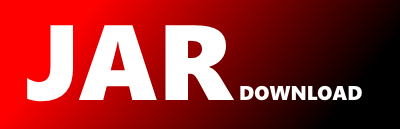
com.azure.communication.messages.models.MessageTemplateVideo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-communication-messages Show documentation
Show all versions of azure-communication-messages Show documentation
This package contains a Java SDK for Azure Communication Messages Services.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) TypeSpec Code Generator.
package com.azure.communication.messages.models;
import com.azure.core.annotation.Fluent;
import com.azure.core.annotation.Generated;
import com.azure.json.JsonReader;
import com.azure.json.JsonToken;
import com.azure.json.JsonWriter;
import java.io.IOException;
/**
* The message template's video value information.
*/
@Fluent
public final class MessageTemplateVideo extends MessageTemplateValue {
/*
* The type discriminator describing a template parameter type.
*/
@Generated
private MessageTemplateValueKind kind = MessageTemplateValueKind.VIDEO;
/*
* The (public) URL of the media.
*/
@Generated
private final String url;
/*
* The [optional] caption of the media object.
*/
@Generated
private String caption;
/*
* The [optional] filename of the media file.
*/
@Generated
private String fileName;
/**
* Creates an instance of MessageTemplateVideo class.
*
* @param refValue the refValue value to set.
* @param url the url value to set.
*/
@Generated
public MessageTemplateVideo(String refValue, String url) {
super(refValue);
this.url = url;
}
/**
* Get the kind property: The type discriminator describing a template parameter type.
*
* @return the kind value.
*/
@Generated
@Override
public MessageTemplateValueKind getKind() {
return this.kind;
}
/**
* Get the url property: The (public) URL of the media.
*
* @return the url value.
*/
@Generated
public String getUrl() {
return this.url;
}
/**
* Get the caption property: The [optional] caption of the media object.
*
* @return the caption value.
*/
@Generated
public String getCaption() {
return this.caption;
}
/**
* Set the caption property: The [optional] caption of the media object.
*
* @param caption the caption value to set.
* @return the MessageTemplateVideo object itself.
*/
@Generated
public MessageTemplateVideo setCaption(String caption) {
this.caption = caption;
return this;
}
/**
* Get the fileName property: The [optional] filename of the media file.
*
* @return the fileName value.
*/
@Generated
public String getFileName() {
return this.fileName;
}
/**
* Set the fileName property: The [optional] filename of the media file.
*
* @param fileName the fileName value to set.
* @return the MessageTemplateVideo object itself.
*/
@Generated
public MessageTemplateVideo setFileName(String fileName) {
this.fileName = fileName;
return this;
}
/**
* {@inheritDoc}
*/
@Generated
@Override
public JsonWriter toJson(JsonWriter jsonWriter) throws IOException {
jsonWriter.writeStartObject();
jsonWriter.writeStringField("name", getRefValue());
jsonWriter.writeStringField("url", this.url);
jsonWriter.writeStringField("kind", this.kind == null ? null : this.kind.toString());
jsonWriter.writeStringField("caption", this.caption);
jsonWriter.writeStringField("fileName", this.fileName);
return jsonWriter.writeEndObject();
}
/**
* Reads an instance of MessageTemplateVideo from the JsonReader.
*
* @param jsonReader The JsonReader being read.
* @return An instance of MessageTemplateVideo if the JsonReader was pointing to an instance of it, or null if it
* was pointing to JSON null.
* @throws IllegalStateException If the deserialized JSON object was missing any required properties.
* @throws IOException If an error occurs while reading the MessageTemplateVideo.
*/
@Generated
public static MessageTemplateVideo fromJson(JsonReader jsonReader) throws IOException {
return jsonReader.readObject(reader -> {
String refValue = null;
String url = null;
MessageTemplateValueKind kind = MessageTemplateValueKind.VIDEO;
String caption = null;
String fileName = null;
while (reader.nextToken() != JsonToken.END_OBJECT) {
String fieldName = reader.getFieldName();
reader.nextToken();
if ("name".equals(fieldName)) {
refValue = reader.getString();
} else if ("url".equals(fieldName)) {
url = reader.getString();
} else if ("kind".equals(fieldName)) {
kind = MessageTemplateValueKind.fromString(reader.getString());
} else if ("caption".equals(fieldName)) {
caption = reader.getString();
} else if ("fileName".equals(fieldName)) {
fileName = reader.getString();
} else {
reader.skipChildren();
}
}
MessageTemplateVideo deserializedMessageTemplateVideo = new MessageTemplateVideo(refValue, url);
deserializedMessageTemplateVideo.kind = kind;
deserializedMessageTemplateVideo.caption = caption;
deserializedMessageTemplateVideo.fileName = fileName;
return deserializedMessageTemplateVideo;
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy