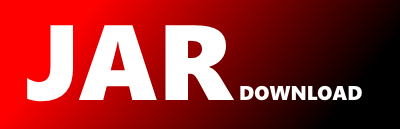
com.azure.identity.implementation.SynchronizedAccessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-identity Show documentation
Show all versions of azure-identity Show documentation
This module contains client library for Microsoft Azure Identity.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.identity.implementation;
import reactor.core.publisher.FluxSink;
import reactor.core.publisher.Mono;
import reactor.core.publisher.ReplayProcessor;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.function.Supplier;
/**
* Synchronizes reactor threads accessing/instantiating a common value {@code T}.
*
* @param The value being instantiated / accessed.
*/
public class SynchronizedAccessor {
private final AtomicBoolean wip;
private volatile T cache;
private final ReplayProcessor replayProcessor = ReplayProcessor.create(1);
private final FluxSink sink = replayProcessor.sink(FluxSink.OverflowStrategy.BUFFER);
private final Supplier supplier;
public SynchronizedAccessor(Supplier supplier) {
this.wip = new AtomicBoolean(false);
this.supplier = supplier;
}
/**
* Get the value from the configured supplier.
*
* @return the output {@code T}
*/
public Mono getValue() {
return Mono.defer(() -> {
if (cache != null) {
return Mono.just(cache);
}
if (!wip.getAndSet(true)) {
try {
cache = supplier.get();
sink.next(cache);
} catch (Exception e) {
sink.error(e);
}
}
return replayProcessor.next();
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy