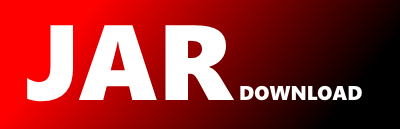
com.azure.identity.ClientSecretCredentialBuilder Maven / Gradle / Ivy
Show all versions of azure-identity Show documentation
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.identity;
import com.azure.core.util.logging.ClientLogger;
import com.azure.identity.implementation.util.ValidationUtil;
/**
* Fluent credential builder for instantiating a {@link ClientSecretCredential}.
*
* The {@link ClientSecretCredential} acquires a token via service principal authentication. It is a type of
* authentication in Azure that enables a non-interactive login to
* Microsoft Entra ID, allowing an
* application or service to authenticate itself with Azure resources.
* A Service Principal is essentially an identity created for an application in Microsoft Entra ID that can be used to
* authenticate with Azure resources. It's like a "user identity" for the application or service, and it provides
* a way for the application to authenticate itself with Azure resources without needing to use a user's credentials.
* Microsoft Entra ID allows users to
* register service principals which can be used as an identity for authentication.
* A client secret associated with the registered service principal is used as the password when authenticating the
* service principal.
* The {@link ClientSecretCredential} acquires an access token with a client secret for a service principal/registered
* Microsoft Entra application. The tenantId, clientId and clientSecret of the service principal are required for this credential
* to acquire an access token. It can be used both in Azure hosted and local development environments for
* authentication. For more information refer to the
* conceptual knowledge and configuration
* details.
*
* Sample: Construct a simple ClientSecretCredential
*
* The following code sample demonstrates the creation of a {@link com.azure.identity.ClientSecretCredential},
* using the {@link com.azure.identity.ClientSecretCredentialBuilder} to configure it. The {@code tenantId},
* {@code clientId} and {@code clientSecret} parameters are required to create
* {@link com.azure.identity.ClientSecretCredential} .Once this credential is created, it may be passed into the
* builder of many of the Azure SDK for Java client builders as the 'credential' parameter.
*
*
*
* TokenCredential clientSecretCredential = new ClientSecretCredentialBuilder().tenantId(tenantId)
* .clientId(clientId)
* .clientSecret(clientSecret)
* .build();
*
*
*
* Sample: Construct a ClientSecretCredential behind a proxy
*
* The following code sample demonstrates the creation of a {@link com.azure.identity.ClientSecretCredential},
* using the {@link com.azure.identity.ClientSecretCredentialBuilder} to configure it. The {@code tenantId},
* {@code clientId} and {@code clientSecret} parameters are required to create
* {@link com.azure.identity.ClientSecretCredential}. The {@code proxyOptions} can be optionally configured to target
* a proxy. Once this credential is created, it may be passed into the builder of many of the Azure SDK for Java
* client builders as the 'credential' parameter.
*
*
*
* TokenCredential secretCredential = new ClientSecretCredentialBuilder().tenantId(tenantId)
* .clientId(clientId)
* .clientSecret(clientSecret)
* .proxyOptions(new ProxyOptions(Type.HTTP, new InetSocketAddress("10.21.32.43", 5465)))
* .build();
*
*
*
* @see ClientSecretCredential
*/
public class ClientSecretCredentialBuilder extends AadCredentialBuilderBase {
private static final ClientLogger LOGGER = new ClientLogger(ClientSecretCredentialBuilder.class);
private static final String CLASS_NAME = ClientSecretCredentialBuilder.class.getSimpleName();
private String clientSecret;
/**
* Constructs an instance of ClientSecretCredentialBuilder.
*/
public ClientSecretCredentialBuilder() {
super();
}
/**
* Sets the client secret for the authentication.
* @param clientSecret the secret value of the Microsoft Entra application.
* @return An updated instance of this builder.
*/
public ClientSecretCredentialBuilder clientSecret(String clientSecret) {
this.clientSecret = clientSecret;
return this;
}
/**
* Enables the shared token cache which is disabled by default. If enabled, the credential will store tokens
* in a cache persisted to the machine, protected to the current user, which can be shared by other credentials
* and processes.
*
* @return An updated instance of this builder.
*/
ClientSecretCredentialBuilder enablePersistentCache() {
this.identityClientOptions.enablePersistentCache();
return this;
}
/**
* Allows to use an unprotected file specified by cacheFileLocation()
instead of
* Gnome keyring on Linux. This is restricted by default.
*
* @return An updated instance of this builder.
*/
ClientSecretCredentialBuilder allowUnencryptedCache() {
this.identityClientOptions.setAllowUnencryptedCache(true);
return this;
}
/**
* Configures the persistent shared token cache options and enables the persistent token cache which is disabled
* by default. If configured, the credential will store tokens in a cache persisted to the machine, protected to
* the current user, which can be shared by other credentials and processes.
*
* @param tokenCachePersistenceOptions the token cache configuration options
* @return An updated instance of this builder with the token cache options configured.
*/
public ClientSecretCredentialBuilder
tokenCachePersistenceOptions(TokenCachePersistenceOptions tokenCachePersistenceOptions) {
this.identityClientOptions.setTokenCacheOptions(tokenCachePersistenceOptions);
return this;
}
/**
* Creates a new {@link ClientCertificateCredential} with the current configurations.
*
* @return a {@link ClientSecretCredentialBuilder} with the current configurations.
*/
public ClientSecretCredential build() {
ValidationUtil.validate(CLASS_NAME, LOGGER, "clientId", clientId, "tenantId", tenantId, "clientSecret",
clientSecret);
return new ClientSecretCredential(tenantId, clientId, clientSecret, identityClientOptions);
}
}