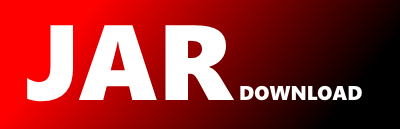
com.azure.identity.AzurePowerShellCredential Maven / Gradle / Ivy
Show all versions of azure-identity Show documentation
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.identity;
import com.azure.core.annotation.Immutable;
import com.azure.core.credential.AccessToken;
import com.azure.core.credential.TokenCredential;
import com.azure.core.credential.TokenRequestContext;
import com.azure.core.util.logging.ClientLogger;
import com.azure.identity.implementation.IdentityClient;
import com.azure.identity.implementation.IdentityClientBuilder;
import com.azure.identity.implementation.IdentityClientOptions;
import com.azure.identity.implementation.util.LoggingUtil;
import reactor.core.publisher.Mono;
/**
* The Azure Powershell is a command-line tool that allows users to manage Azure resources from their local machine
* or terminal. It allows users to
* authenticate interactively
* as a user and/or a service principal against
* Microsoft Entra ID.
* The AzurePowershellCredential authenticates in a development environment and acquires a token on behalf of the
* logged-in user or service principal in Azure Powershell. It acts as the Azure Powershell logged in user or
* service principal and executes an Azure Powershell command underneath to authenticate the application against
* Microsoft Entra ID.
*
* Configure AzurePowershellCredential
*
* To use this credential, the developer needs to authenticate locally in Azure Powershell using one of the
* commands below:
*
*
* - Run "Connect-AzAccount" in Azure Powershell to authenticate as a user.
* - Run "Connect-AzAccount -ServicePrincipal -ApplicationId {servicePrincipalId} -Tenant {tenantId}
* -CertificateThumbprint {thumbprint} to authenticate as a service principal."
*
*
* You may need to repeat this process after a certain time period, depending on the refresh token validity in your
* organization. Generally, the refresh token validity period is a few weeks to a few months. AzurePowershellCredential
* will prompt you to sign in again.
*
* Sample: Construct AzurePowershellCredential
*
* The following code sample demonstrates the creation of a {@link com.azure.identity.AzurePowerShellCredential},
* using the {@link com.azure.identity.AzurePowerShellCredentialBuilder} to configure it. Once this credential is
* created, it may be passed into the builder of many of the Azure SDK for Java client builders as the 'credential'
* parameter.
*
*
*
* TokenCredential powerShellCredential = new AzurePowerShellCredentialBuilder().build();
*
*
*
* @see com.azure.identity
* @see AzurePowerShellCredentialBuilder
*/
@Immutable
public class AzurePowerShellCredential implements TokenCredential {
private static final ClientLogger LOGGER = new ClientLogger(AzurePowerShellCredential.class);
private final IdentityClient identityClient;
AzurePowerShellCredential(String tenantId, IdentityClientOptions options) {
identityClient = new IdentityClientBuilder().identityClientOptions(options).tenantId(tenantId).build();
}
@Override
public Mono getToken(TokenRequestContext request) {
return identityClient.authenticateWithAzurePowerShell(request)
.doOnNext(token -> LoggingUtil.logTokenSuccess(LOGGER, request))
.doOnError(
error -> LoggingUtil.logTokenError(LOGGER, identityClient.getIdentityClientOptions(), request, error))
.onErrorMap(error -> {
if (identityClient.getIdentityClientOptions().isChained()) {
return new CredentialUnavailableException(error.getMessage(), error);
} else {
return error;
}
});
}
}