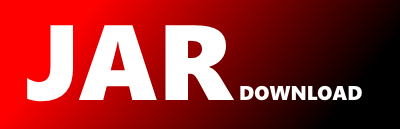
com.azure.communication.callautomation.implementation.models.CallConnectionPropertiesInternal Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-communication-callautomation Show documentation
Show all versions of azure-communication-callautomation Show documentation
This package contains clients and data structures used to make call with Azure Communication Call Automation Service.
For this release, see notes - https://github.com/Azure/azure-sdk-for-java/blob/master/sdk/communication/azure-communication-callautomation/README.md and https://github.com/Azure/azure-sdk-for-java/blob/master/sdk/communication/azure-communication-callautomation/CHANGELOG.md.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.communication.callautomation.implementation.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* Properties of a call connection.
*/
@Fluent
public final class CallConnectionPropertiesInternal implements JsonSerializable {
/*
* The call connection id.
*/
private String callConnectionId;
/*
* The server call id.
*/
private String serverCallId;
/*
* The targets of the call.
*/
private List targets;
/*
* The state of the call connection.
*/
private CallConnectionStateModelInternal callConnectionState;
/*
* The callback URI.
*/
private String callbackUri;
/*
* The source caller Id, a phone number, that's shown to the PSTN participant being invited.
* Required only when calling a PSTN callee.
*/
private PhoneNumberIdentifierModel sourceCallerIdNumber;
/*
* Display name of the call if dialing out to a pstn number.
*/
private String sourceDisplayName;
/*
* Source identity.
*/
private CommunicationIdentifierModel source;
/*
* The correlation ID.
*/
private String correlationId;
/*
* Identity of the answering entity. Only populated when identity is provided in the request.
*/
private CommunicationUserIdentifierModel answeredBy;
/*
* The state of media streaming subscription for the call
*/
private MediaStreamingSubscriptionInternal mediaStreamingSubscription;
/*
* Transcription Subscription.
*/
private TranscriptionSubscriptionInternal transcriptionSubscription;
/*
* Identity of the original Pstn target of an incoming Call. Only populated when the original target is a Pstn number.
*/
private PhoneNumberIdentifierModel answeredFor;
/**
* Creates an instance of CallConnectionPropertiesInternal class.
*/
public CallConnectionPropertiesInternal() {
}
/**
* Get the callConnectionId property: The call connection id.
*
* @return the callConnectionId value.
*/
public String getCallConnectionId() {
return this.callConnectionId;
}
/**
* Set the callConnectionId property: The call connection id.
*
* @param callConnectionId the callConnectionId value to set.
* @return the CallConnectionPropertiesInternal object itself.
*/
public CallConnectionPropertiesInternal setCallConnectionId(String callConnectionId) {
this.callConnectionId = callConnectionId;
return this;
}
/**
* Get the serverCallId property: The server call id.
*
* @return the serverCallId value.
*/
public String getServerCallId() {
return this.serverCallId;
}
/**
* Set the serverCallId property: The server call id.
*
* @param serverCallId the serverCallId value to set.
* @return the CallConnectionPropertiesInternal object itself.
*/
public CallConnectionPropertiesInternal setServerCallId(String serverCallId) {
this.serverCallId = serverCallId;
return this;
}
/**
* Get the targets property: The targets of the call.
*
* @return the targets value.
*/
public List getTargets() {
return this.targets;
}
/**
* Set the targets property: The targets of the call.
*
* @param targets the targets value to set.
* @return the CallConnectionPropertiesInternal object itself.
*/
public CallConnectionPropertiesInternal setTargets(List targets) {
this.targets = targets;
return this;
}
/**
* Get the callConnectionState property: The state of the call connection.
*
* @return the callConnectionState value.
*/
public CallConnectionStateModelInternal getCallConnectionState() {
return this.callConnectionState;
}
/**
* Set the callConnectionState property: The state of the call connection.
*
* @param callConnectionState the callConnectionState value to set.
* @return the CallConnectionPropertiesInternal object itself.
*/
public CallConnectionPropertiesInternal
setCallConnectionState(CallConnectionStateModelInternal callConnectionState) {
this.callConnectionState = callConnectionState;
return this;
}
/**
* Get the callbackUri property: The callback URI.
*
* @return the callbackUri value.
*/
public String getCallbackUri() {
return this.callbackUri;
}
/**
* Set the callbackUri property: The callback URI.
*
* @param callbackUri the callbackUri value to set.
* @return the CallConnectionPropertiesInternal object itself.
*/
public CallConnectionPropertiesInternal setCallbackUri(String callbackUri) {
this.callbackUri = callbackUri;
return this;
}
/**
* Get the sourceCallerIdNumber property: The source caller Id, a phone number, that's shown to the PSTN participant
* being invited.
* Required only when calling a PSTN callee.
*
* @return the sourceCallerIdNumber value.
*/
public PhoneNumberIdentifierModel getSourceCallerIdNumber() {
return this.sourceCallerIdNumber;
}
/**
* Set the sourceCallerIdNumber property: The source caller Id, a phone number, that's shown to the PSTN participant
* being invited.
* Required only when calling a PSTN callee.
*
* @param sourceCallerIdNumber the sourceCallerIdNumber value to set.
* @return the CallConnectionPropertiesInternal object itself.
*/
public CallConnectionPropertiesInternal setSourceCallerIdNumber(PhoneNumberIdentifierModel sourceCallerIdNumber) {
this.sourceCallerIdNumber = sourceCallerIdNumber;
return this;
}
/**
* Get the sourceDisplayName property: Display name of the call if dialing out to a pstn number.
*
* @return the sourceDisplayName value.
*/
public String getSourceDisplayName() {
return this.sourceDisplayName;
}
/**
* Set the sourceDisplayName property: Display name of the call if dialing out to a pstn number.
*
* @param sourceDisplayName the sourceDisplayName value to set.
* @return the CallConnectionPropertiesInternal object itself.
*/
public CallConnectionPropertiesInternal setSourceDisplayName(String sourceDisplayName) {
this.sourceDisplayName = sourceDisplayName;
return this;
}
/**
* Get the source property: Source identity.
*
* @return the source value.
*/
public CommunicationIdentifierModel getSource() {
return this.source;
}
/**
* Set the source property: Source identity.
*
* @param source the source value to set.
* @return the CallConnectionPropertiesInternal object itself.
*/
public CallConnectionPropertiesInternal setSource(CommunicationIdentifierModel source) {
this.source = source;
return this;
}
/**
* Get the correlationId property: The correlation ID.
*
* @return the correlationId value.
*/
public String getCorrelationId() {
return this.correlationId;
}
/**
* Set the correlationId property: The correlation ID.
*
* @param correlationId the correlationId value to set.
* @return the CallConnectionPropertiesInternal object itself.
*/
public CallConnectionPropertiesInternal setCorrelationId(String correlationId) {
this.correlationId = correlationId;
return this;
}
/**
* Get the answeredBy property: Identity of the answering entity. Only populated when identity is provided in the
* request.
*
* @return the answeredBy value.
*/
public CommunicationUserIdentifierModel getAnsweredBy() {
return this.answeredBy;
}
/**
* Set the answeredBy property: Identity of the answering entity. Only populated when identity is provided in the
* request.
*
* @param answeredBy the answeredBy value to set.
* @return the CallConnectionPropertiesInternal object itself.
*/
public CallConnectionPropertiesInternal setAnsweredBy(CommunicationUserIdentifierModel answeredBy) {
this.answeredBy = answeredBy;
return this;
}
/**
* Get the mediaStreamingSubscription property: The state of media streaming subscription for the call.
*
* @return the mediaStreamingSubscription value.
*/
public MediaStreamingSubscriptionInternal getMediaStreamingSubscription() {
return this.mediaStreamingSubscription;
}
/**
* Set the mediaStreamingSubscription property: The state of media streaming subscription for the call.
*
* @param mediaStreamingSubscription the mediaStreamingSubscription value to set.
* @return the CallConnectionPropertiesInternal object itself.
*/
public CallConnectionPropertiesInternal
setMediaStreamingSubscription(MediaStreamingSubscriptionInternal mediaStreamingSubscription) {
this.mediaStreamingSubscription = mediaStreamingSubscription;
return this;
}
/**
* Get the transcriptionSubscription property: Transcription Subscription.
*
* @return the transcriptionSubscription value.
*/
public TranscriptionSubscriptionInternal getTranscriptionSubscription() {
return this.transcriptionSubscription;
}
/**
* Set the transcriptionSubscription property: Transcription Subscription.
*
* @param transcriptionSubscription the transcriptionSubscription value to set.
* @return the CallConnectionPropertiesInternal object itself.
*/
public CallConnectionPropertiesInternal
setTranscriptionSubscription(TranscriptionSubscriptionInternal transcriptionSubscription) {
this.transcriptionSubscription = transcriptionSubscription;
return this;
}
/**
* Get the answeredFor property: Identity of the original Pstn target of an incoming Call. Only populated when the
* original target is a Pstn number.
*
* @return the answeredFor value.
*/
public PhoneNumberIdentifierModel getAnsweredFor() {
return this.answeredFor;
}
/**
* Set the answeredFor property: Identity of the original Pstn target of an incoming Call. Only populated when the
* original target is a Pstn number.
*
* @param answeredFor the answeredFor value to set.
* @return the CallConnectionPropertiesInternal object itself.
*/
public CallConnectionPropertiesInternal setAnsweredFor(PhoneNumberIdentifierModel answeredFor) {
this.answeredFor = answeredFor;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("callConnectionId", this.callConnectionId);
jsonWriter.writeStringField("serverCallId", this.serverCallId);
jsonWriter.writeArrayField("targets", this.targets, (writer, element) -> writer.writeJson(element));
jsonWriter.writeStringField("callConnectionState",
this.callConnectionState == null ? null : this.callConnectionState.toString());
jsonWriter.writeStringField("callbackUri", this.callbackUri);
jsonWriter.writeJsonField("sourceCallerIdNumber", this.sourceCallerIdNumber);
jsonWriter.writeStringField("sourceDisplayName", this.sourceDisplayName);
jsonWriter.writeJsonField("source", this.source);
jsonWriter.writeStringField("correlationId", this.correlationId);
jsonWriter.writeJsonField("answeredBy", this.answeredBy);
jsonWriter.writeJsonField("mediaStreamingSubscription", this.mediaStreamingSubscription);
jsonWriter.writeJsonField("transcriptionSubscription", this.transcriptionSubscription);
jsonWriter.writeJsonField("answeredFor", this.answeredFor);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of CallConnectionPropertiesInternal from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of CallConnectionPropertiesInternal if the JsonReader was pointing to an instance of it, or
* null if it was pointing to JSON null.
* @throws IOException If an error occurs while reading the CallConnectionPropertiesInternal.
*/
public static CallConnectionPropertiesInternal fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
CallConnectionPropertiesInternal deserializedCallConnectionPropertiesInternal
= new CallConnectionPropertiesInternal();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("callConnectionId".equals(fieldName)) {
deserializedCallConnectionPropertiesInternal.callConnectionId = reader.getString();
} else if ("serverCallId".equals(fieldName)) {
deserializedCallConnectionPropertiesInternal.serverCallId = reader.getString();
} else if ("targets".equals(fieldName)) {
List targets
= reader.readArray(reader1 -> CommunicationIdentifierModel.fromJson(reader1));
deserializedCallConnectionPropertiesInternal.targets = targets;
} else if ("callConnectionState".equals(fieldName)) {
deserializedCallConnectionPropertiesInternal.callConnectionState
= CallConnectionStateModelInternal.fromString(reader.getString());
} else if ("callbackUri".equals(fieldName)) {
deserializedCallConnectionPropertiesInternal.callbackUri = reader.getString();
} else if ("sourceCallerIdNumber".equals(fieldName)) {
deserializedCallConnectionPropertiesInternal.sourceCallerIdNumber
= PhoneNumberIdentifierModel.fromJson(reader);
} else if ("sourceDisplayName".equals(fieldName)) {
deserializedCallConnectionPropertiesInternal.sourceDisplayName = reader.getString();
} else if ("source".equals(fieldName)) {
deserializedCallConnectionPropertiesInternal.source = CommunicationIdentifierModel.fromJson(reader);
} else if ("correlationId".equals(fieldName)) {
deserializedCallConnectionPropertiesInternal.correlationId = reader.getString();
} else if ("answeredBy".equals(fieldName)) {
deserializedCallConnectionPropertiesInternal.answeredBy
= CommunicationUserIdentifierModel.fromJson(reader);
} else if ("mediaStreamingSubscription".equals(fieldName)) {
deserializedCallConnectionPropertiesInternal.mediaStreamingSubscription
= MediaStreamingSubscriptionInternal.fromJson(reader);
} else if ("transcriptionSubscription".equals(fieldName)) {
deserializedCallConnectionPropertiesInternal.transcriptionSubscription
= TranscriptionSubscriptionInternal.fromJson(reader);
} else if ("answeredFor".equals(fieldName)) {
deserializedCallConnectionPropertiesInternal.answeredFor
= PhoneNumberIdentifierModel.fromJson(reader);
} else {
reader.skipChildren();
}
}
return deserializedCallConnectionPropertiesInternal;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy