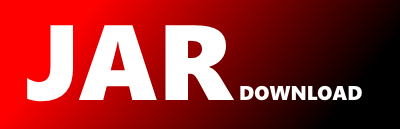
com.azure.communication.networktraversal.models.CommunicationIceServer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-communication-networktraversal Show documentation
Show all versions of azure-communication-networktraversal Show documentation
Please note, this package has been deprecated and will no longer be maintained after 03/31/2024.
Refer to our deprecation policy (https://aka.ms/azsdk/support-policies) for more details.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.communication.networktraversal.models;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.List;
/** An instance of a STUN/TURN server with credentials to be used for ICE negotiation. */
public final class CommunicationIceServer {
/*
* List of STUN/TURN server URLs.
*/
@JsonProperty(value = "urls", required = true)
private List urls;
/*
* User account name which uniquely identifies the credentials.
*/
@JsonProperty(value = "username", required = true)
private String username;
/*
* Credential for the server.
*/
@JsonProperty(value = "credential", required = true)
private String credential;
/*
* The routing methodology to where the ICE server will be located from the
* client. "any" will have higher reliability while "nearest" will have
* lower latency. It is recommended to default to use the "any" routing
* method unless there are specific scenarios which minimizing latency is
* critical.
*/
@JsonProperty(value = "routeType", required = true)
private RouteType routeType;
/**
* Default constructor for CommunicationIceServer.
*/
public CommunicationIceServer() {
}
/**
* Get the urls property: List of STUN/TURN server URLs.
*
* @return the urls value.
*/
public List getUrls() {
return this.urls;
}
/**
* Set the urls property: List of STUN/TURN server URLs.
*
* @param urls the urls value to set.
* @return the CommunicationIceServer object itself.
*/
CommunicationIceServer setUrls(List urls) {
this.urls = urls;
return this;
}
/**
* Get the username property: User account name which uniquely identifies the credentials.
*
* @return the username value.
*/
public String getUsername() {
return this.username;
}
/**
* Set the username property: User account name which uniquely identifies the credentials.
*
* @param username the username value to set.
* @return the CommunicationIceServer object itself.
*/
CommunicationIceServer setUsername(String username) {
this.username = username;
return this;
}
/**
* Get the credential property: Credential for the server.
*
* @return the credential value.
*/
public String getCredential() {
return this.credential;
}
/**
* Set the credential property: Credential for the server.
*
* @param credential the credential value to set.
* @return the CommunicationIceServer object itself.
*/
CommunicationIceServer setCredential(String credential) {
this.credential = credential;
return this;
}
/**
* Get the routeType property: The routing methodology to where the ICE server will be located from the client.
* "any" will have higher reliability while "nearest" will have lower latency. It is recommended to default to use
* the "any" routing method unless there are specific scenarios which minimizing latency is critical.
*
* @return the routeType value.
*/
public RouteType getRouteType() {
return this.routeType;
}
/**
* Set the routeType property: The routing methodology to where the ICE server will be located from the client.
* "any" will have higher reliability while "nearest" will have lower latency. It is recommended to default to use
* the "any" routing method unless there are specific scenarios which minimizing latency is critical.
*
* @param routeType the routeType value to set.
* @return the CommunicationIceServer object itself.
*/
CommunicationIceServer setRouteType(RouteType routeType) {
this.routeType = routeType;
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy