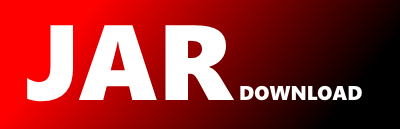
com.azure.identity.implementation.MsalToken Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-identity Show documentation
Show all versions of azure-identity Show documentation
This module contains client library for Microsoft Azure Identity.
The newest version!
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
package com.azure.identity.implementation;
import com.azure.core.credential.AccessToken;
import com.microsoft.aad.msal4j.IAccount;
import com.microsoft.aad.msal4j.IAuthenticationResult;
import java.time.Instant;
import java.time.OffsetDateTime;
import java.time.ZoneOffset;
/**
* Type representing authentication result from the MSAL (Microsoft Authentication Library).
*/
public final class MsalToken extends AccessToken {
private IAuthenticationResult authenticationResult;
/**
* Creates an access token instance.
*
* @param msalResult the raw authentication result returned by MSAL
*/
public MsalToken(IAuthenticationResult msalResult) {
super(msalResult.accessToken(),
OffsetDateTime.ofInstant(msalResult.expiresOnDate().toInstant(), ZoneOffset.UTC),
msalResult.metadata() != null
? msalResult.metadata().refreshOn() == null
? null
: OffsetDateTime.ofInstant(Instant.ofEpochSecond(msalResult.metadata().refreshOn()), ZoneOffset.UTC)
: null);
authenticationResult = msalResult;
}
public MsalToken(IAuthenticationResult msalResult, String tokenType) {
super(msalResult.accessToken(),
OffsetDateTime.ofInstant(msalResult.expiresOnDate().toInstant(), ZoneOffset.UTC),
msalResult.metadata() != null
? msalResult.metadata().refreshOn() == null
? null
: OffsetDateTime.ofInstant(Instant.ofEpochSecond(msalResult.metadata().refreshOn()), ZoneOffset.UTC)
: null,
tokenType);
authenticationResult = msalResult;
}
/**
* @return the signed in account
*/
public IAccount getAccount() {
return authenticationResult.account();
}
/**
* Get the MSAL Authentication result.
*
* @return the authentication result.
*/
public IAuthenticationResult getAuthenticationResult() {
return authenticationResult;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy