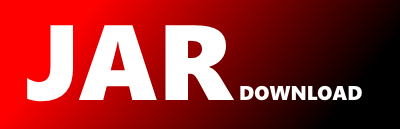
com.azure.communication.jobrouter.JobRouterAdministrationAsyncClient Maven / Gradle / Ivy
Show all versions of azure-communication-jobrouter Show documentation
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) TypeSpec Code Generator.
package com.azure.communication.jobrouter;
import com.azure.communication.jobrouter.implementation.JobRouterAdministrationClientImpl;
import com.azure.communication.jobrouter.implementation.accesshelpers.ClassificationPolicyConstructorProxy;
import com.azure.communication.jobrouter.implementation.accesshelpers.DistributionPolicyConstructorProxy;
import com.azure.communication.jobrouter.implementation.accesshelpers.ExceptionPolicyConstructorProxy;
import com.azure.communication.jobrouter.implementation.accesshelpers.RouterQueueConstructorProxy;
import com.azure.communication.jobrouter.implementation.converters.ClassificationPolicyAdapter;
import com.azure.communication.jobrouter.implementation.converters.DistributionPolicyAdapter;
import com.azure.communication.jobrouter.implementation.converters.ExceptionPolicyAdapter;
import com.azure.communication.jobrouter.implementation.converters.QueueAdapter;
import com.azure.communication.jobrouter.implementation.models.ClassificationPolicyInternal;
import com.azure.communication.jobrouter.implementation.models.DistributionPolicyInternal;
import com.azure.communication.jobrouter.implementation.models.ExceptionPolicyInternal;
import com.azure.communication.jobrouter.implementation.models.RouterQueueInternal;
import com.azure.communication.jobrouter.models.ClassificationPolicy;
import com.azure.communication.jobrouter.models.CreateClassificationPolicyOptions;
import com.azure.communication.jobrouter.models.CreateDistributionPolicyOptions;
import com.azure.communication.jobrouter.models.CreateExceptionPolicyOptions;
import com.azure.communication.jobrouter.models.CreateQueueOptions;
import com.azure.communication.jobrouter.models.DistributionPolicy;
import com.azure.communication.jobrouter.models.ExceptionPolicy;
import com.azure.communication.jobrouter.models.RouterQueue;
import com.azure.core.annotation.Generated;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceClient;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.exception.ClientAuthenticationException;
import com.azure.core.exception.HttpResponseException;
import com.azure.core.exception.ResourceModifiedException;
import com.azure.core.exception.ResourceNotFoundException;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.http.rest.PagedResponse;
import com.azure.core.http.rest.PagedResponseBase;
import com.azure.core.http.rest.RequestOptions;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.SimpleResponse;
import com.azure.core.util.BinaryData;
import com.azure.core.util.FluxUtil;
import java.util.stream.Collectors;
import reactor.core.publisher.Flux;
import reactor.core.publisher.Mono;
/**
* Initializes a new instance of the asynchronous JobRouterAdministrationClient type.
*/
@ServiceClient(builder = JobRouterAdministrationClientBuilder.class, isAsync = true)
public final class JobRouterAdministrationAsyncClient {
@Generated
private final JobRouterAdministrationClientImpl serviceClient;
/**
* Initializes an instance of JobRouterAdministrationAsyncClient class.
*
* @param serviceClient the service client implementation.
*/
@Generated
JobRouterAdministrationAsyncClient(JobRouterAdministrationClientImpl serviceClient) {
this.serviceClient = serviceClient;
}
/**
* Creates or updates a distribution policy.
*
* Header Parameters
*
*
* Header Parameters
*
* Name
* Type
* Required
* Description
*
*
* If-Match
* String
* No
* The request should only proceed if an entity matches this string.
*
*
* If-Unmodified-Since
* OffsetDateTime
* No
* The request should only proceed if the entity was not modified after this time.
*
*
* You can add these to a request with {@link RequestOptions#addHeader}
*
* Request Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* name: String (Optional)
* offerExpiresAfterSeconds: Double (Optional)
* mode (Optional): {
* minConcurrentOffers: Integer (Optional)
* maxConcurrentOffers: Integer (Optional)
* bypassSelectors: Boolean (Optional)
* }
* }
* }
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* name: String (Optional)
* offerExpiresAfterSeconds: Double (Optional)
* mode (Optional): {
* minConcurrentOffers: Integer (Optional)
* maxConcurrentOffers: Integer (Optional)
* bypassSelectors: Boolean (Optional)
* }
* }
* }
*
* @param distributionPolicyId Id of a distribution policy.
* @param resource The resource instance.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return policy governing how jobs are distributed to workers along with {@link Response} on successful completion
* of {@link Mono}.
*/
@Generated
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> upsertDistributionPolicyWithResponse(String distributionPolicyId, BinaryData resource,
RequestOptions requestOptions) {
// Convenience API is not generated, as operation 'upsertDistributionPolicy' is 'application/merge-patch+json'
// and stream-style-serialization is not enabled
return this.serviceClient.upsertDistributionPolicyWithResponseAsync(distributionPolicyId, resource,
requestOptions);
}
/**
* Updates a distribution policy.
*
*
* Header Parameters
*
*
* Header Parameters
*
* Name
* Type
* Required
* Description
*
*
* If-Match
* String
* No
* The request should only proceed if an entity matches this string.
*
*
* If-Unmodified-Since
* OffsetDateTime
* No
* The request should only proceed if the entity was not modified after this time.
*
*
*
* You can add these to a request with {@link RequestOptions#addHeader}
*
*
* Request Body Schema
*
*
{@code
* {
* distributionPolicyId: String (Required)
* name: String (Optional)
* offerExpiresAfterSeconds: Double (Optional)
* mode (Optional): {
* minConcurrentOffers: Integer (Optional)
* maxConcurrentOffers: Integer (Optional)
* bypassSelectors: Boolean (Optional)
* }
* }
* }
*
*
* Response Body Schema
*
*
{@code
* {
* distributionPolicyId: String (Required)
* name: String (Optional)
* offerExpiresAfterSeconds: Double (Optional)
* mode (Optional): {
* minConcurrentOffers: Integer (Optional)
* maxConcurrentOffers: Integer (Optional)
* bypassSelectors: Boolean (Optional)
* }
* }
* }
*
* @param distributionPolicyId The unique identifier of the policy.
* @param resource The resource instance.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return policy governing how jobs are distributed to workers along with {@link Response} on successful completion
* of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> updateDistributionPolicyWithResponse(String distributionPolicyId,
BinaryData resource, RequestOptions requestOptions) {
return this.serviceClient.upsertDistributionPolicyWithResponseAsync(distributionPolicyId, resource,
requestOptions);
}
/**
* Updates a distribution policy.
*
*
* Header Parameters
*
*
* Header Parameters
*
* Name
* Type
* Required
* Description
*
*
* If-Match
* String
* No
* The request should only proceed if an entity matches this string.
*
*
* If-Unmodified-Since
* OffsetDateTime
* No
* The request should only proceed if the entity was not modified after this time.
*
*
*
* You can add these to a request with {@link RequestOptions#addHeader}
*
*
* Request Body Schema
*
*
{@code
* {
* distributionPolicyId: String (Required)
* name: String (Optional)
* offerExpiresAfterSeconds: Double (Optional)
* mode (Optional): {
* minConcurrentOffers: Integer (Optional)
* maxConcurrentOffers: Integer (Optional)
* bypassSelectors: Boolean (Optional)
* }
* }
* }
*
*
* Response Body Schema
*
*
{@code
* {
* distributionPolicyId: String (Required)
* name: String (Optional)
* offerExpiresAfterSeconds: Double (Optional)
* mode (Optional): {
* minConcurrentOffers: Integer (Optional)
* maxConcurrentOffers: Integer (Optional)
* bypassSelectors: Boolean (Optional)
* }
* }
* }
*
* @param distributionPolicyId The unique identifier of the policy.
* @param resource The resource instance.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @return policy governing how jobs are distributed to workers along with {@link Response} on successful completion
* of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono updateDistributionPolicy(String distributionPolicyId, BinaryData resource,
RequestOptions requestOptions) {
return this.updateDistributionPolicyWithResponse(distributionPolicyId, resource, requestOptions)
.map(response -> response.getValue());
}
/**
* Creates a distribution policy.
*
* @param createDistributionPolicyOptions Container for inputs to create a distribution policy.
* @return response The response instance.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>
createDistributionPolicyWithResponse(CreateDistributionPolicyOptions createDistributionPolicyOptions) {
RequestOptions requestOptions = new RequestOptions();
DistributionPolicyInternal distributionPolicy
= DistributionPolicyAdapter.convertCreateOptionsToDistributionPolicy(createDistributionPolicyOptions);
return upsertDistributionPolicyWithResponse(createDistributionPolicyOptions.getDistributionPolicyId(),
BinaryData.fromObject(distributionPolicy), requestOptions)
.map(response -> new SimpleResponse(response.getRequest(), response.getStatusCode(),
response.getHeaders(), DistributionPolicyConstructorProxy
.create(response.getValue().toObject(DistributionPolicyInternal.class))));
}
/**
* Creates a distribution policy.
*
* @param createDistributionPolicyOptions Container for inputs to create a distribution policy.
* @return response The response instance.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono
createDistributionPolicy(CreateDistributionPolicyOptions createDistributionPolicyOptions) {
return createDistributionPolicyWithResponse(createDistributionPolicyOptions)
.map(response -> response.getValue());
}
/**
* Retrieves an existing distribution policy by Id.
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* name: String (Optional)
* offerExpiresAfterSeconds: Double (Optional)
* mode (Optional): {
* minConcurrentOffers: Integer (Optional)
* maxConcurrentOffers: Integer (Optional)
* bypassSelectors: Boolean (Optional)
* }
* }
* }
*
* @param distributionPolicyId Id of a distribution policy.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return policy governing how jobs are distributed to workers along with {@link Response} on successful completion
* of {@link Mono}.
*/
@Generated
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getDistributionPolicyWithResponse(String distributionPolicyId,
RequestOptions requestOptions) {
return this.serviceClient.getDistributionPolicyWithResponseAsync(distributionPolicyId, requestOptions);
}
/**
* Retrieves existing distribution policies.
*
* Query Parameters
*
*
* Query Parameters
*
* Name
* Type
* Required
* Description
*
*
* maxpagesize
* Integer
* No
* Number of objects to return per page.
*
*
* You can add these to a request with {@link RequestOptions#addQueryParam}
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* name: String (Optional)
* offerExpiresAfterSeconds: Double (Optional)
* mode (Optional): {
* minConcurrentOffers: Integer (Optional)
* maxConcurrentOffers: Integer (Optional)
* bypassSelectors: Boolean (Optional)
* }
* }
* }
*
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return paged collection of DistributionPolicy items as paginated response with {@link PagedFlux}.
*/
@Generated
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listDistributionPolicies(RequestOptions requestOptions) {
return this.serviceClient.listDistributionPoliciesAsync(requestOptions);
}
/**
* Delete a distribution policy by Id.
*
* @param distributionPolicyId Id of a distribution policy.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@Generated
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> deleteDistributionPolicyWithResponse(String distributionPolicyId,
RequestOptions requestOptions) {
return this.serviceClient.deleteDistributionPolicyWithResponseAsync(distributionPolicyId, requestOptions);
}
/**
* Creates or updates a classification policy.
*
* Header Parameters
*
*
* Header Parameters
*
* Name
* Type
* Required
* Description
*
*
* If-Match
* String
* No
* The request should only proceed if an entity matches this string.
*
*
* If-Unmodified-Since
* OffsetDateTime
* No
* The request should only proceed if the entity was not modified after this time.
*
*
* You can add these to a request with {@link RequestOptions#addHeader}
*
* Request Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* name: String (Optional)
* fallbackQueueId: String (Optional)
* queueSelectorAttachments (Optional): [
* (Optional){
* }
* ]
* prioritizationRule (Optional): {
* }
* workerSelectorAttachments (Optional): [
* (Optional){
* }
* ]
* }
* }
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* name: String (Optional)
* fallbackQueueId: String (Optional)
* queueSelectorAttachments (Optional): [
* (Optional){
* }
* ]
* prioritizationRule (Optional): {
* }
* workerSelectorAttachments (Optional): [
* (Optional){
* }
* ]
* }
* }
*
* @param classificationPolicyId Id of a classification policy.
* @param resource The resource instance.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return a container for the rules that govern how jobs are classified along with {@link Response} on successful
* completion of {@link Mono}.
*/
@Generated
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> upsertClassificationPolicyWithResponse(String classificationPolicyId,
BinaryData resource, RequestOptions requestOptions) {
// Convenience API is not generated, as operation 'upsertClassificationPolicy' is 'application/merge-patch+json'
// and stream-style-serialization is not enabled
return this.serviceClient.upsertClassificationPolicyWithResponseAsync(classificationPolicyId, resource,
requestOptions);
}
/**
* Updates a classification policy.
*
*
* Header Parameters
*
*
* Header Parameters
*
* Name
* Type
* Required
* Description
*
*
* If-Match
* String
* No
* The request should only proceed if an entity matches this string.
*
*
* If-Unmodified-Since
* OffsetDateTime
* No
* The request should only proceed if the entity was not modified after this time.
*
*
*
* You can add these to a request with {@link RequestOptions#addHeader}
*
*
* Request Body Schema
*
*
{@code
* {
* classificationPolicyId: String (Required)
* name: String (Optional)
* fallbackQueueId: String (Optional)
* queueSelectors (Optional): [
* (Optional){
* }
* ]
* prioritizationRule (Optional): {
* }
* workerSelectors (Optional): [
* (Optional){
* }
* ]
* }
* }
*
*
* Response Body Schema
*
*
{@code
* {
* classificationPolicyId: String (Required)
* name: String (Optional)
* fallbackQueueId: String (Optional)
* queueSelectors (Optional): [
* (Optional){
* }
* ]
* prioritizationRule (Optional): {
* }
* workerSelectors (Optional): [
* (Optional){
* }
* ]
* }
* }
*
* @param classificationPolicyId Unique identifier of this policy.
* @param resource The resource instance.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return a container for the rules that govern how jobs are classified along with {@link Response} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> updateClassificationPolicyWithResponse(String classificationPolicyId,
BinaryData resource, RequestOptions requestOptions) {
return this.serviceClient.upsertClassificationPolicyWithResponseAsync(classificationPolicyId, resource,
requestOptions);
}
/**
* Updates a classification policy.
*
*
* Header Parameters
*
*
* Header Parameters
*
* Name
* Type
* Required
* Description
*
*
* If-Match
* String
* No
* The request should only proceed if an entity matches this string.
*
*
* If-Unmodified-Since
* OffsetDateTime
* No
* The request should only proceed if the entity was not modified after this time.
*
*
*
* You can add these to a request with {@link RequestOptions#addHeader}
*
*
* Request Body Schema
*
*
{@code
* {
* classificationPolicyId: String (Required)
* name: String (Optional)
* fallbackQueueId: String (Optional)
* queueSelectors (Optional): [
* (Optional){
* }
* ]
* prioritizationRule (Optional): {
* }
* workerSelectors (Optional): [
* (Optional){
* }
* ]
* }
* }
*
*
* Response Body Schema
*
*
{@code
* {
* classificationPolicyId: String (Required)
* name: String (Optional)
* fallbackQueueId: String (Optional)
* queueSelectors (Optional): [
* (Optional){
* }
* ]
* prioritizationRule (Optional): {
* }
* workerSelectors (Optional): [
* (Optional){
* }
* ]
* }
* }
*
* @param classificationPolicyId Unique identifier of this policy.
* @param resource The resource instance.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @return result object.
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono updateClassificationPolicy(String classificationPolicyId, BinaryData resource,
RequestOptions requestOptions) {
return this.updateClassificationPolicyWithResponse(classificationPolicyId, resource, requestOptions)
.map(response -> response.getValue());
}
/**
* Creates a classification policy.
*
* @param createClassificationPolicyOptions Container for inputs to create a classification policy.
* @return response The response instance.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>
createClassificationPolicyWithResponse(CreateClassificationPolicyOptions createClassificationPolicyOptions) {
RequestOptions requestOptions = new RequestOptions();
ClassificationPolicyInternal classificationPolicy = ClassificationPolicyAdapter
.convertCreateOptionsToClassificationPolicyInternal(createClassificationPolicyOptions);
return upsertClassificationPolicyWithResponse(createClassificationPolicyOptions.getClassificationPolicyId(),
BinaryData.fromObject(classificationPolicy), requestOptions)
.map(response -> new SimpleResponse(response.getRequest(),
response.getStatusCode(), response.getHeaders(), ClassificationPolicyConstructorProxy
.create(response.getValue().toObject(ClassificationPolicyInternal.class))));
}
/**
* Convenience method to create a classification policy.
*
* @param createClassificationPolicyOptions Container for inputs to create a classification policy.
* @return a container for the rules that govern how jobs are classified.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono
createClassificationPolicy(CreateClassificationPolicyOptions createClassificationPolicyOptions) {
return createClassificationPolicyWithResponse(createClassificationPolicyOptions)
.map(response -> response.getValue());
}
/**
* Retrieves an existing classification policy by Id.
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* name: String (Optional)
* fallbackQueueId: String (Optional)
* queueSelectorAttachments (Optional): [
* (Optional){
* }
* ]
* prioritizationRule (Optional): {
* }
* workerSelectorAttachments (Optional): [
* (Optional){
* }
* ]
* }
* }
*
* @param classificationPolicyId Id of a classification policy.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return a container for the rules that govern how jobs are classified along with {@link Response} on successful
* completion of {@link Mono}.
*/
@Generated
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getClassificationPolicyWithResponse(String classificationPolicyId,
RequestOptions requestOptions) {
return this.serviceClient.getClassificationPolicyWithResponseAsync(classificationPolicyId, requestOptions);
}
/**
* Retrieves existing classification policies.
*
* Query Parameters
*
*
* Query Parameters
*
* Name
* Type
* Required
* Description
*
*
* maxpagesize
* Integer
* No
* Number of objects to return per page.
*
*
* You can add these to a request with {@link RequestOptions#addQueryParam}
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* name: String (Optional)
* fallbackQueueId: String (Optional)
* queueSelectorAttachments (Optional): [
* (Optional){
* }
* ]
* prioritizationRule (Optional): {
* }
* workerSelectorAttachments (Optional): [
* (Optional){
* }
* ]
* }
* }
*
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return paged collection of ClassificationPolicy items as paginated response with {@link PagedFlux}.
*/
@Generated
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listClassificationPolicies(RequestOptions requestOptions) {
return this.serviceClient.listClassificationPoliciesAsync(requestOptions);
}
/**
* Delete a classification policy by Id.
*
* @param classificationPolicyId Id of a classification policy.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@Generated
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> deleteClassificationPolicyWithResponse(String classificationPolicyId,
RequestOptions requestOptions) {
return this.serviceClient.deleteClassificationPolicyWithResponseAsync(classificationPolicyId, requestOptions);
}
/**
* Creates or updates a exception policy.
*
* Header Parameters
*
*
* Header Parameters
*
* Name
* Type
* Required
* Description
*
*
* If-Match
* String
* No
* The request should only proceed if an entity matches this string.
*
*
* If-Unmodified-Since
* OffsetDateTime
* No
* The request should only proceed if the entity was not modified after this time.
*
*
* You can add these to a request with {@link RequestOptions#addHeader}
*
* Request Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* name: String (Optional)
* exceptionRules (Optional): [
* (Optional){
* id: String (Required)
* trigger (Required): {
* }
* actions (Required): [
* (Required){
* id: String (Optional)
* }
* ]
* }
* ]
* }
* }
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* name: String (Optional)
* exceptionRules (Optional): [
* (Optional){
* id: String (Required)
* trigger (Required): {
* }
* actions (Required): [
* (Required){
* id: String (Optional)
* }
* ]
* }
* ]
* }
* }
*
* @param exceptionPolicyId Id of an exception policy.
* @param resource The resource instance.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return a policy that defines actions to execute when exception are triggered along with {@link Response} on
* successful completion of {@link Mono}.
*/
@Generated
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> upsertExceptionPolicyWithResponse(String exceptionPolicyId, BinaryData resource,
RequestOptions requestOptions) {
// Convenience API is not generated, as operation 'upsertExceptionPolicy' is 'application/merge-patch+json' and
// stream-style-serialization is not enabled
return this.serviceClient.upsertExceptionPolicyWithResponseAsync(exceptionPolicyId, resource, requestOptions);
}
/**
* Updates a exception policy.
*
*
* Header Parameters
*
*
* Header Parameters
*
* Name
* Type
* Required
* Description
*
*
* If-Match
* String
* No
* The request should only proceed if an entity matches this string.
*
*
* If-Unmodified-Since
* OffsetDateTime
* No
* The request should only proceed if the entity was not modified after this time.
*
*
*
* You can add these to a request with {@link RequestOptions#addHeader}
*
*
* Request Body Schema
*
*
{@code
* {
* exceptionPolicyId: String (Required)
* name: String (Optional)
* exceptionRules (Optional): {
* String (Optional): {
* trigger (Required): {
* }
* actions (Required): {
* String (Required): {
* }
* }
* }
* }
* }
* }
*
*
* Response Body Schema
*
*
{@code
* {
* exceptionPolicyId: String (Required)
* name: String (Optional)
* exceptionRules (Optional): {
* String (Optional): {
* trigger (Required): {
* }
* actions (Required): {
* String (Required): {
* }
* }
* }
* }
* }
* }
*
* @param exceptionPolicyId The Id of the exception policy.
* @param resource The resource instance.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return a policy that defines actions to execute when exception are triggered along with {@link Response} on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> updateExceptionPolicyWithResponse(String exceptionPolicyId, BinaryData resource,
RequestOptions requestOptions) {
return this.serviceClient.upsertExceptionPolicyWithResponseAsync(exceptionPolicyId, resource, requestOptions);
}
/**
* Updates a exception policy.
*
*
* Header Parameters
*
*
* Header Parameters
*
* Name
* Type
* Required
* Description
*
*
* If-Match
* String
* No
* The request should only proceed if an entity matches this string.
*
*
* If-Unmodified-Since
* OffsetDateTime
* No
* The request should only proceed if the entity was not modified after this time.
*
*
*
* You can add these to a request with {@link RequestOptions#addHeader}
*
*
* Request Body Schema
*
*
{@code
* {
* exceptionPolicyId: String (Required)
* name: String (Optional)
* exceptionRules (Optional): {
* String (Optional): {
* trigger (Required): {
* }
* actions (Required): {
* String (Required): {
* }
* }
* }
* }
* }
* }
*
*
* Response Body Schema
*
*
{@code
* {
* exceptionPolicyId: String (Required)
* name: String (Optional)
* exceptionRules (Optional): {
* String (Optional): {
* trigger (Required): {
* }
* actions (Required): {
* String (Required): {
* }
* }
* }
* }
* }
* }
*
* @param exceptionPolicyId The Id of the exception policy.
* @param resource The resource instance.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @return a policy that defines actions to execute when exception are triggered along with {@link Response} on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono updateExceptionPolicy(String exceptionPolicyId, BinaryData resource,
RequestOptions requestOptions) {
return this.updateExceptionPolicyWithResponse(exceptionPolicyId, resource, requestOptions)
.map(response -> response.getValue());
}
/**
* Creates an exception policy.
*
* @param createExceptionPolicyOptions Create options for Exception Policy.
* @return response The response instance.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono>
createExceptionPolicyWithResponse(CreateExceptionPolicyOptions createExceptionPolicyOptions) {
RequestOptions requestOptions = new RequestOptions();
ExceptionPolicyInternal exceptionPolicy
= ExceptionPolicyAdapter.convertCreateOptionsToExceptionPolicy(createExceptionPolicyOptions);
return upsertExceptionPolicyWithResponse(createExceptionPolicyOptions.getExceptionPolicyId(),
BinaryData.fromObject(exceptionPolicy), requestOptions)
.map(response -> new SimpleResponse(response.getRequest(), response.getStatusCode(),
response.getHeaders(), ExceptionPolicyConstructorProxy
.create(response.getValue().toObject(ExceptionPolicyInternal.class))));
}
/**
* Creates an exception policy.
*
* @param createExceptionPolicyOptions Create options for Exception Policy.
* @return response The response instance.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono createExceptionPolicy(CreateExceptionPolicyOptions createExceptionPolicyOptions) {
return createExceptionPolicyWithResponse(createExceptionPolicyOptions).map(response -> response.getValue());
}
/**
* Retrieves an existing exception policy by Id.
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* name: String (Optional)
* exceptionRules (Optional): [
* (Optional){
* id: String (Required)
* trigger (Required): {
* }
* actions (Required): [
* (Required){
* id: String (Optional)
* }
* ]
* }
* ]
* }
* }
*
* @param exceptionPolicyId Id of an exception policy.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return a policy that defines actions to execute when exception are triggered along with {@link Response} on
* successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getExceptionPolicyWithResponse(String exceptionPolicyId,
RequestOptions requestOptions) {
return this.serviceClient.getExceptionPolicyWithResponseAsync(exceptionPolicyId, requestOptions);
}
/**
* Retrieves existing exception policies.
*
* Query Parameters
*
*
* Query Parameters
*
* Name
* Type
* Required
* Description
*
*
* maxpagesize
* Integer
* No
* Number of objects to return per page.
*
*
* You can add these to a request with {@link RequestOptions#addQueryParam}
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* name: String (Optional)
* exceptionRules (Optional): [
* (Optional){
* id: String (Required)
* trigger (Required): {
* }
* actions (Required): [
* (Required){
* id: String (Optional)
* }
* ]
* }
* ]
* }
* }
*
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return paged collection of ExceptionPolicy items as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listExceptionPolicies(RequestOptions requestOptions) {
return this.serviceClient.listExceptionPoliciesAsync(requestOptions);
}
/**
* Deletes a exception policy by Id.
*
* @param exceptionPolicyId Id of an exception policy.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@Generated
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> deleteExceptionPolicyWithResponse(String exceptionPolicyId,
RequestOptions requestOptions) {
return this.serviceClient.deleteExceptionPolicyWithResponseAsync(exceptionPolicyId, requestOptions);
}
/**
* Creates or updates a queue.
*
* Header Parameters
*
*
* Header Parameters
*
* Name
* Type
* Required
* Description
*
*
* If-Match
* String
* No
* The request should only proceed if an entity matches this string.
*
*
* If-Unmodified-Since
* OffsetDateTime
* No
* The request should only proceed if the entity was not modified after this time.
*
*
* You can add these to a request with {@link RequestOptions#addHeader}
*
* Request Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* name: String (Optional)
* distributionPolicyId: String (Optional)
* labels (Optional): {
* String: Object (Required)
* }
* exceptionPolicyId: String (Optional)
* }
* }
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* name: String (Optional)
* distributionPolicyId: String (Optional)
* labels (Optional): {
* String: Object (Required)
* }
* exceptionPolicyId: String (Optional)
* }
* }
*
* @param queueId Id of a queue.
* @param resource The resource instance.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return a queue that can contain jobs to be routed along with {@link Response} on successful completion of
* {@link Mono}.
*/
@Generated
@ServiceMethod(returns = ReturnType.SINGLE)
Mono> upsertQueueWithResponse(String queueId, BinaryData resource,
RequestOptions requestOptions) {
// Convenience API is not generated, as operation 'upsertQueue' is 'application/merge-patch+json' and
// stream-style-serialization is not enabled
return this.serviceClient.upsertQueueWithResponseAsync(queueId, resource, requestOptions);
}
/**
* Updates a queue.
*
*
* Header Parameters
*
*
* Header Parameters
*
* Name
* Type
* Required
* Description
*
*
* If-Match
* String
* No
* The request should only proceed if an entity matches this string.
*
*
* If-Unmodified-Since
* OffsetDateTime
* No
* The request should only proceed if the entity was not modified after this time.
*
*
*
* You can add these to a request with {@link RequestOptions#addHeader}
*
*
* Request Body Schema
*
*
{@code
* {
* queueId: String (Required)
* name: String (Optional)
* distributionPolicyId: String (Optional)
* labels (Optional): {
* String: Object (Optional)
* }
* exceptionPolicyId: String (Optional)
* }
* }
*
*
* Response Body Schema
*
*
{@code
* {
* queueId: String (Required)
* name: String (Optional)
* distributionPolicyId: String (Optional)
* labels (Optional): {
* String: Object (Optional)
* }
* exceptionPolicyId: String (Optional)
* }
* }
*
* @param queueId The Id of this queue.
* @param resource The resource instance.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return a queue that can contain jobs to be routed along with {@link Response} on successful completion of {@link
* Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> updateQueueWithResponse(String queueId, BinaryData resource,
RequestOptions requestOptions) {
return this.serviceClient.upsertQueueWithResponseAsync(queueId, resource, requestOptions);
}
/**
* Updates a queue.
*
*
* Header Parameters
*
*
* Header Parameters
*
* Name
* Type
* Required
* Description
*
*
* If-Match
* String
* No
* The request should only proceed if an entity matches this string.
*
*
* If-Unmodified-Since
* OffsetDateTime
* No
* The request should only proceed if the entity was not modified after this time.
*
*
*
* You can add these to a request with {@link RequestOptions#addHeader}
*
*
* Request Body Schema
*
*
{@code
* {
* queueId: String (Required)
* name: String (Optional)
* distributionPolicyId: String (Optional)
* labels (Optional): {
* String: Object (Optional)
* }
* exceptionPolicyId: String (Optional)
* }
* }
*
*
* Response Body Schema
*
*
{@code
* {
* queueId: String (Required)
* name: String (Optional)
* distributionPolicyId: String (Optional)
* labels (Optional): {
* String: Object (Optional)
* }
* exceptionPolicyId: String (Optional)
* }
* }
*
* @param queueId The Id of this queue.
* @param resource The resource instance.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @return result object.
* Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono updateQueue(String queueId, BinaryData resource, RequestOptions requestOptions) {
return this.upsertQueueWithResponse(queueId, resource, requestOptions).map(response -> response.getValue());
}
/**
* Create a queue.
*
* @param createQueueOptions Container for inputs to create a queue.
* @return response The response instance.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> createQueueWithResponse(CreateQueueOptions createQueueOptions) {
RequestOptions requestOptions = new RequestOptions();
RouterQueueInternal queue = QueueAdapter.convertCreateQueueOptionsToRouterQueueInternal(createQueueOptions);
return upsertQueueWithResponse(createQueueOptions.getQueueId(), BinaryData.fromObject(queue), requestOptions)
.map(response -> new SimpleResponse(response.getRequest(), response.getStatusCode(),
response.getHeaders(),
RouterQueueConstructorProxy.create(response.getValue().toObject(RouterQueueInternal.class))));
}
/**
* Create a queue.
*
* @param createQueueOptions Container for inputs to create a queue.
* @return response The response instance.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono createQueue(CreateQueueOptions createQueueOptions) {
RequestOptions requestOptions = new RequestOptions();
RouterQueueInternal queue = QueueAdapter.convertCreateQueueOptionsToRouterQueueInternal(createQueueOptions);
return upsertQueueWithResponse(createQueueOptions.getQueueId(), BinaryData.fromObject(queue), requestOptions)
.map(response -> response.getValue().toObject(RouterQueueInternal.class))
.map(internal -> RouterQueueConstructorProxy.create(internal));
}
/**
* Retrieves an existing queue by Id.
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* name: String (Optional)
* distributionPolicyId: String (Optional)
* labels (Optional): {
* String: Object (Required)
* }
* exceptionPolicyId: String (Optional)
* }
* }
*
* @param queueId Id of a queue.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return a queue that can contain jobs to be routed along with {@link Response} on successful completion of
* {@link Mono}.
*/
@Generated
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getQueueWithResponse(String queueId, RequestOptions requestOptions) {
return this.serviceClient.getQueueWithResponseAsync(queueId, requestOptions);
}
/**
* Retrieves existing queues.
*
* Query Parameters
*
*
* Query Parameters
*
* Name
* Type
* Required
* Description
*
*
* maxpagesize
* Integer
* No
* Number of objects to return per page.
*
*
* You can add these to a request with {@link RequestOptions#addQueryParam}
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* name: String (Optional)
* distributionPolicyId: String (Optional)
* labels (Optional): {
* String: Object (Required)
* }
* exceptionPolicyId: String (Optional)
* }
* }
*
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return paged collection of RouterQueue items as paginated response with {@link PagedFlux}.
*/
@Generated
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listQueues(RequestOptions requestOptions) {
return this.serviceClient.listQueuesAsync(requestOptions);
}
/**
* Deletes a queue by Id.
*
* @param queueId Id of a queue.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@Generated
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> deleteQueueWithResponse(String queueId, RequestOptions requestOptions) {
return this.serviceClient.deleteQueueWithResponseAsync(queueId, requestOptions);
}
/**
* Retrieves an existing distribution policy by Id.
*
* @param distributionPolicyId The unique identifier of the policy.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return policy governing how jobs are distributed to workers on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getDistributionPolicy(String distributionPolicyId) {
// Generated convenience method for getDistributionPolicyWithResponse
RequestOptions requestOptions = new RequestOptions();
return getDistributionPolicyWithResponse(distributionPolicyId, requestOptions).flatMap(FluxUtil::toMono)
.map(protocolMethodData -> protocolMethodData.toObject(DistributionPolicy.class));
}
/**
* Retrieves existing distribution policies.
*
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a paged collection of distribution policies as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listDistributionPolicies() {
// Generated convenience method for listDistributionPolicies
RequestOptions requestOptions = new RequestOptions();
PagedFlux pagedFluxResponse = listDistributionPolicies(requestOptions);
return PagedFlux.create(() -> (continuationToken, pageSize) -> {
Flux> flux = (continuationToken == null)
? pagedFluxResponse.byPage().take(1)
: pagedFluxResponse.byPage(continuationToken).take(1);
return flux.map(pagedResponse -> new PagedResponseBase(pagedResponse.getRequest(),
pagedResponse.getStatusCode(), pagedResponse.getHeaders(),
pagedResponse.getValue()
.stream()
.map(protocolMethodData -> protocolMethodData.toObject(DistributionPolicy.class))
.collect(Collectors.toList()),
pagedResponse.getContinuationToken(), null));
});
}
/**
* Delete a distribution policy by Id.
*
* @param distributionPolicyId Id of a distribution policy.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@Generated
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono deleteDistributionPolicy(String distributionPolicyId) {
// Generated convenience method for deleteDistributionPolicyWithResponse
RequestOptions requestOptions = new RequestOptions();
return deleteDistributionPolicyWithResponse(distributionPolicyId, requestOptions).flatMap(FluxUtil::toMono);
}
/**
* Retrieves an existing classification policy by Id.
*
* @param classificationPolicyId Unique identifier of this policy.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a container for the rules that govern how jobs are classified on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getClassificationPolicy(String classificationPolicyId) {
// Generated convenience method for getClassificationPolicyWithResponse
RequestOptions requestOptions = new RequestOptions();
return getClassificationPolicyWithResponse(classificationPolicyId, requestOptions).flatMap(FluxUtil::toMono)
.map(protocolMethodData -> protocolMethodData.toObject(ClassificationPolicy.class));
}
/**
* Retrieves existing classification policies.
*
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a paged collection of classification policies as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listClassificationPolicies() {
// Generated convenience method for listClassificationPolicies
RequestOptions requestOptions = new RequestOptions();
PagedFlux pagedFluxResponse = listClassificationPolicies(requestOptions);
return PagedFlux.create(() -> (continuationToken, pageSize) -> {
Flux> flux = (continuationToken == null)
? pagedFluxResponse.byPage().take(1)
: pagedFluxResponse.byPage(continuationToken).take(1);
return flux
.map(pagedResponse -> new PagedResponseBase(pagedResponse.getRequest(),
pagedResponse.getStatusCode(), pagedResponse.getHeaders(),
pagedResponse.getValue()
.stream()
.map(protocolMethodData -> protocolMethodData.toObject(ClassificationPolicy.class))
.collect(Collectors.toList()),
pagedResponse.getContinuationToken(), null));
});
}
/**
* Delete a classification policy by Id.
*
* @param classificationPolicyId Id of a classification policy.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@Generated
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono deleteClassificationPolicy(String classificationPolicyId) {
// Generated convenience method for deleteClassificationPolicyWithResponse
RequestOptions requestOptions = new RequestOptions();
return deleteClassificationPolicyWithResponse(classificationPolicyId, requestOptions).flatMap(FluxUtil::toMono);
}
/**
* Retrieves an existing exception policy by Id.
*
* @param exceptionPolicyId Id of an exception policy.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a policy that defines actions to execute when exception are triggered on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getExceptionPolicy(String exceptionPolicyId) {
// Generated convenience method for getExceptionPolicyWithResponse
RequestOptions requestOptions = new RequestOptions();
return getExceptionPolicyWithResponse(exceptionPolicyId, requestOptions).flatMap(FluxUtil::toMono)
.map(protocolMethodData -> protocolMethodData.toObject(ExceptionPolicy.class));
}
/**
* Retrieves existing exception policies.
*
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return paged collection of ExceptionPolicy items as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listExceptionPolicies() {
// Generated convenience method for listExceptionPolicies
RequestOptions requestOptions = new RequestOptions();
PagedFlux pagedFluxResponse = listExceptionPolicies(requestOptions);
return PagedFlux.create(() -> (continuationToken, pageSize) -> {
Flux> flux = (continuationToken == null)
? pagedFluxResponse.byPage().take(1)
: pagedFluxResponse.byPage(continuationToken).take(1);
return flux.map(pagedResponse -> new PagedResponseBase(pagedResponse.getRequest(),
pagedResponse.getStatusCode(), pagedResponse.getHeaders(),
pagedResponse.getValue()
.stream()
.map(protocolMethodData -> protocolMethodData.toObject(ExceptionPolicy.class))
.collect(Collectors.toList()),
pagedResponse.getContinuationToken(), null));
});
}
/**
* Deletes a exception policy by Id.
*
* @param exceptionPolicyId Id of an exception policy.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@Generated
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono deleteExceptionPolicy(String exceptionPolicyId) {
// Generated convenience method for deleteExceptionPolicyWithResponse
RequestOptions requestOptions = new RequestOptions();
return deleteExceptionPolicyWithResponse(exceptionPolicyId, requestOptions).flatMap(FluxUtil::toMono);
}
/**
* Retrieves an existing queue by Id.
*
* @param queueId The Id of this queue.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a queue that can contain jobs to be routed on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getQueue(String queueId) {
// Generated convenience method for getQueueWithResponse
RequestOptions requestOptions = new RequestOptions();
return getQueueWithResponse(queueId, requestOptions).flatMap(FluxUtil::toMono)
.map(protocolMethodData -> protocolMethodData.toObject(RouterQueue.class));
}
/**
* Retrieves existing queues.
*
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return a paged collection of queues as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listQueues() {
// Generated convenience method for listQueues
RequestOptions requestOptions = new RequestOptions();
PagedFlux pagedFluxResponse = listQueues(requestOptions);
return PagedFlux.create(() -> (continuationToken, pageSize) -> {
Flux> flux = (continuationToken == null)
? pagedFluxResponse.byPage().take(1)
: pagedFluxResponse.byPage(continuationToken).take(1);
return flux.map(pagedResponse -> new PagedResponseBase(pagedResponse.getRequest(),
pagedResponse.getStatusCode(), pagedResponse.getHeaders(),
pagedResponse.getValue()
.stream()
.map(protocolMethodData -> protocolMethodData.toObject(RouterQueue.class))
.collect(Collectors.toList()),
pagedResponse.getContinuationToken(), null));
});
}
/**
* Deletes a queue by Id.
*
* @param queueId Id of a queue.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return A {@link Mono} that completes when a successful response is received.
*/
@Generated
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono deleteQueue(String queueId) {
// Generated convenience method for deleteQueueWithResponse
RequestOptions requestOptions = new RequestOptions();
return deleteQueueWithResponse(queueId, requestOptions).flatMap(FluxUtil::toMono);
}
}