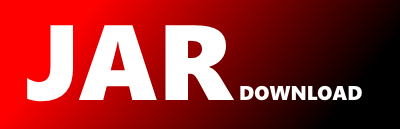
com.azure.communication.jobrouter.models.DistributionPolicy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-communication-jobrouter Show documentation
Show all versions of azure-communication-jobrouter Show documentation
This package contains a Java SDK for JobRouter Azure Communication Service.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.communication.jobrouter.models;
import com.azure.communication.jobrouter.implementation.accesshelpers.DistributionPolicyConstructorProxy;
import com.azure.communication.jobrouter.implementation.converters.DistributionPolicyAdapter;
import com.azure.communication.jobrouter.implementation.models.DistributionPolicyInternal;
import com.azure.core.annotation.Fluent;
import com.azure.core.annotation.Generated;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.time.Duration;
/** Policy governing how jobs are distributed to workers. */
@Fluent
public final class DistributionPolicy {
/*
* The unique identifier of the policy.
*/
@Generated
@JsonProperty(value = "id", access = JsonProperty.Access.WRITE_ONLY)
private String id;
/*
* The human readable name of the policy.
*/
@Generated
@JsonProperty(value = "name")
private String name;
/*
* The duration after which any offers created under this policy will be
* expired.
*/
private Duration offerExpiresAfter;
/*
* Abstract base class for defining a distribution mode
*/
@Generated
@JsonProperty(value = "mode")
private DistributionMode mode;
/** Creates an instance of DistributionPolicy class. */
@Generated
public DistributionPolicy() {
}
/**
* Package-private constructor of the class, used internally.
*
* @param internal The internal DistributionPolicy
*/
DistributionPolicy(DistributionPolicyInternal internal) {
id = internal.getId();
setName(internal.getName());
setMode(DistributionPolicyAdapter.convertDistributionModeToPublic(internal.getMode()));
setOfferExpiresAfter(Duration.ofSeconds(internal.getOfferExpiresAfterSeconds().longValue()));
}
static {
DistributionPolicyConstructorProxy.setAccessor(internal -> new DistributionPolicy(internal));
}
/**
* Get the id property: The unique identifier of the policy.
*
* @return the id value.
*/
@Generated
public String getId() {
return this.id;
}
/**
* Get the name property: The human readable name of the policy.
*
* @return the name value.
*/
@Generated
public String getName() {
return this.name;
}
/**
* Set the name property: The human readable name of the policy.
*
* @param name the name value to set.
* @return the DistributionPolicy object itself.
*/
@Generated
public DistributionPolicy setName(String name) {
this.name = name;
return this;
}
/**
* Get the offerExpiresAfter property: The duration after which any offers created under this policy
* will be expired.
*
* @return the offerExpiresAfter value.
*/
public Duration getOfferExpiresAfter() {
return this.offerExpiresAfter;
}
/**
* Set the offerExpiresAfter property: The duration after which any offers created under this policy
* will be expired.
*
* @param offerExpiresAfter the offerExpiresAfter value to set.
* @return the DistributionPolicy object itself.
*/
public DistributionPolicy setOfferExpiresAfter(Duration offerExpiresAfter) {
this.offerExpiresAfter = offerExpiresAfter;
return this;
}
/**
* Get the mode property: Abstract base class for defining a distribution mode.
*
* @return the mode value.
*/
@Generated
public DistributionMode getMode() {
return this.mode;
}
/**
* Set the mode property: Abstract base class for defining a distribution mode.
*
* @param mode the mode value to set.
* @return the DistributionPolicy object itself.
*/
@Generated
public DistributionPolicy setMode(DistributionMode mode) {
this.mode = mode;
return this;
}
/*
* Concurrency Token.
*/
@Generated
@JsonProperty(value = "etag", access = JsonProperty.Access.WRITE_ONLY)
private String etag;
/**
* Get the etag property: Concurrency Token.
*
* @return the etag value.
*/
@Generated
public String getEtag() {
return this.etag;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy