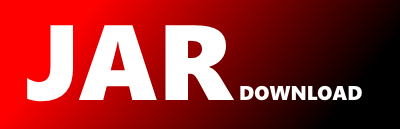
com.azure.communication.jobrouter.implementation.JobRouterClientImpl Maven / Gradle / Ivy
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) TypeSpec Code Generator.
package com.azure.communication.jobrouter.implementation;
import com.azure.communication.jobrouter.JobRouterServiceVersion;
import com.azure.core.annotation.BodyParam;
import com.azure.core.annotation.Delete;
import com.azure.core.annotation.ExpectedResponses;
import com.azure.core.annotation.Get;
import com.azure.core.annotation.HeaderParam;
import com.azure.core.annotation.Host;
import com.azure.core.annotation.HostParam;
import com.azure.core.annotation.Patch;
import com.azure.core.annotation.PathParam;
import com.azure.core.annotation.Post;
import com.azure.core.annotation.QueryParam;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceInterface;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.annotation.UnexpectedResponseExceptionType;
import com.azure.core.exception.ClientAuthenticationException;
import com.azure.core.exception.HttpResponseException;
import com.azure.core.exception.ResourceModifiedException;
import com.azure.core.exception.ResourceNotFoundException;
import com.azure.core.http.HttpHeaderName;
import com.azure.core.http.HttpPipeline;
import com.azure.core.http.HttpPipelineBuilder;
import com.azure.core.http.policy.RetryPolicy;
import com.azure.core.http.policy.UserAgentPolicy;
import com.azure.core.http.rest.PagedFlux;
import com.azure.core.http.rest.PagedIterable;
import com.azure.core.http.rest.PagedResponse;
import com.azure.core.http.rest.PagedResponseBase;
import com.azure.core.http.rest.RequestOptions;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.RestProxy;
import com.azure.core.util.BinaryData;
import com.azure.core.util.Context;
import com.azure.core.util.FluxUtil;
import com.azure.core.util.UrlBuilder;
import com.azure.core.util.serializer.JacksonAdapter;
import com.azure.core.util.serializer.SerializerAdapter;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import reactor.core.publisher.Mono;
/**
* Initializes a new instance of the JobRouterClient type.
*/
public final class JobRouterClientImpl {
/**
* The proxy service used to perform REST calls.
*/
private final JobRouterClientService service;
/**
* Uri of your Communication resource.
*/
private final String endpoint;
/**
* Gets Uri of your Communication resource.
*
* @return the endpoint value.
*/
public String getEndpoint() {
return this.endpoint;
}
/**
* Service version.
*/
private final JobRouterServiceVersion serviceVersion;
/**
* Gets Service version.
*
* @return the serviceVersion value.
*/
public JobRouterServiceVersion getServiceVersion() {
return this.serviceVersion;
}
/**
* The HTTP pipeline to send requests through.
*/
private final HttpPipeline httpPipeline;
/**
* Gets The HTTP pipeline to send requests through.
*
* @return the httpPipeline value.
*/
public HttpPipeline getHttpPipeline() {
return this.httpPipeline;
}
/**
* The serializer to serialize an object into a string.
*/
private final SerializerAdapter serializerAdapter;
/**
* Gets The serializer to serialize an object into a string.
*
* @return the serializerAdapter value.
*/
public SerializerAdapter getSerializerAdapter() {
return this.serializerAdapter;
}
/**
* Initializes an instance of JobRouterClient client.
*
* @param endpoint Uri of your Communication resource.
* @param serviceVersion Service version.
*/
public JobRouterClientImpl(String endpoint, JobRouterServiceVersion serviceVersion) {
this(new HttpPipelineBuilder().policies(new UserAgentPolicy(), new RetryPolicy()).build(),
JacksonAdapter.createDefaultSerializerAdapter(), endpoint, serviceVersion);
}
/**
* Initializes an instance of JobRouterClient client.
*
* @param httpPipeline The HTTP pipeline to send requests through.
* @param endpoint Uri of your Communication resource.
* @param serviceVersion Service version.
*/
public JobRouterClientImpl(HttpPipeline httpPipeline, String endpoint, JobRouterServiceVersion serviceVersion) {
this(httpPipeline, JacksonAdapter.createDefaultSerializerAdapter(), endpoint, serviceVersion);
}
/**
* Initializes an instance of JobRouterClient client.
*
* @param httpPipeline The HTTP pipeline to send requests through.
* @param serializerAdapter The serializer to serialize an object into a string.
* @param endpoint Uri of your Communication resource.
* @param serviceVersion Service version.
*/
public JobRouterClientImpl(HttpPipeline httpPipeline, SerializerAdapter serializerAdapter, String endpoint,
JobRouterServiceVersion serviceVersion) {
this.httpPipeline = httpPipeline;
this.serializerAdapter = serializerAdapter;
this.endpoint = endpoint;
this.serviceVersion = serviceVersion;
this.service = RestProxy.create(JobRouterClientService.class, this.httpPipeline, this.getSerializerAdapter());
}
/**
* The interface defining all the services for JobRouterClient to be used by the proxy service to perform REST
* calls.
*/
@Host("{endpoint}")
@ServiceInterface(name = "JobRouterClient")
public interface JobRouterClientService {
@Patch("/routing/jobs/{jobId}")
@ExpectedResponses({ 200, 201 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> upsertJob(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("jobId") String jobId,
@HeaderParam("Content-Type") String contentType, @HeaderParam("accept") String accept,
@BodyParam("application/merge-patch+json") BinaryData resource, RequestOptions requestOptions,
Context context);
@Patch("/routing/jobs/{jobId}")
@ExpectedResponses({ 200, 201 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response upsertJobSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("jobId") String jobId,
@HeaderParam("Content-Type") String contentType, @HeaderParam("accept") String accept,
@BodyParam("application/merge-patch+json") BinaryData resource, RequestOptions requestOptions,
Context context);
@Get("/routing/jobs/{jobId}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> getJob(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("jobId") String jobId,
@HeaderParam("accept") String accept, RequestOptions requestOptions, Context context);
@Get("/routing/jobs/{jobId}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response getJobSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("jobId") String jobId,
@HeaderParam("accept") String accept, RequestOptions requestOptions, Context context);
@Delete("/routing/jobs/{jobId}")
@ExpectedResponses({ 204 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> deleteJob(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("jobId") String jobId,
@HeaderParam("accept") String accept, RequestOptions requestOptions, Context context);
@Delete("/routing/jobs/{jobId}")
@ExpectedResponses({ 204 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response deleteJobSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("jobId") String jobId,
@HeaderParam("accept") String accept, RequestOptions requestOptions, Context context);
@Post("/routing/jobs/{jobId}:reclassify")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> reclassifyJob(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("jobId") String jobId,
@HeaderParam("accept") String accept, RequestOptions requestOptions, Context context);
@Post("/routing/jobs/{jobId}:reclassify")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response reclassifyJobSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("jobId") String jobId,
@HeaderParam("accept") String accept, RequestOptions requestOptions, Context context);
@Post("/routing/jobs/{jobId}:cancel")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> cancelJob(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("jobId") String jobId,
@HeaderParam("accept") String accept, RequestOptions requestOptions, Context context);
@Post("/routing/jobs/{jobId}:cancel")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response cancelJobSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("jobId") String jobId,
@HeaderParam("accept") String accept, RequestOptions requestOptions, Context context);
@Post("/routing/jobs/{jobId}/assignments/{assignmentId}:complete")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> completeJob(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("jobId") String jobId,
@PathParam("assignmentId") String assignmentId, @HeaderParam("accept") String accept,
RequestOptions requestOptions, Context context);
@Post("/routing/jobs/{jobId}/assignments/{assignmentId}:complete")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response completeJobSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("jobId") String jobId,
@PathParam("assignmentId") String assignmentId, @HeaderParam("accept") String accept,
RequestOptions requestOptions, Context context);
@Post("/routing/jobs/{jobId}/assignments/{assignmentId}:close")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> closeJob(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("jobId") String jobId,
@PathParam("assignmentId") String assignmentId, @HeaderParam("accept") String accept,
RequestOptions requestOptions, Context context);
@Post("/routing/jobs/{jobId}/assignments/{assignmentId}:close")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response closeJobSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("jobId") String jobId,
@PathParam("assignmentId") String assignmentId, @HeaderParam("accept") String accept,
RequestOptions requestOptions, Context context);
@Get("/routing/jobs")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> listJobs(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @HeaderParam("accept") String accept,
RequestOptions requestOptions, Context context);
@Get("/routing/jobs")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response listJobsSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @HeaderParam("accept") String accept,
RequestOptions requestOptions, Context context);
@Get("/routing/jobs/{jobId}/position")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> getQueuePosition(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("jobId") String jobId,
@HeaderParam("accept") String accept, RequestOptions requestOptions, Context context);
@Get("/routing/jobs/{jobId}/position")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response getQueuePositionSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("jobId") String jobId,
@HeaderParam("accept") String accept, RequestOptions requestOptions, Context context);
@Post("/routing/jobs/{jobId}/assignments/{assignmentId}:unassign")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> unassignJob(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("jobId") String jobId,
@PathParam("assignmentId") String assignmentId, @HeaderParam("accept") String accept,
RequestOptions requestOptions, Context context);
@Post("/routing/jobs/{jobId}/assignments/{assignmentId}:unassign")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response unassignJobSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("jobId") String jobId,
@PathParam("assignmentId") String assignmentId, @HeaderParam("accept") String accept,
RequestOptions requestOptions, Context context);
@Post("/routing/workers/{workerId}/offers/{offerId}:accept")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> acceptJobOffer(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("workerId") String workerId,
@PathParam("offerId") String offerId, @HeaderParam("accept") String accept, RequestOptions requestOptions,
Context context);
@Post("/routing/workers/{workerId}/offers/{offerId}:accept")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response acceptJobOfferSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("workerId") String workerId,
@PathParam("offerId") String offerId, @HeaderParam("accept") String accept, RequestOptions requestOptions,
Context context);
@Post("/routing/workers/{workerId}/offers/{offerId}:decline")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> declineJobOffer(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("workerId") String workerId,
@PathParam("offerId") String offerId, @HeaderParam("accept") String accept, RequestOptions requestOptions,
Context context);
@Post("/routing/workers/{workerId}/offers/{offerId}:decline")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response declineJobOfferSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("workerId") String workerId,
@PathParam("offerId") String offerId, @HeaderParam("accept") String accept, RequestOptions requestOptions,
Context context);
@Get("/routing/queues/{queueId}/statistics")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> getQueueStatistics(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("queueId") String queueId,
@HeaderParam("accept") String accept, RequestOptions requestOptions, Context context);
@Get("/routing/queues/{queueId}/statistics")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response getQueueStatisticsSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("queueId") String queueId,
@HeaderParam("accept") String accept, RequestOptions requestOptions, Context context);
@Patch("/routing/workers/{workerId}")
@ExpectedResponses({ 200, 201 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> upsertWorker(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("workerId") String workerId,
@HeaderParam("Content-Type") String contentType, @HeaderParam("accept") String accept,
@BodyParam("application/merge-patch+json") BinaryData resource, RequestOptions requestOptions,
Context context);
@Patch("/routing/workers/{workerId}")
@ExpectedResponses({ 200, 201 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response upsertWorkerSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("workerId") String workerId,
@HeaderParam("Content-Type") String contentType, @HeaderParam("accept") String accept,
@BodyParam("application/merge-patch+json") BinaryData resource, RequestOptions requestOptions,
Context context);
@Get("/routing/workers/{workerId}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> getWorker(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("workerId") String workerId,
@HeaderParam("accept") String accept, RequestOptions requestOptions, Context context);
@Get("/routing/workers/{workerId}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response getWorkerSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("workerId") String workerId,
@HeaderParam("accept") String accept, RequestOptions requestOptions, Context context);
@Delete("/routing/workers/{workerId}")
@ExpectedResponses({ 204 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> deleteWorker(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("workerId") String workerId,
@HeaderParam("accept") String accept, RequestOptions requestOptions, Context context);
@Delete("/routing/workers/{workerId}")
@ExpectedResponses({ 204 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response deleteWorkerSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @PathParam("workerId") String workerId,
@HeaderParam("accept") String accept, RequestOptions requestOptions, Context context);
@Get("/routing/workers")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> listWorkers(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @HeaderParam("accept") String accept,
RequestOptions requestOptions, Context context);
@Get("/routing/workers")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response listWorkersSync(@HostParam("endpoint") String endpoint,
@QueryParam("api-version") String apiVersion, @HeaderParam("accept") String accept,
RequestOptions requestOptions, Context context);
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> listJobsNext(@PathParam(value = "nextLink", encoded = true) String nextLink,
@HostParam("endpoint") String endpoint, @HeaderParam("accept") String accept, RequestOptions requestOptions,
Context context);
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response listJobsNextSync(@PathParam(value = "nextLink", encoded = true) String nextLink,
@HostParam("endpoint") String endpoint, @HeaderParam("accept") String accept, RequestOptions requestOptions,
Context context);
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Mono> listWorkersNext(@PathParam(value = "nextLink", encoded = true) String nextLink,
@HostParam("endpoint") String endpoint, @HeaderParam("accept") String accept, RequestOptions requestOptions,
Context context);
@Get("{nextLink}")
@ExpectedResponses({ 200 })
@UnexpectedResponseExceptionType(value = ClientAuthenticationException.class, code = { 401 })
@UnexpectedResponseExceptionType(value = ResourceNotFoundException.class, code = { 404 })
@UnexpectedResponseExceptionType(value = ResourceModifiedException.class, code = { 409 })
@UnexpectedResponseExceptionType(HttpResponseException.class)
Response listWorkersNextSync(@PathParam(value = "nextLink", encoded = true) String nextLink,
@HostParam("endpoint") String endpoint, @HeaderParam("accept") String accept, RequestOptions requestOptions,
Context context);
}
/**
* Creates or updates a router job.
*
* Header Parameters
*
*
* Header Parameters
*
* Name
* Type
* Required
* Description
*
*
* If-Match
* String
* No
* The request should only proceed if an entity matches this string.
*
*
* If-Unmodified-Since
* OffsetDateTime
* No
* The request should only proceed if the entity was not modified after this time.
*
*
* You can add these to a request with {@link RequestOptions#addHeader}
*
* Request Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* channelReference: String (Optional)
* status: String(pendingClassification/queued/assigned/completed/closed/cancelled/classificationFailed/created/pendingSchedule/scheduled/scheduleFailed/waitingForActivation) (Optional)
* enqueuedAt: OffsetDateTime (Optional)
* channelId: String (Optional)
* classificationPolicyId: String (Optional)
* queueId: String (Optional)
* priority: Integer (Optional)
* dispositionCode: String (Optional)
* requestedWorkerSelectors (Optional): [
* (Optional){
* key: String (Required)
* labelOperator: String(equal/notEqual/lessThan/lessThanOrEqual/greaterThan/greaterThanOrEqual) (Required)
* value: Object (Optional)
* expiresAfterSeconds: Double (Optional)
* expedite: Boolean (Optional)
* status: String(active/expired) (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* attachedWorkerSelectors (Optional): [
* (recursive schema, see above)
* ]
* labels (Optional): {
* String: Object (Required)
* }
* assignments (Optional): {
* String (Required): {
* assignmentId: String (Required)
* workerId: String (Optional)
* assignedAt: OffsetDateTime (Required)
* completedAt: OffsetDateTime (Optional)
* closedAt: OffsetDateTime (Optional)
* }
* }
* tags (Optional): {
* String: Object (Required)
* }
* notes (Optional): [
* (Optional){
* message: String (Required)
* addedAt: OffsetDateTime (Optional)
* }
* ]
* scheduledAt: OffsetDateTime (Optional)
* matchingMode (Optional): {
* }
* }
* }
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* channelReference: String (Optional)
* status: String(pendingClassification/queued/assigned/completed/closed/cancelled/classificationFailed/created/pendingSchedule/scheduled/scheduleFailed/waitingForActivation) (Optional)
* enqueuedAt: OffsetDateTime (Optional)
* channelId: String (Optional)
* classificationPolicyId: String (Optional)
* queueId: String (Optional)
* priority: Integer (Optional)
* dispositionCode: String (Optional)
* requestedWorkerSelectors (Optional): [
* (Optional){
* key: String (Required)
* labelOperator: String(equal/notEqual/lessThan/lessThanOrEqual/greaterThan/greaterThanOrEqual) (Required)
* value: Object (Optional)
* expiresAfterSeconds: Double (Optional)
* expedite: Boolean (Optional)
* status: String(active/expired) (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* attachedWorkerSelectors (Optional): [
* (recursive schema, see above)
* ]
* labels (Optional): {
* String: Object (Required)
* }
* assignments (Optional): {
* String (Required): {
* assignmentId: String (Required)
* workerId: String (Optional)
* assignedAt: OffsetDateTime (Required)
* completedAt: OffsetDateTime (Optional)
* closedAt: OffsetDateTime (Optional)
* }
* }
* tags (Optional): {
* String: Object (Required)
* }
* notes (Optional): [
* (Optional){
* message: String (Required)
* addedAt: OffsetDateTime (Optional)
* }
* ]
* scheduledAt: OffsetDateTime (Optional)
* matchingMode (Optional): {
* }
* }
* }
*
* @param jobId Id of a job.
* @param resource The resource instance.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return a unit of work to be routed along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> upsertJobWithResponseAsync(String jobId, BinaryData resource,
RequestOptions requestOptions) {
final String contentType = "application/merge-patch+json";
final String accept = "application/json";
return FluxUtil.withContext(context -> service.upsertJob(this.getEndpoint(),
this.getServiceVersion().getVersion(), jobId, contentType, accept, resource, requestOptions, context));
}
/**
* Creates or updates a router job.
*
* Header Parameters
*
*
* Header Parameters
*
* Name
* Type
* Required
* Description
*
*
* If-Match
* String
* No
* The request should only proceed if an entity matches this string.
*
*
* If-Unmodified-Since
* OffsetDateTime
* No
* The request should only proceed if the entity was not modified after this time.
*
*
* You can add these to a request with {@link RequestOptions#addHeader}
*
* Request Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* channelReference: String (Optional)
* status: String(pendingClassification/queued/assigned/completed/closed/cancelled/classificationFailed/created/pendingSchedule/scheduled/scheduleFailed/waitingForActivation) (Optional)
* enqueuedAt: OffsetDateTime (Optional)
* channelId: String (Optional)
* classificationPolicyId: String (Optional)
* queueId: String (Optional)
* priority: Integer (Optional)
* dispositionCode: String (Optional)
* requestedWorkerSelectors (Optional): [
* (Optional){
* key: String (Required)
* labelOperator: String(equal/notEqual/lessThan/lessThanOrEqual/greaterThan/greaterThanOrEqual) (Required)
* value: Object (Optional)
* expiresAfterSeconds: Double (Optional)
* expedite: Boolean (Optional)
* status: String(active/expired) (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* attachedWorkerSelectors (Optional): [
* (recursive schema, see above)
* ]
* labels (Optional): {
* String: Object (Required)
* }
* assignments (Optional): {
* String (Required): {
* assignmentId: String (Required)
* workerId: String (Optional)
* assignedAt: OffsetDateTime (Required)
* completedAt: OffsetDateTime (Optional)
* closedAt: OffsetDateTime (Optional)
* }
* }
* tags (Optional): {
* String: Object (Required)
* }
* notes (Optional): [
* (Optional){
* message: String (Required)
* addedAt: OffsetDateTime (Optional)
* }
* ]
* scheduledAt: OffsetDateTime (Optional)
* matchingMode (Optional): {
* }
* }
* }
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* channelReference: String (Optional)
* status: String(pendingClassification/queued/assigned/completed/closed/cancelled/classificationFailed/created/pendingSchedule/scheduled/scheduleFailed/waitingForActivation) (Optional)
* enqueuedAt: OffsetDateTime (Optional)
* channelId: String (Optional)
* classificationPolicyId: String (Optional)
* queueId: String (Optional)
* priority: Integer (Optional)
* dispositionCode: String (Optional)
* requestedWorkerSelectors (Optional): [
* (Optional){
* key: String (Required)
* labelOperator: String(equal/notEqual/lessThan/lessThanOrEqual/greaterThan/greaterThanOrEqual) (Required)
* value: Object (Optional)
* expiresAfterSeconds: Double (Optional)
* expedite: Boolean (Optional)
* status: String(active/expired) (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* attachedWorkerSelectors (Optional): [
* (recursive schema, see above)
* ]
* labels (Optional): {
* String: Object (Required)
* }
* assignments (Optional): {
* String (Required): {
* assignmentId: String (Required)
* workerId: String (Optional)
* assignedAt: OffsetDateTime (Required)
* completedAt: OffsetDateTime (Optional)
* closedAt: OffsetDateTime (Optional)
* }
* }
* tags (Optional): {
* String: Object (Required)
* }
* notes (Optional): [
* (Optional){
* message: String (Required)
* addedAt: OffsetDateTime (Optional)
* }
* ]
* scheduledAt: OffsetDateTime (Optional)
* matchingMode (Optional): {
* }
* }
* }
*
* @param jobId Id of a job.
* @param resource The resource instance.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return a unit of work to be routed along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response upsertJobWithResponse(String jobId, BinaryData resource,
RequestOptions requestOptions) {
final String contentType = "application/merge-patch+json";
final String accept = "application/json";
return service.upsertJobSync(this.getEndpoint(), this.getServiceVersion().getVersion(), jobId, contentType,
accept, resource, requestOptions, Context.NONE);
}
/**
* Retrieves an existing job by Id.
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* channelReference: String (Optional)
* status: String(pendingClassification/queued/assigned/completed/closed/cancelled/classificationFailed/created/pendingSchedule/scheduled/scheduleFailed/waitingForActivation) (Optional)
* enqueuedAt: OffsetDateTime (Optional)
* channelId: String (Optional)
* classificationPolicyId: String (Optional)
* queueId: String (Optional)
* priority: Integer (Optional)
* dispositionCode: String (Optional)
* requestedWorkerSelectors (Optional): [
* (Optional){
* key: String (Required)
* labelOperator: String(equal/notEqual/lessThan/lessThanOrEqual/greaterThan/greaterThanOrEqual) (Required)
* value: Object (Optional)
* expiresAfterSeconds: Double (Optional)
* expedite: Boolean (Optional)
* status: String(active/expired) (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* attachedWorkerSelectors (Optional): [
* (recursive schema, see above)
* ]
* labels (Optional): {
* String: Object (Required)
* }
* assignments (Optional): {
* String (Required): {
* assignmentId: String (Required)
* workerId: String (Optional)
* assignedAt: OffsetDateTime (Required)
* completedAt: OffsetDateTime (Optional)
* closedAt: OffsetDateTime (Optional)
* }
* }
* tags (Optional): {
* String: Object (Required)
* }
* notes (Optional): [
* (Optional){
* message: String (Required)
* addedAt: OffsetDateTime (Optional)
* }
* ]
* scheduledAt: OffsetDateTime (Optional)
* matchingMode (Optional): {
* }
* }
* }
*
* @param jobId Id of a job.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return a unit of work to be routed along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getJobWithResponseAsync(String jobId, RequestOptions requestOptions) {
final String accept = "application/json";
return FluxUtil.withContext(context -> service.getJob(this.getEndpoint(), this.getServiceVersion().getVersion(),
jobId, accept, requestOptions, context));
}
/**
* Retrieves an existing job by Id.
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* channelReference: String (Optional)
* status: String(pendingClassification/queued/assigned/completed/closed/cancelled/classificationFailed/created/pendingSchedule/scheduled/scheduleFailed/waitingForActivation) (Optional)
* enqueuedAt: OffsetDateTime (Optional)
* channelId: String (Optional)
* classificationPolicyId: String (Optional)
* queueId: String (Optional)
* priority: Integer (Optional)
* dispositionCode: String (Optional)
* requestedWorkerSelectors (Optional): [
* (Optional){
* key: String (Required)
* labelOperator: String(equal/notEqual/lessThan/lessThanOrEqual/greaterThan/greaterThanOrEqual) (Required)
* value: Object (Optional)
* expiresAfterSeconds: Double (Optional)
* expedite: Boolean (Optional)
* status: String(active/expired) (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* attachedWorkerSelectors (Optional): [
* (recursive schema, see above)
* ]
* labels (Optional): {
* String: Object (Required)
* }
* assignments (Optional): {
* String (Required): {
* assignmentId: String (Required)
* workerId: String (Optional)
* assignedAt: OffsetDateTime (Required)
* completedAt: OffsetDateTime (Optional)
* closedAt: OffsetDateTime (Optional)
* }
* }
* tags (Optional): {
* String: Object (Required)
* }
* notes (Optional): [
* (Optional){
* message: String (Required)
* addedAt: OffsetDateTime (Optional)
* }
* ]
* scheduledAt: OffsetDateTime (Optional)
* matchingMode (Optional): {
* }
* }
* }
*
* @param jobId Id of a job.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return a unit of work to be routed along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getJobWithResponse(String jobId, RequestOptions requestOptions) {
final String accept = "application/json";
return service.getJobSync(this.getEndpoint(), this.getServiceVersion().getVersion(), jobId, accept,
requestOptions, Context.NONE);
}
/**
* Deletes a job and all of its traces.
*
* @param jobId Id of a job.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> deleteJobWithResponseAsync(String jobId, RequestOptions requestOptions) {
final String accept = "application/json";
return FluxUtil.withContext(context -> service.deleteJob(this.getEndpoint(),
this.getServiceVersion().getVersion(), jobId, accept, requestOptions, context));
}
/**
* Deletes a job and all of its traces.
*
* @param jobId Id of a job.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return the {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response deleteJobWithResponse(String jobId, RequestOptions requestOptions) {
final String accept = "application/json";
return service.deleteJobSync(this.getEndpoint(), this.getServiceVersion().getVersion(), jobId, accept,
requestOptions, Context.NONE);
}
/**
* Reclassify a job.
*
* Request Body Schema
*
* {@code
* {
* }
* }
*
* Response Body Schema
*
* {@code
* {
* }
* }
*
* @param jobId Id of a job.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return response payload from reclassifying a job along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> reclassifyJobWithResponseAsync(String jobId, RequestOptions requestOptions) {
final String accept = "application/json";
RequestOptions requestOptionsLocal = requestOptions == null ? new RequestOptions() : requestOptions;
requestOptionsLocal.addRequestCallback(requestLocal -> {
if (requestLocal.getBody() != null && requestLocal.getHeaders().get(HttpHeaderName.CONTENT_TYPE) == null) {
requestLocal.getHeaders().set(HttpHeaderName.CONTENT_TYPE, "application/json");
}
});
return FluxUtil.withContext(context -> service.reclassifyJob(this.getEndpoint(),
this.getServiceVersion().getVersion(), jobId, accept, requestOptionsLocal, context));
}
/**
* Reclassify a job.
*
* Request Body Schema
*
* {@code
* {
* }
* }
*
* Response Body Schema
*
* {@code
* {
* }
* }
*
* @param jobId Id of a job.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return response payload from reclassifying a job along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response reclassifyJobWithResponse(String jobId, RequestOptions requestOptions) {
final String accept = "application/json";
RequestOptions requestOptionsLocal = requestOptions == null ? new RequestOptions() : requestOptions;
requestOptionsLocal.addRequestCallback(requestLocal -> {
if (requestLocal.getBody() != null && requestLocal.getHeaders().get(HttpHeaderName.CONTENT_TYPE) == null) {
requestLocal.getHeaders().set(HttpHeaderName.CONTENT_TYPE, "application/json");
}
});
return service.reclassifyJobSync(this.getEndpoint(), this.getServiceVersion().getVersion(), jobId, accept,
requestOptionsLocal, Context.NONE);
}
/**
* Submits request to cancel an existing job by Id while supplying free-form cancellation reason.
*
* Request Body Schema
*
* {@code
* {
* note: String (Optional)
* dispositionCode: String (Optional)
* }
* }
*
* Response Body Schema
*
* {@code
* {
* }
* }
*
* @param jobId Id of a job.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return response payload from cancelling a job along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> cancelJobWithResponseAsync(String jobId, RequestOptions requestOptions) {
final String accept = "application/json";
RequestOptions requestOptionsLocal = requestOptions == null ? new RequestOptions() : requestOptions;
requestOptionsLocal.addRequestCallback(requestLocal -> {
if (requestLocal.getBody() != null && requestLocal.getHeaders().get(HttpHeaderName.CONTENT_TYPE) == null) {
requestLocal.getHeaders().set(HttpHeaderName.CONTENT_TYPE, "application/json");
}
});
return FluxUtil.withContext(context -> service.cancelJob(this.getEndpoint(),
this.getServiceVersion().getVersion(), jobId, accept, requestOptionsLocal, context));
}
/**
* Submits request to cancel an existing job by Id while supplying free-form cancellation reason.
*
* Request Body Schema
*
* {@code
* {
* note: String (Optional)
* dispositionCode: String (Optional)
* }
* }
*
* Response Body Schema
*
* {@code
* {
* }
* }
*
* @param jobId Id of a job.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return response payload from cancelling a job along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response cancelJobWithResponse(String jobId, RequestOptions requestOptions) {
final String accept = "application/json";
RequestOptions requestOptionsLocal = requestOptions == null ? new RequestOptions() : requestOptions;
requestOptionsLocal.addRequestCallback(requestLocal -> {
if (requestLocal.getBody() != null && requestLocal.getHeaders().get(HttpHeaderName.CONTENT_TYPE) == null) {
requestLocal.getHeaders().set(HttpHeaderName.CONTENT_TYPE, "application/json");
}
});
return service.cancelJobSync(this.getEndpoint(), this.getServiceVersion().getVersion(), jobId, accept,
requestOptionsLocal, Context.NONE);
}
/**
* Completes an assigned job.
*
* Request Body Schema
*
* {@code
* {
* note: String (Optional)
* }
* }
*
* Response Body Schema
*
* {@code
* {
* }
* }
*
* @param jobId Id of a job.
* @param assignmentId Id of a job assignment.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return response payload from completing a job along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> completeJobWithResponseAsync(String jobId, String assignmentId,
RequestOptions requestOptions) {
final String accept = "application/json";
RequestOptions requestOptionsLocal = requestOptions == null ? new RequestOptions() : requestOptions;
requestOptionsLocal.addRequestCallback(requestLocal -> {
if (requestLocal.getBody() != null && requestLocal.getHeaders().get(HttpHeaderName.CONTENT_TYPE) == null) {
requestLocal.getHeaders().set(HttpHeaderName.CONTENT_TYPE, "application/json");
}
});
return FluxUtil.withContext(context -> service.completeJob(this.getEndpoint(),
this.getServiceVersion().getVersion(), jobId, assignmentId, accept, requestOptionsLocal, context));
}
/**
* Completes an assigned job.
*
* Request Body Schema
*
* {@code
* {
* note: String (Optional)
* }
* }
*
* Response Body Schema
*
* {@code
* {
* }
* }
*
* @param jobId Id of a job.
* @param assignmentId Id of a job assignment.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return response payload from completing a job along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response completeJobWithResponse(String jobId, String assignmentId,
RequestOptions requestOptions) {
final String accept = "application/json";
RequestOptions requestOptionsLocal = requestOptions == null ? new RequestOptions() : requestOptions;
requestOptionsLocal.addRequestCallback(requestLocal -> {
if (requestLocal.getBody() != null && requestLocal.getHeaders().get(HttpHeaderName.CONTENT_TYPE) == null) {
requestLocal.getHeaders().set(HttpHeaderName.CONTENT_TYPE, "application/json");
}
});
return service.completeJobSync(this.getEndpoint(), this.getServiceVersion().getVersion(), jobId, assignmentId,
accept, requestOptionsLocal, Context.NONE);
}
/**
* Closes a completed job.
*
* Request Body Schema
*
* {@code
* {
* dispositionCode: String (Optional)
* closeAt: OffsetDateTime (Optional)
* note: String (Optional)
* }
* }
*
* Response Body Schema
*
* {@code
* {
* }
* }
*
* @param jobId Id of a job.
* @param assignmentId Id of a job assignment.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return response payload from closing a job along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> closeJobWithResponseAsync(String jobId, String assignmentId,
RequestOptions requestOptions) {
final String accept = "application/json";
RequestOptions requestOptionsLocal = requestOptions == null ? new RequestOptions() : requestOptions;
requestOptionsLocal.addRequestCallback(requestLocal -> {
if (requestLocal.getBody() != null && requestLocal.getHeaders().get(HttpHeaderName.CONTENT_TYPE) == null) {
requestLocal.getHeaders().set(HttpHeaderName.CONTENT_TYPE, "application/json");
}
});
return FluxUtil.withContext(context -> service.closeJob(this.getEndpoint(),
this.getServiceVersion().getVersion(), jobId, assignmentId, accept, requestOptionsLocal, context));
}
/**
* Closes a completed job.
*
* Request Body Schema
*
* {@code
* {
* dispositionCode: String (Optional)
* closeAt: OffsetDateTime (Optional)
* note: String (Optional)
* }
* }
*
* Response Body Schema
*
* {@code
* {
* }
* }
*
* @param jobId Id of a job.
* @param assignmentId Id of a job assignment.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return response payload from closing a job along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response closeJobWithResponse(String jobId, String assignmentId, RequestOptions requestOptions) {
final String accept = "application/json";
RequestOptions requestOptionsLocal = requestOptions == null ? new RequestOptions() : requestOptions;
requestOptionsLocal.addRequestCallback(requestLocal -> {
if (requestLocal.getBody() != null && requestLocal.getHeaders().get(HttpHeaderName.CONTENT_TYPE) == null) {
requestLocal.getHeaders().set(HttpHeaderName.CONTENT_TYPE, "application/json");
}
});
return service.closeJobSync(this.getEndpoint(), this.getServiceVersion().getVersion(), jobId, assignmentId,
accept, requestOptionsLocal, Context.NONE);
}
/**
* Retrieves list of jobs based on filter parameters.
*
* Query Parameters
*
*
* Query Parameters
*
* Name
* Type
* Required
* Description
*
*
* maxpagesize
* Integer
* No
* Number of objects to return per page.
*
*
* status
* String
* No
* If specified, filter jobs by status. Allowed values: "all", "pendingClassification", "queued", "assigned",
* "completed", "closed", "cancelled", "classificationFailed", "created", "pendingSchedule", "scheduled",
* "scheduleFailed", "waitingForActivation", "active".
*
*
* queueId
* String
* No
* If specified, filter jobs by queue.
*
*
* channelId
* String
* No
* If specified, filter jobs by channel.
*
*
* classificationPolicyId
* String
* No
* If specified, filter jobs by classificationPolicy.
*
*
* scheduledBefore
* OffsetDateTime
* No
* If specified, filter on jobs that was scheduled before or at given timestamp. Range: (-Inf,
* scheduledBefore].
*
*
* scheduledAfter
* OffsetDateTime
* No
* If specified, filter on jobs that was scheduled at or after given value. Range: [scheduledAfter, +Inf).
*
*
* You can add these to a request with {@link RequestOptions#addQueryParam}
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* channelReference: String (Optional)
* status: String(pendingClassification/queued/assigned/completed/closed/cancelled/classificationFailed/created/pendingSchedule/scheduled/scheduleFailed/waitingForActivation) (Optional)
* enqueuedAt: OffsetDateTime (Optional)
* channelId: String (Optional)
* classificationPolicyId: String (Optional)
* queueId: String (Optional)
* priority: Integer (Optional)
* dispositionCode: String (Optional)
* requestedWorkerSelectors (Optional): [
* (Optional){
* key: String (Required)
* labelOperator: String(equal/notEqual/lessThan/lessThanOrEqual/greaterThan/greaterThanOrEqual) (Required)
* value: Object (Optional)
* expiresAfterSeconds: Double (Optional)
* expedite: Boolean (Optional)
* status: String(active/expired) (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* attachedWorkerSelectors (Optional): [
* (recursive schema, see above)
* ]
* labels (Optional): {
* String: Object (Required)
* }
* assignments (Optional): {
* String (Required): {
* assignmentId: String (Required)
* workerId: String (Optional)
* assignedAt: OffsetDateTime (Required)
* completedAt: OffsetDateTime (Optional)
* closedAt: OffsetDateTime (Optional)
* }
* }
* tags (Optional): {
* String: Object (Required)
* }
* notes (Optional): [
* (Optional){
* message: String (Required)
* addedAt: OffsetDateTime (Optional)
* }
* ]
* scheduledAt: OffsetDateTime (Optional)
* matchingMode (Optional): {
* }
* }
* }
*
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return paged collection of RouterJob items along with {@link PagedResponse} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listJobsSinglePageAsync(RequestOptions requestOptions) {
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.listJobs(this.getEndpoint(), this.getServiceVersion().getVersion(), accept,
requestOptions, context))
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
getValues(res.getValue(), "value"), getNextLink(res.getValue(), "nextLink"), null));
}
/**
* Retrieves list of jobs based on filter parameters.
*
* Query Parameters
*
*
* Query Parameters
*
* Name
* Type
* Required
* Description
*
*
* maxpagesize
* Integer
* No
* Number of objects to return per page.
*
*
* status
* String
* No
* If specified, filter jobs by status. Allowed values: "all", "pendingClassification", "queued", "assigned",
* "completed", "closed", "cancelled", "classificationFailed", "created", "pendingSchedule", "scheduled",
* "scheduleFailed", "waitingForActivation", "active".
*
*
* queueId
* String
* No
* If specified, filter jobs by queue.
*
*
* channelId
* String
* No
* If specified, filter jobs by channel.
*
*
* classificationPolicyId
* String
* No
* If specified, filter jobs by classificationPolicy.
*
*
* scheduledBefore
* OffsetDateTime
* No
* If specified, filter on jobs that was scheduled before or at given timestamp. Range: (-Inf,
* scheduledBefore].
*
*
* scheduledAfter
* OffsetDateTime
* No
* If specified, filter on jobs that was scheduled at or after given value. Range: [scheduledAfter, +Inf).
*
*
* You can add these to a request with {@link RequestOptions#addQueryParam}
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* channelReference: String (Optional)
* status: String(pendingClassification/queued/assigned/completed/closed/cancelled/classificationFailed/created/pendingSchedule/scheduled/scheduleFailed/waitingForActivation) (Optional)
* enqueuedAt: OffsetDateTime (Optional)
* channelId: String (Optional)
* classificationPolicyId: String (Optional)
* queueId: String (Optional)
* priority: Integer (Optional)
* dispositionCode: String (Optional)
* requestedWorkerSelectors (Optional): [
* (Optional){
* key: String (Required)
* labelOperator: String(equal/notEqual/lessThan/lessThanOrEqual/greaterThan/greaterThanOrEqual) (Required)
* value: Object (Optional)
* expiresAfterSeconds: Double (Optional)
* expedite: Boolean (Optional)
* status: String(active/expired) (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* attachedWorkerSelectors (Optional): [
* (recursive schema, see above)
* ]
* labels (Optional): {
* String: Object (Required)
* }
* assignments (Optional): {
* String (Required): {
* assignmentId: String (Required)
* workerId: String (Optional)
* assignedAt: OffsetDateTime (Required)
* completedAt: OffsetDateTime (Optional)
* closedAt: OffsetDateTime (Optional)
* }
* }
* tags (Optional): {
* String: Object (Required)
* }
* notes (Optional): [
* (Optional){
* message: String (Required)
* addedAt: OffsetDateTime (Optional)
* }
* ]
* scheduledAt: OffsetDateTime (Optional)
* matchingMode (Optional): {
* }
* }
* }
*
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return paged collection of RouterJob items as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listJobsAsync(RequestOptions requestOptions) {
RequestOptions requestOptionsForNextPage = new RequestOptions();
requestOptionsForNextPage.setContext(
requestOptions != null && requestOptions.getContext() != null ? requestOptions.getContext() : Context.NONE);
return new PagedFlux<>((pageSize) -> {
RequestOptions requestOptionsLocal = requestOptions == null ? new RequestOptions() : requestOptions;
if (pageSize != null) {
requestOptionsLocal.addRequestCallback(requestLocal -> {
UrlBuilder urlBuilder = UrlBuilder.parse(requestLocal.getUrl());
urlBuilder.setQueryParameter("maxpagesize", String.valueOf(pageSize));
requestLocal.setUrl(urlBuilder.toString());
});
}
return listJobsSinglePageAsync(requestOptionsLocal);
}, (nextLink, pageSize) -> {
RequestOptions requestOptionsLocal = new RequestOptions();
requestOptionsLocal.setContext(requestOptionsForNextPage.getContext());
if (pageSize != null) {
requestOptionsLocal.addRequestCallback(requestLocal -> {
UrlBuilder urlBuilder = UrlBuilder.parse(requestLocal.getUrl());
urlBuilder.setQueryParameter("maxpagesize", String.valueOf(pageSize));
requestLocal.setUrl(urlBuilder.toString());
});
}
return listJobsNextSinglePageAsync(nextLink, requestOptionsLocal);
});
}
/**
* Retrieves list of jobs based on filter parameters.
*
* Query Parameters
*
*
* Query Parameters
*
* Name
* Type
* Required
* Description
*
*
* maxpagesize
* Integer
* No
* Number of objects to return per page.
*
*
* status
* String
* No
* If specified, filter jobs by status. Allowed values: "all", "pendingClassification", "queued", "assigned",
* "completed", "closed", "cancelled", "classificationFailed", "created", "pendingSchedule", "scheduled",
* "scheduleFailed", "waitingForActivation", "active".
*
*
* queueId
* String
* No
* If specified, filter jobs by queue.
*
*
* channelId
* String
* No
* If specified, filter jobs by channel.
*
*
* classificationPolicyId
* String
* No
* If specified, filter jobs by classificationPolicy.
*
*
* scheduledBefore
* OffsetDateTime
* No
* If specified, filter on jobs that was scheduled before or at given timestamp. Range: (-Inf,
* scheduledBefore].
*
*
* scheduledAfter
* OffsetDateTime
* No
* If specified, filter on jobs that was scheduled at or after given value. Range: [scheduledAfter, +Inf).
*
*
* You can add these to a request with {@link RequestOptions#addQueryParam}
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* channelReference: String (Optional)
* status: String(pendingClassification/queued/assigned/completed/closed/cancelled/classificationFailed/created/pendingSchedule/scheduled/scheduleFailed/waitingForActivation) (Optional)
* enqueuedAt: OffsetDateTime (Optional)
* channelId: String (Optional)
* classificationPolicyId: String (Optional)
* queueId: String (Optional)
* priority: Integer (Optional)
* dispositionCode: String (Optional)
* requestedWorkerSelectors (Optional): [
* (Optional){
* key: String (Required)
* labelOperator: String(equal/notEqual/lessThan/lessThanOrEqual/greaterThan/greaterThanOrEqual) (Required)
* value: Object (Optional)
* expiresAfterSeconds: Double (Optional)
* expedite: Boolean (Optional)
* status: String(active/expired) (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* attachedWorkerSelectors (Optional): [
* (recursive schema, see above)
* ]
* labels (Optional): {
* String: Object (Required)
* }
* assignments (Optional): {
* String (Required): {
* assignmentId: String (Required)
* workerId: String (Optional)
* assignedAt: OffsetDateTime (Required)
* completedAt: OffsetDateTime (Optional)
* closedAt: OffsetDateTime (Optional)
* }
* }
* tags (Optional): {
* String: Object (Required)
* }
* notes (Optional): [
* (Optional){
* message: String (Required)
* addedAt: OffsetDateTime (Optional)
* }
* ]
* scheduledAt: OffsetDateTime (Optional)
* matchingMode (Optional): {
* }
* }
* }
*
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return paged collection of RouterJob items along with {@link PagedResponse}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private PagedResponse listJobsSinglePage(RequestOptions requestOptions) {
final String accept = "application/json";
Response res = service.listJobsSync(this.getEndpoint(), this.getServiceVersion().getVersion(),
accept, requestOptions, Context.NONE);
return new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
getValues(res.getValue(), "value"), getNextLink(res.getValue(), "nextLink"), null);
}
/**
* Retrieves list of jobs based on filter parameters.
*
* Query Parameters
*
*
* Query Parameters
*
* Name
* Type
* Required
* Description
*
*
* maxpagesize
* Integer
* No
* Number of objects to return per page.
*
*
* status
* String
* No
* If specified, filter jobs by status. Allowed values: "all", "pendingClassification", "queued", "assigned",
* "completed", "closed", "cancelled", "classificationFailed", "created", "pendingSchedule", "scheduled",
* "scheduleFailed", "waitingForActivation", "active".
*
*
* queueId
* String
* No
* If specified, filter jobs by queue.
*
*
* channelId
* String
* No
* If specified, filter jobs by channel.
*
*
* classificationPolicyId
* String
* No
* If specified, filter jobs by classificationPolicy.
*
*
* scheduledBefore
* OffsetDateTime
* No
* If specified, filter on jobs that was scheduled before or at given timestamp. Range: (-Inf,
* scheduledBefore].
*
*
* scheduledAfter
* OffsetDateTime
* No
* If specified, filter on jobs that was scheduled at or after given value. Range: [scheduledAfter, +Inf).
*
*
* You can add these to a request with {@link RequestOptions#addQueryParam}
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* channelReference: String (Optional)
* status: String(pendingClassification/queued/assigned/completed/closed/cancelled/classificationFailed/created/pendingSchedule/scheduled/scheduleFailed/waitingForActivation) (Optional)
* enqueuedAt: OffsetDateTime (Optional)
* channelId: String (Optional)
* classificationPolicyId: String (Optional)
* queueId: String (Optional)
* priority: Integer (Optional)
* dispositionCode: String (Optional)
* requestedWorkerSelectors (Optional): [
* (Optional){
* key: String (Required)
* labelOperator: String(equal/notEqual/lessThan/lessThanOrEqual/greaterThan/greaterThanOrEqual) (Required)
* value: Object (Optional)
* expiresAfterSeconds: Double (Optional)
* expedite: Boolean (Optional)
* status: String(active/expired) (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* attachedWorkerSelectors (Optional): [
* (recursive schema, see above)
* ]
* labels (Optional): {
* String: Object (Required)
* }
* assignments (Optional): {
* String (Required): {
* assignmentId: String (Required)
* workerId: String (Optional)
* assignedAt: OffsetDateTime (Required)
* completedAt: OffsetDateTime (Optional)
* closedAt: OffsetDateTime (Optional)
* }
* }
* tags (Optional): {
* String: Object (Required)
* }
* notes (Optional): [
* (Optional){
* message: String (Required)
* addedAt: OffsetDateTime (Optional)
* }
* ]
* scheduledAt: OffsetDateTime (Optional)
* matchingMode (Optional): {
* }
* }
* }
*
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return paged collection of RouterJob items as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listJobs(RequestOptions requestOptions) {
RequestOptions requestOptionsForNextPage = new RequestOptions();
requestOptionsForNextPage.setContext(
requestOptions != null && requestOptions.getContext() != null ? requestOptions.getContext() : Context.NONE);
return new PagedIterable<>((pageSize) -> {
RequestOptions requestOptionsLocal = requestOptions == null ? new RequestOptions() : requestOptions;
if (pageSize != null) {
requestOptionsLocal.addRequestCallback(requestLocal -> {
UrlBuilder urlBuilder = UrlBuilder.parse(requestLocal.getUrl());
urlBuilder.setQueryParameter("maxpagesize", String.valueOf(pageSize));
requestLocal.setUrl(urlBuilder.toString());
});
}
return listJobsSinglePage(requestOptionsLocal);
}, (nextLink, pageSize) -> {
RequestOptions requestOptionsLocal = new RequestOptions();
requestOptionsLocal.setContext(requestOptionsForNextPage.getContext());
if (pageSize != null) {
requestOptionsLocal.addRequestCallback(requestLocal -> {
UrlBuilder urlBuilder = UrlBuilder.parse(requestLocal.getUrl());
urlBuilder.setQueryParameter("maxpagesize", String.valueOf(pageSize));
requestLocal.setUrl(urlBuilder.toString());
});
}
return listJobsNextSinglePage(nextLink, requestOptionsLocal);
});
}
/**
* Gets a job's position details.
*
* Response Body Schema
*
* {@code
* {
* jobId: String (Required)
* position: int (Required)
* queueId: String (Required)
* queueLength: int (Required)
* estimatedWaitTimeMinutes: double (Required)
* }
* }
*
* @param jobId Id of the job.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return a job's position details along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getQueuePositionWithResponseAsync(String jobId, RequestOptions requestOptions) {
final String accept = "application/json";
return FluxUtil.withContext(context -> service.getQueuePosition(this.getEndpoint(),
this.getServiceVersion().getVersion(), jobId, accept, requestOptions, context));
}
/**
* Gets a job's position details.
*
* Response Body Schema
*
* {@code
* {
* jobId: String (Required)
* position: int (Required)
* queueId: String (Required)
* queueLength: int (Required)
* estimatedWaitTimeMinutes: double (Required)
* }
* }
*
* @param jobId Id of the job.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return a job's position details along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getQueuePositionWithResponse(String jobId, RequestOptions requestOptions) {
final String accept = "application/json";
return service.getQueuePositionSync(this.getEndpoint(), this.getServiceVersion().getVersion(), jobId, accept,
requestOptions, Context.NONE);
}
/**
* Unassign a job.
*
* Request Body Schema
*
* {@code
* {
* suspendMatching: Boolean (Optional)
* }
* }
*
* Response Body Schema
*
* {@code
* {
* jobId: String (Required)
* unassignmentCount: int (Required)
* }
* }
*
* @param jobId Id of a job.
* @param assignmentId Id of a job assignment.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return response payload after a job has been successfully unassigned along with {@link Response} on successful
* completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> unassignJobWithResponseAsync(String jobId, String assignmentId,
RequestOptions requestOptions) {
final String accept = "application/json";
RequestOptions requestOptionsLocal = requestOptions == null ? new RequestOptions() : requestOptions;
requestOptionsLocal.addRequestCallback(requestLocal -> {
if (requestLocal.getBody() != null && requestLocal.getHeaders().get(HttpHeaderName.CONTENT_TYPE) == null) {
requestLocal.getHeaders().set(HttpHeaderName.CONTENT_TYPE, "application/json");
}
});
return FluxUtil.withContext(context -> service.unassignJob(this.getEndpoint(),
this.getServiceVersion().getVersion(), jobId, assignmentId, accept, requestOptionsLocal, context));
}
/**
* Unassign a job.
*
* Request Body Schema
*
* {@code
* {
* suspendMatching: Boolean (Optional)
* }
* }
*
* Response Body Schema
*
* {@code
* {
* jobId: String (Required)
* unassignmentCount: int (Required)
* }
* }
*
* @param jobId Id of a job.
* @param assignmentId Id of a job assignment.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return response payload after a job has been successfully unassigned along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response unassignJobWithResponse(String jobId, String assignmentId,
RequestOptions requestOptions) {
final String accept = "application/json";
RequestOptions requestOptionsLocal = requestOptions == null ? new RequestOptions() : requestOptions;
requestOptionsLocal.addRequestCallback(requestLocal -> {
if (requestLocal.getBody() != null && requestLocal.getHeaders().get(HttpHeaderName.CONTENT_TYPE) == null) {
requestLocal.getHeaders().set(HttpHeaderName.CONTENT_TYPE, "application/json");
}
});
return service.unassignJobSync(this.getEndpoint(), this.getServiceVersion().getVersion(), jobId, assignmentId,
accept, requestOptionsLocal, Context.NONE);
}
/**
* Accepts an offer to work on a job and returns a 409/Conflict if another agent accepted the job already.
*
* Response Body Schema
*
* {@code
* {
* assignmentId: String (Required)
* jobId: String (Required)
* workerId: String (Required)
* }
* }
*
* @param workerId Id of a worker.
* @param offerId Id of an offer.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return response containing ids for the worker, job, and assignment from an accepted offer along with
* {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> acceptJobOfferWithResponseAsync(String workerId, String offerId,
RequestOptions requestOptions) {
final String accept = "application/json";
return FluxUtil.withContext(context -> service.acceptJobOffer(this.getEndpoint(),
this.getServiceVersion().getVersion(), workerId, offerId, accept, requestOptions, context));
}
/**
* Accepts an offer to work on a job and returns a 409/Conflict if another agent accepted the job already.
*
* Response Body Schema
*
* {@code
* {
* assignmentId: String (Required)
* jobId: String (Required)
* workerId: String (Required)
* }
* }
*
* @param workerId Id of a worker.
* @param offerId Id of an offer.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return response containing ids for the worker, job, and assignment from an accepted offer along with
* {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response acceptJobOfferWithResponse(String workerId, String offerId,
RequestOptions requestOptions) {
final String accept = "application/json";
return service.acceptJobOfferSync(this.getEndpoint(), this.getServiceVersion().getVersion(), workerId, offerId,
accept, requestOptions, Context.NONE);
}
/**
* Declines an offer to work on a job.
*
* Request Body Schema
*
* {@code
* {
* retryOfferAt: OffsetDateTime (Optional)
* }
* }
*
* Response Body Schema
*
* {@code
* {
* }
* }
*
* @param workerId Id of a worker.
* @param offerId Id of an offer.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return response payload from declining a job along with {@link Response} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> declineJobOfferWithResponseAsync(String workerId, String offerId,
RequestOptions requestOptions) {
final String accept = "application/json";
RequestOptions requestOptionsLocal = requestOptions == null ? new RequestOptions() : requestOptions;
requestOptionsLocal.addRequestCallback(requestLocal -> {
if (requestLocal.getBody() != null && requestLocal.getHeaders().get(HttpHeaderName.CONTENT_TYPE) == null) {
requestLocal.getHeaders().set(HttpHeaderName.CONTENT_TYPE, "application/json");
}
});
return FluxUtil.withContext(context -> service.declineJobOffer(this.getEndpoint(),
this.getServiceVersion().getVersion(), workerId, offerId, accept, requestOptionsLocal, context));
}
/**
* Declines an offer to work on a job.
*
* Request Body Schema
*
* {@code
* {
* retryOfferAt: OffsetDateTime (Optional)
* }
* }
*
* Response Body Schema
*
* {@code
* {
* }
* }
*
* @param workerId Id of a worker.
* @param offerId Id of an offer.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return response payload from declining a job along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response declineJobOfferWithResponse(String workerId, String offerId,
RequestOptions requestOptions) {
final String accept = "application/json";
RequestOptions requestOptionsLocal = requestOptions == null ? new RequestOptions() : requestOptions;
requestOptionsLocal.addRequestCallback(requestLocal -> {
if (requestLocal.getBody() != null && requestLocal.getHeaders().get(HttpHeaderName.CONTENT_TYPE) == null) {
requestLocal.getHeaders().set(HttpHeaderName.CONTENT_TYPE, "application/json");
}
});
return service.declineJobOfferSync(this.getEndpoint(), this.getServiceVersion().getVersion(), workerId, offerId,
accept, requestOptionsLocal, Context.NONE);
}
/**
* Retrieves a queue's statistics.
*
* Response Body Schema
*
* {@code
* {
* queueId: String (Required)
* length: int (Required)
* estimatedWaitTimeMinutes (Optional): {
* String: double (Required)
* }
* longestJobWaitTimeMinutes: Double (Optional)
* }
* }
*
* @param queueId Id of the queue to retrieve statistics.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return statistics for the queue along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getQueueStatisticsWithResponseAsync(String queueId,
RequestOptions requestOptions) {
final String accept = "application/json";
return FluxUtil.withContext(context -> service.getQueueStatistics(this.getEndpoint(),
this.getServiceVersion().getVersion(), queueId, accept, requestOptions, context));
}
/**
* Retrieves a queue's statistics.
*
* Response Body Schema
*
* {@code
* {
* queueId: String (Required)
* length: int (Required)
* estimatedWaitTimeMinutes (Optional): {
* String: double (Required)
* }
* longestJobWaitTimeMinutes: Double (Optional)
* }
* }
*
* @param queueId Id of the queue to retrieve statistics.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return statistics for the queue along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getQueueStatisticsWithResponse(String queueId, RequestOptions requestOptions) {
final String accept = "application/json";
return service.getQueueStatisticsSync(this.getEndpoint(), this.getServiceVersion().getVersion(), queueId,
accept, requestOptions, Context.NONE);
}
/**
* Creates or updates a worker.
*
* Header Parameters
*
*
* Header Parameters
*
* Name
* Type
* Required
* Description
*
*
* If-Match
* String
* No
* The request should only proceed if an entity matches this string.
*
*
* If-Unmodified-Since
* OffsetDateTime
* No
* The request should only proceed if the entity was not modified after this time.
*
*
* You can add these to a request with {@link RequestOptions#addHeader}
*
* Request Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* state: String(active/draining/inactive) (Optional)
* queues (Optional): [
* String (Optional)
* ]
* capacity: Integer (Optional)
* labels (Optional): {
* String: Object (Required)
* }
* tags (Optional): {
* String: Object (Required)
* }
* channels (Optional): [
* (Optional){
* channelId: String (Required)
* capacityCostPerJob: int (Required)
* maxNumberOfJobs: Integer (Optional)
* }
* ]
* offers (Optional): [
* (Optional){
* offerId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* offeredAt: OffsetDateTime (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* assignedJobs (Optional): [
* (Optional){
* assignmentId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* assignedAt: OffsetDateTime (Required)
* }
* ]
* loadRatio: Double (Optional)
* availableForOffers: Boolean (Optional)
* }
* }
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* state: String(active/draining/inactive) (Optional)
* queues (Optional): [
* String (Optional)
* ]
* capacity: Integer (Optional)
* labels (Optional): {
* String: Object (Required)
* }
* tags (Optional): {
* String: Object (Required)
* }
* channels (Optional): [
* (Optional){
* channelId: String (Required)
* capacityCostPerJob: int (Required)
* maxNumberOfJobs: Integer (Optional)
* }
* ]
* offers (Optional): [
* (Optional){
* offerId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* offeredAt: OffsetDateTime (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* assignedJobs (Optional): [
* (Optional){
* assignmentId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* assignedAt: OffsetDateTime (Required)
* }
* ]
* loadRatio: Double (Optional)
* availableForOffers: Boolean (Optional)
* }
* }
*
* @param workerId Id of a worker.
* @param resource The resource instance.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return an entity for jobs to be routed to along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> upsertWorkerWithResponseAsync(String workerId, BinaryData resource,
RequestOptions requestOptions) {
final String contentType = "application/merge-patch+json";
final String accept = "application/json";
return FluxUtil.withContext(context -> service.upsertWorker(this.getEndpoint(),
this.getServiceVersion().getVersion(), workerId, contentType, accept, resource, requestOptions, context));
}
/**
* Creates or updates a worker.
*
* Header Parameters
*
*
* Header Parameters
*
* Name
* Type
* Required
* Description
*
*
* If-Match
* String
* No
* The request should only proceed if an entity matches this string.
*
*
* If-Unmodified-Since
* OffsetDateTime
* No
* The request should only proceed if the entity was not modified after this time.
*
*
* You can add these to a request with {@link RequestOptions#addHeader}
*
* Request Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* state: String(active/draining/inactive) (Optional)
* queues (Optional): [
* String (Optional)
* ]
* capacity: Integer (Optional)
* labels (Optional): {
* String: Object (Required)
* }
* tags (Optional): {
* String: Object (Required)
* }
* channels (Optional): [
* (Optional){
* channelId: String (Required)
* capacityCostPerJob: int (Required)
* maxNumberOfJobs: Integer (Optional)
* }
* ]
* offers (Optional): [
* (Optional){
* offerId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* offeredAt: OffsetDateTime (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* assignedJobs (Optional): [
* (Optional){
* assignmentId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* assignedAt: OffsetDateTime (Required)
* }
* ]
* loadRatio: Double (Optional)
* availableForOffers: Boolean (Optional)
* }
* }
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* state: String(active/draining/inactive) (Optional)
* queues (Optional): [
* String (Optional)
* ]
* capacity: Integer (Optional)
* labels (Optional): {
* String: Object (Required)
* }
* tags (Optional): {
* String: Object (Required)
* }
* channels (Optional): [
* (Optional){
* channelId: String (Required)
* capacityCostPerJob: int (Required)
* maxNumberOfJobs: Integer (Optional)
* }
* ]
* offers (Optional): [
* (Optional){
* offerId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* offeredAt: OffsetDateTime (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* assignedJobs (Optional): [
* (Optional){
* assignmentId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* assignedAt: OffsetDateTime (Required)
* }
* ]
* loadRatio: Double (Optional)
* availableForOffers: Boolean (Optional)
* }
* }
*
* @param workerId Id of a worker.
* @param resource The resource instance.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return an entity for jobs to be routed to along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response upsertWorkerWithResponse(String workerId, BinaryData resource,
RequestOptions requestOptions) {
final String contentType = "application/merge-patch+json";
final String accept = "application/json";
return service.upsertWorkerSync(this.getEndpoint(), this.getServiceVersion().getVersion(), workerId,
contentType, accept, resource, requestOptions, Context.NONE);
}
/**
* Retrieves an existing worker by Id.
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* state: String(active/draining/inactive) (Optional)
* queues (Optional): [
* String (Optional)
* ]
* capacity: Integer (Optional)
* labels (Optional): {
* String: Object (Required)
* }
* tags (Optional): {
* String: Object (Required)
* }
* channels (Optional): [
* (Optional){
* channelId: String (Required)
* capacityCostPerJob: int (Required)
* maxNumberOfJobs: Integer (Optional)
* }
* ]
* offers (Optional): [
* (Optional){
* offerId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* offeredAt: OffsetDateTime (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* assignedJobs (Optional): [
* (Optional){
* assignmentId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* assignedAt: OffsetDateTime (Required)
* }
* ]
* loadRatio: Double (Optional)
* availableForOffers: Boolean (Optional)
* }
* }
*
* @param workerId Id of a worker.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return an entity for jobs to be routed to along with {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getWorkerWithResponseAsync(String workerId, RequestOptions requestOptions) {
final String accept = "application/json";
return FluxUtil.withContext(context -> service.getWorker(this.getEndpoint(),
this.getServiceVersion().getVersion(), workerId, accept, requestOptions, context));
}
/**
* Retrieves an existing worker by Id.
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* state: String(active/draining/inactive) (Optional)
* queues (Optional): [
* String (Optional)
* ]
* capacity: Integer (Optional)
* labels (Optional): {
* String: Object (Required)
* }
* tags (Optional): {
* String: Object (Required)
* }
* channels (Optional): [
* (Optional){
* channelId: String (Required)
* capacityCostPerJob: int (Required)
* maxNumberOfJobs: Integer (Optional)
* }
* ]
* offers (Optional): [
* (Optional){
* offerId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* offeredAt: OffsetDateTime (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* assignedJobs (Optional): [
* (Optional){
* assignmentId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* assignedAt: OffsetDateTime (Required)
* }
* ]
* loadRatio: Double (Optional)
* availableForOffers: Boolean (Optional)
* }
* }
*
* @param workerId Id of a worker.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return an entity for jobs to be routed to along with {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response getWorkerWithResponse(String workerId, RequestOptions requestOptions) {
final String accept = "application/json";
return service.getWorkerSync(this.getEndpoint(), this.getServiceVersion().getVersion(), workerId, accept,
requestOptions, Context.NONE);
}
/**
* Deletes a worker and all of its traces.
*
* @param workerId Id of a worker.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return the {@link Response} on successful completion of {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> deleteWorkerWithResponseAsync(String workerId, RequestOptions requestOptions) {
final String accept = "application/json";
return FluxUtil.withContext(context -> service.deleteWorker(this.getEndpoint(),
this.getServiceVersion().getVersion(), workerId, accept, requestOptions, context));
}
/**
* Deletes a worker and all of its traces.
*
* @param workerId Id of a worker.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return the {@link Response}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Response deleteWorkerWithResponse(String workerId, RequestOptions requestOptions) {
final String accept = "application/json";
return service.deleteWorkerSync(this.getEndpoint(), this.getServiceVersion().getVersion(), workerId, accept,
requestOptions, Context.NONE);
}
/**
* Retrieves existing workers.
*
* Query Parameters
*
*
* Query Parameters
*
* Name
* Type
* Required
* Description
*
*
* maxpagesize
* Integer
* No
* Number of objects to return per page.
*
*
* state
* String
* No
* If specified, select workers by worker state. Allowed values: "active", "draining", "inactive", "all".
*
*
* channelId
* String
* No
* If specified, select workers who have a channel configuration with this channel.
*
*
* queueId
* String
* No
* If specified, select workers who are assigned to this queue.
*
*
* hasCapacity
* Boolean
* No
* If set to true, select only workers who have capacity for the channel specified by `channelId` or for any
* channel if `channelId` not specified. If set to false, then will return all workers including workers without any
* capacity for jobs. Defaults to false.
*
*
* You can add these to a request with {@link RequestOptions#addQueryParam}
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* state: String(active/draining/inactive) (Optional)
* queues (Optional): [
* String (Optional)
* ]
* capacity: Integer (Optional)
* labels (Optional): {
* String: Object (Required)
* }
* tags (Optional): {
* String: Object (Required)
* }
* channels (Optional): [
* (Optional){
* channelId: String (Required)
* capacityCostPerJob: int (Required)
* maxNumberOfJobs: Integer (Optional)
* }
* ]
* offers (Optional): [
* (Optional){
* offerId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* offeredAt: OffsetDateTime (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* assignedJobs (Optional): [
* (Optional){
* assignmentId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* assignedAt: OffsetDateTime (Required)
* }
* ]
* loadRatio: Double (Optional)
* availableForOffers: Boolean (Optional)
* }
* }
*
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return paged collection of RouterWorker items along with {@link PagedResponse} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listWorkersSinglePageAsync(RequestOptions requestOptions) {
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.listWorkers(this.getEndpoint(), this.getServiceVersion().getVersion(),
accept, requestOptions, context))
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
getValues(res.getValue(), "value"), getNextLink(res.getValue(), "nextLink"), null));
}
/**
* Retrieves existing workers.
*
* Query Parameters
*
*
* Query Parameters
*
* Name
* Type
* Required
* Description
*
*
* maxpagesize
* Integer
* No
* Number of objects to return per page.
*
*
* state
* String
* No
* If specified, select workers by worker state. Allowed values: "active", "draining", "inactive", "all".
*
*
* channelId
* String
* No
* If specified, select workers who have a channel configuration with this channel.
*
*
* queueId
* String
* No
* If specified, select workers who are assigned to this queue.
*
*
* hasCapacity
* Boolean
* No
* If set to true, select only workers who have capacity for the channel specified by `channelId` or for any
* channel if `channelId` not specified. If set to false, then will return all workers including workers without any
* capacity for jobs. Defaults to false.
*
*
* You can add these to a request with {@link RequestOptions#addQueryParam}
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* state: String(active/draining/inactive) (Optional)
* queues (Optional): [
* String (Optional)
* ]
* capacity: Integer (Optional)
* labels (Optional): {
* String: Object (Required)
* }
* tags (Optional): {
* String: Object (Required)
* }
* channels (Optional): [
* (Optional){
* channelId: String (Required)
* capacityCostPerJob: int (Required)
* maxNumberOfJobs: Integer (Optional)
* }
* ]
* offers (Optional): [
* (Optional){
* offerId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* offeredAt: OffsetDateTime (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* assignedJobs (Optional): [
* (Optional){
* assignmentId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* assignedAt: OffsetDateTime (Required)
* }
* ]
* loadRatio: Double (Optional)
* availableForOffers: Boolean (Optional)
* }
* }
*
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return paged collection of RouterWorker items as paginated response with {@link PagedFlux}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedFlux listWorkersAsync(RequestOptions requestOptions) {
RequestOptions requestOptionsForNextPage = new RequestOptions();
requestOptionsForNextPage.setContext(
requestOptions != null && requestOptions.getContext() != null ? requestOptions.getContext() : Context.NONE);
return new PagedFlux<>((pageSize) -> {
RequestOptions requestOptionsLocal = requestOptions == null ? new RequestOptions() : requestOptions;
if (pageSize != null) {
requestOptionsLocal.addRequestCallback(requestLocal -> {
UrlBuilder urlBuilder = UrlBuilder.parse(requestLocal.getUrl());
urlBuilder.setQueryParameter("maxpagesize", String.valueOf(pageSize));
requestLocal.setUrl(urlBuilder.toString());
});
}
return listWorkersSinglePageAsync(requestOptionsLocal);
}, (nextLink, pageSize) -> {
RequestOptions requestOptionsLocal = new RequestOptions();
requestOptionsLocal.setContext(requestOptionsForNextPage.getContext());
if (pageSize != null) {
requestOptionsLocal.addRequestCallback(requestLocal -> {
UrlBuilder urlBuilder = UrlBuilder.parse(requestLocal.getUrl());
urlBuilder.setQueryParameter("maxpagesize", String.valueOf(pageSize));
requestLocal.setUrl(urlBuilder.toString());
});
}
return listWorkersNextSinglePageAsync(nextLink, requestOptionsLocal);
});
}
/**
* Retrieves existing workers.
*
* Query Parameters
*
*
* Query Parameters
*
* Name
* Type
* Required
* Description
*
*
* maxpagesize
* Integer
* No
* Number of objects to return per page.
*
*
* state
* String
* No
* If specified, select workers by worker state. Allowed values: "active", "draining", "inactive", "all".
*
*
* channelId
* String
* No
* If specified, select workers who have a channel configuration with this channel.
*
*
* queueId
* String
* No
* If specified, select workers who are assigned to this queue.
*
*
* hasCapacity
* Boolean
* No
* If set to true, select only workers who have capacity for the channel specified by `channelId` or for any
* channel if `channelId` not specified. If set to false, then will return all workers including workers without any
* capacity for jobs. Defaults to false.
*
*
* You can add these to a request with {@link RequestOptions#addQueryParam}
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* state: String(active/draining/inactive) (Optional)
* queues (Optional): [
* String (Optional)
* ]
* capacity: Integer (Optional)
* labels (Optional): {
* String: Object (Required)
* }
* tags (Optional): {
* String: Object (Required)
* }
* channels (Optional): [
* (Optional){
* channelId: String (Required)
* capacityCostPerJob: int (Required)
* maxNumberOfJobs: Integer (Optional)
* }
* ]
* offers (Optional): [
* (Optional){
* offerId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* offeredAt: OffsetDateTime (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* assignedJobs (Optional): [
* (Optional){
* assignmentId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* assignedAt: OffsetDateTime (Required)
* }
* ]
* loadRatio: Double (Optional)
* availableForOffers: Boolean (Optional)
* }
* }
*
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return paged collection of RouterWorker items along with {@link PagedResponse}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private PagedResponse listWorkersSinglePage(RequestOptions requestOptions) {
final String accept = "application/json";
Response res = service.listWorkersSync(this.getEndpoint(), this.getServiceVersion().getVersion(),
accept, requestOptions, Context.NONE);
return new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
getValues(res.getValue(), "value"), getNextLink(res.getValue(), "nextLink"), null);
}
/**
* Retrieves existing workers.
*
* Query Parameters
*
*
* Query Parameters
*
* Name
* Type
* Required
* Description
*
*
* maxpagesize
* Integer
* No
* Number of objects to return per page.
*
*
* state
* String
* No
* If specified, select workers by worker state. Allowed values: "active", "draining", "inactive", "all".
*
*
* channelId
* String
* No
* If specified, select workers who have a channel configuration with this channel.
*
*
* queueId
* String
* No
* If specified, select workers who are assigned to this queue.
*
*
* hasCapacity
* Boolean
* No
* If set to true, select only workers who have capacity for the channel specified by `channelId` or for any
* channel if `channelId` not specified. If set to false, then will return all workers including workers without any
* capacity for jobs. Defaults to false.
*
*
* You can add these to a request with {@link RequestOptions#addQueryParam}
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* state: String(active/draining/inactive) (Optional)
* queues (Optional): [
* String (Optional)
* ]
* capacity: Integer (Optional)
* labels (Optional): {
* String: Object (Required)
* }
* tags (Optional): {
* String: Object (Required)
* }
* channels (Optional): [
* (Optional){
* channelId: String (Required)
* capacityCostPerJob: int (Required)
* maxNumberOfJobs: Integer (Optional)
* }
* ]
* offers (Optional): [
* (Optional){
* offerId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* offeredAt: OffsetDateTime (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* assignedJobs (Optional): [
* (Optional){
* assignmentId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* assignedAt: OffsetDateTime (Required)
* }
* ]
* loadRatio: Double (Optional)
* availableForOffers: Boolean (Optional)
* }
* }
*
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return paged collection of RouterWorker items as paginated response with {@link PagedIterable}.
*/
@ServiceMethod(returns = ReturnType.COLLECTION)
public PagedIterable listWorkers(RequestOptions requestOptions) {
RequestOptions requestOptionsForNextPage = new RequestOptions();
requestOptionsForNextPage.setContext(
requestOptions != null && requestOptions.getContext() != null ? requestOptions.getContext() : Context.NONE);
return new PagedIterable<>((pageSize) -> {
RequestOptions requestOptionsLocal = requestOptions == null ? new RequestOptions() : requestOptions;
if (pageSize != null) {
requestOptionsLocal.addRequestCallback(requestLocal -> {
UrlBuilder urlBuilder = UrlBuilder.parse(requestLocal.getUrl());
urlBuilder.setQueryParameter("maxpagesize", String.valueOf(pageSize));
requestLocal.setUrl(urlBuilder.toString());
});
}
return listWorkersSinglePage(requestOptionsLocal);
}, (nextLink, pageSize) -> {
RequestOptions requestOptionsLocal = new RequestOptions();
requestOptionsLocal.setContext(requestOptionsForNextPage.getContext());
if (pageSize != null) {
requestOptionsLocal.addRequestCallback(requestLocal -> {
UrlBuilder urlBuilder = UrlBuilder.parse(requestLocal.getUrl());
urlBuilder.setQueryParameter("maxpagesize", String.valueOf(pageSize));
requestLocal.setUrl(urlBuilder.toString());
});
}
return listWorkersNextSinglePage(nextLink, requestOptionsLocal);
});
}
/**
* Retrieves list of jobs based on filter parameters.
*
* Get the next page of items.
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* channelReference: String (Optional)
* status: String(pendingClassification/queued/assigned/completed/closed/cancelled/classificationFailed/created/pendingSchedule/scheduled/scheduleFailed/waitingForActivation) (Optional)
* enqueuedAt: OffsetDateTime (Optional)
* channelId: String (Optional)
* classificationPolicyId: String (Optional)
* queueId: String (Optional)
* priority: Integer (Optional)
* dispositionCode: String (Optional)
* requestedWorkerSelectors (Optional): [
* (Optional){
* key: String (Required)
* labelOperator: String(equal/notEqual/lessThan/lessThanOrEqual/greaterThan/greaterThanOrEqual) (Required)
* value: Object (Optional)
* expiresAfterSeconds: Double (Optional)
* expedite: Boolean (Optional)
* status: String(active/expired) (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* attachedWorkerSelectors (Optional): [
* (recursive schema, see above)
* ]
* labels (Optional): {
* String: Object (Required)
* }
* assignments (Optional): {
* String (Required): {
* assignmentId: String (Required)
* workerId: String (Optional)
* assignedAt: OffsetDateTime (Required)
* completedAt: OffsetDateTime (Optional)
* closedAt: OffsetDateTime (Optional)
* }
* }
* tags (Optional): {
* String: Object (Required)
* }
* notes (Optional): [
* (Optional){
* message: String (Required)
* addedAt: OffsetDateTime (Optional)
* }
* ]
* scheduledAt: OffsetDateTime (Optional)
* matchingMode (Optional): {
* }
* }
* }
*
* @param nextLink The URL to get the next list of items
*
* The nextLink parameter.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return paged collection of RouterJob items along with {@link PagedResponse} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listJobsNextSinglePageAsync(String nextLink,
RequestOptions requestOptions) {
final String accept = "application/json";
return FluxUtil
.withContext(context -> service.listJobsNext(nextLink, this.getEndpoint(), accept, requestOptions, context))
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
getValues(res.getValue(), "value"), getNextLink(res.getValue(), "nextLink"), null));
}
/**
* Retrieves list of jobs based on filter parameters.
*
* Get the next page of items.
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* channelReference: String (Optional)
* status: String(pendingClassification/queued/assigned/completed/closed/cancelled/classificationFailed/created/pendingSchedule/scheduled/scheduleFailed/waitingForActivation) (Optional)
* enqueuedAt: OffsetDateTime (Optional)
* channelId: String (Optional)
* classificationPolicyId: String (Optional)
* queueId: String (Optional)
* priority: Integer (Optional)
* dispositionCode: String (Optional)
* requestedWorkerSelectors (Optional): [
* (Optional){
* key: String (Required)
* labelOperator: String(equal/notEqual/lessThan/lessThanOrEqual/greaterThan/greaterThanOrEqual) (Required)
* value: Object (Optional)
* expiresAfterSeconds: Double (Optional)
* expedite: Boolean (Optional)
* status: String(active/expired) (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* attachedWorkerSelectors (Optional): [
* (recursive schema, see above)
* ]
* labels (Optional): {
* String: Object (Required)
* }
* assignments (Optional): {
* String (Required): {
* assignmentId: String (Required)
* workerId: String (Optional)
* assignedAt: OffsetDateTime (Required)
* completedAt: OffsetDateTime (Optional)
* closedAt: OffsetDateTime (Optional)
* }
* }
* tags (Optional): {
* String: Object (Required)
* }
* notes (Optional): [
* (Optional){
* message: String (Required)
* addedAt: OffsetDateTime (Optional)
* }
* ]
* scheduledAt: OffsetDateTime (Optional)
* matchingMode (Optional): {
* }
* }
* }
*
* @param nextLink The URL to get the next list of items
*
* The nextLink parameter.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return paged collection of RouterJob items along with {@link PagedResponse}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private PagedResponse listJobsNextSinglePage(String nextLink, RequestOptions requestOptions) {
final String accept = "application/json";
Response res
= service.listJobsNextSync(nextLink, this.getEndpoint(), accept, requestOptions, Context.NONE);
return new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
getValues(res.getValue(), "value"), getNextLink(res.getValue(), "nextLink"), null);
}
/**
* Retrieves existing workers.
*
* Get the next page of items.
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* state: String(active/draining/inactive) (Optional)
* queues (Optional): [
* String (Optional)
* ]
* capacity: Integer (Optional)
* labels (Optional): {
* String: Object (Required)
* }
* tags (Optional): {
* String: Object (Required)
* }
* channels (Optional): [
* (Optional){
* channelId: String (Required)
* capacityCostPerJob: int (Required)
* maxNumberOfJobs: Integer (Optional)
* }
* ]
* offers (Optional): [
* (Optional){
* offerId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* offeredAt: OffsetDateTime (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* assignedJobs (Optional): [
* (Optional){
* assignmentId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* assignedAt: OffsetDateTime (Required)
* }
* ]
* loadRatio: Double (Optional)
* availableForOffers: Boolean (Optional)
* }
* }
*
* @param nextLink The URL to get the next list of items
*
* The nextLink parameter.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return paged collection of RouterWorker items along with {@link PagedResponse} on successful completion of
* {@link Mono}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private Mono> listWorkersNextSinglePageAsync(String nextLink,
RequestOptions requestOptions) {
final String accept = "application/json";
return FluxUtil
.withContext(
context -> service.listWorkersNext(nextLink, this.getEndpoint(), accept, requestOptions, context))
.map(res -> new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
getValues(res.getValue(), "value"), getNextLink(res.getValue(), "nextLink"), null));
}
/**
* Retrieves existing workers.
*
* Get the next page of items.
*
* Response Body Schema
*
* {@code
* {
* etag: String (Required)
* id: String (Required)
* state: String(active/draining/inactive) (Optional)
* queues (Optional): [
* String (Optional)
* ]
* capacity: Integer (Optional)
* labels (Optional): {
* String: Object (Required)
* }
* tags (Optional): {
* String: Object (Required)
* }
* channels (Optional): [
* (Optional){
* channelId: String (Required)
* capacityCostPerJob: int (Required)
* maxNumberOfJobs: Integer (Optional)
* }
* ]
* offers (Optional): [
* (Optional){
* offerId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* offeredAt: OffsetDateTime (Optional)
* expiresAt: OffsetDateTime (Optional)
* }
* ]
* assignedJobs (Optional): [
* (Optional){
* assignmentId: String (Required)
* jobId: String (Required)
* capacityCost: int (Required)
* assignedAt: OffsetDateTime (Required)
* }
* ]
* loadRatio: Double (Optional)
* availableForOffers: Boolean (Optional)
* }
* }
*
* @param nextLink The URL to get the next list of items
*
* The nextLink parameter.
* @param requestOptions The options to configure the HTTP request before HTTP client sends it.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws ClientAuthenticationException thrown if the request is rejected by server on status code 401.
* @throws ResourceNotFoundException thrown if the request is rejected by server on status code 404.
* @throws ResourceModifiedException thrown if the request is rejected by server on status code 409.
* @return paged collection of RouterWorker items along with {@link PagedResponse}.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
private PagedResponse listWorkersNextSinglePage(String nextLink, RequestOptions requestOptions) {
final String accept = "application/json";
Response res
= service.listWorkersNextSync(nextLink, this.getEndpoint(), accept, requestOptions, Context.NONE);
return new PagedResponseBase<>(res.getRequest(), res.getStatusCode(), res.getHeaders(),
getValues(res.getValue(), "value"), getNextLink(res.getValue(), "nextLink"), null);
}
private List getValues(BinaryData binaryData, String path) {
try {
Map, ?> obj = binaryData.toObject(Map.class);
List> values = (List>) obj.get(path);
return values.stream().map(BinaryData::fromObject).collect(Collectors.toList());
} catch (RuntimeException e) {
return null;
}
}
private String getNextLink(BinaryData binaryData, String path) {
try {
Map, ?> obj = binaryData.toObject(Map.class);
return (String) obj.get(path);
} catch (RuntimeException e) {
return null;
}
}
}