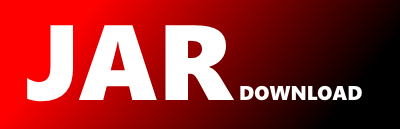
com.azure.ai.metricsadvisor.implementation.models.MetricAnomalyAlertSnoozeCondition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-ai-metricsadvisor Show documentation
Show all versions of azure-ai-metricsadvisor Show documentation
This package contains the Microsoft Azure Cognitive Services Metrics Advisor SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.ai.metricsadvisor.implementation.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* The MetricAnomalyAlertSnoozeCondition model.
*/
@Fluent
public final class MetricAnomalyAlertSnoozeCondition implements JsonSerializable {
/*
* snooze point count, value range : [0, +∞)
*/
private int autoSnooze;
/*
* snooze scope
*/
private SnoozeScope snoozeScope;
/*
* only snooze for successive anomalies
*/
private boolean onlyForSuccessive;
/**
* Creates an instance of MetricAnomalyAlertSnoozeCondition class.
*/
public MetricAnomalyAlertSnoozeCondition() {
}
/**
* Get the autoSnooze property: snooze point count, value range : [0, +∞).
*
* @return the autoSnooze value.
*/
public int getAutoSnooze() {
return this.autoSnooze;
}
/**
* Set the autoSnooze property: snooze point count, value range : [0, +∞).
*
* @param autoSnooze the autoSnooze value to set.
* @return the MetricAnomalyAlertSnoozeCondition object itself.
*/
public MetricAnomalyAlertSnoozeCondition setAutoSnooze(int autoSnooze) {
this.autoSnooze = autoSnooze;
return this;
}
/**
* Get the snoozeScope property: snooze scope.
*
* @return the snoozeScope value.
*/
public SnoozeScope getSnoozeScope() {
return this.snoozeScope;
}
/**
* Set the snoozeScope property: snooze scope.
*
* @param snoozeScope the snoozeScope value to set.
* @return the MetricAnomalyAlertSnoozeCondition object itself.
*/
public MetricAnomalyAlertSnoozeCondition setSnoozeScope(SnoozeScope snoozeScope) {
this.snoozeScope = snoozeScope;
return this;
}
/**
* Get the onlyForSuccessive property: only snooze for successive anomalies.
*
* @return the onlyForSuccessive value.
*/
public boolean isOnlyForSuccessive() {
return this.onlyForSuccessive;
}
/**
* Set the onlyForSuccessive property: only snooze for successive anomalies.
*
* @param onlyForSuccessive the onlyForSuccessive value to set.
* @return the MetricAnomalyAlertSnoozeCondition object itself.
*/
public MetricAnomalyAlertSnoozeCondition setOnlyForSuccessive(boolean onlyForSuccessive) {
this.onlyForSuccessive = onlyForSuccessive;
return this;
}
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeIntField("autoSnooze", this.autoSnooze);
jsonWriter.writeStringField("snoozeScope", this.snoozeScope == null ? null : this.snoozeScope.toString());
jsonWriter.writeBooleanField("onlyForSuccessive", this.onlyForSuccessive);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of MetricAnomalyAlertSnoozeCondition from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of MetricAnomalyAlertSnoozeCondition if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the MetricAnomalyAlertSnoozeCondition.
*/
public static MetricAnomalyAlertSnoozeCondition fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
MetricAnomalyAlertSnoozeCondition deserializedMetricAnomalyAlertSnoozeCondition
= new MetricAnomalyAlertSnoozeCondition();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("autoSnooze".equals(fieldName)) {
deserializedMetricAnomalyAlertSnoozeCondition.autoSnooze = reader.getInt();
} else if ("snoozeScope".equals(fieldName)) {
deserializedMetricAnomalyAlertSnoozeCondition.snoozeScope
= SnoozeScope.fromString(reader.getString());
} else if ("onlyForSuccessive".equals(fieldName)) {
deserializedMetricAnomalyAlertSnoozeCondition.onlyForSuccessive = reader.getBoolean();
} else {
reader.skipChildren();
}
}
return deserializedMetricAnomalyAlertSnoozeCondition;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy