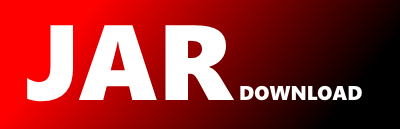
com.azure.ai.metricsadvisor.models.MetricEnrichedSeriesData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-ai-metricsadvisor Show documentation
Show all versions of azure-ai-metricsadvisor Show documentation
This package contains the Microsoft Azure Cognitive Services Metrics Advisor SDK.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.ai.metricsadvisor.models;
import com.azure.ai.metricsadvisor.implementation.util.MetricEnrichedSeriesDataHelper;
import java.time.OffsetDateTime;
import java.util.Collections;
import java.util.List;
/**
* Enriched time series data which includes additional service computed
* values for the time series data points.
*/
public final class MetricEnrichedSeriesData {
private DimensionKey seriesKey;
private List timestamps;
private List metricValues;
private List isAnomaly;
private List periods;
private List expectedMetricValues;
private List lowerBoundaryValues;
private List upperBoundaryValues;
/**
* Creates a new instance of MetricEnrichedSeriesData.
*/
public MetricEnrichedSeriesData() {
this.timestamps = Collections.emptyList();
this.metricValues = Collections.emptyList();
this.isAnomaly = Collections.emptyList();
this.periods = Collections.emptyList();
this.expectedMetricValues = Collections.emptyList();
this.lowerBoundaryValues = Collections.emptyList();
this.upperBoundaryValues = Collections.emptyList();
}
static {
MetricEnrichedSeriesDataHelper
.setAccessor(new MetricEnrichedSeriesDataHelper.MetricEnrichedSeriesDataAccessor() {
@Override
public void setSeriesKey(MetricEnrichedSeriesData seriesData, DimensionKey seriesKey) {
seriesData.setSeriesKey(seriesKey);
}
@Override
public void setTimestamps(MetricEnrichedSeriesData seriesData, List timestamps) {
seriesData.setTimestampList(timestamps);
}
@Override
public void setMetricValues(MetricEnrichedSeriesData seriesData, List metricValues) {
seriesData.setValueList(metricValues);
}
@Override
public void setIsAnomalyList(MetricEnrichedSeriesData seriesData, List isAnomaly) {
seriesData.setIsAnomalyList(isAnomaly);
}
@Override
public void setPeriods(MetricEnrichedSeriesData seriesData, List periods) {
seriesData.setPeriodList(periods);
}
@Override
public void setExpectedMetricValues(MetricEnrichedSeriesData seriesData,
List expectedMetricValues) {
seriesData.setExpectedValueList(expectedMetricValues);
}
@Override
public void setLowerBoundaryValues(MetricEnrichedSeriesData seriesData,
List lowerBoundaryValues) {
seriesData.setLowerBoundaryList(lowerBoundaryValues);
}
@Override
public void setUpperBoundaryValues(MetricEnrichedSeriesData seriesData,
List upperBoundaryValues) {
seriesData.setUpperBoundaryList(upperBoundaryValues);
}
});
}
/**
* Gets the key of the time series.
*
* @return The time series key.
*/
public DimensionKey getSeriesKey() {
return seriesKey;
}
/**
* Gets the timestamps of the data points in the time series.
*
* @return The timestamps.
*/
public List getTimestamps() {
return Collections.unmodifiableList(this.timestamps);
}
/**
* Gets the values of the data points in the time series.
*
* @return The values.
*/
public List getMetricValues() {
return Collections.unmodifiableList(this.metricValues);
}
/**
* Gets the anomaly status of the data points in the time series.
*
* @return The anomaly statuses.
*/
public List isAnomaly() {
return Collections.unmodifiableList(this.isAnomaly);
}
/**
* Gets the periods calculated for the data points in the time series.
*
* @return The periods.
*/
public List getPeriods() {
return Collections.unmodifiableList(this.periods);
}
/**
* Gets the expected values of the data points calculated by the smart detector.
*
* @return The expected values.
*/
public List getExpectedMetricValues() {
return Collections.unmodifiableList(this.expectedMetricValues);
}
/**
* Gets the lower boundary values of the data points calculated by smart detector.
*
* @return The lower bound values.
*/
public List getLowerBoundaryValues() {
return Collections.unmodifiableList(this.lowerBoundaryValues);
}
/**
* Gets the upper boundary values of the data points calculated by smart detector.
*
* @return The upper bound values.
*/
public List getUpperBoundaryValues() {
return Collections.unmodifiableList(this.upperBoundaryValues);
}
void setSeriesKey(DimensionKey seriesKey) {
this.seriesKey = seriesKey;
}
void setTimestampList(List timestamps) {
this.timestamps = timestamps;
}
void setValueList(List metricValues) {
this.metricValues = metricValues;
}
void setIsAnomalyList(List isAnomaly) {
this.isAnomaly = isAnomaly;
}
void setPeriodList(List periods) {
this.periods = periods;
}
void setExpectedValueList(List expectedMetricValues) {
this.expectedMetricValues = expectedMetricValues;
}
void setLowerBoundaryList(List lowerBoundaryValues) {
this.lowerBoundaryValues = lowerBoundaryValues;
}
void setUpperBoundaryList(List upperBoundaryValues) {
this.upperBoundaryValues = upperBoundaryValues;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy