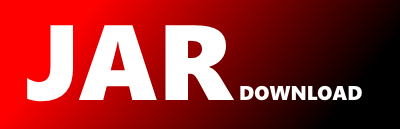
com.azure.ai.metricsadvisor.implementation.models.MetricAlertingConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-ai-metricsadvisor Show documentation
Show all versions of azure-ai-metricsadvisor Show documentation
This package contains the Microsoft Azure Cognitive Services Metrics Advisor SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.ai.metricsadvisor.implementation.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.Objects;
import java.util.UUID;
/**
* The MetricAlertingConfiguration model.
*/
@Fluent
public final class MetricAlertingConfiguration implements JsonSerializable {
/*
* Anomaly detection configuration unique id
*/
private UUID anomalyDetectionConfigurationId;
/*
* Anomaly scope
*/
private AnomalyScope anomalyScopeType;
/*
* Negation operation
*/
private Boolean negationOperation;
/*
* The dimensionAnomalyScope property.
*/
private DimensionGroupIdentity dimensionAnomalyScope;
/*
* The topNAnomalyScope property.
*/
private TopNGroupScope topNAnomalyScope;
/*
* The severityFilter property.
*/
private SeverityCondition severityFilter;
/*
* The snoozeFilter property.
*/
private MetricAnomalyAlertSnoozeCondition snoozeFilter;
/*
* The valueFilter property.
*/
private ValueCondition valueFilter;
/**
* Creates an instance of MetricAlertingConfiguration class.
*/
public MetricAlertingConfiguration() {
}
/**
* Get the anomalyDetectionConfigurationId property: Anomaly detection configuration unique id.
*
* @return the anomalyDetectionConfigurationId value.
*/
public UUID getAnomalyDetectionConfigurationId() {
return this.anomalyDetectionConfigurationId;
}
/**
* Set the anomalyDetectionConfigurationId property: Anomaly detection configuration unique id.
*
* @param anomalyDetectionConfigurationId the anomalyDetectionConfigurationId value to set.
* @return the MetricAlertingConfiguration object itself.
*/
public MetricAlertingConfiguration setAnomalyDetectionConfigurationId(UUID anomalyDetectionConfigurationId) {
this.anomalyDetectionConfigurationId = anomalyDetectionConfigurationId;
return this;
}
/**
* Get the anomalyScopeType property: Anomaly scope.
*
* @return the anomalyScopeType value.
*/
public AnomalyScope getAnomalyScopeType() {
return this.anomalyScopeType;
}
/**
* Set the anomalyScopeType property: Anomaly scope.
*
* @param anomalyScopeType the anomalyScopeType value to set.
* @return the MetricAlertingConfiguration object itself.
*/
public MetricAlertingConfiguration setAnomalyScopeType(AnomalyScope anomalyScopeType) {
this.anomalyScopeType = anomalyScopeType;
return this;
}
/**
* Get the negationOperation property: Negation operation.
*
* @return the negationOperation value.
*/
public Boolean isNegationOperation() {
return this.negationOperation;
}
/**
* Set the negationOperation property: Negation operation.
*
* @param negationOperation the negationOperation value to set.
* @return the MetricAlertingConfiguration object itself.
*/
public MetricAlertingConfiguration setNegationOperation(Boolean negationOperation) {
this.negationOperation = negationOperation;
return this;
}
/**
* Get the dimensionAnomalyScope property: The dimensionAnomalyScope property.
*
* @return the dimensionAnomalyScope value.
*/
public DimensionGroupIdentity getDimensionAnomalyScope() {
return this.dimensionAnomalyScope;
}
/**
* Set the dimensionAnomalyScope property: The dimensionAnomalyScope property.
*
* @param dimensionAnomalyScope the dimensionAnomalyScope value to set.
* @return the MetricAlertingConfiguration object itself.
*/
public MetricAlertingConfiguration setDimensionAnomalyScope(DimensionGroupIdentity dimensionAnomalyScope) {
this.dimensionAnomalyScope = dimensionAnomalyScope;
return this;
}
/**
* Get the topNAnomalyScope property: The topNAnomalyScope property.
*
* @return the topNAnomalyScope value.
*/
public TopNGroupScope getTopNAnomalyScope() {
return this.topNAnomalyScope;
}
/**
* Set the topNAnomalyScope property: The topNAnomalyScope property.
*
* @param topNAnomalyScope the topNAnomalyScope value to set.
* @return the MetricAlertingConfiguration object itself.
*/
public MetricAlertingConfiguration setTopNAnomalyScope(TopNGroupScope topNAnomalyScope) {
this.topNAnomalyScope = topNAnomalyScope;
return this;
}
/**
* Get the severityFilter property: The severityFilter property.
*
* @return the severityFilter value.
*/
public SeverityCondition getSeverityFilter() {
return this.severityFilter;
}
/**
* Set the severityFilter property: The severityFilter property.
*
* @param severityFilter the severityFilter value to set.
* @return the MetricAlertingConfiguration object itself.
*/
public MetricAlertingConfiguration setSeverityFilter(SeverityCondition severityFilter) {
this.severityFilter = severityFilter;
return this;
}
/**
* Get the snoozeFilter property: The snoozeFilter property.
*
* @return the snoozeFilter value.
*/
public MetricAnomalyAlertSnoozeCondition getSnoozeFilter() {
return this.snoozeFilter;
}
/**
* Set the snoozeFilter property: The snoozeFilter property.
*
* @param snoozeFilter the snoozeFilter value to set.
* @return the MetricAlertingConfiguration object itself.
*/
public MetricAlertingConfiguration setSnoozeFilter(MetricAnomalyAlertSnoozeCondition snoozeFilter) {
this.snoozeFilter = snoozeFilter;
return this;
}
/**
* Get the valueFilter property: The valueFilter property.
*
* @return the valueFilter value.
*/
public ValueCondition getValueFilter() {
return this.valueFilter;
}
/**
* Set the valueFilter property: The valueFilter property.
*
* @param valueFilter the valueFilter value to set.
* @return the MetricAlertingConfiguration object itself.
*/
public MetricAlertingConfiguration setValueFilter(ValueCondition valueFilter) {
this.valueFilter = valueFilter;
return this;
}
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("anomalyDetectionConfigurationId",
Objects.toString(this.anomalyDetectionConfigurationId, null));
jsonWriter.writeStringField("anomalyScopeType",
this.anomalyScopeType == null ? null : this.anomalyScopeType.toString());
jsonWriter.writeBooleanField("negationOperation", this.negationOperation);
jsonWriter.writeJsonField("dimensionAnomalyScope", this.dimensionAnomalyScope);
jsonWriter.writeJsonField("topNAnomalyScope", this.topNAnomalyScope);
jsonWriter.writeJsonField("severityFilter", this.severityFilter);
jsonWriter.writeJsonField("snoozeFilter", this.snoozeFilter);
jsonWriter.writeJsonField("valueFilter", this.valueFilter);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of MetricAlertingConfiguration from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of MetricAlertingConfiguration if the JsonReader was pointing to an instance of it, or null
* if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the MetricAlertingConfiguration.
*/
public static MetricAlertingConfiguration fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
MetricAlertingConfiguration deserializedMetricAlertingConfiguration = new MetricAlertingConfiguration();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("anomalyDetectionConfigurationId".equals(fieldName)) {
deserializedMetricAlertingConfiguration.anomalyDetectionConfigurationId
= reader.getNullable(nonNullReader -> UUID.fromString(nonNullReader.getString()));
} else if ("anomalyScopeType".equals(fieldName)) {
deserializedMetricAlertingConfiguration.anomalyScopeType
= AnomalyScope.fromString(reader.getString());
} else if ("negationOperation".equals(fieldName)) {
deserializedMetricAlertingConfiguration.negationOperation
= reader.getNullable(JsonReader::getBoolean);
} else if ("dimensionAnomalyScope".equals(fieldName)) {
deserializedMetricAlertingConfiguration.dimensionAnomalyScope
= DimensionGroupIdentity.fromJson(reader);
} else if ("topNAnomalyScope".equals(fieldName)) {
deserializedMetricAlertingConfiguration.topNAnomalyScope = TopNGroupScope.fromJson(reader);
} else if ("severityFilter".equals(fieldName)) {
deserializedMetricAlertingConfiguration.severityFilter = SeverityCondition.fromJson(reader);
} else if ("snoozeFilter".equals(fieldName)) {
deserializedMetricAlertingConfiguration.snoozeFilter
= MetricAnomalyAlertSnoozeCondition.fromJson(reader);
} else if ("valueFilter".equals(fieldName)) {
deserializedMetricAlertingConfiguration.valueFilter = ValueCondition.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedMetricAlertingConfiguration;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy