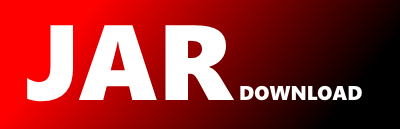
com.azure.identity.AzurePipelinesCredentialBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-identity Show documentation
Show all versions of azure-identity Show documentation
This module contains client library for Microsoft Azure Identity.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.identity;
import com.azure.core.http.policy.HttpLogOptions;
import com.azure.core.util.Configuration;
import com.azure.core.util.logging.ClientLogger;
import com.azure.identity.implementation.util.ValidationUtil;
import java.util.Arrays;
/**
* The {@link AzurePipelinesCredentialBuilder} provides a fluent builder for {@link AzurePipelinesCredential}.
*
*
*
* // serviceConnectionId is retrieved from the portal.
* // systemAccessToken is retrieved from the pipeline environment as shown.
* // You may choose another name for this variable.
*
* String systemAccessToken = System.getenv("SYSTEM_ACCESSTOKEN");
* AzurePipelinesCredential credential = new AzurePipelinesCredentialBuilder().clientId(clientId)
* .tenantId(tenantId)
* .serviceConnectionId(serviceConnectionId)
* .systemAccessToken(systemAccessToken)
* .build();
*
*
*/
public class AzurePipelinesCredentialBuilder extends AadCredentialBuilderBase {
private static final ClientLogger LOGGER = new ClientLogger(AzurePipelinesCredentialBuilder.class);
private static final String OIDC_API_VERSION = "7.1";
private String serviceConnectionId;
private String systemAccessToken;
/**
* Creates an instance of the {@link AzurePipelinesCredentialBuilder}.
*/
public AzurePipelinesCredentialBuilder() {
super();
}
/**
* Sets the service connection id for the Azure Pipelines service connection. The service connection ID is
* retrieved from the Service Connection in the portal.
*
* @param serviceConnectionId The service connection ID, as found in the query string's resourceId key.
* @return the updated instance of the builder.
*/
public AzurePipelinesCredentialBuilder serviceConnectionId(String serviceConnectionId) {
this.serviceConnectionId = serviceConnectionId;
return this;
}
/**
* Sets the System Access Token for the Azure Pipelines service connection. The system access token is
* retrieved from the pipeline variables by assigning it to an environment variable and reading it.
* See {@link AzurePipelinesCredential} for more information.
*
* @param systemAccessToken the system access token for the Azure Pipelines service connection.
* @return The updated instance of the builder.
*/
public AzurePipelinesCredentialBuilder systemAccessToken(String systemAccessToken) {
this.systemAccessToken = systemAccessToken;
return this;
}
/**
* Configures the persistent shared token cache options and enables the persistent token cache which is disabled
* by default. If configured, the credential will store tokens in a cache persisted to the machine, protected to
* the current user, which can be shared by other credentials and processes.
*
* @param tokenCachePersistenceOptions the token cache configuration options
* @return An updated instance of this builder with the token cache options configured.
*/
public AzurePipelinesCredentialBuilder
tokenCachePersistenceOptions(TokenCachePersistenceOptions tokenCachePersistenceOptions) {
this.identityClientOptions.setTokenCacheOptions(tokenCachePersistenceOptions);
return this;
}
/**
* Builds an instance of the {@link AzurePipelinesCredential} with the current configurations. Requires setting
* the following parameters:
*
* - Client ID via {@link #clientId(String)}
* - Tenant ID via {@link #tenantId(String)}
* - Service Connection ID via {@link #serviceConnectionId(String)}
* - System Access Token via {@link #systemAccessToken(String)}
*
* Requires the {@code SYSTEM_OIDCREQUESTURI} environment variable to be set.
*
* @throws IllegalArgumentException Thrown when required parameters are set or the environment is not correctly
* configured.
* @return an instance of the {@link AzurePipelinesCredential}.
*/
public AzurePipelinesCredential build() {
Configuration configuration = identityClientOptions.getConfiguration();
if (configuration == null) {
configuration = Configuration.getGlobalConfiguration();
}
String oidcEndpoint = configuration.get("SYSTEM_OIDCREQUESTURI");
ValidationUtil.validate(getClass().getSimpleName(), LOGGER,
Arrays.asList("clientId", "tenantId", "serviceConnectionId", "systemAccessToken", "oidcEndpoint"),
Arrays.asList(clientId, tenantId, serviceConnectionId, this.systemAccessToken, oidcEndpoint));
String requestUrl = String.format("%s?api-version=%s&serviceConnectionId=%s", oidcEndpoint, OIDC_API_VERSION,
serviceConnectionId);
// We need to make a best effort to log these headers.
// If the user gives us options, we'll sneak the headers in, or make an options with them.
// If the user gave us a pipeline none of this matters as we can't change a user created pipeline.
if (identityClientOptions.getHttpPipeline() != null) {
HttpLogOptions options = identityClientOptions.getHttpLogOptions();
if (options == null) {
options = new HttpLogOptions();
}
options.addAllowedHeaderName("x-vss-e2eid");
options.addAllowedHeaderName("x-msedge-ref");
identityClientOptions.setHttpLogOptions(options);
}
return new AzurePipelinesCredential(clientId, tenantId, requestUrl, systemAccessToken,
identityClientOptions.clone());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy