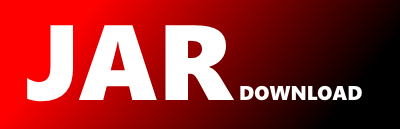
com.azure.ai.metricsadvisor.implementation.models.IncidentResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-ai-metricsadvisor Show documentation
Show all versions of azure-ai-metricsadvisor Show documentation
This package contains the Microsoft Azure Cognitive Services Metrics Advisor SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.ai.metricsadvisor.implementation.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
import java.util.UUID;
/**
* The IncidentResult model.
*/
@Fluent
public final class IncidentResult implements JsonSerializable {
/*
* data feed unique id
*
* only return for alerting anomaly result
*/
private UUID dataFeedId;
/*
* metric unique id
*
* only return for alerting incident result
*/
private UUID metricId;
/*
* anomaly detection configuration unique id
*
* only return for alerting incident result
*/
private UUID anomalyDetectionConfigurationId;
/*
* incident id
*/
private String incidentId;
/*
* incident start time
*/
private OffsetDateTime startTime;
/*
* incident last time
*/
private OffsetDateTime lastTime;
/*
* The rootNode property.
*/
private SeriesIdentity rootNode;
/*
* The property property.
*/
private IncidentProperty property;
/**
* Creates an instance of IncidentResult class.
*/
public IncidentResult() {
}
/**
* Get the dataFeedId property: data feed unique id
*
* only return for alerting anomaly result.
*
* @return the dataFeedId value.
*/
public UUID getDataFeedId() {
return this.dataFeedId;
}
/**
* Get the metricId property: metric unique id
*
* only return for alerting incident result.
*
* @return the metricId value.
*/
public UUID getMetricId() {
return this.metricId;
}
/**
* Get the anomalyDetectionConfigurationId property: anomaly detection configuration unique id
*
* only return for alerting incident result.
*
* @return the anomalyDetectionConfigurationId value.
*/
public UUID getAnomalyDetectionConfigurationId() {
return this.anomalyDetectionConfigurationId;
}
/**
* Get the incidentId property: incident id.
*
* @return the incidentId value.
*/
public String getIncidentId() {
return this.incidentId;
}
/**
* Set the incidentId property: incident id.
*
* @param incidentId the incidentId value to set.
* @return the IncidentResult object itself.
*/
public IncidentResult setIncidentId(String incidentId) {
this.incidentId = incidentId;
return this;
}
/**
* Get the startTime property: incident start time.
*
* @return the startTime value.
*/
public OffsetDateTime getStartTime() {
return this.startTime;
}
/**
* Set the startTime property: incident start time.
*
* @param startTime the startTime value to set.
* @return the IncidentResult object itself.
*/
public IncidentResult setStartTime(OffsetDateTime startTime) {
this.startTime = startTime;
return this;
}
/**
* Get the lastTime property: incident last time.
*
* @return the lastTime value.
*/
public OffsetDateTime getLastTime() {
return this.lastTime;
}
/**
* Set the lastTime property: incident last time.
*
* @param lastTime the lastTime value to set.
* @return the IncidentResult object itself.
*/
public IncidentResult setLastTime(OffsetDateTime lastTime) {
this.lastTime = lastTime;
return this;
}
/**
* Get the rootNode property: The rootNode property.
*
* @return the rootNode value.
*/
public SeriesIdentity getRootNode() {
return this.rootNode;
}
/**
* Set the rootNode property: The rootNode property.
*
* @param rootNode the rootNode value to set.
* @return the IncidentResult object itself.
*/
public IncidentResult setRootNode(SeriesIdentity rootNode) {
this.rootNode = rootNode;
return this;
}
/**
* Get the property property: The property property.
*
* @return the property value.
*/
public IncidentProperty getProperty() {
return this.property;
}
/**
* Set the property property: The property property.
*
* @param property the property value to set.
* @return the IncidentResult object itself.
*/
public IncidentResult setProperty(IncidentProperty property) {
this.property = property;
return this;
}
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("incidentId", this.incidentId);
jsonWriter.writeStringField("startTime",
this.startTime == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.startTime));
jsonWriter.writeStringField("lastTime",
this.lastTime == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(this.lastTime));
jsonWriter.writeJsonField("rootNode", this.rootNode);
jsonWriter.writeJsonField("property", this.property);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of IncidentResult from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of IncidentResult if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the IncidentResult.
*/
public static IncidentResult fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
IncidentResult deserializedIncidentResult = new IncidentResult();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("incidentId".equals(fieldName)) {
deserializedIncidentResult.incidentId = reader.getString();
} else if ("startTime".equals(fieldName)) {
deserializedIncidentResult.startTime
= reader.getNullable(nonNullReader -> OffsetDateTime.parse(nonNullReader.getString()));
} else if ("lastTime".equals(fieldName)) {
deserializedIncidentResult.lastTime
= reader.getNullable(nonNullReader -> OffsetDateTime.parse(nonNullReader.getString()));
} else if ("rootNode".equals(fieldName)) {
deserializedIncidentResult.rootNode = SeriesIdentity.fromJson(reader);
} else if ("property".equals(fieldName)) {
deserializedIncidentResult.property = IncidentProperty.fromJson(reader);
} else if ("dataFeedId".equals(fieldName)) {
deserializedIncidentResult.dataFeedId
= reader.getNullable(nonNullReader -> UUID.fromString(nonNullReader.getString()));
} else if ("metricId".equals(fieldName)) {
deserializedIncidentResult.metricId
= reader.getNullable(nonNullReader -> UUID.fromString(nonNullReader.getString()));
} else if ("anomalyDetectionConfigurationId".equals(fieldName)) {
deserializedIncidentResult.anomalyDetectionConfigurationId
= reader.getNullable(nonNullReader -> UUID.fromString(nonNullReader.getString()));
} else {
reader.skipChildren();
}
}
return deserializedIncidentResult;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy