDescription());
* System.out.printf("Data feed source type: %s%n", createdDataFeed.getSourceType());
* System.out.printf("Data feed creator: %s%n", createdDataFeed.getCreator());
*
*
*
* @param dataFeed The data feed to be created.
* @param context Additional context that is passed through the HTTP pipeline during the service call.
*
* @return A {@link Response} containing the created data feed.
* @throws NullPointerException If {@code dataFeed}, {@code dataFeedName}, {@code dataFeedSource}, {@code metrics},
* {@code granularityType} or {@code ingestionStartTime} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response createDataFeedWithResponse(DataFeed dataFeed, Context context) {
return createDataFeedWithResponseSync(dataFeed, context);
}
Response createDataFeedWithResponseSync(DataFeed dataFeed, Context context) {
Objects.requireNonNull(dataFeed, "'dataFeed' is required and cannot be null.");
Objects.requireNonNull(dataFeed.getSource(), "'dataFeedSource' is required and cannot be null.");
Objects.requireNonNull(dataFeed.getName(), "'dataFeedName' cannot be null or empty.");
final DataFeedSchema dataFeedSchema = dataFeed.getSchema();
final DataFeedGranularity dataFeedGranularity = dataFeed.getGranularity();
final DataFeedIngestionSettings dataFeedIngestionSettings = dataFeed.getIngestionSettings();
if (dataFeedSchema == null) {
throw logger
.logExceptionAsError(new NullPointerException("'dataFeedSchema.metrics' cannot be null or empty."));
} else {
Objects.requireNonNull(dataFeedSchema.getMetrics(), "'dataFeedSchema.metrics' cannot be null or empty.");
}
if (dataFeedGranularity == null) {
throw logger.logExceptionAsError(
new NullPointerException("'dataFeedGranularity.granularityType' is required and cannot be null."));
} else {
Objects.requireNonNull(dataFeedGranularity.getGranularityType(),
"'dataFeedGranularity.granularityType' is required.");
if (CUSTOM.equals(dataFeedGranularity.getGranularityType())) {
Objects.requireNonNull(dataFeedGranularity.getCustomGranularityValue(),
"'dataFeedGranularity.customGranularityValue' is required when granularity type is CUSTOM");
}
}
if (dataFeedIngestionSettings == null) {
throw logger.logExceptionAsError(new NullPointerException(
"'dataFeedIngestionSettings.ingestionStartTime' is required and cannot be null."));
} else {
Objects.requireNonNull(dataFeedIngestionSettings.getIngestionStartTime(),
"'dataFeedIngestionSettings.ingestionStartTime' is required and cannot be null.");
}
final DataFeedOptions finalDataFeedOptions
= dataFeed.getOptions() == null ? new DataFeedOptions() : dataFeed.getOptions();
final DataFeedRollupSettings dataFeedRollupSettings = finalDataFeedOptions.getRollupSettings() == null
? new DataFeedRollupSettings()
: finalDataFeedOptions.getRollupSettings();
final DataFeedMissingDataPointFillSettings dataFeedMissingDataPointFillSettings
= finalDataFeedOptions.getMissingDataPointFillSettings() == null
? new DataFeedMissingDataPointFillSettings()
: finalDataFeedOptions.getMissingDataPointFillSettings();
CreateDataFeedResponse createDataFeedResponse = service.createDataFeedWithResponse(DataFeedTransforms
.toDataFeedDetailSource(dataFeed.getSource())
.setDataFeedName(dataFeed.getName())
.setDataFeedDescription(finalDataFeedOptions.getDescription())
.setGranularityName(Granularity.fromString(dataFeedGranularity.getGranularityType() == null
? null
: dataFeedGranularity.getGranularityType().toString()))
.setGranularityAmount(dataFeedGranularity.getCustomGranularityValue())
.setDimension(DataFeedTransforms.toInnerDimensionsListForCreate(dataFeedSchema.getDimensions()))
.setMetrics(DataFeedTransforms.toInnerMetricsListForCreate(dataFeedSchema.getMetrics()))
.setTimestampColumn(dataFeedSchema.getTimestampColumn())
.setDataStartFrom(dataFeedIngestionSettings.getIngestionStartTime())
.setStartOffsetInSeconds(dataFeedIngestionSettings.getIngestionStartOffset() == null
? null
: dataFeedIngestionSettings.getIngestionStartOffset().getSeconds())
.setMaxConcurrency(dataFeedIngestionSettings.getDataSourceRequestConcurrency())
.setStopRetryAfterInSeconds(dataFeedIngestionSettings.getStopRetryAfter() == null
? null
: dataFeedIngestionSettings.getStopRetryAfter().getSeconds())
.setMinRetryIntervalInSeconds(dataFeedIngestionSettings.getIngestionRetryDelay() == null
? null
: dataFeedIngestionSettings.getIngestionRetryDelay().getSeconds())
.setRollUpColumns(dataFeedRollupSettings.getAutoRollupGroupByColumnNames())
.setRollUpMethod(RollUpMethod.fromString(dataFeedRollupSettings.getDataFeedAutoRollUpMethod() == null
? null
: dataFeedRollupSettings.getDataFeedAutoRollUpMethod().toString()))
.setNeedRollup(NeedRollupEnum.fromString(dataFeedRollupSettings.getRollupType() == null
? null
: dataFeedRollupSettings.getRollupType().toString()))
.setAllUpIdentification(dataFeedRollupSettings.getRollupIdentificationValue())
.setFillMissingPointType(
FillMissingPointType.fromString(dataFeedMissingDataPointFillSettings.getFillType() == null
? null
: dataFeedMissingDataPointFillSettings.getFillType().toString()))
.setFillMissingPointValue(dataFeedMissingDataPointFillSettings.getCustomFillValue())
.setViewMode(ViewMode.fromString(
finalDataFeedOptions.getAccessMode() == null ? null : finalDataFeedOptions.getAccessMode().toString()))
.setViewers(finalDataFeedOptions.getViewers())
.setAdmins(finalDataFeedOptions.getAdmins())
.setActionLinkTemplate(finalDataFeedOptions.getActionLinkTemplate()), context);
final String dataFeedId = parseOperationId(createDataFeedResponse.getDeserializedHeaders().getLocation());
return getDataFeedWithResponse(dataFeedId, context);
}
/**
* Get a data feed by its id.
*
* Code sample
*
*
* final String dataFeedId = "r47053f1-9080-09lo-bacf-8dccf2e86f";
* DataFeed dataFeed = metricsAdvisorAdminClient.getDataFeed(dataFeedId);
* System.out.printf("Data feed Id: %s%n", dataFeed.getId());
* System.out.printf("Data feed description: %s%n", dataFeed.getOptions().getDescription());
* System.out.printf("Data feed source type: %s%n", dataFeed.getSourceType());
* System.out.printf("Data feed creator: %s%n", dataFeed.getCreator());
*
*
*
* @param dataFeedId The data feed unique id.
*
* @return The data feed for the provided id.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code dataFeedId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public DataFeed getDataFeed(String dataFeedId) {
return getDataFeedWithResponse(dataFeedId, Context.NONE).getValue();
}
/**
* Get a data feed by its id with REST response.
*
* Code sample
*
*
* final String dataFeedId = "r47053f1-9080-09lo-bacf-8dccf2e86f";
* final Response<DataFeed> dataFeedResponse =
* metricsAdvisorAdminClient.getDataFeedWithResponse(dataFeedId, Context.NONE);
*
* System.out.printf("Data feed get operation status: %s%n", dataFeedResponse.getStatusCode());
* DataFeed dataFeed = dataFeedResponse.getValue();
* System.out.printf("Data feed Id: %s%n", dataFeed.getId());
* System.out.printf("Data feed description: %s%n", dataFeed.getOptions().getDescription());
* System.out.printf("Data feed source type: %s%n", dataFeed.getSourceType());
* System.out.printf("Data feed creator: %s%n", dataFeed.getCreator());
*
*
*
* @param dataFeedId The data feed unique id.
* @param context Additional context that is passed through the HTTP pipeline during the service call.
*
* @return The data feed for the provided id.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code dataFeedId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getDataFeedWithResponse(String dataFeedId, Context context) {
return getDataFeedWithResponseSync(dataFeedId, context);
}
Response getDataFeedWithResponseSync(String dataFeedId, Context context) {
Objects.requireNonNull(dataFeedId, "'dataFeedId' cannot be null.");
Response response = service.getDataFeedByIdWithResponse(UUID.fromString(dataFeedId), context);
return new SimpleResponse<>(response, DataFeedTransforms.fromInner(response.getValue()));
}
/**
* Update a data feed.
*
* Code sample
*
*
* final String dataFeedId = "r47053f1-9080-09lo-bacf-8dccf2e86f";
* DataFeed existingDataFeed = metricsAdvisorAdminClient.getDataFeed(dataFeedId);
* final DataFeed updatedDataFeed = metricsAdvisorAdminClient.updateDataFeed(
* existingDataFeed.setOptions(new DataFeedOptions().setDescription("set updated description")));
*
* System.out.printf("Data feed Id: %s%n", updatedDataFeed.getId());
* System.out.printf("Data feed updated description: %s%n", updatedDataFeed.getOptions().getDescription());
*
*
*
* @param dataFeed the data feed that needs to be updated.
*
* @return the updated data feed.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public DataFeed updateDataFeed(DataFeed dataFeed) {
return updateDataFeedWithResponse(dataFeed, Context.NONE).getValue();
}
/**
* Update a data feed with REST response.
*
* Code sample
*
*
* DataFeed existingDataFeed = new DataFeed();
* final Response<DataFeed> updateDataFeedWithResponse =
* metricsAdvisorAdminClient.updateDataFeedWithResponse(
* existingDataFeed.setOptions(new DataFeedOptions().setDescription("set updated description")),
* Context.NONE);
*
* System.out.printf("Data feed update operation status: %s%n", updateDataFeedWithResponse.getStatusCode());
* DataFeed dataFeed = updateDataFeedWithResponse.getValue();
* System.out.printf("Data feed Id: %s%n", dataFeed.getId());
* System.out.printf("Data feed updated description: %s%n", dataFeed.getOptions().getDescription());
*
*
*
* @param dataFeed the data feed that needs to be updated.
* @param context Additional context that is passed through the HTTP pipeline during the service call.
*
* @return the updated data feed.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response updateDataFeedWithResponse(DataFeed dataFeed, Context context) {
return updateDataFeedWithResponseSync(dataFeed, context);
}
Response updateDataFeedWithResponseSync(DataFeed dataFeed, Context context) {
final DataFeedIngestionSettings dataFeedIngestionSettings = dataFeed.getIngestionSettings();
final DataFeedOptions dataFeedOptions
= dataFeed.getOptions() == null ? new DataFeedOptions() : dataFeed.getOptions();
final DataFeedRollupSettings dataFeedRollupSettings = dataFeedOptions.getRollupSettings() == null
? new DataFeedRollupSettings()
: dataFeedOptions.getRollupSettings();
final DataFeedMissingDataPointFillSettings dataFeedMissingDataPointFillSettings
= dataFeedOptions.getMissingDataPointFillSettings() == null
? new DataFeedMissingDataPointFillSettings()
: dataFeedOptions.getMissingDataPointFillSettings();
service.updateDataFeedWithResponse(UUID.fromString(dataFeed.getId()),
DataFeedTransforms.toInnerForUpdate(dataFeed.getSource())
.setDataFeedName(dataFeed.getName())
.setDataFeedDescription(dataFeedOptions.getDescription())
.setTimestampColumn(dataFeed.getSchema() == null ? null : dataFeed.getSchema().getTimestampColumn())
.setDataStartFrom(dataFeed.getIngestionSettings().getIngestionStartTime())
.setStartOffsetInSeconds(dataFeedIngestionSettings.getIngestionStartOffset() == null
? null
: dataFeedIngestionSettings.getIngestionStartOffset().getSeconds())
.setMaxConcurrency(dataFeedIngestionSettings.getDataSourceRequestConcurrency())
.setStopRetryAfterInSeconds(dataFeedIngestionSettings.getStopRetryAfter() == null
? null
: dataFeedIngestionSettings.getStopRetryAfter().getSeconds())
.setMinRetryIntervalInSeconds(dataFeedIngestionSettings.getIngestionRetryDelay() == null
? null
: dataFeedIngestionSettings.getIngestionRetryDelay().getSeconds())
.setNeedRollup(dataFeedRollupSettings.getRollupType() != null
? NeedRollupEnum.fromString(dataFeedRollupSettings.getRollupType().toString())
: null)
.setRollUpColumns(dataFeedRollupSettings.getAutoRollupGroupByColumnNames())
.setRollUpMethod(dataFeedRollupSettings.getDataFeedAutoRollUpMethod() != null
? RollUpMethod.fromString(dataFeedRollupSettings.getDataFeedAutoRollUpMethod().toString())
: null)
.setAllUpIdentification(dataFeedRollupSettings.getRollupIdentificationValue())
.setFillMissingPointType(dataFeedMissingDataPointFillSettings.getFillType() != null
? FillMissingPointType.fromString(dataFeedMissingDataPointFillSettings.getFillType().toString())
: null)
.setFillMissingPointValue(
// For PATCH send 'fill-custom-value' over wire only for 'fill-custom-type'.
dataFeedMissingDataPointFillSettings.getFillType() == DataFeedMissingDataPointFillType.CUSTOM_VALUE
? dataFeedMissingDataPointFillSettings.getCustomFillValue()
: null)
.setViewMode(dataFeedOptions.getAccessMode() != null
? ViewMode.fromString(dataFeedOptions.getAccessMode().toString())
: null)
.setViewers(dataFeedOptions.getViewers())
.setAdmins(dataFeedOptions.getAdmins())
.setStatus(
dataFeed.getStatus() != null ? EntityStatus.fromString(dataFeed.getStatus().toString()) : null)
.setActionLinkTemplate(dataFeedOptions.getActionLinkTemplate()),
context);
return getDataFeedWithResponse(dataFeed.getId(), context);
}
/**
* Delete a data feed.
*
* Code sample
*
*
* final String dataFeedId = "r47053f1-9080-09lo-bacf-8dccf2e86f";
* metricsAdvisorAdminClient.deleteDataFeed(dataFeedId);
*
*
*
* @param dataFeedId The data feed unique id.
*
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code dataFeedId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void deleteDataFeed(String dataFeedId) {
deleteDataFeedWithResponse(dataFeedId, Context.NONE);
}
/**
* Delete a data feed with REST response.
*
* Code sample
*
*
* final String dataFeedId = "r47053f1-9080-09lo-bacf-8dccf2e86f";
* final Response<Void> response = metricsAdvisorAdminClient
* .deleteDataFeedWithResponse(dataFeedId, Context.NONE);
* System.out.printf("Data feed delete operation status : %s%n", response.getStatusCode());
*
*
*
* @param dataFeedId The data feed unique id.
* @param context Additional context that is passed through the HTTP pipeline during the service call.
*
* @return a REST Response.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code dataFeedId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response deleteDataFeedWithResponse(String dataFeedId, Context context) {
return deleteDataFeedWithResponseSync(dataFeedId, context);
}
Response deleteDataFeedWithResponseSync(String dataFeedId, Context context) {
Objects.requireNonNull(dataFeedId, "'dataFeedId' cannot be null.");
Response response = service.deleteDataFeedWithResponse(UUID.fromString(dataFeedId), context);
return new SimpleResponse<>(response, null);
}
/**
* List information of all data feeds on the metrics advisor account.
*
* Code sample
*
*
* metricsAdvisorAdminClient.listDataFeeds()
* .forEach(dataFeed -> {
* System.out.printf("Data feed Id: %s%n", dataFeed.getId());
* System.out.printf("Data feed description: %s%n", dataFeed.getOptions().getDescription());
* System.out.printf("Data feed source type: %s%n", dataFeed.getSourceType());
* System.out.printf("Data feed creator: %s%n", dataFeed.getCreator());
* });
*
*
*
* @return A {@link PagedIterable} containing information of all the {@link DataFeed data feeds} in the account.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listDataFeeds() {
return listDataFeeds(null, Context.NONE);
}
/**
* List information of all data feeds on the metrics advisor account with REST response.
*
* Code sample
*
*
* metricsAdvisorAdminClient.listDataFeeds(
* new ListDataFeedOptions()
* .setListDataFeedFilter(
* new ListDataFeedFilter()
* .setDataFeedStatus(DataFeedStatus.ACTIVE)
* .setDataFeedGranularityType(DataFeedGranularityType.DAILY))
* .setMaxPageSize(3), Context.NONE)
* .forEach(dataFeed -> {
* System.out.printf("Data feed Id: %s%n", dataFeed.getId());
* System.out.printf("Data feed description: %s%n", dataFeed.getOptions().getDescription());
* System.out.printf("Data feed source type: %s%n", dataFeed.getSourceType());
* System.out.printf("Data feed creator: %s%n", dataFeed.getCreator());
* System.out.printf("Data feed status: %s%n", dataFeed.getStatus());
* System.out.printf("Data feed granularity type: %s%n", dataFeed.getGranularity().getGranularityType());
* });
*
*
*
* @param options The configurable {@link ListDataFeedOptions options} to pass for filtering the output result.
* @param context Additional context that is passed through the Http pipeline during the service call.
*
* @return A {@link PagedIterable} containing information of all the {@link DataFeed data feeds} in the account.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listDataFeeds(ListDataFeedOptions options, Context context) {
return listDataFeedsSync(options, context);
}
PagedIterable listDataFeedsSync(ListDataFeedOptions options, Context context) {
return new PagedIterable<>(() -> listDataFeedsSinglePageSync(options, context),
continuationToken -> listDataFeedsNextPageSync(continuationToken, context));
}
private PagedResponse listDataFeedsSinglePageSync(ListDataFeedOptions options, Context context) {
options = options != null ? options : new ListDataFeedOptions();
final ListDataFeedFilter dataFeedFilter
= options.getListDataFeedFilter() != null ? options.getListDataFeedFilter() : new ListDataFeedFilter();
PagedResponse res = service.listDataFeedsSinglePage(dataFeedFilter.getName(),
dataFeedFilter.getSourceType() != null
? DataSourceType.fromString(dataFeedFilter.getSourceType().toString())
: null,
dataFeedFilter.getGranularityType() != null
? Granularity.fromString(dataFeedFilter.getGranularityType().toString())
: null,
dataFeedFilter.getStatus() != null ? EntityStatus.fromString(dataFeedFilter.getStatus().toString()) : null,
dataFeedFilter.getCreator(), options.getSkip(), options.getMaxPageSize(), context);
return new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().stream().map(DataFeedTransforms::fromInner).collect(Collectors.toList()),
res.getContinuationToken(), null);
}
private PagedResponse listDataFeedsNextPageSync(String nextPageLink, Context context) {
if (CoreUtils.isNullOrEmpty(nextPageLink)) {
return null;
}
PagedResponse res = service.listDataFeedsNextSinglePage(nextPageLink, context);
return new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().stream().map(DataFeedTransforms::fromInner).collect(Collectors.toList()),
res.getContinuationToken(), null);
}
/**
* Fetch the ingestion status of a data feed.
*
* Code sample
*
*
* final String dataFeedId = "4957a2f7-a0f4-4fc0-b8d7-d866c1df0f4c";
* final OffsetDateTime startTime = OffsetDateTime.parse("2020-01-01T00:00:00Z");
* final OffsetDateTime endTime = OffsetDateTime.parse("2020-09-09T00:00:00Z");
* final ListDataFeedIngestionOptions options = new ListDataFeedIngestionOptions(startTime, endTime);
* PagedIterable<DataFeedIngestionStatus> ingestionStatuses
* = metricsAdvisorAdminClient.listDataFeedIngestionStatus(dataFeedId, options);
*
* for (DataFeedIngestionStatus ingestionStatus : ingestionStatuses) {
* System.out.printf("Timestamp: %s%n", ingestionStatus.getTimestamp());
* System.out.printf("Status: %s%n", ingestionStatus.getStatus());
* System.out.printf("Message: %s%n", ingestionStatus.getMessage());
* }
*
*
*
* @param dataFeedId The data feed id.
* @param options The additional parameters.
* @return The ingestion statuses.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException If {@code dataFeedId}, {@code options}, {@code options.startTime},
* {@code options.endTime} is null.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listDataFeedIngestionStatus(String dataFeedId,
ListDataFeedIngestionOptions options) {
return listDataFeedIngestionStatus(dataFeedId, options, Context.NONE);
}
/**
* Fetch the ingestion status of a data feed.
*
* Code sample
*
*
* final String dataFeedId = "4957a2f7-a0f4-4fc0-b8d7-d866c1df0f4c";
* final OffsetDateTime startTime = OffsetDateTime.parse("2020-01-01T00:00:00Z");
* final OffsetDateTime endTime = OffsetDateTime.parse("2020-09-09T00:00:00Z");
* final ListDataFeedIngestionOptions options = new ListDataFeedIngestionOptions(startTime, endTime);
* PagedIterable<DataFeedIngestionStatus> ingestionStatuses
* = metricsAdvisorAdminClient.listDataFeedIngestionStatus(dataFeedId, options, Context.NONE);
* Stream<PagedResponse<DataFeedIngestionStatus>> ingestionStatusPageStream = ingestionStatuses.streamByPage();
* int[] pageCount = new int[1];
* ingestionStatusPageStream.forEach(ingestionStatusPage -> {
* System.out.printf("Page: %d%n", pageCount[0]++);
* for (DataFeedIngestionStatus ingestionStatus : ingestionStatusPage.getElements()) {
* System.out.printf("Timestamp: %s%n", ingestionStatus.getTimestamp());
* System.out.printf("Status: %s%n", ingestionStatus.getStatus());
* System.out.printf("Message: %s%n", ingestionStatus.getMessage());
* }
* });
*
*
*
* @param dataFeedId The data feed id.
* @param options The additional parameters.
* @param context Additional context that is passed through the Http pipeline during the service call.
* @return The ingestion statuses.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException If {@code dataFeedId}, {@code options}, {@code options.startTime},
* {@code options.endTime} is null.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listDataFeedIngestionStatus(String dataFeedId,
ListDataFeedIngestionOptions options, Context context) {
return listDataFeedIngestionStatusSync(dataFeedId, options, context);
}
PagedIterable listDataFeedIngestionStatusSync(String dataFeedId,
ListDataFeedIngestionOptions options, Context context) {
return new PagedIterable<>(() -> listDataFeedIngestionStatusSinglePageSync(dataFeedId, options, context),
continuationToken -> listDataFeedIngestionStatusNextPageSync(continuationToken, options, context));
}
private PagedResponse listDataFeedIngestionStatusSinglePageSync(String dataFeedId,
ListDataFeedIngestionOptions options, Context context) {
Objects.requireNonNull(dataFeedId, "'dataFeedId' is required.");
Objects.requireNonNull(options, "'options' is required.");
Objects.requireNonNull(options.getStartTime(), "'options.startTime' is required.");
Objects.requireNonNull(options.getEndTime(), "'options.endTime' is required.");
IngestionStatusQueryOptions queryOptions
= new IngestionStatusQueryOptions().setStartTime(options.getStartTime()).setEndTime(options.getEndTime());
PagedResponse res
= service.getDataFeedIngestionStatusSinglePage(UUID.fromString(dataFeedId), queryOptions, options.getSkip(),
options.getMaxPageSize(), context);
return new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
toDataFeedIngestionStatus(res.getValue()), res.getContinuationToken(), null);
}
private PagedResponse listDataFeedIngestionStatusNextPageSync(String nextPageLink,
ListDataFeedIngestionOptions options, Context context) {
if (CoreUtils.isNullOrEmpty(nextPageLink)) {
return null;
}
IngestionStatusQueryOptions queryOptions
= new IngestionStatusQueryOptions().setStartTime(options.getStartTime()).setEndTime(options.getEndTime());
PagedResponse res
= service.getDataFeedIngestionStatusNextSinglePage(nextPageLink, queryOptions, context);
return new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
toDataFeedIngestionStatus(res.getValue()), res.getContinuationToken(), null);
}
/**
* Refresh data ingestion for a period.
*
* The data in the data source for the given period will be re-ingested
* and any ingested data for the same period will be overwritten.
*
*
* Code sample
*
*
* final String dataFeedId = "4957a2f7-a0f4-4fc0-b8d7-d866c1df0f4c";
* final OffsetDateTime startTime = OffsetDateTime.parse("2020-01-01T00:00:00Z");
* final OffsetDateTime endTime = OffsetDateTime.parse("2020-03-03T00:00:00Z");
* metricsAdvisorAdminClient.refreshDataFeedIngestion(dataFeedId,
* startTime,
* endTime);
*
*
*
* @param dataFeedId The data feed id.
* @param startTime The start point of the period.
* @param endTime The end point of the period.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException If {@code dataFeedId}, {@code startTime}, {@code endTime} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void refreshDataFeedIngestion(String dataFeedId, OffsetDateTime startTime, OffsetDateTime endTime) {
refreshDataFeedIngestionWithResponse(dataFeedId, startTime, endTime, Context.NONE);
}
/**
* Refresh data ingestion for a period.
*
* The data in the data source for the given period will be re-ingested
* and any ingested data for the same period will be overwritten.
*
*
* Code sample
*
*
* final String dataFeedId = "4957a2f7-a0f4-4fc0-b8d7-d866c1df0f4c";
* final OffsetDateTime startTime = OffsetDateTime.parse("2020-01-01T00:00:00Z");
* final OffsetDateTime endTime = OffsetDateTime.parse("2020-03-03T00:00:00Z");
* Response<Void> response = metricsAdvisorAdminClient.refreshDataFeedIngestionWithResponse(dataFeedId,
* startTime,
* endTime,
* Context.NONE);
* System.out.printf("Response statusCode: %d%n", response.getStatusCode());
*
*
*
* @param dataFeedId The data feed id.
* @param startTime The start point of the period.
* @param endTime The end point of the period.
* @param context Additional context that is passed through the Http pipeline during the service call.
* @return The response.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException If {@code dataFeedId}, {@code startTime}, {@code endTime} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response refreshDataFeedIngestionWithResponse(String dataFeedId, OffsetDateTime startTime,
OffsetDateTime endTime, Context context) {
return refreshDataFeedIngestionWithResponseSync(dataFeedId, startTime, endTime, context);
}
Response refreshDataFeedIngestionWithResponseSync(String dataFeedId, OffsetDateTime startTime,
OffsetDateTime endTime, Context context) {
Objects.requireNonNull(dataFeedId, "'dataFeedId' is required.");
Objects.requireNonNull(startTime, "'startTime' is required.");
Objects.requireNonNull(endTime, "'endTime' is required.");
return service.resetDataFeedIngestionStatusWithResponse(UUID.fromString(dataFeedId),
new IngestionProgressResetOptions().setStartTime(startTime).setEndTime(endTime), context);
}
/**
* Retrieve the ingestion progress of a data feed.
*
* Code sample
*
*
* final String dataFeedId = "4957a2f7-a0f4-4fc0-b8d7-d866c1df0f4c";
* DataFeedIngestionProgress ingestionProgress
* = metricsAdvisorAdminClient.getDataFeedIngestionProgress(dataFeedId);
* System.out.printf("Latest active timestamp: %s%n", ingestionProgress.getLatestActiveTimestamp());
* System.out.printf("Latest successful timestamp: %s%n", ingestionProgress.getLatestSuccessTimestamp());
*
*
*
* @param dataFeedId The data feed id.
* @return The {@link DataFeedIngestionProgress} of the data feed.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code dataFeedId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public DataFeedIngestionProgress getDataFeedIngestionProgress(String dataFeedId) {
return getDataFeedIngestionProgressWithResponse(dataFeedId, Context.NONE).getValue();
}
/**
* Retrieve the ingestion progress of a data feed.
*
* Code sample
*
*
* final String dataFeedId = "4957a2f7-a0f4-4fc0-b8d7-d866c1df0f4c";
* Response<DataFeedIngestionProgress> response
* = metricsAdvisorAdminClient.getDataFeedIngestionProgressWithResponse(dataFeedId, Context.NONE);
* System.out.printf("Response statusCode: %d%n", response.getStatusCode());
* DataFeedIngestionProgress ingestionProgress = response.getValue();
* System.out.printf("Latest active timestamp: %s%n", ingestionProgress.getLatestActiveTimestamp());
* System.out.printf("Latest successful timestamp: %s%n", ingestionProgress.getLatestSuccessTimestamp());
*
*
*
* @param dataFeedId The data feed id.
* @param context Additional context that is passed through the Http pipeline during the service call.
* @return A {@link Response} containing {@link DataFeedIngestionProgress} of the data feed.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code dataFeedId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getDataFeedIngestionProgressWithResponse(String dataFeedId,
Context context) {
return getDataFeedIngestionProgressWithResponseSync(dataFeedId, context);
}
Response getDataFeedIngestionProgressWithResponseSync(String dataFeedId,
Context context) {
Objects.requireNonNull(dataFeedId, "'dataFeedId' is required.");
Response response
= service.getIngestionProgressWithResponse(UUID.fromString(dataFeedId), context);
return new SimpleResponse<>(response, toDataFeedIngestionProgress(response.getValue()));
}
/**
* Create a configuration to detect anomalies in the time series of a metric.
*
* Code sample
*
*
* final MetricWholeSeriesDetectionCondition wholeSeriesCondition = new MetricWholeSeriesDetectionCondition()
* .setConditionOperator(DetectionConditionOperator.OR)
* .setSmartDetectionCondition(new SmartDetectionCondition(
* 50,
* AnomalyDetectorDirection.BOTH,
* new SuppressCondition(50, 50)))
* .setHardThresholdCondition(new HardThresholdCondition(
* AnomalyDetectorDirection.BOTH,
* new SuppressCondition(5, 5))
* .setLowerBound(0.0)
* .setUpperBound(100.0))
* .setChangeThresholdCondition(new ChangeThresholdCondition(
* 50,
* 30,
* true,
* AnomalyDetectorDirection.BOTH,
* new SuppressCondition(2, 2)));
*
* final String detectionConfigName = "my_detection_config";
* final String detectionConfigDescription = "anomaly detection config for metric";
* final AnomalyDetectionConfiguration detectionConfig
* = new AnomalyDetectionConfiguration(detectionConfigName)
* .setDescription(detectionConfigDescription)
* .setWholeSeriesDetectionCondition(wholeSeriesCondition);
*
* final String metricId = "0b836da8-10e6-46cd-8f4f-28262e113a62";
* AnomalyDetectionConfiguration createdDetectionConfig = metricsAdvisorAdminClient
* .createDetectionConfig(metricId, detectionConfig);
* System.out.printf("Detection config Id: %s%n", createdDetectionConfig.getId());
* System.out.printf("Name: %s%n", createdDetectionConfig.getName());
* System.out.printf("Description: %s%n", createdDetectionConfig.getDescription());
* System.out.printf("MetricId: %s%n", createdDetectionConfig.getMetricId());
*
*
*
* @param metricId The metric id to associate the configuration with.
* @param detectionConfiguration The anomaly detection configuration.
* @return The created {@link AnomalyDetectionConfiguration}.
* @throws IllegalArgumentException If {@code metricId} does not conform to the UUID
* format specification, or {@code detectionConfiguration.name} is not set.
* @throws NullPointerException thrown if the {@code metricId} is null
* or {@code detectionConfiguration} is null
* or {@code detectionConfiguration.wholeSeriesCondition} is null
* or {@code seriesKey} is missing for any {@code MetricSingleSeriesDetectionCondition} in the configuration
* or {@code seriesGroupKey} is missing for any {@code MetricSeriesGroupDetectionCondition} in the configuration
* or {@code conditionOperator} is missing when multiple nested conditions are set in a
* {@code MetricSingleSeriesDetectionCondition} or {@code MetricSeriesGroupDetectionCondition}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public AnomalyDetectionConfiguration createDetectionConfig(String metricId,
AnomalyDetectionConfiguration detectionConfiguration) {
return createDetectionConfigWithResponse(metricId, detectionConfiguration, Context.NONE).getValue();
}
/**
* Create a configuration to detect anomalies in the time series of a metric.
*
* Code sample
*
*
* final MetricWholeSeriesDetectionCondition wholeSeriesCondition = new MetricWholeSeriesDetectionCondition()
* .setConditionOperator(DetectionConditionOperator.OR)
* .setSmartDetectionCondition(new SmartDetectionCondition(
* 50,
* AnomalyDetectorDirection.BOTH,
* new SuppressCondition(50, 50)))
* .setHardThresholdCondition(new HardThresholdCondition(
* AnomalyDetectorDirection.BOTH,
* new SuppressCondition(5, 5))
* .setLowerBound(0.0)
* .setUpperBound(100.0))
* .setChangeThresholdCondition(new ChangeThresholdCondition(
* 50,
* 30,
* true,
* AnomalyDetectorDirection.BOTH,
* new SuppressCondition(2, 2)));
*
* final String detectionConfigName = "my_detection_config";
* final String detectionConfigDescription = "anomaly detection config for metric";
* final AnomalyDetectionConfiguration detectionConfig
* = new AnomalyDetectionConfiguration(detectionConfigName)
* .setDescription(detectionConfigDescription)
* .setWholeSeriesDetectionCondition(wholeSeriesCondition);
*
* final String metricId = "0b836da8-10e6-46cd-8f4f-28262e113a62";
* Response<AnomalyDetectionConfiguration> response = metricsAdvisorAdminClient
* .createDetectionConfigWithResponse(metricId, detectionConfig, Context.NONE);
* System.out.printf("Response statusCode: %d%n", response.getStatusCode());
* AnomalyDetectionConfiguration createdDetectionConfig = response.getValue();
* System.out.printf("Detection config Id: %s%n", createdDetectionConfig.getId());
* System.out.printf("Name: %s%n", createdDetectionConfig.getName());
* System.out.printf("Description: %s%n", createdDetectionConfig.getDescription());
* System.out.printf("MetricId: %s%n", createdDetectionConfig.getMetricId());
*
*
*
* @param metricId The metric id to associate the configuration with.
* @param detectionConfiguration The anomaly detection configuration.
* @param context Additional context that is passed through the Http pipeline during the service call.
* @return A {@link Response} containing the created {@link AnomalyDetectionConfiguration}.
* @throws IllegalArgumentException If {@code metricId} does not conform to the UUID
* format specification, or {@code detectionConfiguration.name} is not set.
* @throws NullPointerException thrown if the {@code metricId} is null
* or {@code detectionConfiguration} is null
* or {@code detectionConfiguration.wholeSeriesCondition} is null
* or {@code seriesKey} is missing for any {@code MetricSingleSeriesDetectionCondition} in the configuration
* or {@code seriesGroupKey} is missing for any {@code MetricSeriesGroupDetectionCondition} in the configuration
* or {@code conditionOperator} is missing when multiple nested conditions are set in a
* {@code MetricSingleSeriesDetectionCondition} or {@code MetricSeriesGroupDetectionCondition}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response createDetectionConfigWithResponse(String metricId,
AnomalyDetectionConfiguration detectionConfiguration, Context context) {
return createDetectionConfigWithResponseSync(metricId, detectionConfiguration, context);
}
Response createDetectionConfigWithResponseSync(String metricId,
AnomalyDetectionConfiguration detectionConfiguration, Context context) {
Objects.requireNonNull(metricId, "metricId is required");
Objects.requireNonNull(detectionConfiguration, "detectionConfiguration is required");
final com.azure.ai.metricsadvisor.implementation.models.AnomalyDetectionConfiguration innerDetectionConfiguration
= DetectionConfigurationTransforms.toInnerForCreate(logger, metricId, detectionConfiguration);
CreateAnomalyDetectionConfigurationResponse response
= service.createAnomalyDetectionConfigurationWithResponse(innerDetectionConfiguration, context);
final String configurationId = Utility.parseOperationId(response.getDeserializedHeaders().getLocation());
Response configurationResponse
= getDetectionConfigWithResponse(configurationId, context);
return new ResponseBase(response.getRequest(), response.getStatusCode(),
response.getHeaders(), configurationResponse.getValue(), null);
}
/**
* Get the anomaly detection configuration by its id.
*
* Code sample
*
*
* final String detectionConfigId = "7b8069a1-1564-46da-9f50-b5d0dd9129ab";
* AnomalyDetectionConfiguration detectionConfig = metricsAdvisorAdminClient
* .getDetectionConfig(detectionConfigId);
* System.out.printf("Detection config Id: %s%n", detectionConfig.getId());
* System.out.printf("Name: %s%n", detectionConfig.getName());
* System.out.printf("Description: %s%n", detectionConfig.getDescription());
* System.out.printf("MetricId: %s%n", detectionConfig.getMetricId());
*
* System.out.printf("Detection conditions specified for configuration...%n");
*
* System.out.printf("Whole Series Detection Conditions:%n");
* MetricWholeSeriesDetectionCondition wholeSeriesDetectionCondition
* = detectionConfig.getWholeSeriesDetectionCondition();
*
* System.out.printf("- Use %s operator for multiple detection conditions:%n",
* wholeSeriesDetectionCondition.getConditionOperator());
*
* System.out.printf("- Smart Detection Condition:%n");
* System.out.printf(" - Sensitivity: %s%n",
* wholeSeriesDetectionCondition.getSmartDetectionCondition()
* .getSensitivity());
* System.out.printf(" - Detection direction: %s%n",
* wholeSeriesDetectionCondition.getSmartDetectionCondition()
* .getAnomalyDetectorDirection());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* wholeSeriesDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinNumber(),
* wholeSeriesDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf("- Hard Threshold Condition:%n");
* System.out.printf(" - Lower bound: %s%n",
* wholeSeriesDetectionCondition.getHardThresholdCondition()
* .getLowerBound());
* System.out.printf(" - Upper bound: %s%n",
* wholeSeriesDetectionCondition.getHardThresholdCondition()
* .getUpperBound());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* wholeSeriesDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* wholeSeriesDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf("- Change Threshold Condition:%n");
* System.out.printf(" - Change percentage: %s%n",
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .getChangePercentage());
* System.out.printf(" - Shift point: %s%n",
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .getShiftPoint());
* System.out.printf(" - Detect anomaly if within range: %s%n",
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .isWithinRange());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinRatio());
*
* List<MetricSingleSeriesDetectionCondition> seriesDetectionConditions
* = detectionConfig.getSeriesDetectionConditions();
* System.out.printf("Series Detection Conditions:%n");
* for (MetricSingleSeriesDetectionCondition seriesDetectionCondition : seriesDetectionConditions) {
* DimensionKey seriesKey = seriesDetectionCondition.getSeriesKey();
* final String seriesKeyStr
* = Arrays.toString(seriesKey.asMap().entrySet().toArray());
* System.out.printf("- Series Key:%s%n", seriesKeyStr);
* System.out.printf(" - Use %s operator for multiple detection conditions:%n",
* seriesDetectionCondition.getConditionOperator());
*
* System.out.printf(" - Smart Detection Condition:%n");
* System.out.printf(" - Sensitivity: %s%n",
* seriesDetectionCondition.getSmartDetectionCondition()
* .getSensitivity());
* System.out.printf(" - Detection direction: %s%n",
* seriesDetectionCondition.getSmartDetectionCondition()
* .getAnomalyDetectorDirection());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinNumber(),
* seriesDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf(" - Hard Threshold Condition:%n");
* System.out.printf(" - Lower bound: %s%n",
* seriesDetectionCondition.getHardThresholdCondition()
* .getLowerBound());
* System.out.printf(" - Upper bound: %s%n",
* seriesDetectionCondition.getHardThresholdCondition()
* .getUpperBound());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* seriesDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf(" - Change Threshold Condition:%n");
* System.out.printf(" - Change percentage: %s%n",
* seriesDetectionCondition.getChangeThresholdCondition()
* .getChangePercentage());
* System.out.printf(" - Shift point: %s%n",
* seriesDetectionCondition.getChangeThresholdCondition()
* .getShiftPoint());
* System.out.printf(" - Detect anomaly if within range: %s%n",
* seriesDetectionCondition.getChangeThresholdCondition()
* .isWithinRange());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* seriesDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinRatio());
* }
*
* List<MetricSeriesGroupDetectionCondition> seriesGroupDetectionConditions
* = detectionConfig.getSeriesGroupDetectionConditions();
* System.out.printf("Series Group Detection Conditions:%n");
* for (MetricSeriesGroupDetectionCondition seriesGroupDetectionCondition
* : seriesGroupDetectionConditions) {
* DimensionKey seriesGroupKey = seriesGroupDetectionCondition.getSeriesGroupKey();
* final String seriesGroupKeyStr
* = Arrays.toString(seriesGroupKey.asMap().entrySet().toArray());
* System.out.printf("- Series Group Key:%s%n", seriesGroupKeyStr);
* System.out.printf(" - Use %s operator for multiple detection conditions:%n",
* seriesGroupDetectionCondition.getConditionOperator());
*
* System.out.printf(" - Smart Detection Condition:%n");
* System.out.printf(" - Sensitivity: %s%n",
* seriesGroupDetectionCondition.getSmartDetectionCondition()
* .getSensitivity());
* System.out.printf(" - Detection direction: %s%n",
* seriesGroupDetectionCondition.getSmartDetectionCondition()
* .getAnomalyDetectorDirection());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesGroupDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinNumber(),
* seriesGroupDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf(" - Hard Threshold Condition:%n");
* System.out.printf(" - Lower bound: %s%n",
* seriesGroupDetectionCondition.getHardThresholdCondition()
* .getLowerBound());
* System.out.printf(" - Upper bound: %s%n",
* seriesGroupDetectionCondition.getHardThresholdCondition()
* .getUpperBound());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesGroupDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* seriesGroupDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf(" - Change Threshold Condition:%n");
* System.out.printf(" - Change percentage: %s%n",
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .getChangePercentage());
* System.out.printf(" - Shift point: %s%n",
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .getShiftPoint());
* System.out.printf(" - Detect anomaly if within range: %s%n",
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .isWithinRange());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinRatio());
* }
*
*
*
* @param detectionConfigurationId The anomaly detection configuration id.
* @return The {@link AnomalyDetectionConfiguration} identified by the given id.
* @throws IllegalArgumentException If {@code detectionConfigurationId} does not conform to the UUID
* format specification.
* @throws NullPointerException thrown if the {@code detectionConfigurationId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public AnomalyDetectionConfiguration getDetectionConfig(String detectionConfigurationId) {
return getDetectionConfigWithResponse(detectionConfigurationId, Context.NONE).getValue();
}
/**
* Get the anomaly detection configuration by its id.
*
* Code sample
*
*
* final String detectionConfigId = "7b8069a1-1564-46da-9f50-b5d0dd9129ab";
* Response<AnomalyDetectionConfiguration> response = metricsAdvisorAdminClient
* .getDetectionConfigWithResponse(detectionConfigId, Context.NONE);
* System.out.printf("Response statusCode: %d%n", response.getStatusCode());
* AnomalyDetectionConfiguration detectionConfig = response.getValue();
* System.out.printf("Detection config Id: %s%n", detectionConfig.getId());
* System.out.printf("Name: %s%n", detectionConfig.getName());
* System.out.printf("Description: %s%n", detectionConfig.getDescription());
* System.out.printf("MetricId: %s%n", detectionConfig.getMetricId());
*
* System.out.printf("Detection conditions specified for configuration...%n");
*
* System.out.printf("Whole Series Detection Conditions:%n");
* MetricWholeSeriesDetectionCondition wholeSeriesDetectionCondition
* = detectionConfig.getWholeSeriesDetectionCondition();
*
* System.out.printf("- Use %s operator for multiple detection conditions:%n",
* wholeSeriesDetectionCondition.getConditionOperator());
*
* System.out.printf("- Smart Detection Condition:%n");
* System.out.printf(" - Sensitivity: %s%n",
* wholeSeriesDetectionCondition.getSmartDetectionCondition()
* .getSensitivity());
* System.out.printf(" - Detection direction: %s%n",
* wholeSeriesDetectionCondition.getSmartDetectionCondition()
* .getAnomalyDetectorDirection());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* wholeSeriesDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinNumber(),
* wholeSeriesDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf("- Hard Threshold Condition:%n");
* System.out.printf(" - Lower bound: %s%n",
* wholeSeriesDetectionCondition.getHardThresholdCondition()
* .getLowerBound());
* System.out.printf(" - Upper bound: %s%n",
* wholeSeriesDetectionCondition.getHardThresholdCondition()
* .getUpperBound());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* wholeSeriesDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* wholeSeriesDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf("- Change Threshold Condition:%n");
* System.out.printf(" - Change percentage: %s%n",
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .getChangePercentage());
* System.out.printf(" - Shift point: %s%n",
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .getShiftPoint());
* System.out.printf(" - Detect anomaly if within range: %s%n",
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .isWithinRange());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* wholeSeriesDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinRatio());
*
* List<MetricSingleSeriesDetectionCondition> seriesDetectionConditions
* = detectionConfig.getSeriesDetectionConditions();
* System.out.printf("Series Detection Conditions:%n");
* for (MetricSingleSeriesDetectionCondition seriesDetectionCondition : seriesDetectionConditions) {
* DimensionKey seriesKey = seriesDetectionCondition.getSeriesKey();
* final String seriesKeyStr
* = Arrays.toString(seriesKey.asMap().entrySet().toArray());
* System.out.printf("- Series Key:%s%n", seriesKeyStr);
* System.out.printf(" - Use %s operator for multiple detection conditions:%n",
* seriesDetectionCondition.getConditionOperator());
*
* System.out.printf(" - Smart Detection Condition:%n");
* System.out.printf(" - Sensitivity: %s%n",
* seriesDetectionCondition.getSmartDetectionCondition()
* .getSensitivity());
* System.out.printf(" - Detection direction: %s%n",
* seriesDetectionCondition.getSmartDetectionCondition()
* .getAnomalyDetectorDirection());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinNumber(),
* seriesDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf(" - Hard Threshold Condition:%n");
* System.out.printf(" - Lower bound: %s%n",
* seriesDetectionCondition.getHardThresholdCondition()
* .getLowerBound());
* System.out.printf(" - Upper bound: %s%n",
* seriesDetectionCondition.getHardThresholdCondition()
* .getUpperBound());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* seriesDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf(" - Change Threshold Condition:%n");
* System.out.printf(" - Change percentage: %s%n",
* seriesDetectionCondition.getChangeThresholdCondition()
* .getChangePercentage());
* System.out.printf(" - Shift point: %s%n",
* seriesDetectionCondition.getChangeThresholdCondition()
* .getShiftPoint());
* System.out.printf(" - Detect anomaly if within range: %s%n",
* seriesDetectionCondition.getChangeThresholdCondition()
* .isWithinRange());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* seriesDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinRatio());
* }
*
* List<MetricSeriesGroupDetectionCondition> seriesGroupDetectionConditions
* = detectionConfig.getSeriesGroupDetectionConditions();
* System.out.printf("Series Group Detection Conditions:%n");
* for (MetricSeriesGroupDetectionCondition seriesGroupDetectionCondition
* : seriesGroupDetectionConditions) {
* DimensionKey seriesGroupKey = seriesGroupDetectionCondition.getSeriesGroupKey();
* final String seriesGroupKeyStr
* = Arrays.toString(seriesGroupKey.asMap().entrySet().toArray());
* System.out.printf("- Series Group Key:%s%n", seriesGroupKeyStr);
* System.out.printf(" - Use %s operator for multiple detection conditions:%n",
* seriesGroupDetectionCondition.getConditionOperator());
*
* System.out.printf(" - Smart Detection Condition:%n");
* System.out.printf(" - Sensitivity: %s%n",
* seriesGroupDetectionCondition.getSmartDetectionCondition()
* .getSensitivity());
* System.out.printf(" - Detection direction: %s%n",
* seriesGroupDetectionCondition.getSmartDetectionCondition()
* .getAnomalyDetectorDirection());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesGroupDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinNumber(),
* seriesGroupDetectionCondition.getSmartDetectionCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf(" - Hard Threshold Condition:%n");
* System.out.printf(" - Lower bound: %s%n",
* seriesGroupDetectionCondition.getHardThresholdCondition()
* .getLowerBound());
* System.out.printf(" - Upper bound: %s%n",
* seriesGroupDetectionCondition.getHardThresholdCondition()
* .getUpperBound());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesGroupDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* seriesGroupDetectionCondition.getHardThresholdCondition()
* .getSuppressCondition().getMinRatio());
*
* System.out.printf(" - Change Threshold Condition:%n");
* System.out.printf(" - Change percentage: %s%n",
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .getChangePercentage());
* System.out.printf(" - Shift point: %s%n",
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .getShiftPoint());
* System.out.printf(" - Detect anomaly if within range: %s%n",
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .isWithinRange());
* System.out.printf(" - Suppress conditions: minimum number: %s; minimum ratio: %s%n",
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinNumber(),
* seriesGroupDetectionCondition.getChangeThresholdCondition()
* .getSuppressCondition().getMinRatio());
* }
*
*
*
* @param detectionConfigurationId The anomaly detection configuration id.
* @param context Additional context that is passed through the Http pipeline during the service call.
* @return A {@link Response} containing the {@link AnomalyDetectionConfiguration} for the provided id.
* @throws IllegalArgumentException If {@code detectionConfigurationId} does not conform to the UUID
* format specification.
* @throws NullPointerException thrown if the {@code detectionConfigurationId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getDetectionConfigWithResponse(String detectionConfigurationId,
Context context) {
return getDetectionConfigWithResponseSync(detectionConfigurationId, context);
}
Response getDetectionConfigWithResponseSync(String detectionConfigurationId,
Context context) {
Objects.requireNonNull(detectionConfigurationId, "detectionConfigurationId is required.");
Response response
= service.getAnomalyDetectionConfigurationWithResponse(UUID.fromString(detectionConfigurationId), context);
AnomalyDetectionConfiguration configuration = DetectionConfigurationTransforms.fromInner(response.getValue());
return new ResponseBase(response.getRequest(), response.getStatusCode(),
response.getHeaders(), configuration, null);
}
/**
* Update a configuration to detect anomalies in the time series of a metric.
*
* Code sample
*
*
* final String detectionConfigId = "7b8069a1-1564-46da-9f50-b5d0dd9129ab";
* AnomalyDetectionConfiguration detectionConfig = metricsAdvisorAdminClient
* .getDetectionConfig(detectionConfigId);
*
* detectionConfig.setName("updated config name");
* detectionConfig.setDescription("updated with more detection conditions");
* DimensionKey seriesGroupKey = new DimensionKey()
* .put("city", "Seoul");
* detectionConfig.addSeriesGroupDetectionCondition(
* new MetricSeriesGroupDetectionCondition(seriesGroupKey)
* .setSmartDetectionCondition(new SmartDetectionCondition(
* 10.0,
* AnomalyDetectorDirection.UP,
* new SuppressCondition(2, 2))));
*
* AnomalyDetectionConfiguration updatedDetectionConfig = metricsAdvisorAdminClient
* .updateDetectionConfig(detectionConfig);
*
* System.out.printf("Detection config Id: %s%n", updatedDetectionConfig.getId());
* System.out.printf("Name: %s%n", updatedDetectionConfig.getName());
* System.out.printf("Description: %s%n", updatedDetectionConfig.getDescription());
* System.out.printf("MetricId: %s%n", updatedDetectionConfig.getMetricId());
*
*
*
* @param detectionConfiguration The anomaly detection configuration.
* @return The updated {@link AnomalyDetectionConfiguration}.
* @throws NullPointerException thrown if the {@code detectionConfiguration} is null
* or {@code detectionConfiguration.id} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public AnomalyDetectionConfiguration updateDetectionConfig(AnomalyDetectionConfiguration detectionConfiguration) {
return updateDetectionConfigWithResponse(detectionConfiguration, Context.NONE).getValue();
}
/**
* Update a configuration to detect anomalies in the time series of a metric.
*
* Code sample
*
*
* final String detectionConfigId = "7b8069a1-1564-46da-9f50-b5d0dd9129ab";
* Response<AnomalyDetectionConfiguration> getResponse = metricsAdvisorAdminClient
* .getDetectionConfigWithResponse(detectionConfigId, Context.NONE);
* AnomalyDetectionConfiguration detectionConfig = getResponse.getValue();
* detectionConfig.setName("updated config name");
* detectionConfig.setDescription("updated with more detection conditions");
* DimensionKey seriesGroupKey = new DimensionKey()
* .put("city", "Seoul");
* detectionConfig.addSeriesGroupDetectionCondition(
* new MetricSeriesGroupDetectionCondition(seriesGroupKey)
* .setSmartDetectionCondition(new SmartDetectionCondition(
* 10.0,
* AnomalyDetectorDirection.UP,
* new SuppressCondition(2, 2))));
*
* Response<AnomalyDetectionConfiguration> updateResponse = metricsAdvisorAdminClient
* .updateDetectionConfigWithResponse(detectionConfig, Context.NONE);
*
* System.out.printf("Response StatusCode: %s%n", updateResponse.getStatusCode());
* AnomalyDetectionConfiguration updatedDetectionConfig = updateResponse.getValue();
* System.out.printf("Detection config Id: %s%n", updatedDetectionConfig.getId());
* System.out.printf("Name: %s%n", updatedDetectionConfig.getName());
* System.out.printf("Description: %s%n", updatedDetectionConfig.getDescription());
* System.out.printf("MetricId: %s%n", updatedDetectionConfig.getMetricId());
*
*
*
* @param detectionConfiguration The anomaly detection configuration.
* @param context Additional context that is passed through the Http pipeline during the service call.
* @return A {@link Response} containing the updated {@link AnomalyDetectionConfiguration}.
* @throws NullPointerException thrown if the {@code detectionConfiguration} is null
* or {@code detectionConfiguration.id} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response
updateDetectionConfigWithResponse(AnomalyDetectionConfiguration detectionConfiguration, Context context) {
return updateDetectionConfigWithResponseSync(detectionConfiguration, context);
}
Response
updateDetectionConfigWithResponseSync(AnomalyDetectionConfiguration detectionConfiguration, Context context) {
Objects.requireNonNull(detectionConfiguration, "detectionConfiguration is required");
Objects.requireNonNull(detectionConfiguration.getId(), "detectionConfiguration.id is required");
final AnomalyDetectionConfigurationPatch innerDetectionConfigurationPatch
= DetectionConfigurationTransforms.toInnerForUpdate(logger, detectionConfiguration);
Response response
= service.updateAnomalyDetectionConfigurationWithResponse(UUID.fromString(detectionConfiguration.getId()),
innerDetectionConfigurationPatch, context);
Response configurationResponse
= getDetectionConfigWithResponse(detectionConfiguration.getId(), context);
return new ResponseBase(response.getRequest(), response.getStatusCode(),
response.getHeaders(), configurationResponse.getValue(), null);
}
/**
* Delete a metric anomaly detection configuration.
*
* Code sample
*
*
* final String detectionConfigId = "7b8069a1-1564-46da-9f50-b5d0dd9129ab";
* metricsAdvisorAdminClient
* .deleteDetectionConfig(detectionConfigId);
*
*
*
* @param detectionConfigurationId The metric anomaly detection configuration unique id.
*
* @throws NullPointerException thrown if the {@code detectionConfigurationId} is null.
* @throws IllegalArgumentException If {@code detectionConfigurationId} does not conform to the UUID
* format specification.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void deleteDetectionConfig(String detectionConfigurationId) {
deleteDetectionConfigWithResponse(detectionConfigurationId, Context.NONE).getValue();
}
/**
* Delete a metric anomaly detection configuration.
*
* Code sample
*
*
* final String detectionConfigId = "7b8069a1-1564-46da-9f50-b5d0dd9129ab";
* Response<Void> response = metricsAdvisorAdminClient
* .deleteDetectionConfigWithResponse(detectionConfigId, Context.NONE);
* System.out.printf("Response Status Code: %s%n", response.getStatusCode());
*
*
*
* @param detectionConfigurationId The metric anomaly detection configuration unique id.
* @param context Additional context that is passed through the Http pipeline during the service call.
* @return A {@link Response} of containing result of delete operation.
* @throws NullPointerException thrown if the {@code detectionConfigurationId} is null.
* @throws IllegalArgumentException If {@code detectionConfigurationId} does not conform to the UUID
* format specification.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response deleteDetectionConfigWithResponse(String detectionConfigurationId, Context context) {
return deleteDetectionConfigWithResponseSync(detectionConfigurationId, context);
}
Response deleteDetectionConfigWithResponseSync(String detectionConfigurationId, Context context) {
Objects.requireNonNull(detectionConfigurationId, "detectionConfigurationId is required.");
return service.deleteHookWithResponse(UUID.fromString(detectionConfigurationId), context);
}
/**
* Given a metric id, retrieve all anomaly detection configurations applied to it.
*
* Code sample
*
*
* final String metricId = "0b836da8-10e6-46cd-8f4f-28262e113a62";
* PagedIterable<AnomalyDetectionConfiguration> configsIterable
* = metricsAdvisorAdminClient.listDetectionConfigs(metricId);
*
* for (AnomalyDetectionConfiguration detectionConfig : configsIterable) {
* System.out.printf("Detection config Id: %s%n", detectionConfig.getId());
* System.out.printf("Name: %s%n", detectionConfig.getName());
* System.out.printf("Description: %s%n", detectionConfig.getDescription());
* System.out.printf("MetricId: %s%n", detectionConfig.getMetricId());
* }
*
*
*
* @param metricId The metric id.
* @return The anomaly detection configurations.
* @throws NullPointerException thrown if the {@code metricId} is null.
* @throws IllegalArgumentException If {@code metricId} does not conform to the UUID format specification.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listDetectionConfigs(String metricId) {
return listDetectionConfigs(metricId, null, Context.NONE);
}
/**
* Given a metric id, retrieve all anomaly detection configurations applied to it.
*
* Code sample
*
*
* final String metricId = "0b836da8-10e6-46cd-8f4f-28262e113a62";
* PagedIterable<AnomalyDetectionConfiguration> configsIterable
* = metricsAdvisorAdminClient.listDetectionConfigs(metricId,
* new ListDetectionConfigsOptions(), Context.NONE);
*
* Stream<PagedResponse<AnomalyDetectionConfiguration>> configByPageStream
* = configsIterable.streamByPage();
*
* configByPageStream.forEach(configPage -> {
* IterableStream<AnomalyDetectionConfiguration> pageElements = configPage.getElements();
* for (AnomalyDetectionConfiguration detectionConfig : pageElements) {
* System.out.printf("Detection config Id: %s%n", detectionConfig.getId());
* System.out.printf("Name: %s%n", detectionConfig.getName());
* System.out.printf("Description: %s%n", detectionConfig.getDescription());
* System.out.printf("MetricId: %s%n", detectionConfig.getMetricId());
* }
* });
*
*
*
* @param metricId The metric id.
* @param options th e additional configurable options to specify when querying the result.
* @param context Additional context that is passed through the Http pipeline during the service call.
* @return The anomaly detection configurations.
* @throws NullPointerException thrown if the {@code metricId} is null.
* @throws IllegalArgumentException If {@code metricId} does not conform to the UUID format specification.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listDetectionConfigs(String metricId,
ListDetectionConfigsOptions options, Context context) {
return listDetectionConfigsSync(metricId, options, context);
}
PagedIterable listDetectionConfigsSync(String metricId,
ListDetectionConfigsOptions options, Context context) {
return new PagedIterable<>(() -> listAnomalyDetectionConfigsSinglePageSync(metricId, options, context),
continuationToken -> listAnomalyDetectionConfigsNextPageSync(continuationToken, context));
}
private PagedResponse listAnomalyDetectionConfigsSinglePageSync(String metricId,
ListDetectionConfigsOptions options, Context context) {
if (options == null) {
options = new ListDetectionConfigsOptions();
}
PagedResponse response
= service.getAnomalyDetectionConfigurationsByMetricSinglePage(UUID.fromString(metricId), options.getSkip(),
options.getMaxPageSize(), context);
return DetectionConfigurationTransforms.fromInnerPagedResponse(response);
}
private PagedResponse listAnomalyDetectionConfigsNextPageSync(String nextPageLink,
Context context) {
if (CoreUtils.isNullOrEmpty(nextPageLink)) {
return null;
}
PagedResponse response
= service.getAnomalyDetectionConfigurationsByMetricNextSinglePage(nextPageLink, context);
return DetectionConfigurationTransforms.fromInnerPagedResponse(response);
}
/**
* Creates a notificationHook that receives anomaly incident alerts.
*
* Code sample
*
*
* NotificationHook emailNotificationHook = new EmailNotificationHook("email notificationHook")
* .setDescription("my email notificationHook")
* .setEmailsToAlert(new ArrayList<String>() {{
* add("alertme@alertme.com");
* }})
* .setExternalLink("https://adwiki.azurewebsites.net/articles/howto/alerts/create-hooks.html");
*
* NotificationHook notificationHook = metricsAdvisorAdminClient.createHook(emailNotificationHook);
* EmailNotificationHook createdEmailHook = (EmailNotificationHook) notificationHook;
* System.out.printf("NotificationHook Id: %s%n", createdEmailHook.getId());
* System.out.printf("NotificationHook Name: %s%n", createdEmailHook.getName());
* System.out.printf("NotificationHook Description: %s%n", createdEmailHook.getDescription());
* System.out.printf("NotificationHook External Link: %s%n", createdEmailHook.getExternalLink());
* System.out.printf("NotificationHook Emails: %s%n", String.join(",",
* createdEmailHook.getEmailsToAlert()));
*
*
*
* @param notificationHook The notificationHook.
* @return The created {@link NotificationHook}.
* @throws NullPointerException If {@code notificationHook}, {@code notificationHook.name},
* {@code notificationHook.endpoint} (for web notificationHook) is null.
* @throws IllegalArgumentException If at least one email not present for email notificationHook.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public NotificationHook createHook(NotificationHook notificationHook) {
return createHookWithResponse(notificationHook, Context.NONE).getValue();
}
/**
* Creates a notificationHook that receives anomaly incident alerts.
*
* Code sample
*
*
* NotificationHook emailNotificationHook = new EmailNotificationHook("email hook")
* .setDescription("my email hook")
* .setEmailsToAlert(new ArrayList<String>() {{
* add("alertme@alertme.com");
* }})
* .setExternalLink("https://adwiki.azurewebsites.net/articles/howto/alerts/create-hooks.html");
*
* Response<NotificationHook> response
* = metricsAdvisorAdminClient.createHookWithResponse(emailNotificationHook, Context.NONE);
* System.out.printf("Response statusCode: %d%n", response.getStatusCode());
* EmailNotificationHook createdEmailHook = (EmailNotificationHook) response.getValue();
* System.out.printf("NotificationHook Id: %s%n", createdEmailHook.getId());
* System.out.printf("NotificationHook Name: %s%n", createdEmailHook.getName());
* System.out.printf("NotificationHook Description: %s%n", createdEmailHook.getDescription());
* System.out.printf("NotificationHook External Link: %s%n", createdEmailHook.getExternalLink());
* System.out.printf("NotificationHook Emails: %s%n", String.join(",",
* createdEmailHook.getEmailsToAlert()));
*
*
*
* @param notificationHook The notificationHook.
* @param context Additional context that is passed through the Http pipeline during the service call.
* @return A {@link Response} containing the created {@link NotificationHook}.
* @throws NullPointerException If {@code notificationHook}, {@code notificationHook.name},
* {@code notificationHook.endpoint} (for web notificationHook) is null.
* @throws IllegalArgumentException If at least one email not present for email notificationHook.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response createHookWithResponse(NotificationHook notificationHook, Context context) {
return createHookWithResponseSync(notificationHook, context);
}
Response createHookWithResponseSync(NotificationHook notificationHook, Context context) {
Objects.requireNonNull(notificationHook, "'notificationHook' cannot be null.");
CreateHookResponse response
= service.createHookWithResponse(HookTransforms.toInnerForCreate(logger, notificationHook), context);
final String hookUri = response.getDeserializedHeaders().getLocation();
final String hookId = parseOperationId(hookUri);
Response hookResponse = getHookWithResponse(hookId, context);
return new ResponseBase(response.getRequest(), response.getStatusCode(),
response.getHeaders(), hookResponse.getValue(), null);
}
/**
* Get a hook by its id.
*
* Code sample
*
*
* final String hookId = "f00853f1-6627-447f-bacf-8dccf2e86fed";
* NotificationHook notificationHook = metricsAdvisorAdminClient.getHook(hookId);
* if (notificationHook instanceof EmailNotificationHook) {
* EmailNotificationHook emailHook = (EmailNotificationHook) notificationHook;
* System.out.printf("NotificationHook Id: %s%n", emailHook.getId());
* System.out.printf("NotificationHook Name: %s%n", emailHook.getName());
* System.out.printf("NotificationHook Description: %s%n", emailHook.getDescription());
* System.out.printf("NotificationHook External Link: %s%n", emailHook.getExternalLink());
* System.out.printf("NotificationHook Emails: %s%n", String.join(",", emailHook.getEmailsToAlert()));
* } else if (notificationHook instanceof WebNotificationHook) {
* WebNotificationHook webHook = (WebNotificationHook) notificationHook;
* System.out.printf("NotificationHook Id: %s%n", webHook.getId());
* System.out.printf("NotificationHook Name: %s%n", webHook.getName());
* System.out.printf("NotificationHook Description: %s%n", webHook.getDescription());
* System.out.printf("NotificationHook External Link: %s%n", webHook.getExternalLink());
* System.out.printf("NotificationHook Endpoint: %s%n", webHook.getEndpoint());
* System.out.printf("NotificationHook Headers: %s%n", webHook.getHttpHeaders());
* }
*
*
*
* @param hookId The hook unique id.
* @return The {@link NotificationHook} for the provided id.
* @throws IllegalArgumentException If {@code hookId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code hookId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public NotificationHook getHook(String hookId) {
return getHookWithResponse(hookId, Context.NONE).getValue();
}
/**
* Get a hook by its id.
*
* Code sample
*
*
* final String hookId = "f00853f1-6627-447f-bacf-8dccf2e86fed";
* Response<NotificationHook> response = metricsAdvisorAdminClient.getHookWithResponse(hookId, Context.NONE);
* System.out.printf("Response statusCode: %d%n", response.getStatusCode());
* NotificationHook notificationHook = response.getValue();
* if (notificationHook instanceof EmailNotificationHook) {
* EmailNotificationHook emailHook = (EmailNotificationHook) notificationHook;
* System.out.printf("NotificationHook Id: %s%n", emailHook.getId());
* System.out.printf("NotificationHook Name: %s%n", emailHook.getName());
* System.out.printf("NotificationHook Description: %s%n", emailHook.getDescription());
* System.out.printf("NotificationHook External Link: %s%n", emailHook.getExternalLink());
* System.out.printf("NotificationHook Emails: %s%n", String.join(",", emailHook.getEmailsToAlert()));
* } else if (notificationHook instanceof WebNotificationHook) {
* WebNotificationHook webHook = (WebNotificationHook) notificationHook;
* System.out.printf("NotificationHook Id: %s%n", webHook.getId());
* System.out.printf("NotificationHook Name: %s%n", webHook.getName());
* System.out.printf("NotificationHook Description: %s%n", webHook.getDescription());
* System.out.printf("NotificationHook External Link: %s%n", webHook.getExternalLink());
* System.out.printf("NotificationHook Endpoint: %s%n", webHook.getEndpoint());
* System.out.printf("NotificationHook Headers: %s%n", webHook.getHttpHeaders());
* }
*
*
*
* @param hookId The hook unique id.
* @param context Additional context that is passed through the Http pipeline during the service call.
* @return A {@link Response} containing the {@link NotificationHook} for the provided id.
* @throws IllegalArgumentException If {@code hookId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code hookId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getHookWithResponse(String hookId, Context context) {
return getHookWithResponseSync(hookId, context);
}
Response getHookWithResponseSync(String hookId, Context context) {
Objects.requireNonNull(hookId, "hookId is required.");
Response innerResponse = service.getHookWithResponse(UUID.fromString(hookId), context);
return new ResponseBase(innerResponse.getRequest(), innerResponse.getStatusCode(),
innerResponse.getHeaders(), HookTransforms.fromInner(logger, innerResponse.getValue()), null);
}
/**
* Update an existing notificationHook.
*
* Code sample
*
*
* final String emailHookId = "f00853f1-6627-447f-bacf-8dccf2e86fed";
* NotificationHook notificationHook = metricsAdvisorAdminClient.getHook(emailHookId);
* EmailNotificationHook emailHook = (EmailNotificationHook) notificationHook;
* List<String> emailsToUpdate = new ArrayList<>(emailHook.getEmailsToAlert());
* emailsToUpdate.remove("alertme@alertme.com");
* emailsToUpdate.add("alertme2@alertme.com");
* emailsToUpdate.add("alertme3@alertme.com");
* emailHook.setEmailsToAlert(emailsToUpdate);
* NotificationHook updatedNotificationHook = metricsAdvisorAdminClient.updateHook(emailHook);
* EmailNotificationHook updatedEmailHook = (EmailNotificationHook) updatedNotificationHook;
* System.out.printf("NotificationHook Id: %s%n", updatedEmailHook.getId());
* System.out.printf("NotificationHook Name: %s%n", updatedEmailHook.getName());
* System.out.printf("NotificationHook Description: %s%n", updatedEmailHook.getDescription());
* System.out.printf("NotificationHook External Link: %s%n", updatedEmailHook.getExternalLink());
* System.out.printf("NotificationHook Emails: %s%n", String.join(",",
* updatedEmailHook.getEmailsToAlert()));
*
*
*
* @param notificationHook The notificationHook to update.
* @return The updated {@link NotificationHook}.
* @throws NullPointerException If {@code notificationHook.id} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public NotificationHook updateHook(NotificationHook notificationHook) {
return updateHookWithResponse(notificationHook, Context.NONE).getValue();
}
/**
* Update an existing notificationHook.
*
* Code sample
*
*
* final String emailHookId = "f00853f1-6627-447f-bacf-8dccf2e86fed";
* Response<NotificationHook> response
* = metricsAdvisorAdminClient.getHookWithResponse(emailHookId, Context.NONE);
* EmailNotificationHook emailHook = (EmailNotificationHook) response.getValue();
* List<String> emailsToUpdate = new ArrayList<>(emailHook.getEmailsToAlert());
* emailsToUpdate.remove("alertme@alertme.com");
* emailsToUpdate.add("alertme2@alertme.com");
* emailsToUpdate.add("alertme3@alertme.com");
* emailHook.setEmailsToAlert(emailsToUpdate);
* Response<NotificationHook> updateResponse
* = metricsAdvisorAdminClient.updateHookWithResponse(emailHook, Context.NONE);
* EmailNotificationHook updatedEmailHook = (EmailNotificationHook) updateResponse.getValue();
* System.out.printf("Email Hook Id: %s%n", updatedEmailHook.getId());
* System.out.printf("Email Hook Name: %s%n", updatedEmailHook.getName());
* System.out.printf("Email Hook Description: %s%n", updatedEmailHook.getDescription());
* System.out.printf("Email Hook External Link: %s%n", updatedEmailHook.getExternalLink());
* System.out.printf("Email Hook Emails: %s%n", String.join(",",
* updatedEmailHook.getEmailsToAlert()));
*
*
*
* @param notificationHook The notificationHook to update.
* @param context Additional context that is passed through the Http pipeline during the service call.
* @return A {@link Response} containing the updated {@link NotificationHook}.
* @throws NullPointerException If {@code notificationHook.id} is null.
* @throws IllegalArgumentException If {@code notificationHook.Id} does not conform to the UUID format
* specification.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response updateHookWithResponse(NotificationHook notificationHook, Context context) {
return updateHookWithResponseSync(notificationHook, context);
}
Response updateHookWithResponseSync(NotificationHook notificationHook, Context context) {
Objects.requireNonNull(notificationHook, "'notificationHook' cannot be null.");
Objects.requireNonNull(notificationHook.getId(), "'notificationHook.id' cannot be null.");
Response response = service.updateHookWithResponse(UUID.fromString(notificationHook.getId()),
HookTransforms.toInnerForUpdate(logger, notificationHook), context);
Response hookResponse = getHookWithResponse(notificationHook.getId(), context);
return new ResponseBase(response.getRequest(), response.getStatusCode(),
response.getHeaders(), hookResponse.getValue(), null);
}
/**
* Delete a hook.
*
* Code sample
*
*
* final String emailHookId = "f00853f1-6627-447f-bacf-8dccf2e86fed";
* metricsAdvisorAdminClient.deleteHook(emailHookId);
*
*
*
* @param hookId The hook unique id.
*
* @throws NullPointerException thrown if the {@code hookId} is null.
* @throws IllegalArgumentException If {@code hookId} does not conform to the UUID format specification.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void deleteHook(String hookId) {
deleteHookWithResponse(hookId, Context.NONE);
}
/**
* Delete a hook.
*
* Code sample
*
*
* final String emailHookId = "f00853f1-6627-447f-bacf-8dccf2e86fed";
* Response<Void> response
* = metricsAdvisorAdminClient.deleteHookWithResponse(emailHookId, Context.NONE);
* System.out.printf("Response status code: %d%n", response.getStatusCode());
*
*
*
* @param hookId The hook unique id.
* @param context Additional context that is passed through the Http pipeline during the service call.
* @return A {@link Response}.
* @throws NullPointerException thrown if the {@code hookId} is null.
* @throws IllegalArgumentException If {@code hookId} does not conform to the UUID format specification.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response deleteHookWithResponse(String hookId, Context context) {
return deleteHookWithResponseSync(hookId, context);
}
Response deleteHookWithResponseSync(String hookId, Context context) {
Objects.requireNonNull(hookId, "hookId is required.");
return service.deleteHookWithResponse(UUID.fromString(hookId), context);
}
/**
* List information of hooks on the metrics advisor account.
*
* Code sample
*
*
* PagedIterable<NotificationHook> hooks = metricsAdvisorAdminClient.listHooks();
* for (NotificationHook notificationHook : hooks) {
* if (notificationHook instanceof EmailNotificationHook) {
* EmailNotificationHook emailHook = (EmailNotificationHook) notificationHook;
* System.out.printf("Email Hook Id: %s%n", emailHook.getId());
* System.out.printf("Email Hook Name: %s%n", emailHook.getName());
* System.out.printf("Email Hook Description: %s%n", emailHook.getDescription());
* System.out.printf("Email Hook External Link: %s%n", emailHook.getExternalLink());
* System.out.printf("Email Hook Emails: %s%n", String.join(",", emailHook.getEmailsToAlert()));
* } else if (notificationHook instanceof WebNotificationHook) {
* WebNotificationHook webHook = (WebNotificationHook) notificationHook;
* System.out.printf("Web Hook Id: %s%n", webHook.getId());
* System.out.printf("Web Hook Name: %s%n", webHook.getName());
* System.out.printf("Web Hook Description: %s%n", webHook.getDescription());
* System.out.printf("Web Hook External Link: %s%n", webHook.getExternalLink());
* System.out.printf("Web Hook Endpoint: %s%n", webHook.getEndpoint());
* System.out.printf("Web Hook Headers: %s%n", webHook.getHttpHeaders());
* }
* }
*
*
*
* @return A {@link PagedIterable} containing information of all the {@link NotificationHook} in the account.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listHooks() {
return listHooks(new ListHookOptions(), Context.NONE);
}
/**
* List information of hooks.
*
* Code sample
*
*
* ListHookOptions options = new ListHookOptions()
* .setSkip(100)
* .setMaxPageSize(20);
* PagedIterable<NotificationHook> hooks = metricsAdvisorAdminClient.listHooks(options, Context.NONE);
* Stream<PagedResponse<NotificationHook>> hooksPageStream = hooks.streamByPage();
* int[] pageCount = new int[1];
* hooksPageStream.forEach(hookPage -> {
* System.out.printf("Page: %d%n", pageCount[0]++);
* for (NotificationHook notificationHook : hookPage.getElements()) {
* if (notificationHook instanceof EmailNotificationHook) {
* EmailNotificationHook emailHook = (EmailNotificationHook) notificationHook;
* System.out.printf("Email Hook Id: %s%n", emailHook.getId());
* System.out.printf("Email Hook Name: %s%n", emailHook.getName());
* System.out.printf("Email Hook Description: %s%n", emailHook.getDescription());
* System.out.printf("Email Hook External Link: %s%n", emailHook.getExternalLink());
* System.out.printf("Email Hook Emails: %s%n", String.join(",",
* emailHook.getEmailsToAlert()));
* System.out.printf("Email Hook Admins: %s%n", String.join(",", emailHook.getAdmins()));
* } else if (notificationHook instanceof WebNotificationHook) {
* WebNotificationHook webHook = (WebNotificationHook) notificationHook;
* System.out.printf("Web Hook Id: %s%n", webHook.getId());
* System.out.printf("Web Hook Name: %s%n", webHook.getName());
* System.out.printf("Web Hook Description: %s%n", webHook.getDescription());
* System.out.printf("Web Hook External Link: %s%n", webHook.getExternalLink());
* System.out.printf("Web Hook Endpoint: %s%n", webHook.getEndpoint());
* System.out.printf("Web Hook Headers: %s%n", webHook.getHttpHeaders());
* System.out.printf("Web Hook Admins: %s%n", String.join(",", webHook.getAdmins()));
* }
* }
* });
*
*
*
* @param options The additional parameters.
* @param context Additional context that is passed through the Http pipeline during the service call.
* @return A {@link PagedIterable} containing information of the {@link NotificationHook} resources.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listHooks(ListHookOptions options, Context context) {
return listHooksSync(options, context);
}
PagedIterable listHooksSync(ListHookOptions options, Context context) {
return new PagedIterable<>(() -> listHooksSinglePageSync(options, context),
continuationToken -> listHooksNextPageSync(continuationToken, context));
}
private PagedResponse listHooksSinglePageSync(ListHookOptions options, Context context) {
PagedResponse response
= service.listHooksSinglePage(options != null ? options.getHookNameFilter() : null,
options != null ? options.getSkip() : null, options != null ? options.getMaxPageSize() : null, context);
return HookTransforms.fromInnerPagedResponse(logger, response);
}
private PagedResponse listHooksNextPageSync(String nextPageLink, Context context) {
if (CoreUtils.isNullOrEmpty(nextPageLink)) {
return null;
}
PagedResponse response = service.listHooksNextSinglePage(nextPageLink, context);
return HookTransforms.fromInnerPagedResponse(logger, response);
}
/**
* Create a configuration to trigger alert when anomalies are detected.
*
* Code sample
*
*
* String detectionConfigurationId1 = "9ol48er30-6e6e-4391-b78f-b00dfee1e6f5";
* String detectionConfigurationId2 = "3e58er30-6e6e-4391-b78f-b00dfee1e6f5";
* String hookId1 = "5f48er30-6e6e-4391-b78f-b00dfee1e6f5";
* String hookId2 = "8i48er30-6e6e-4391-b78f-b00dfee1e6f5";
*
* final AnomalyAlertConfiguration anomalyAlertConfiguration
* = metricsAdvisorAdminClient.createAlertConfig(
* new AnomalyAlertConfiguration("My AnomalyAlert config name")
* .setDescription("alert config description")
* .setMetricAlertConfigurations(Arrays.asList(
* new MetricAlertConfiguration(detectionConfigurationId1,
* MetricAnomalyAlertScope.forWholeSeries()),
* new MetricAlertConfiguration(detectionConfigurationId2,
* MetricAnomalyAlertScope.forWholeSeries())
* .setAlertConditions(new MetricAnomalyAlertConditions()
* .setSeverityRangeCondition(new SeverityCondition(AnomalySeverity.HIGH,
* AnomalySeverity.HIGH)))))
* .setCrossMetricsOperator(MetricAlertConfigurationsOperator.AND)
* .setHookIdsToAlert(Arrays.asList(hookId1, hookId2)));
*
* System.out.printf("DataPoint Anomaly alert configuration Id: %s%n",
* anomalyAlertConfiguration.getId());
* System.out.printf("DataPoint Anomaly alert configuration description: %s%n",
* anomalyAlertConfiguration.getDescription());
* System.out.printf("DataPoint Anomaly alert configuration hook ids: %s%n",
* anomalyAlertConfiguration.getHookIdsToAlert());
* System.out.printf("DataPoint Anomaly alert configuration cross metrics operator: %s%n",
* anomalyAlertConfiguration.getCrossMetricsOperator().toString());
*
*
*
* @param alertConfiguration The anomaly alerting configuration.
*
* @return The {@link AnomalyAlertConfiguration} that was created.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public AnomalyAlertConfiguration createAlertConfig(AnomalyAlertConfiguration alertConfiguration) {
return createAlertConfigWithResponse(alertConfiguration, Context.NONE).getValue();
}
/**
* Create a configuration to trigger alert when anomalies are detected.
*
* Code sample
*
*
*
* String detectionConfigurationId1 = "9ol48er30-6e6e-4391-b78f-b00dfee1e6f5";
* String detectionConfigurationId2 = "3e58er30-6e6e-4391-b78f-b00dfee1e6f5";
* String hookId1 = "5f48er30-6e6e-4391-b78f-b00dfee1e6f5";
* String hookId2 = "8i48er30-6e6e-4391-b78f-b00dfee1e6f5";
*
* final Response<AnomalyAlertConfiguration> alertConfigurationResponse
* = metricsAdvisorAdminClient.createAlertConfigWithResponse(
* new AnomalyAlertConfiguration("My AnomalyAlert config name")
* .setDescription("alert config description")
* .setMetricAlertConfigurations(Arrays.asList(
* new MetricAlertConfiguration(detectionConfigurationId1,
* MetricAnomalyAlertScope.forWholeSeries()),
* new MetricAlertConfiguration(detectionConfigurationId2,
* MetricAnomalyAlertScope.forWholeSeries())
* .setAlertConditions(new MetricAnomalyAlertConditions()
* .setSeverityRangeCondition(new SeverityCondition(AnomalySeverity.HIGH,
* AnomalySeverity.HIGH)))))
* .setCrossMetricsOperator(MetricAlertConfigurationsOperator.AND)
* .setHookIdsToAlert(Arrays.asList(hookId1, hookId2)), Context.NONE);
*
* System.out.printf("DataPoint Anomaly alert creation operation status: %s%n",
* alertConfigurationResponse.getStatusCode());
* final AnomalyAlertConfiguration anomalyAlertConfiguration = alertConfigurationResponse.getValue();
* System.out.printf("DataPoint Anomaly alert configuration Id: %s%n", anomalyAlertConfiguration.getId());
* System.out.printf("DataPoint Anomaly alert configuration description: %s%n",
* anomalyAlertConfiguration.getDescription());
* System.out.printf("DataPoint Anomaly alert configuration hook ids: %s%n",
* anomalyAlertConfiguration.getHookIdsToAlert());
* System.out.printf("DataPoint Anomaly alert configuration cross metrics operator: %s%n",
* anomalyAlertConfiguration.getCrossMetricsOperator().toString());
*
*
*
* @param alertConfiguration The anomaly alerting configuration.
* @param context Additional context that is passed through the Http pipeline during the service call.
*
* @return A {@link Response} containing the created {@link AnomalyAlertConfiguration}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response
createAlertConfigWithResponse(AnomalyAlertConfiguration alertConfiguration, Context context) {
return createAlertConfigWithResponseSync(alertConfiguration, context);
}
Response createAlertConfigWithResponseSync(AnomalyAlertConfiguration alertConfiguration,
Context context) {
Objects.requireNonNull(alertConfiguration, "'alertConfiguration' is required.");
if (CoreUtils.isNullOrEmpty(alertConfiguration.getMetricAlertConfigurations())) {
throw logger.logExceptionAsError(
new NullPointerException("'alertConfiguration.metricAnomalyAlertConfigurations' is required"));
}
if (alertConfiguration.getCrossMetricsOperator() == null
&& alertConfiguration.getMetricAlertConfigurations().size() > 1) {
throw logger.logExceptionAsError(new IllegalArgumentException("crossMetricsOperator is required"
+ " when there are more than one metric level alert configuration."));
}
final AnomalyAlertingConfiguration innerAlertConfiguration
= AlertConfigurationTransforms.toInnerForCreate(alertConfiguration);
CreateAnomalyAlertingConfigurationResponse response
= service.createAnomalyAlertingConfigurationWithResponse(innerAlertConfiguration, context);
final String configurationId = parseOperationId(response.getDeserializedHeaders().getLocation());
Response getResponse = getAlertConfigWithResponse(configurationId, context);
return new ResponseBase(response.getRequest(), response.getStatusCode(),
response.getHeaders(), getResponse.getValue(), null);
}
/**
* Get the anomaly alert configuration identified by {@code alertConfigurationId}.
*
* Code sample
*
*
* String alertConfigId = "1p0f8er30-6e6e-4391-b78f-bpfdfee1e6f5";
*
* AnomalyAlertConfiguration anomalyAlertConfiguration
* = metricsAdvisorAdminClient.getAlertConfig(alertConfigId);
* System.out.printf("DataPoint Anomaly alert configuration Id: %s%n",
* anomalyAlertConfiguration.getId());
* System.out.printf("DataPoint Anomaly alert configuration description: %s%n",
* anomalyAlertConfiguration.getDescription());
* System.out.printf("DataPoint Anomaly alert configuration hook ids: %s%n",
* anomalyAlertConfiguration.getHookIdsToAlert());
* System.out.printf("DataPoint Anomaly alert configuration cross metrics operator: %s%n",
* anomalyAlertConfiguration.getCrossMetricsOperator().toString());
*
*
*
* @param alertConfigurationId The anomaly alert configuration id.
*
* @return The {@link AnomalyAlertConfiguration} identified by the given id.
* @throws NullPointerException thrown if the {@code alertConfigurationId} is null.
* @throws IllegalArgumentException If {@code alertConfigurationId} does not conform to the
* UUID format specification.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public AnomalyAlertConfiguration getAlertConfig(String alertConfigurationId) {
return getAlertConfigWithResponse(alertConfigurationId, Context.NONE).getValue();
}
/**
* Get the anomaly alert configuration identified by {@code alertConfigurationId}.
*
* Code sample
*
*
* String alertConfigId = "1p0f8er30-6e6e-4391-b78f-bpfdfee1e6f5";
*
* Response<AnomalyAlertConfiguration> alertConfigurationResponse
* = metricsAdvisorAdminClient.getAlertConfigWithResponse(alertConfigId, Context.NONE);
*
* System.out.printf("DataPoint Anomaly alert creation operation status: %s%n",
* alertConfigurationResponse.getStatusCode());
* final AnomalyAlertConfiguration anomalyAlertConfiguration = alertConfigurationResponse.getValue();
* System.out.printf("DataPoint Anomaly alert configuration Id: %s%n", anomalyAlertConfiguration.getId());
* System.out.printf("DataPoint Anomaly alert configuration description: %s%n",
* anomalyAlertConfiguration.getDescription());
* System.out.printf("DataPoint Anomaly alert configuration hook ids: %s%n",
* anomalyAlertConfiguration.getHookIdsToAlert());
* System.out.printf("DataPoint Anomaly alert configuration cross metrics operator: %s%n",
* anomalyAlertConfiguration.getCrossMetricsOperator().toString());
*
*
*
* @param alertConfigurationId The anomaly alert configuration id.
* @param context Additional context that is passed through the Http pipeline during the service call.
*
* @return A {@link Response response} containing the {@link AnomalyAlertConfiguration} identified by the given id.
* @throws IllegalArgumentException If {@code alertConfigurationId} does not conform to the
* UUID format specification.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getAlertConfigWithResponse(String alertConfigurationId,
Context context) {
return getAlertConfigWithResponseSync(alertConfigurationId, context);
}
Response getAlertConfigWithResponseSync(String alertConfigurationId, Context context) {
Objects.requireNonNull(alertConfigurationId, "'alertConfigurationId' is required.");
Response response
= service.getAnomalyAlertingConfigurationWithResponse(UUID.fromString(alertConfigurationId), context);
return new ResponseBase(response.getRequest(), response.getStatusCode(),
response.getHeaders(), AlertConfigurationTransforms.fromInner(response.getValue()), null);
}
/**
* Update anomaly alert configuration.
*
* Code sample
*
*
*
* String alertConfigId = "1p0f8er30-6e6e-4391-b78f-bpfdfee1e6f5";
* String additionalHookId = "2gh8er30-6e6e-4391-b78f-bpfdfee1e6f5";
*
* AnomalyAlertConfiguration existingAnomalyConfig
* = metricsAdvisorAdminClient.getAlertConfig(alertConfigId);
* List<String> hookIds = new ArrayList<>(existingAnomalyConfig.getHookIdsToAlert());
* hookIds.add(additionalHookId);
* final AnomalyAlertConfiguration updatedAnomalyAlertConfiguration
* = metricsAdvisorAdminClient.updateAlertConfig(
* existingAnomalyConfig
* .setHookIdsToAlert(hookIds)
* .setDescription("updated to add more hook ids")
* );
*
* System.out.printf("Updated anomaly alert configuration Id: %s%n", updatedAnomalyAlertConfiguration.getId());
* System.out.printf("Updated anomaly alert configuration description: %s%n",
* updatedAnomalyAlertConfiguration.getDescription());
* System.out.printf("Updated anomaly alert configuration hook ids: %s%n",
* updatedAnomalyAlertConfiguration.getHookIdsToAlert());
*
*
*
* @param alertConfiguration The anomaly alert configuration to update.
*
* @return The {@link AnomalyAlertConfiguration} that was updated.
* @throws NullPointerException thrown if {@code alertConfiguration} or
* {@code alertConfiguration.metricAnomalyAlertConfigurations} is null or empty.
* @throws IllegalArgumentException If {@code alertConfigurationId} does not conform to the
* UUID format specification.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public AnomalyAlertConfiguration updateAlertConfig(AnomalyAlertConfiguration alertConfiguration) {
return updateAlertConfigWithResponse(alertConfiguration, Context.NONE).getValue();
}
/**
* Update anomaly alert configuration.
*
* Code sample
*
*
*
* String alertConfigId = "1p0f8er30-6e6e-4391-b78f-bpfdfee1e6f5";
* String additionalHookId = "2gh8er30-6e6e-4391-b78f-bpfdfee1e6f5";
*
* AnomalyAlertConfiguration existingAnomalyConfig
* = metricsAdvisorAdminClient.getAlertConfig(alertConfigId);
* List<String> hookIds = new ArrayList<>(existingAnomalyConfig.getHookIdsToAlert());
* hookIds.add(additionalHookId);
* final Response<AnomalyAlertConfiguration> alertConfigurationResponse
* = metricsAdvisorAdminClient.updateAlertConfigWithResponse(
* existingAnomalyConfig
* .setHookIdsToAlert(hookIds)
* .setDescription("updated to add more hook ids"), Context.NONE);
*
* System.out.printf("Update anomaly alert operation status: %s%n", alertConfigurationResponse.getStatusCode());
* final AnomalyAlertConfiguration updatedAnomalyAlertConfiguration = alertConfigurationResponse.getValue();
* System.out.printf("Updated anomaly alert configuration Id: %s%n", updatedAnomalyAlertConfiguration.getId());
* System.out.printf("Updated anomaly alert configuration description: %s%n",
* updatedAnomalyAlertConfiguration.getDescription());
* System.out.printf("Updated anomaly alert configuration hook ids: %sf%n",
* updatedAnomalyAlertConfiguration.getHookIdsToAlert());
*
*
*
* @param alertConfiguration The anomaly alert configuration to update.
* @param context Additional context that is passed through the Http pipeline during the service call.
*
* @return A {@link Response} containing the {@link AnomalyAlertConfiguration} that was updated.
* @throws NullPointerException thrown if {@code alertConfiguration} or
* {@code alertConfiguration.metricAnomalyAlertConfigurations} is null or empty.
* @throws IllegalArgumentException If {@code alertConfigurationId} does not conform to the
* UUID format specification.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response
updateAlertConfigWithResponse(AnomalyAlertConfiguration alertConfiguration, Context context) {
return updateAlertConfigWithResponseSync(alertConfiguration, context);
}
Response updateAlertConfigWithResponseSync(AnomalyAlertConfiguration alertConfiguration,
Context context) {
Objects.requireNonNull(alertConfiguration, "'alertConfiguration' is required");
if (CoreUtils.isNullOrEmpty(alertConfiguration.getMetricAlertConfigurations())) {
throw logger.logExceptionAsError(new NullPointerException(
"'alertConfiguration.metricAnomalyAlertConfigurations' is required and cannot be empty"));
}
final AnomalyAlertingConfigurationPatch innerAlertConfiguration
= AlertConfigurationTransforms.toInnerForUpdate(alertConfiguration);
Response response = service.updateAnomalyAlertingConfigurationWithResponse(
UUID.fromString(alertConfiguration.getId()), innerAlertConfiguration, context);
Response getResponse
= getAlertConfigWithResponse(alertConfiguration.getId(), context);
return new ResponseBase(response.getRequest(), response.getStatusCode(),
response.getHeaders(), getResponse.getValue(), null);
}
/**
* Deletes the anomaly alert configuration identified by {@code alertConfigurationId}.
*
* Code sample
*
*
* String alertConfigId = "1p0f8er30-6e6e-4391-b78f-bpfdfee1e6f5";
* metricsAdvisorAdminClient.deleteAlertConfig(alertConfigId);
*
*
*
* @param alertConfigurationId The anomaly alert configuration id.
* @throws NullPointerException thrown if the {@code alertConfigurationId} is null.
* @throws IllegalArgumentException If {@code alertConfigurationId} does not conform to the
* UUID format specification.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void deleteAlertConfig(String alertConfigurationId) {
deleteAlertConfigWithResponse(alertConfigurationId, Context.NONE);
}
/**
* Deletes the anomaly alert configuration identified by {@code alertConfigurationId}.
*
* Code sample
*
*
* String alertConfigId = "1p0f8er30-6e6e-4391-b78f-bpfdfee1e6f5";
* final Response<Void> response =
* metricsAdvisorAdminClient.deleteAlertConfigWithResponse(alertConfigId, Context.NONE);
*
* System.out.printf("DataPoint Anomaly alert config delete operation status : %s%n", response.getStatusCode());
*
*
*
* @param alertConfigurationId The anomaly alert configuration id.
* @param context Additional context that is passed through the Http pipeline during the service call.
*
* @return A response containing status code and headers returned after the operation.
* @throws NullPointerException thrown if the {@code alertConfigurationId} is null.
* @throws IllegalArgumentException If {@code alertConfigurationId} does not conform to the
* UUID format specification.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response deleteAlertConfigWithResponse(String alertConfigurationId, Context context) {
return deleteAlertConfigWithResponseSync(alertConfigurationId, context);
}
Response deleteAlertConfigWithResponseSync(String alertConfigurationId, Context context) {
Objects.requireNonNull(alertConfigurationId, "'alertConfigurationId' is required.");
return service.deleteAnomalyAlertingConfigurationWithResponse(UUID.fromString(alertConfigurationId), context);
}
/**
* Fetch the anomaly alert configurations associated with a detection configuration.
*
* Code sample
*
*
* String detectionConfigId = "3rt98er30-6e6e-4391-b78f-bpfdfee1e6f5";
* metricsAdvisorAdminClient.listAlertConfigs(detectionConfigId, new ListAnomalyAlertConfigsOptions())
* .forEach(anomalyAlertConfiguration -> {
* System.out.printf("DataPoint Anomaly alert configuration Id: %s%n", anomalyAlertConfiguration.getId());
* System.out.printf("DataPoint Anomaly alert configuration description: %s%n",
* anomalyAlertConfiguration.getDescription());
* System.out.printf("DataPoint Anomaly alert configuration hook ids: %s%n",
* anomalyAlertConfiguration.getHookIdsToAlert());
* System.out.printf("DataPoint Anomaly alert configuration cross metrics operator: %s%n",
* anomalyAlertConfiguration.getCrossMetricsOperator().toString());
* });
*
*
*
* @param detectionConfigurationId The id of the detection configuration.
* @param options th e additional configurable options to specify when querying the result.
*
* @return A {@link PagedIterable} containing information of all the
* {@link AnomalyAlertConfiguration anomaly alert configurations} for the specified detection configuration.
* @throws NullPointerException thrown if the {@code detectionConfigurationId} is null.
* @throws IllegalArgumentException If {@code detectionConfigurationId} does not conform to the
* UUID format specification.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listAlertConfigs(String detectionConfigurationId,
ListAnomalyAlertConfigsOptions options) {
return listAlertConfigs(detectionConfigurationId, options, Context.NONE);
}
/**
* Fetch the anomaly alert configurations associated with a detection configuration.
*
* Code sample
*
*
* String detectionConfigId = "3rt98er30-6e6e-4391-b78f-bpfdfee1e6f5";
* metricsAdvisorAdminClient.listAlertConfigs(detectionConfigId,
* new ListAnomalyAlertConfigsOptions(), Context.NONE)
* .forEach(anomalyAlertConfiguration -> {
* System.out.printf("DataPoint Anomaly alert configuration Id: %s%n", anomalyAlertConfiguration.getId());
* System.out.printf("DataPoint Anomaly alert configuration description: %s%n",
* anomalyAlertConfiguration.getDescription());
* System.out.printf("DataPoint Anomaly alert configuration hook ids: %s%n",
* anomalyAlertConfiguration.getHookIdsToAlert());
* System.out.printf("DataPoint Anomaly alert configuration cross metrics operator: %s%n",
* anomalyAlertConfiguration.getCrossMetricsOperator().toString());
* });
*
*
*
* @param detectionConfigurationId The id of the detection configuration.
* @param options th e additional configurable options to specify when querying the result.
* @param context Additional context that is passed through the Http pipeline during the service call.
*
* @return A {@link PagedIterable} containing information of all the
* {@link AnomalyAlertConfiguration anomaly alert configurations} for the specified detection configuration.
* @throws NullPointerException thrown if the {@code detectionConfigurationId} is null.
* @throws IllegalArgumentException If {@code detectionConfigurationId} does not conform to the
* UUID format specification.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listAlertConfigs(String detectionConfigurationId,
ListAnomalyAlertConfigsOptions options, Context context) {
return listAlertConfigsSync(detectionConfigurationId, options, context);
}
PagedIterable listAlertConfigsSync(String detectionConfigurationId,
ListAnomalyAlertConfigsOptions options, Context context) {
return new PagedIterable<>(
() -> listAnomalyAlertConfigsSinglePageSync(detectionConfigurationId, options, context),
continuationToken -> listAnomalyAlertConfigsNextPageSync(continuationToken, context));
}
private PagedResponse listAnomalyAlertConfigsSinglePageSync(
String detectionConfigurationId, ListAnomalyAlertConfigsOptions options, Context context) {
Objects.requireNonNull(detectionConfigurationId, "'detectionConfigurationId' is required.");
if (options == null) {
options = new ListAnomalyAlertConfigsOptions();
}
PagedResponse response
= service.getAnomalyAlertingConfigurationsByAnomalyDetectionConfigurationSinglePage(
UUID.fromString(detectionConfigurationId), options.getSkip(), options.getMaxPageSize(), context);
return AlertConfigurationTransforms.fromInnerPagedResponse(response);
}
private PagedResponse listAnomalyAlertConfigsNextPageSync(String nextPageLink,
Context context) {
if (CoreUtils.isNullOrEmpty(nextPageLink)) {
return null;
}
PagedResponse response = service
.getAnomalyAlertingConfigurationsByAnomalyDetectionConfigurationNextSinglePage(nextPageLink, context);
return AlertConfigurationTransforms.fromInnerPagedResponse(response);
}
/**
* Create a data source credential entity.
*
* Code sample
*
*
* DataSourceCredentialEntity datasourceCredential;
* final String name = "sample_name" + UUID.randomUUID();
* final String cId = "f45668b2-bffa-11eb-8529-0246ac130003";
* final String tId = "67890ded-5e07-4e52-b225-4ae8f905afb5";
* final String mockSecret = "890hy69-5e07-4e52-b225-4ae8f905afb5";
*
* datasourceCredential = new DataSourceServicePrincipalInKeyVault()
* .setName(name)
* .setKeyVaultForDataSourceSecrets("kv", cId, mockSecret)
* .setTenantId(tId)
* .setSecretNameForDataSourceClientId("DSClientID_1")
* .setSecretNameForDataSourceClientSecret("DSClientSer_1");
*
* DataSourceCredentialEntity credentialEntity =
* metricsAdvisorAdminClient.createDataSourceCredential(datasourceCredential);
* if (credentialEntity instanceof DataSourceServicePrincipalInKeyVault) {
* DataSourceServicePrincipalInKeyVault actualCredentialSPInKV
* = (DataSourceServicePrincipalInKeyVault) credentialEntity;
* System.out
* .printf("Actual credential entity key vault endpoint: %s%n",
* actualCredentialSPInKV.getKeyVaultEndpoint());
* System.out.printf("Actual credential entity key vault client Id: %s%n",
* actualCredentialSPInKV.getKeyVaultClientId());
* System.out.printf("Actual credential entity key vault secret name for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientId());
* System.out.printf("Actual credential entity key vault secret for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientSecret());
* }
*
*
*
* @param dataSourceCredential The credential entity.
* @return The created {@link DataSourceCredentialEntity}.
* @throws NullPointerException thrown if the {@code credentialEntity} is null
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public DataSourceCredentialEntity createDataSourceCredential(DataSourceCredentialEntity dataSourceCredential) {
return createDataSourceCredentialWithResponse(dataSourceCredential, Context.NONE).getValue();
}
/**
* Create a data source credential entity with REST response.
*
* Code sample
*
*
* DataSourceCredentialEntity datasourceCredential;
* final String name = "sample_name" + UUID.randomUUID();
* final String cId = "f45668b2-bffa-11eb-8529-0246ac130003";
* final String tId = "67890ded-5e07-4e52-b225-4ae8f905afb5";
* final String mockSecret = "890hy69-5e07-4e52-b225-4ae8f905afb5";
*
* datasourceCredential = new DataSourceServicePrincipalInKeyVault()
* .setName(name)
* .setKeyVaultForDataSourceSecrets("kv", cId, mockSecret)
* .setTenantId(tId)
* .setSecretNameForDataSourceClientId("DSClientID_1")
* .setSecretNameForDataSourceClientSecret("DSClientSer_1");
*
* Response<DataSourceCredentialEntity> credentialEntityWithResponse =
* metricsAdvisorAdminClient.createDataSourceCredentialWithResponse(datasourceCredential, Context.NONE);
*
* System.out.printf("Credential Entity creation operation status: %s%n",
* credentialEntityWithResponse.getStatusCode());
* if (credentialEntityWithResponse.getValue() instanceof DataSourceServicePrincipalInKeyVault) {
* DataSourceServicePrincipalInKeyVault actualCredentialSPInKV
* = (DataSourceServicePrincipalInKeyVault) credentialEntityWithResponse.getValue();
* System.out
* .printf("Actual credential entity key vault endpoint: %s%n",
* actualCredentialSPInKV.getKeyVaultEndpoint());
* System.out.printf("Actual credential entity key vault client Id: %s%n",
* actualCredentialSPInKV.getKeyVaultClientId());
* System.out.printf("Actual credential entity key vault secret name for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientId());
* System.out.printf("Actual credential entity key vault secret for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientSecret());
* }
*
*
*
* @param dataSourceCredential The credential entity.
* @param context Additional context that is passed through the HTTP pipeline during the service call.
*
* @return A {@link Response} containing the created {@link DataSourceCredentialEntity}.
* @throws NullPointerException thrown if the {@code credentialEntity} is null
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response
createDataSourceCredentialWithResponse(DataSourceCredentialEntity dataSourceCredential, Context context) {
return createDataSourceCredentialWithResponseSync(dataSourceCredential, context);
}
Response
createDataSourceCredentialWithResponseSync(DataSourceCredentialEntity dataSourceCredential, Context context) {
Objects.requireNonNull(dataSourceCredential, "dataSourceCredential is required");
final DataSourceCredential innerDataSourceCredential
= DataSourceCredentialEntityTransforms.toInnerForCreate(dataSourceCredential);
CreateCredentialResponse response = service.createCredentialWithResponse(innerDataSourceCredential, context);
final String credentialId = Utility.parseOperationId(response.getDeserializedHeaders().getLocation());
Response configurationResponse
= this.getDataSourceCredentialWithResponse(credentialId, context);
return new ResponseBase(response.getRequest(), response.getStatusCode(),
response.getHeaders(), configurationResponse.getValue(), null);
}
/**
* Get a data source credential entity by its id.
*
* Code sample
*
*
* final String datasourceCredentialId = "f45668b2-bffa-11eb-8529-0246ac130003";
*
* DataSourceCredentialEntity credentialEntity =
* metricsAdvisorAdminClient.getDataSourceCredential(datasourceCredentialId);
* if (credentialEntity instanceof DataSourceServicePrincipalInKeyVault) {
* DataSourceServicePrincipalInKeyVault actualCredentialSPInKV
* = (DataSourceServicePrincipalInKeyVault) credentialEntity;
* System.out
* .printf("Actual credential entity key vault endpoint: %s%n",
* actualCredentialSPInKV.getKeyVaultEndpoint());
* System.out.printf("Actual credential entity key vault client Id: %s%n",
* actualCredentialSPInKV.getKeyVaultClientId());
* System.out.printf("Actual credential entity key vault secret name for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientId());
* System.out.printf("Actual credential entity key vault secret for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientSecret());
* }
*
*
*
* @param credentialId The data source credential entity unique id.
*
* @return The data source credential entity for the provided id.
* @throws IllegalArgumentException If {@code credentialId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code credentialId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public DataSourceCredentialEntity getDataSourceCredential(String credentialId) {
return getDataSourceCredentialWithResponse(credentialId, Context.NONE).getValue();
}
/**
* Get a data source credential entity by its id with REST response.
*
* Code sample
*
*
* final String datasourceCredentialId = "f45668b2-bffa-11eb-8529-0246ac130003";
*
* Response<DataSourceCredentialEntity> credentialEntityWithResponse =
* metricsAdvisorAdminClient.getDataSourceCredentialWithResponse(datasourceCredentialId, Context.NONE);
* System.out.printf("Credential Entity creation operation status: %s%n",
* credentialEntityWithResponse.getStatusCode());
* if (credentialEntityWithResponse.getValue() instanceof DataSourceServicePrincipalInKeyVault) {
* DataSourceServicePrincipalInKeyVault actualCredentialSPInKV
* = (DataSourceServicePrincipalInKeyVault) credentialEntityWithResponse.getValue();
* System.out
* .printf("Actual credential entity key vault endpoint: %s%n",
* actualCredentialSPInKV.getKeyVaultEndpoint());
* System.out.printf("Actual credential entity key vault client Id: %s%n",
* actualCredentialSPInKV.getKeyVaultClientId());
* System.out.printf("Actual credential entity key vault secret name for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientId());
* System.out.printf("Actual credential entity key vault secret for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientSecret());
* }
*
*
*
* @param credentialId The data source credential entity unique id.
* @param context Additional context that is passed through the HTTP pipeline during the service call.
*
* @return The data feed for the provided id.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code dataFeedId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getDataSourceCredentialWithResponse(String credentialId,
Context context) {
return getDataSourceCredentialWithResponseSync(credentialId, context);
}
Response getDataSourceCredentialWithResponseSync(String credentialId, Context context) {
Objects.requireNonNull(credentialId, "'credentialId' cannot be null.");
Response response
= service.getCredentialWithResponse(UUID.fromString(credentialId), context);
return new SimpleResponse<>(response, DataSourceCredentialEntityTransforms.fromInner(response.getValue()));
}
/**
* Update a data source credential entity.
*
* Code sample
*
*
* final String datasourceCredentialId = "f45668b2-bffa-11eb-8529-0246ac130003";
* DataSourceCredentialEntity existingDatasourceCredential =
* metricsAdvisorAdminClient.getDataSourceCredential(datasourceCredentialId);
* DataSourceServicePrincipalInKeyVault actualCredentialSPInKV = null;
* if (existingDatasourceCredential instanceof DataSourceServicePrincipalInKeyVault) {
* actualCredentialSPInKV = (DataSourceServicePrincipalInKeyVault) existingDatasourceCredential;
* }
*
* DataSourceCredentialEntity credentialEntity =
* metricsAdvisorAdminClient.updateDataSourceCredential(
* actualCredentialSPInKV.setDescription("set updated description"));
*
* if (credentialEntity instanceof DataSourceServicePrincipalInKeyVault) {
* DataSourceServicePrincipalInKeyVault updatedCredentialSPInKV
* = (DataSourceServicePrincipalInKeyVault) credentialEntity;
* System.out.printf("Actual credential entity key vault endpoint: %s%n",
* updatedCredentialSPInKV.getKeyVaultEndpoint());
* System.out.printf("Actual credential entity key vault updated description: %s%n",
* updatedCredentialSPInKV.getDescription());
* }
*
*
*
* @param dataSourceCredential The credential entity.
*
* @return The updated {@link DataSourceCredentialEntity}.
* @throws NullPointerException thrown if the {@code credentialEntity} is null
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public DataSourceCredentialEntity updateDataSourceCredential(DataSourceCredentialEntity dataSourceCredential) {
return updateDataSourceCredentialWithResponse(dataSourceCredential, Context.NONE).getValue();
}
/**
* Update a data source credential entity.
*
* Code sample
*
*
* final String datasourceCredentialId = "f45668b2-bffa-11eb-8529-0246ac130003";
* DataSourceCredentialEntity existingDatasourceCredential =
* metricsAdvisorAdminClient.getDataSourceCredential(datasourceCredentialId);
* DataSourceServicePrincipalInKeyVault actualCredentialSPInKV = null;
* if (existingDatasourceCredential instanceof DataSourceServicePrincipalInKeyVault) {
* actualCredentialSPInKV = (DataSourceServicePrincipalInKeyVault) existingDatasourceCredential;
* }
* Response<DataSourceCredentialEntity> credentialEntityWithResponse =
* metricsAdvisorAdminClient.updateDataSourceCredentialWithResponse(
* actualCredentialSPInKV.setDescription("set updated description"), Context.NONE);
*
* System.out.printf("Credential Entity creation operation status: %s%n",
* credentialEntityWithResponse.getStatusCode());
* if (credentialEntityWithResponse.getValue() instanceof DataSourceServicePrincipalInKeyVault) {
* DataSourceServicePrincipalInKeyVault updatedCredentialSPInKV
* = (DataSourceServicePrincipalInKeyVault) credentialEntityWithResponse.getValue();
* System.out.printf("Actual credential entity key vault endpoint: %s%n",
* updatedCredentialSPInKV.getKeyVaultEndpoint());
* System.out.printf("Actual credential entity key vault updated description: %s%n",
* updatedCredentialSPInKV.getDescription());
* }
*
*
*
* @param dataSourceCredential The credential entity.
* @param context Additional context that is passed through the HTTP pipeline during the service call.
*
* @return A {@link Response} containing the updated {@link DataSourceCredentialEntity}.
* @throws NullPointerException thrown if the {@code credentialEntity} is null
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response
updateDataSourceCredentialWithResponse(DataSourceCredentialEntity dataSourceCredential, Context context) {
return updateDataSourceCredentialWithResponseSync(dataSourceCredential, context);
}
Response
updateDataSourceCredentialWithResponseSync(DataSourceCredentialEntity dataSourceCredential, Context context) {
Objects.requireNonNull(dataSourceCredential, "dataSourceCredential is required");
final DataSourceCredentialPatch innerDataSourceCredential
= DataSourceCredentialEntityTransforms.toInnerForUpdate(dataSourceCredential);
Response response = service.updateCredentialWithResponse(
UUID.fromString(dataSourceCredential.getId()), innerDataSourceCredential, context);
Response configurationResponse
= getDataSourceCredentialWithResponse(dataSourceCredential.getId(), context);
return new ResponseBase(response.getRequest(), response.getStatusCode(),
response.getHeaders(), configurationResponse.getValue(), null);
}
/**
* Delete a data source credential entity.
*
* Code sample
*
*
* final String datasourceCredentialId = "t00853f1-9080-447f-bacf-8dccf2e86f";
* metricsAdvisorAdminClient.deleteDataFeed(datasourceCredentialId);
*
*
*
* @param credentialId The data source credential entity unique id.
*
* @throws IllegalArgumentException If {@code credentialId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code credentialId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public void deleteDataSourceCredential(String credentialId) {
deleteDataSourceCredentialWithResponse(credentialId, Context.NONE);
}
/**
* Delete a data source credential entity with REST response.
*
* Code sample
*
*
* final String datasourceCredentialId = "eh0854f1-8927-447f-bacf-8dccf2e86fwe";
* Response<Void> response =
* metricsAdvisorAdminClient.deleteDataSourceCredentialWithResponse(datasourceCredentialId, Context.NONE);
* System.out.printf("Datasource credential delete operation status : %s%n", response.getStatusCode());
*
*
*
* @param credentialId The data source credential entity unique id.
* @param context Additional context that is passed through the HTTP pipeline during the service call.
*
* @return a REST Response.
* @throws IllegalArgumentException If {@code dataFeedId} does not conform to the UUID format specification.
* @throws NullPointerException thrown if the {@code dataFeedId} is null.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response deleteDataSourceCredentialWithResponse(String credentialId, Context context) {
return deleteDataSourceCredentialWithResponseSync(credentialId, context);
}
Response deleteDataSourceCredentialWithResponseSync(String credentialId, Context context) {
Objects.requireNonNull(credentialId, "'credentialId' is required.");
return service.deleteCredentialWithResponse(UUID.fromString(credentialId), context);
}
/**
* List information of all data source credential entities on the metrics advisor account.
*
* Code sample
*
*
* metricsAdvisorAdminClient.listDataSourceCredentials()
* .forEach(datasourceCredentialEntity -> {
* if (datasourceCredentialEntity instanceof DataSourceServicePrincipalInKeyVault) {
* DataSourceServicePrincipalInKeyVault actualCredentialSPInKV
* = (DataSourceServicePrincipalInKeyVault) datasourceCredentialEntity;
* System.out
* .printf("Actual credential entity key vault endpoint: %s%n",
* actualCredentialSPInKV.getKeyVaultEndpoint());
* System.out.printf("Actual credential entity key vault client Id: %s%n",
* actualCredentialSPInKV.getKeyVaultClientId());
* System.out.printf("Actual credential entity key vault secret name for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientId());
* System.out.printf("Actual credential entity key vault secret for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientSecret());
* }
* });
*
*
*
* @return A {@link PagedIterable} containing information of all the {@link DataSourceCredentialEntity}
* in the account.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listDataSourceCredentials() {
return listDataSourceCredentials(null, Context.NONE);
}
/**
* List information of all data source credential entities on the metrics advisor account.
*
* Code sample
*
*
* metricsAdvisorAdminClient.listDataSourceCredentials(
* new ListCredentialEntityOptions()
* .setMaxPageSize(3),
* Context.NONE)
* .forEach(datasourceCredentialEntity -> {
* if (datasourceCredentialEntity instanceof DataSourceServicePrincipalInKeyVault) {
* DataSourceServicePrincipalInKeyVault actualCredentialSPInKV
* = (DataSourceServicePrincipalInKeyVault) datasourceCredentialEntity;
* System.out
* .printf("Actual credential entity key vault endpoint: %s%n",
* actualCredentialSPInKV.getKeyVaultEndpoint());
* System.out.printf("Actual credential entity key vault client Id: %s%n",
* actualCredentialSPInKV.getKeyVaultClientId());
* System.out.printf("Actual credential entity key vault secret name for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientId());
* System.out.printf("Actual credential entity key vault secret for data source: %s%n",
* actualCredentialSPInKV.getSecretNameForDataSourceClientSecret());
* }
* });
*
*
*
* @param options The configurable {@link ListCredentialEntityOptions options} to pass for filtering the output
* result.
* @param context Additional context that is passed through the Http pipeline during the service call.
*
* @return A {@link PagedIterable} containing information of all the {@link DataSourceCredentialEntity}
* in the account.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listDataSourceCredentials(ListCredentialEntityOptions options,
Context context) {
return listDataSourceCredentialsSync(options, context);
}
PagedIterable listDataSourceCredentialsSync(ListCredentialEntityOptions options,
Context context) {
return new PagedIterable<>(() -> listCredentialEntitiesSinglePageSync(options, context),
continuationToken -> listCredentialEntitiesSNextPageSync(continuationToken, context));
}
private PagedResponse
listCredentialEntitiesSinglePageSync(ListCredentialEntityOptions options, Context context) {
options = options != null ? options : new ListCredentialEntityOptions();
PagedResponse res
= service.listCredentialsSinglePage(options.getSkip(), options.getMaxPageSize(), context);
return new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().stream().map(DataSourceCredentialEntityTransforms::fromInner).collect(Collectors.toList()),
res.getContinuationToken(), null);
}
private PagedResponse listCredentialEntitiesSNextPageSync(String nextPageLink,
Context context) {
if (CoreUtils.isNullOrEmpty(nextPageLink)) {
return null;
}
PagedResponse res = service.listCredentialsNextSinglePage(nextPageLink, context);
return new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
res.getValue().stream().map(DataSourceCredentialEntityTransforms::fromInner).collect(Collectors.toList()),
res.getContinuationToken(), null);
}
}