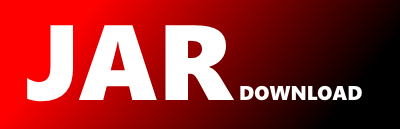
com.azure.ai.metricsadvisor.implementation.models.SeriesResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-ai-metricsadvisor Show documentation
Show all versions of azure-ai-metricsadvisor Show documentation
This package contains the Microsoft Azure Cognitive Services Metrics Advisor SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.ai.metricsadvisor.implementation.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.time.format.DateTimeFormatter;
import java.util.List;
/**
* The SeriesResult model.
*/
@Fluent
public final class SeriesResult implements JsonSerializable {
/*
* The series property.
*/
private SeriesIdentity series;
/*
* timestamps of the series
*/
private List timestampList;
/*
* values of the series
*/
private List valueList;
/*
* whether points of the series are anomalies
*/
private List isAnomalyList;
/*
* period calculated on each point of the series
*/
private List periodList;
/*
* expected values of the series given by smart detector
*/
private List expectedValueList;
/*
* lower boundary list of the series given by smart detector
*/
private List lowerBoundaryList;
/*
* upper boundary list of the series given by smart detector
*/
private List upperBoundaryList;
/**
* Creates an instance of SeriesResult class.
*/
public SeriesResult() {
}
/**
* Get the series property: The series property.
*
* @return the series value.
*/
public SeriesIdentity getSeries() {
return this.series;
}
/**
* Set the series property: The series property.
*
* @param series the series value to set.
* @return the SeriesResult object itself.
*/
public SeriesResult setSeries(SeriesIdentity series) {
this.series = series;
return this;
}
/**
* Get the timestampList property: timestamps of the series.
*
* @return the timestampList value.
*/
public List getTimestampList() {
return this.timestampList;
}
/**
* Set the timestampList property: timestamps of the series.
*
* @param timestampList the timestampList value to set.
* @return the SeriesResult object itself.
*/
public SeriesResult setTimestampList(List timestampList) {
this.timestampList = timestampList;
return this;
}
/**
* Get the valueList property: values of the series.
*
* @return the valueList value.
*/
public List getValueList() {
return this.valueList;
}
/**
* Set the valueList property: values of the series.
*
* @param valueList the valueList value to set.
* @return the SeriesResult object itself.
*/
public SeriesResult setValueList(List valueList) {
this.valueList = valueList;
return this;
}
/**
* Get the isAnomalyList property: whether points of the series are anomalies.
*
* @return the isAnomalyList value.
*/
public List getIsAnomalyList() {
return this.isAnomalyList;
}
/**
* Set the isAnomalyList property: whether points of the series are anomalies.
*
* @param isAnomalyList the isAnomalyList value to set.
* @return the SeriesResult object itself.
*/
public SeriesResult setIsAnomalyList(List isAnomalyList) {
this.isAnomalyList = isAnomalyList;
return this;
}
/**
* Get the periodList property: period calculated on each point of the series.
*
* @return the periodList value.
*/
public List getPeriodList() {
return this.periodList;
}
/**
* Set the periodList property: period calculated on each point of the series.
*
* @param periodList the periodList value to set.
* @return the SeriesResult object itself.
*/
public SeriesResult setPeriodList(List periodList) {
this.periodList = periodList;
return this;
}
/**
* Get the expectedValueList property: expected values of the series given by smart detector.
*
* @return the expectedValueList value.
*/
public List getExpectedValueList() {
return this.expectedValueList;
}
/**
* Set the expectedValueList property: expected values of the series given by smart detector.
*
* @param expectedValueList the expectedValueList value to set.
* @return the SeriesResult object itself.
*/
public SeriesResult setExpectedValueList(List expectedValueList) {
this.expectedValueList = expectedValueList;
return this;
}
/**
* Get the lowerBoundaryList property: lower boundary list of the series given by smart detector.
*
* @return the lowerBoundaryList value.
*/
public List getLowerBoundaryList() {
return this.lowerBoundaryList;
}
/**
* Set the lowerBoundaryList property: lower boundary list of the series given by smart detector.
*
* @param lowerBoundaryList the lowerBoundaryList value to set.
* @return the SeriesResult object itself.
*/
public SeriesResult setLowerBoundaryList(List lowerBoundaryList) {
this.lowerBoundaryList = lowerBoundaryList;
return this;
}
/**
* Get the upperBoundaryList property: upper boundary list of the series given by smart detector.
*
* @return the upperBoundaryList value.
*/
public List getUpperBoundaryList() {
return this.upperBoundaryList;
}
/**
* Set the upperBoundaryList property: upper boundary list of the series given by smart detector.
*
* @param upperBoundaryList the upperBoundaryList value to set.
* @return the SeriesResult object itself.
*/
public SeriesResult setUpperBoundaryList(List upperBoundaryList) {
this.upperBoundaryList = upperBoundaryList;
return this;
}
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeJsonField("series", this.series);
jsonWriter.writeArrayField("timestampList", this.timestampList, (writer, element) -> writer
.writeString(element == null ? null : DateTimeFormatter.ISO_OFFSET_DATE_TIME.format(element)));
jsonWriter.writeArrayField("valueList", this.valueList, (writer, element) -> writer.writeDouble(element));
jsonWriter.writeArrayField("isAnomalyList", this.isAnomalyList,
(writer, element) -> writer.writeBoolean(element));
jsonWriter.writeArrayField("periodList", this.periodList, (writer, element) -> writer.writeInt(element));
jsonWriter.writeArrayField("expectedValueList", this.expectedValueList,
(writer, element) -> writer.writeDouble(element));
jsonWriter.writeArrayField("lowerBoundaryList", this.lowerBoundaryList,
(writer, element) -> writer.writeDouble(element));
jsonWriter.writeArrayField("upperBoundaryList", this.upperBoundaryList,
(writer, element) -> writer.writeDouble(element));
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of SeriesResult from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of SeriesResult if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the SeriesResult.
*/
public static SeriesResult fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
SeriesResult deserializedSeriesResult = new SeriesResult();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("series".equals(fieldName)) {
deserializedSeriesResult.series = SeriesIdentity.fromJson(reader);
} else if ("timestampList".equals(fieldName)) {
List timestampList = reader.readArray(reader1 -> reader1
.getNullable(nonNullReader -> OffsetDateTime.parse(nonNullReader.getString())));
deserializedSeriesResult.timestampList = timestampList;
} else if ("valueList".equals(fieldName)) {
List valueList = reader.readArray(reader1 -> reader1.getDouble());
deserializedSeriesResult.valueList = valueList;
} else if ("isAnomalyList".equals(fieldName)) {
List isAnomalyList = reader.readArray(reader1 -> reader1.getBoolean());
deserializedSeriesResult.isAnomalyList = isAnomalyList;
} else if ("periodList".equals(fieldName)) {
List periodList = reader.readArray(reader1 -> reader1.getInt());
deserializedSeriesResult.periodList = periodList;
} else if ("expectedValueList".equals(fieldName)) {
List expectedValueList = reader.readArray(reader1 -> reader1.getDouble());
deserializedSeriesResult.expectedValueList = expectedValueList;
} else if ("lowerBoundaryList".equals(fieldName)) {
List lowerBoundaryList = reader.readArray(reader1 -> reader1.getDouble());
deserializedSeriesResult.lowerBoundaryList = lowerBoundaryList;
} else if ("upperBoundaryList".equals(fieldName)) {
List upperBoundaryList = reader.readArray(reader1 -> reader1.getDouble());
deserializedSeriesResult.upperBoundaryList = upperBoundaryList;
} else {
reader.skipChildren();
}
}
return deserializedSeriesResult;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy