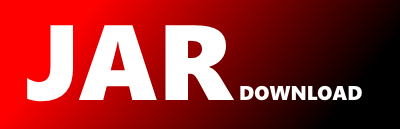
com.azure.ai.metricsadvisor.implementation.models.AnomalyDetectionConfigurationPatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-ai-metricsadvisor Show documentation
Show all versions of azure-ai-metricsadvisor Show documentation
This package contains the Microsoft Azure Cognitive Services Metrics Advisor SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.ai.metricsadvisor.implementation.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* The AnomalyDetectionConfigurationPatch model.
*/
@Fluent
public final class AnomalyDetectionConfigurationPatch implements JsonSerializable {
/*
* anomaly detection configuration name
*/
private String name;
/*
* anomaly detection configuration description
*/
private String description;
/*
* The wholeMetricConfiguration property.
*/
private WholeMetricConfigurationPatch wholeMetricConfiguration;
/*
* detection configuration for series group
*/
private List dimensionGroupOverrideConfigurations;
/*
* detection configuration for specific series
*/
private List seriesOverrideConfigurations;
/**
* Creates an instance of AnomalyDetectionConfigurationPatch class.
*/
public AnomalyDetectionConfigurationPatch() {
}
/**
* Get the name property: anomaly detection configuration name.
*
* @return the name value.
*/
public String getName() {
return this.name;
}
/**
* Set the name property: anomaly detection configuration name.
*
* @param name the name value to set.
* @return the AnomalyDetectionConfigurationPatch object itself.
*/
public AnomalyDetectionConfigurationPatch setName(String name) {
this.name = name;
return this;
}
/**
* Get the description property: anomaly detection configuration description.
*
* @return the description value.
*/
public String getDescription() {
return this.description;
}
/**
* Set the description property: anomaly detection configuration description.
*
* @param description the description value to set.
* @return the AnomalyDetectionConfigurationPatch object itself.
*/
public AnomalyDetectionConfigurationPatch setDescription(String description) {
this.description = description;
return this;
}
/**
* Get the wholeMetricConfiguration property: The wholeMetricConfiguration property.
*
* @return the wholeMetricConfiguration value.
*/
public WholeMetricConfigurationPatch getWholeMetricConfiguration() {
return this.wholeMetricConfiguration;
}
/**
* Set the wholeMetricConfiguration property: The wholeMetricConfiguration property.
*
* @param wholeMetricConfiguration the wholeMetricConfiguration value to set.
* @return the AnomalyDetectionConfigurationPatch object itself.
*/
public AnomalyDetectionConfigurationPatch
setWholeMetricConfiguration(WholeMetricConfigurationPatch wholeMetricConfiguration) {
this.wholeMetricConfiguration = wholeMetricConfiguration;
return this;
}
/**
* Get the dimensionGroupOverrideConfigurations property: detection configuration for series group.
*
* @return the dimensionGroupOverrideConfigurations value.
*/
public List getDimensionGroupOverrideConfigurations() {
return this.dimensionGroupOverrideConfigurations;
}
/**
* Set the dimensionGroupOverrideConfigurations property: detection configuration for series group.
*
* @param dimensionGroupOverrideConfigurations the dimensionGroupOverrideConfigurations value to set.
* @return the AnomalyDetectionConfigurationPatch object itself.
*/
public AnomalyDetectionConfigurationPatch setDimensionGroupOverrideConfigurations(
List dimensionGroupOverrideConfigurations) {
this.dimensionGroupOverrideConfigurations = dimensionGroupOverrideConfigurations;
return this;
}
/**
* Get the seriesOverrideConfigurations property: detection configuration for specific series.
*
* @return the seriesOverrideConfigurations value.
*/
public List getSeriesOverrideConfigurations() {
return this.seriesOverrideConfigurations;
}
/**
* Set the seriesOverrideConfigurations property: detection configuration for specific series.
*
* @param seriesOverrideConfigurations the seriesOverrideConfigurations value to set.
* @return the AnomalyDetectionConfigurationPatch object itself.
*/
public AnomalyDetectionConfigurationPatch
setSeriesOverrideConfigurations(List seriesOverrideConfigurations) {
this.seriesOverrideConfigurations = seriesOverrideConfigurations;
return this;
}
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("name", this.name);
jsonWriter.writeStringField("description", this.description);
jsonWriter.writeJsonField("wholeMetricConfiguration", this.wholeMetricConfiguration);
jsonWriter.writeArrayField("dimensionGroupOverrideConfigurations", this.dimensionGroupOverrideConfigurations,
(writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("seriesOverrideConfigurations", this.seriesOverrideConfigurations,
(writer, element) -> writer.writeJson(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of AnomalyDetectionConfigurationPatch from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of AnomalyDetectionConfigurationPatch if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the AnomalyDetectionConfigurationPatch.
*/
public static AnomalyDetectionConfigurationPatch fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
AnomalyDetectionConfigurationPatch deserializedAnomalyDetectionConfigurationPatch
= new AnomalyDetectionConfigurationPatch();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("name".equals(fieldName)) {
deserializedAnomalyDetectionConfigurationPatch.name = reader.getString();
} else if ("description".equals(fieldName)) {
deserializedAnomalyDetectionConfigurationPatch.description = reader.getString();
} else if ("wholeMetricConfiguration".equals(fieldName)) {
deserializedAnomalyDetectionConfigurationPatch.wholeMetricConfiguration
= WholeMetricConfigurationPatch.fromJson(reader);
} else if ("dimensionGroupOverrideConfigurations".equals(fieldName)) {
List dimensionGroupOverrideConfigurations
= reader.readArray(reader1 -> DimensionGroupConfiguration.fromJson(reader1));
deserializedAnomalyDetectionConfigurationPatch.dimensionGroupOverrideConfigurations
= dimensionGroupOverrideConfigurations;
} else if ("seriesOverrideConfigurations".equals(fieldName)) {
List seriesOverrideConfigurations
= reader.readArray(reader1 -> SeriesConfiguration.fromJson(reader1));
deserializedAnomalyDetectionConfigurationPatch.seriesOverrideConfigurations
= seriesOverrideConfigurations;
} else {
reader.skipChildren();
}
}
return deserializedAnomalyDetectionConfigurationPatch;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy