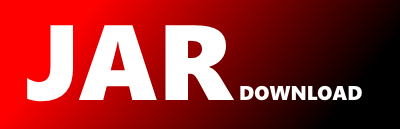
com.azure.ai.metricsadvisor.administration.models.AzureLogAnalyticsDataFeedSource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-ai-metricsadvisor Show documentation
Show all versions of azure-ai-metricsadvisor Show documentation
This package contains the Microsoft Azure Cognitive Services Metrics Advisor SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.ai.metricsadvisor.administration.models;
import com.azure.ai.metricsadvisor.implementation.util.AzureLogAnalyticsDataFeedSourceAccessor;
import com.azure.core.annotation.Immutable;
/** The AzureLogAnalyticsDataFeedSource model. */
@Immutable
public final class AzureLogAnalyticsDataFeedSource extends DataFeedSource {
/*
* The tenant id of service principal that have access to this Log
* Analytics
*/
private final String tenantId;
/*
* The client id of service principal that have access to this Log
* Analytics
*/
private final String clientId;
/*
* The client secret of service principal that have access to this Log
* Analytics
*/
private final String clientSecret;
/*
* The workspace id of this Log Analytics
*/
private final String workspaceId;
/*
* The KQL (Kusto Query Language) query to fetch data from this Log
* Analytics
*/
private final String query;
/*
* The id of the credential resource to authenticate the data source.
*/
private final String credentialId;
/*
* The authentication type to access the data source.
*/
private final DataSourceAuthenticationType authType;
static {
AzureLogAnalyticsDataFeedSourceAccessor.setAccessor(new AzureLogAnalyticsDataFeedSourceAccessor.Accessor() {
@Override
public String getClientSecret(AzureLogAnalyticsDataFeedSource feedSource) {
return feedSource.getClientSecret();
}
});
}
private AzureLogAnalyticsDataFeedSource(final String tenantId, final String clientId, final String clientSecret,
final String workspaceId, final String query, final String credentialId,
final DataSourceAuthenticationType authType) {
this.tenantId = tenantId;
this.clientId = clientId;
this.clientSecret = clientSecret;
this.workspaceId = workspaceId;
this.query = query;
this.credentialId = credentialId;
this.authType = authType;
}
/**
* Create a AzureLogAnalyticsDataFeedSource with the given {@code tenantId}, {@code clientId} and
* {@code clientSecret} for authentication.
*
* @param tenantId The tenant id of service principal that have access to this Log Analytics.
* @param clientId The client id of service principal that have access to this Log Analytics.
* @param clientSecret The client secret of service principal that have access to this Log Analytics.
* @param workspaceId the query script.
* @param query the KQL (Kusto Query Language) query to fetch data from this Log
* Analytics.
*
* @return The AzureLogAnalyticsDataFeedSource.
*/
public static AzureLogAnalyticsDataFeedSource fromBasicCredential(final String tenantId, final String clientId,
final String clientSecret, final String workspaceId, final String query) {
return new AzureLogAnalyticsDataFeedSource(tenantId, clientId, clientSecret, workspaceId, query, null,
DataSourceAuthenticationType.BASIC);
}
/**
* Create a AzureLogAnalyticsDataFeedSource with the {@code credentialId} identifying
* a credential entity of type {@link DataSourceServicePrincipal}, the entity
* contains details of the KeyVault holding the Service Principal to access the Data Lake storage.
*
* @param workspaceId the query script.
* @param query the KQL (Kusto Query Language) query to fetch data from this Log Analytics.
* @param credentialId The unique id of a credential entity of type
*
* @return The AzureLogAnalyticsDataFeedSource.
*/
public static AzureLogAnalyticsDataFeedSource fromServicePrincipalCredential(final String workspaceId,
final String query, final String credentialId) {
return new AzureLogAnalyticsDataFeedSource(null, null, null, workspaceId, query, credentialId,
DataSourceAuthenticationType.SERVICE_PRINCIPAL);
}
/**
* Create a AzureLogAnalyticsDataFeedSource with the {@code credentialId} identifying
* a credential entity of type {@link DataSourceServicePrincipalInKeyVault}, the entity
* contains details of the KeyVault holding the Service Principal to access the Data Lake storage.
*
* @param workspaceId the query script.
* @param query the KQL (Kusto Query Language) query to fetch data from this Log Analytics.
* @param credentialId The unique id of a credential entity of type
*
* @return The AzureLogAnalyticsDataFeedSource.
*/
public static AzureLogAnalyticsDataFeedSource fromServicePrincipalInKeyVaultCredential(final String workspaceId,
final String query, final String credentialId) {
return new AzureLogAnalyticsDataFeedSource(null, null, null, workspaceId, query, credentialId,
DataSourceAuthenticationType.SERVICE_PRINCIPAL_IN_KV);
}
/**
* Get the tenantId property: The tenant id of service principal that have access to this Log Analytics.
*
* @return the tenantId value.
*/
public String getTenantId() {
return this.tenantId;
}
/**
* Get the clientId property: The client id of service principal that have access to this Log Analytics.
*
* @return the clientId value.
*/
public String getClientId() {
return this.clientId;
}
/**
* Get the workspaceId property: The workspace id of this Log Analytics.
*
* @return the workspaceId value.
*/
public String getWorkspaceId() {
return this.workspaceId;
}
/**
* Get the query property: The KQL (Kusto Query Language) query to fetch data from this Log Analytics.
*
* @return the query value.
*/
public String getQuery() {
return this.query;
}
/**
* Gets the id of the {@link DataSourceCredentialEntity credential resource} to authenticate the data source.
*
* @return The credential resource id.
*/
public String getCredentialId() {
return this.credentialId;
}
/**
* Gets the authentication type to access the data source.
*
* @return The authentication type.
*/
public DataSourceAuthenticationType getAuthenticationType() {
return this.authType;
}
private String getClientSecret() {
return this.clientSecret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy