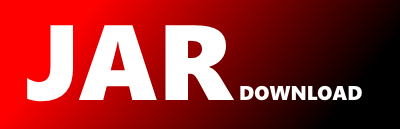
com.azure.core.http.RequestConditions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-core Show documentation
Show all versions of azure-core Show documentation
This package contains core types for Azure Java clients.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.core.http;
import com.azure.core.annotation.Fluent;
import java.time.OffsetDateTime;
/**
* Specifies HTTP options for conditional requests based on modification time.
*/
@Fluent
public class RequestConditions extends MatchConditions {
private OffsetDateTime ifModifiedSince;
private OffsetDateTime ifUnmodifiedSince;
/**
* Creates a new instance of {@link RequestConditions}.
*/
public RequestConditions() {
}
/**
* Optionally limit requests to resources that match the passed ETag.
*
* @param ifMatch ETag that resources must match.
* @return The updated ResourceConditions object.
*/
@Override
public RequestConditions setIfMatch(String ifMatch) {
super.setIfMatch(ifMatch);
return this;
}
/**
* Optionally limit requests to resources that do not match the passed ETag.
*
* @param ifNoneMatch ETag that resources must not match.
* @return The updated ResourceConditions object.
*/
@Override
public RequestConditions setIfNoneMatch(String ifNoneMatch) {
super.setIfNoneMatch(ifNoneMatch);
return this;
}
/**
* Gets the {@link OffsetDateTime datetime} that resources must have been modified since.
*
* @return The datetime that resources must have been modified since.
*/
public OffsetDateTime getIfModifiedSince() {
return ifModifiedSince;
}
/**
* Optionally limit requests to resources that have only been modified since the passed
* {@link OffsetDateTime datetime}.
*
* @param ifModifiedSince The datetime that resources must have been modified since.
* @return The updated ResourceConditions object.
*/
public RequestConditions setIfModifiedSince(OffsetDateTime ifModifiedSince) {
this.ifModifiedSince = ifModifiedSince;
return this;
}
/**
* Gets the {@link OffsetDateTime datetime} that resources must have remained unmodified since.
*
* @return The datetime that resources must have remained unmodified since.
*/
public OffsetDateTime getIfUnmodifiedSince() {
return ifUnmodifiedSince;
}
/**
* Optionally limit requests to resources that have remained unmodified since the passed
* {@link OffsetDateTime datetime}.
*
* @param ifUnmodifiedSince The datetime that resources must have remained unmodified since.
* @return The updated ResourceConditions object.
*/
public RequestConditions setIfUnmodifiedSince(OffsetDateTime ifUnmodifiedSince) {
this.ifUnmodifiedSince = ifUnmodifiedSince;
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy