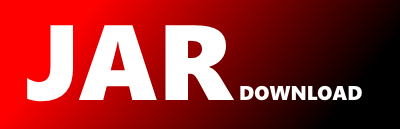
com.azure.ai.metricsadvisor.implementation.models.ServicePrincipalInKVParamPatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-ai-metricsadvisor Show documentation
Show all versions of azure-ai-metricsadvisor Show documentation
This package contains the Microsoft Azure Cognitive Services Metrics Advisor SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.ai.metricsadvisor.implementation.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* The ServicePrincipalInKVParamPatch model.
*/
@Fluent
public final class ServicePrincipalInKVParamPatch implements JsonSerializable {
/*
* The Key Vault endpoint that storing the service principal.
*/
private String keyVaultEndpoint;
/*
* The Client Id to access the Key Vault.
*/
private String keyVaultClientId;
/*
* The Client Secret to access the Key Vault.
*/
private String keyVaultClientSecret;
/*
* The secret name of the service principal's client Id in the Key Vault.
*/
private String servicePrincipalIdNameInKV;
/*
* The secret name of the service principal's client secret in the Key Vault.
*/
private String servicePrincipalSecretNameInKV;
/*
* The tenant id of your service principal.
*/
private String tenantId;
/**
* Creates an instance of ServicePrincipalInKVParamPatch class.
*/
public ServicePrincipalInKVParamPatch() {
}
/**
* Get the keyVaultEndpoint property: The Key Vault endpoint that storing the service principal.
*
* @return the keyVaultEndpoint value.
*/
public String getKeyVaultEndpoint() {
return this.keyVaultEndpoint;
}
/**
* Set the keyVaultEndpoint property: The Key Vault endpoint that storing the service principal.
*
* @param keyVaultEndpoint the keyVaultEndpoint value to set.
* @return the ServicePrincipalInKVParamPatch object itself.
*/
public ServicePrincipalInKVParamPatch setKeyVaultEndpoint(String keyVaultEndpoint) {
this.keyVaultEndpoint = keyVaultEndpoint;
return this;
}
/**
* Get the keyVaultClientId property: The Client Id to access the Key Vault.
*
* @return the keyVaultClientId value.
*/
public String getKeyVaultClientId() {
return this.keyVaultClientId;
}
/**
* Set the keyVaultClientId property: The Client Id to access the Key Vault.
*
* @param keyVaultClientId the keyVaultClientId value to set.
* @return the ServicePrincipalInKVParamPatch object itself.
*/
public ServicePrincipalInKVParamPatch setKeyVaultClientId(String keyVaultClientId) {
this.keyVaultClientId = keyVaultClientId;
return this;
}
/**
* Get the keyVaultClientSecret property: The Client Secret to access the Key Vault.
*
* @return the keyVaultClientSecret value.
*/
public String getKeyVaultClientSecret() {
return this.keyVaultClientSecret;
}
/**
* Set the keyVaultClientSecret property: The Client Secret to access the Key Vault.
*
* @param keyVaultClientSecret the keyVaultClientSecret value to set.
* @return the ServicePrincipalInKVParamPatch object itself.
*/
public ServicePrincipalInKVParamPatch setKeyVaultClientSecret(String keyVaultClientSecret) {
this.keyVaultClientSecret = keyVaultClientSecret;
return this;
}
/**
* Get the servicePrincipalIdNameInKV property: The secret name of the service principal's client Id in the Key
* Vault.
*
* @return the servicePrincipalIdNameInKV value.
*/
public String getServicePrincipalIdNameInKV() {
return this.servicePrincipalIdNameInKV;
}
/**
* Set the servicePrincipalIdNameInKV property: The secret name of the service principal's client Id in the Key
* Vault.
*
* @param servicePrincipalIdNameInKV the servicePrincipalIdNameInKV value to set.
* @return the ServicePrincipalInKVParamPatch object itself.
*/
public ServicePrincipalInKVParamPatch setServicePrincipalIdNameInKV(String servicePrincipalIdNameInKV) {
this.servicePrincipalIdNameInKV = servicePrincipalIdNameInKV;
return this;
}
/**
* Get the servicePrincipalSecretNameInKV property: The secret name of the service principal's client secret in the
* Key Vault.
*
* @return the servicePrincipalSecretNameInKV value.
*/
public String getServicePrincipalSecretNameInKV() {
return this.servicePrincipalSecretNameInKV;
}
/**
* Set the servicePrincipalSecretNameInKV property: The secret name of the service principal's client secret in the
* Key Vault.
*
* @param servicePrincipalSecretNameInKV the servicePrincipalSecretNameInKV value to set.
* @return the ServicePrincipalInKVParamPatch object itself.
*/
public ServicePrincipalInKVParamPatch setServicePrincipalSecretNameInKV(String servicePrincipalSecretNameInKV) {
this.servicePrincipalSecretNameInKV = servicePrincipalSecretNameInKV;
return this;
}
/**
* Get the tenantId property: The tenant id of your service principal.
*
* @return the tenantId value.
*/
public String getTenantId() {
return this.tenantId;
}
/**
* Set the tenantId property: The tenant id of your service principal.
*
* @param tenantId the tenantId value to set.
* @return the ServicePrincipalInKVParamPatch object itself.
*/
public ServicePrincipalInKVParamPatch setTenantId(String tenantId) {
this.tenantId = tenantId;
return this;
}
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("keyVaultEndpoint", this.keyVaultEndpoint);
jsonWriter.writeStringField("keyVaultClientId", this.keyVaultClientId);
jsonWriter.writeStringField("keyVaultClientSecret", this.keyVaultClientSecret);
jsonWriter.writeStringField("servicePrincipalIdNameInKV", this.servicePrincipalIdNameInKV);
jsonWriter.writeStringField("servicePrincipalSecretNameInKV", this.servicePrincipalSecretNameInKV);
jsonWriter.writeStringField("tenantId", this.tenantId);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of ServicePrincipalInKVParamPatch from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of ServicePrincipalInKVParamPatch if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the ServicePrincipalInKVParamPatch.
*/
public static ServicePrincipalInKVParamPatch fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
ServicePrincipalInKVParamPatch deserializedServicePrincipalInKVParamPatch
= new ServicePrincipalInKVParamPatch();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("keyVaultEndpoint".equals(fieldName)) {
deserializedServicePrincipalInKVParamPatch.keyVaultEndpoint = reader.getString();
} else if ("keyVaultClientId".equals(fieldName)) {
deserializedServicePrincipalInKVParamPatch.keyVaultClientId = reader.getString();
} else if ("keyVaultClientSecret".equals(fieldName)) {
deserializedServicePrincipalInKVParamPatch.keyVaultClientSecret = reader.getString();
} else if ("servicePrincipalIdNameInKV".equals(fieldName)) {
deserializedServicePrincipalInKVParamPatch.servicePrincipalIdNameInKV = reader.getString();
} else if ("servicePrincipalSecretNameInKV".equals(fieldName)) {
deserializedServicePrincipalInKVParamPatch.servicePrincipalSecretNameInKV = reader.getString();
} else if ("tenantId".equals(fieldName)) {
deserializedServicePrincipalInKVParamPatch.tenantId = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedServicePrincipalInKVParamPatch;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy