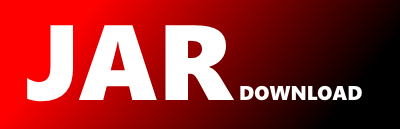
com.azure.core.util.IterableStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-core Show documentation
Show all versions of azure-core Show documentation
This package contains core types for Azure Java clients.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.core.util;
import reactor.core.publisher.Flux;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.Objects;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
/**
* This class provides utility to iterate over values using standard 'for-each' style loops or to convert them into a
* {@link Stream} and operate in that fashion.
*
* Code sample using Stream
*
*
*
* // process the stream
* myIterableStream.stream().forEach(resp -> {
* if (resp.getStatusCode() == HttpURLConnection.HTTP_OK) {
* System.out.printf("Response headers are %s. Url %s%n", resp.getDeserializedHeaders(),
* resp.getRequest().getUrl());
* resp.getElements().forEach(value -> System.out.printf("Response value is %d%n", value));
* }
* });
*
*
*
* Code sample using Iterator
*
*
*
* // Iterate over iterator
* for (PagedResponseBase<String, Integer> resp : myIterableStream) {
* if (resp.getStatusCode() == HttpURLConnection.HTTP_OK) {
* System.out.printf("Response headers are %s. Url %s%n", resp.getDeserializedHeaders(),
* resp.getRequest().getUrl());
* resp.getElements().forEach(value -> System.out.printf("Response value is %d%n", value));
* }
* }
*
*
*
* Code sample using Stream and filter
*
*
*
* // process the stream
* myIterableStream.stream().filter(resp -> resp.getStatusCode() == HttpURLConnection.HTTP_OK)
* .limit(10)
* .forEach(resp -> {
* System.out.printf("Response headers are %s. Url %s%n", resp.getDeserializedHeaders(),
* resp.getRequest().getUrl());
* resp.getElements().forEach(value -> System.out.printf("Response value is %d%n", value));
* });
*
*
*
* @param The type of value in this {@link Iterable}.
* @see Iterable
*/
public class IterableStream implements Iterable {
/*
* This is the default batch size that will be requested when using stream or iterable by page, this will indicate
* to Reactor how many elements should be prefetched before another batch is requested.
*/
private static final int DEFAULT_BATCH_SIZE = 1;
private static final IterableStream
© 2015 - 2024 Weber Informatics LLC | Privacy Policy