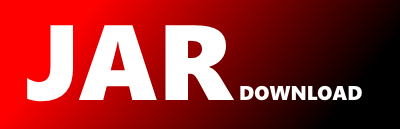
com.azure.communication.jobrouter.implementation.models.DistributionModeInternal Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-communication-jobrouter Show documentation
Show all versions of azure-communication-jobrouter Show documentation
This package contains a Java SDK for JobRouter Azure Communication Service.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) TypeSpec Code Generator.
package com.azure.communication.jobrouter.implementation.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.annotation.Generated;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonSubTypes;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* Abstract base class for defining a distribution mode.
*/
@JsonTypeInfo(
use = JsonTypeInfo.Id.NAME,
include = JsonTypeInfo.As.PROPERTY,
property = "kind",
defaultImpl = DistributionModeInternal.class)
@JsonTypeName("DistributionModeInternal")
@JsonSubTypes({
@JsonSubTypes.Type(name = "bestWorker", value = BestWorkerModeInternal.class),
@JsonSubTypes.Type(name = "longestIdle", value = LongestIdleModeInternal.class),
@JsonSubTypes.Type(name = "roundRobin", value = RoundRobinModeInternal.class) })
@Fluent
public class DistributionModeInternal {
/*
* Governs the minimum desired number of active concurrent offers a job can have.
*/
@Generated
@JsonProperty(value = "minConcurrentOffers")
private Integer minConcurrentOffers;
/*
* Governs the maximum number of active concurrent offers a job can have.
*/
@Generated
@JsonProperty(value = "maxConcurrentOffers")
private Integer maxConcurrentOffers;
/*
* If set to true, then router will match workers to jobs even if they don't match label selectors. Warning: You
* may get workers that are not qualified for a job they are matched with if you set this variable to true. This
* flag is intended more for temporary usage. By default, set to false.
*/
@Generated
@JsonProperty(value = "bypassSelectors")
private Boolean bypassSelectors;
/**
* Creates an instance of DistributionModeInternal class.
*/
@Generated
public DistributionModeInternal() {
}
/**
* Get the minConcurrentOffers property: Governs the minimum desired number of active concurrent offers a job can
* have.
*
* @return the minConcurrentOffers value.
*/
@Generated
public Integer getMinConcurrentOffers() {
return this.minConcurrentOffers;
}
/**
* Set the minConcurrentOffers property: Governs the minimum desired number of active concurrent offers a job can
* have.
*
* @param minConcurrentOffers the minConcurrentOffers value to set.
* @return the DistributionModeInternal object itself.
*/
@Generated
public DistributionModeInternal setMinConcurrentOffers(Integer minConcurrentOffers) {
this.minConcurrentOffers = minConcurrentOffers;
return this;
}
/**
* Get the maxConcurrentOffers property: Governs the maximum number of active concurrent offers a job can have.
*
* @return the maxConcurrentOffers value.
*/
@Generated
public Integer getMaxConcurrentOffers() {
return this.maxConcurrentOffers;
}
/**
* Set the maxConcurrentOffers property: Governs the maximum number of active concurrent offers a job can have.
*
* @param maxConcurrentOffers the maxConcurrentOffers value to set.
* @return the DistributionModeInternal object itself.
*/
@Generated
public DistributionModeInternal setMaxConcurrentOffers(Integer maxConcurrentOffers) {
this.maxConcurrentOffers = maxConcurrentOffers;
return this;
}
/**
* Get the bypassSelectors property: If set to true, then router will match workers to jobs even if they don't
* match label selectors. Warning: You may get workers that are not qualified for a job they are matched with if
* you set this variable to true. This flag is intended more for temporary usage. By default, set to false.
*
* @return the bypassSelectors value.
*/
@Generated
public Boolean isBypassSelectors() {
return this.bypassSelectors;
}
/**
* Set the bypassSelectors property: If set to true, then router will match workers to jobs even if they don't
* match label selectors. Warning: You may get workers that are not qualified for a job they are matched with if
* you set this variable to true. This flag is intended more for temporary usage. By default, set to false.
*
* @param bypassSelectors the bypassSelectors value to set.
* @return the DistributionModeInternal object itself.
*/
@Generated
public DistributionModeInternal setBypassSelectors(Boolean bypassSelectors) {
this.bypassSelectors = bypassSelectors;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy