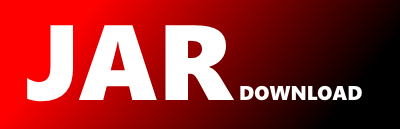
com.azure.identity.ChainedTokenCredentialBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-identity Show documentation
Show all versions of azure-identity Show documentation
This module contains client library for Microsoft Azure Identity.
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.identity;
import com.azure.core.credential.TokenCredential;
import java.util.ArrayDeque;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Deque;
/**
* Fluent credential builder for instantiating a {@link ChainedTokenCredential}.
*
* The {@link ChainedTokenCredential} is a convenience credential that allows users to chain together a set of
* TokenCredential together. The credential executes each credential in the chain sequentially and returns the token
* from the first credential in the chain that successfully authenticates.
*
* Sample: Construct a ChainedTokenCredential.
*
* The following code sample demonstrates the creation of a {@link com.azure.identity.ChainedTokenCredential},
* using the {@link com.azure.identity.ChainedTokenCredentialBuilder} to configure it. The sample below
* tries silent username+password login tried first, then interactive browser login as needed
* (e.g. when 2FA is turned on in the directory). Once this credential is created, it may be passed into the builder
* of many of the Azure SDK for Java client builders as the 'credential' parameter.
*
*
*
* TokenCredential usernamePasswordCredential = new UsernamePasswordCredentialBuilder()
* .clientId(clientId)
* .username(fakeUsernamePlaceholder)
* .password(fakePasswordPlaceholder)
* .build();
* TokenCredential interactiveBrowserCredential = new InteractiveBrowserCredentialBuilder()
* .clientId(clientId)
* .port(8765)
* .build();
* TokenCredential credential = new ChainedTokenCredentialBuilder()
* .addLast(usernamePasswordCredential)
* .addLast(interactiveBrowserCredential)
* .build();
*
*
*
* @see ChainedTokenCredential
*/
public class ChainedTokenCredentialBuilder {
private final Deque credentials;
/**
* Creates an instance of the builder to config the credential.
*/
public ChainedTokenCredentialBuilder() {
this.credentials = new ArrayDeque<>();
}
/**
* Adds a credential to try to authenticate at the front of the chain.
*
* @param credential the credential to be added to the front of chain
* @return the ChainedTokenCredential itself
*/
public ChainedTokenCredentialBuilder addFirst(TokenCredential credential) {
credentials.addFirst(credential);
return this;
}
/**
* Adds a credential to try to authenticate at the last of the chain.
* @param credential the credential to be added to the end of chain
* @return the ChainedTokenCredential itself
*/
public ChainedTokenCredentialBuilder addLast(TokenCredential credential) {
credentials.addLast(credential);
return this;
}
/**
* Adds all of the credentials in the specified collection at the end
* of this chain, as if by calling {@link ChainedTokenCredentialBuilder#addLast(TokenCredential)} on each one,
* in the order that they are returned by the collection's iterator.
*
* @param credentials the collection of credentials to be appended to the chain.
* @return An updated instance of the builder.
*/
public ChainedTokenCredentialBuilder addAll(Collection extends TokenCredential> credentials) {
this.credentials.addAll(credentials);
return this;
}
/**
* Creates a new {@link ChainedTokenCredential} with the current configurations.
*
* @return a {@link ChainedTokenCredential} with the current configurations.
*/
public ChainedTokenCredential build() {
return new ChainedTokenCredential(new ArrayList(credentials));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy