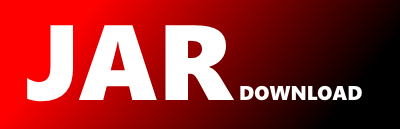
com.azure.ai.metricsadvisor.implementation.models.WebhookHookInfoPatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-ai-metricsadvisor Show documentation
Show all versions of azure-ai-metricsadvisor Show documentation
This package contains the Microsoft Azure Cognitive Services Metrics Advisor SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.ai.metricsadvisor.implementation.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* The WebhookHookInfoPatch model.
*/
@Fluent
public final class WebhookHookInfoPatch extends HookInfoPatch {
/*
* The hookParameter property.
*/
private WebhookHookParameterPatch hookParameter;
/**
* Creates an instance of WebhookHookInfoPatch class.
*/
public WebhookHookInfoPatch() {
}
/**
* Get the hookParameter property: The hookParameter property.
*
* @return the hookParameter value.
*/
public WebhookHookParameterPatch getHookParameter() {
return this.hookParameter;
}
/**
* Set the hookParameter property: The hookParameter property.
*
* @param hookParameter the hookParameter value to set.
* @return the WebhookHookInfoPatch object itself.
*/
public WebhookHookInfoPatch setHookParameter(WebhookHookParameterPatch hookParameter) {
this.hookParameter = hookParameter;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public WebhookHookInfoPatch setHookName(String hookName) {
super.setHookName(hookName);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public WebhookHookInfoPatch setDescription(String description) {
super.setDescription(description);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public WebhookHookInfoPatch setExternalLink(String externalLink) {
super.setExternalLink(externalLink);
return this;
}
/**
* {@inheritDoc}
*/
@Override
public WebhookHookInfoPatch setAdmins(List admins) {
super.setAdmins(admins);
return this;
}
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("hookType", HookType.WEBHOOK == null ? null : HookType.WEBHOOK.toString());
jsonWriter.writeStringField("hookName", getHookName());
jsonWriter.writeStringField("description", getDescription());
jsonWriter.writeStringField("externalLink", getExternalLink());
jsonWriter.writeArrayField("admins", getAdmins(), (writer, element) -> writer.writeString(element));
jsonWriter.writeJsonField("hookParameter", this.hookParameter);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of WebhookHookInfoPatch from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of WebhookHookInfoPatch if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing the polymorphic discriminator.
* @throws IOException If an error occurs while reading the WebhookHookInfoPatch.
*/
public static WebhookHookInfoPatch fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
WebhookHookInfoPatch deserializedWebhookHookInfoPatch = new WebhookHookInfoPatch();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("hookType".equals(fieldName)) {
String hookType = reader.getString();
if (!"Webhook".equals(hookType)) {
throw new IllegalStateException(
"'hookType' was expected to be non-null and equal to 'Webhook'. The found 'hookType' was '"
+ hookType + "'.");
}
} else if ("hookName".equals(fieldName)) {
deserializedWebhookHookInfoPatch.setHookName(reader.getString());
} else if ("description".equals(fieldName)) {
deserializedWebhookHookInfoPatch.setDescription(reader.getString());
} else if ("externalLink".equals(fieldName)) {
deserializedWebhookHookInfoPatch.setExternalLink(reader.getString());
} else if ("admins".equals(fieldName)) {
List admins = reader.readArray(reader1 -> reader1.getString());
deserializedWebhookHookInfoPatch.setAdmins(admins);
} else if ("hookParameter".equals(fieldName)) {
deserializedWebhookHookInfoPatch.hookParameter = WebhookHookParameterPatch.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedWebhookHookInfoPatch;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy