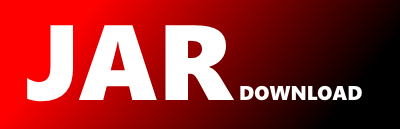
com.azure.communication.callautomation.implementation.models.PlayRequest Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.communication.callautomation.implementation.models;
import com.azure.core.annotation.Fluent;
import com.azure.json.JsonReader;
import com.azure.json.JsonSerializable;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
import java.util.List;
/**
* The PlayRequest model.
*/
@Fluent
public final class PlayRequest implements JsonSerializable {
/*
* The source of the audio to be played.
*/
private List playSources;
/*
* The list of call participants play provided audio to.
* Plays to everyone in the call when not provided.
*/
private List playTo;
/*
* If set play can barge into other existing queued-up/currently-processing requests.
*/
private Boolean interruptCallMediaOperation;
/*
* Defines options for playing the audio.
*/
private PlayOptionsInternal playOptions;
/*
* The value to identify context of the operation.
*/
private String operationContext;
/*
* Set a callback URI that overrides the default callback URI set by CreateCall/AnswerCall for this operation.
* This setup is per-action. If this is not set, the default callback URI set by CreateCall/AnswerCall will be used.
*/
private String operationCallbackUri;
/**
* Creates an instance of PlayRequest class.
*/
public PlayRequest() {
}
/**
* Get the playSources property: The source of the audio to be played.
*
* @return the playSources value.
*/
public List getPlaySources() {
return this.playSources;
}
/**
* Set the playSources property: The source of the audio to be played.
*
* @param playSources the playSources value to set.
* @return the PlayRequest object itself.
*/
public PlayRequest setPlaySources(List playSources) {
this.playSources = playSources;
return this;
}
/**
* Get the playTo property: The list of call participants play provided audio to.
* Plays to everyone in the call when not provided.
*
* @return the playTo value.
*/
public List getPlayTo() {
return this.playTo;
}
/**
* Set the playTo property: The list of call participants play provided audio to.
* Plays to everyone in the call when not provided.
*
* @param playTo the playTo value to set.
* @return the PlayRequest object itself.
*/
public PlayRequest setPlayTo(List playTo) {
this.playTo = playTo;
return this;
}
/**
* Get the interruptCallMediaOperation property: If set play can barge into other existing
* queued-up/currently-processing requests.
*
* @return the interruptCallMediaOperation value.
*/
public Boolean isInterruptCallMediaOperation() {
return this.interruptCallMediaOperation;
}
/**
* Set the interruptCallMediaOperation property: If set play can barge into other existing
* queued-up/currently-processing requests.
*
* @param interruptCallMediaOperation the interruptCallMediaOperation value to set.
* @return the PlayRequest object itself.
*/
public PlayRequest setInterruptCallMediaOperation(Boolean interruptCallMediaOperation) {
this.interruptCallMediaOperation = interruptCallMediaOperation;
return this;
}
/**
* Get the playOptions property: Defines options for playing the audio.
*
* @return the playOptions value.
*/
public PlayOptionsInternal getPlayOptions() {
return this.playOptions;
}
/**
* Set the playOptions property: Defines options for playing the audio.
*
* @param playOptions the playOptions value to set.
* @return the PlayRequest object itself.
*/
public PlayRequest setPlayOptions(PlayOptionsInternal playOptions) {
this.playOptions = playOptions;
return this;
}
/**
* Get the operationContext property: The value to identify context of the operation.
*
* @return the operationContext value.
*/
public String getOperationContext() {
return this.operationContext;
}
/**
* Set the operationContext property: The value to identify context of the operation.
*
* @param operationContext the operationContext value to set.
* @return the PlayRequest object itself.
*/
public PlayRequest setOperationContext(String operationContext) {
this.operationContext = operationContext;
return this;
}
/**
* Get the operationCallbackUri property: Set a callback URI that overrides the default callback URI set by
* CreateCall/AnswerCall for this operation.
* This setup is per-action. If this is not set, the default callback URI set by CreateCall/AnswerCall will be used.
*
* @return the operationCallbackUri value.
*/
public String getOperationCallbackUri() {
return this.operationCallbackUri;
}
/**
* Set the operationCallbackUri property: Set a callback URI that overrides the default callback URI set by
* CreateCall/AnswerCall for this operation.
* This setup is per-action. If this is not set, the default callback URI set by CreateCall/AnswerCall will be used.
*
* @param operationCallbackUri the operationCallbackUri value to set.
* @return the PlayRequest object itself.
*/
public PlayRequest setOperationCallbackUri(String operationCallbackUri) {
this.operationCallbackUri = operationCallbackUri;
return this;
}
/**
* {@inheritDoc}
*/
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeArrayField("playSources", this.playSources, (writer, element) -> writer.writeJson(element));
jsonWriter.writeArrayField("playTo", this.playTo, (writer, element) -> writer.writeJson(element));
jsonWriter.writeBooleanField("interruptCallMediaOperation", this.interruptCallMediaOperation);
jsonWriter.writeJsonField("playOptions", this.playOptions);
jsonWriter.writeStringField("operationContext", this.operationContext);
jsonWriter.writeStringField("operationCallbackUri", this.operationCallbackUri);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of PlayRequest from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of PlayRequest if the JsonReader was pointing to an instance of it, or null if it was
* pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the PlayRequest.
*/
public static PlayRequest fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
PlayRequest deserializedPlayRequest = new PlayRequest();
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("playSources".equals(fieldName)) {
List playSources
= reader.readArray(reader1 -> PlaySourceInternal.fromJson(reader1));
deserializedPlayRequest.playSources = playSources;
} else if ("playTo".equals(fieldName)) {
List playTo
= reader.readArray(reader1 -> CommunicationIdentifierModel.fromJson(reader1));
deserializedPlayRequest.playTo = playTo;
} else if ("interruptCallMediaOperation".equals(fieldName)) {
deserializedPlayRequest.interruptCallMediaOperation = reader.getNullable(JsonReader::getBoolean);
} else if ("playOptions".equals(fieldName)) {
deserializedPlayRequest.playOptions = PlayOptionsInternal.fromJson(reader);
} else if ("operationContext".equals(fieldName)) {
deserializedPlayRequest.operationContext = reader.getString();
} else if ("operationCallbackUri".equals(fieldName)) {
deserializedPlayRequest.operationCallbackUri = reader.getString();
} else {
reader.skipChildren();
}
}
return deserializedPlayRequest;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy