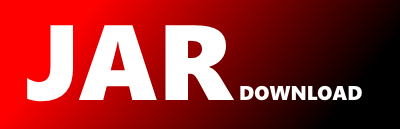
com.azure.maps.traffic.TrafficAsyncClient Maven / Gradle / Ivy
Show all versions of azure-maps-traffic Show documentation
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
// Code generated by Microsoft (R) AutoRest Code Generator.
package com.azure.maps.traffic;
import com.azure.core.annotation.ReturnType;
import com.azure.core.annotation.ServiceClient;
import com.azure.core.annotation.ServiceMethod;
import com.azure.core.exception.HttpResponseException;
import com.azure.core.http.rest.Response;
import com.azure.core.http.rest.StreamResponse;
import com.azure.core.util.BinaryData;
import com.azure.core.util.Context;
import com.azure.core.util.FluxUtil;
import com.azure.core.util.logging.ClientLogger;
import com.azure.core.http.rest.SimpleResponse;
import com.azure.maps.traffic.implementation.TrafficsImpl;
import com.azure.maps.traffic.implementation.helpers.Utility;
import com.azure.maps.traffic.models.TrafficFlowSegmentData;
import com.azure.maps.traffic.implementation.models.ResponseFormat;
import com.azure.maps.traffic.implementation.models.ErrorResponseException;
import com.azure.maps.traffic.models.TrafficIncidentDetail;
import com.azure.maps.traffic.models.TrafficIncidentViewport;
import com.azure.maps.traffic.models.TrafficFlowSegmentOptions;
import com.azure.maps.traffic.models.TrafficFlowTileOptions;
import com.azure.maps.traffic.models.TrafficIncidentDetailOptions;
import com.azure.maps.traffic.models.TrafficIncidentTileOptions;
import com.azure.maps.traffic.models.TrafficIncidentViewportOptions;
import reactor.core.publisher.Mono;
/** Initializes a new instance of the asynchronous TrafficClient type.
* Creating an async client using a {@link com.azure.core.credential.AzureKeyCredential}:
*
*
* // Authenticates using subscription key
* AzureKeyCredential keyCredential = new AzureKeyCredential(System.getenv("SUBSCRIPTION_KEY"));
*
* // Creates a builder
* TrafficClientBuilder builder = new TrafficClientBuilder();
* builder.credential(keyCredential);
* builder.httpLogOptions(new HttpLogOptions().setLogLevel(HttpLogDetailLevel.BODY_AND_HEADERS));
*
* // Builds the client
* TrafficAsyncClient client = builder.buildAsyncClient();
*
*
*
*
* // Authenticates using Azure AD building a default credential
* // This will look for AZURE_CLIENT_ID, AZURE_TENANT_ID, and AZURE_CLIENT_SECRET env variables
* DefaultAzureCredential tokenCredential = new DefaultAzureCredentialBuilder().build();
*
* // Creates a builder
* TrafficClientBuilder builder = new TrafficClientBuilder();
* builder.credential(tokenCredential);
* builder.trafficClientId(System.getenv("MAPS_CLIENT_ID"));
* builder.httpLogOptions(new HttpLogOptions().setLogLevel(HttpLogDetailLevel.BODY_AND_HEADERS));
*
* // Builds a client
* TrafficAsyncClient client = builder.buildAsyncClient();
*
*
*/
@ServiceClient(builder = TrafficClientBuilder.class, isAsync = true)
public final class TrafficAsyncClient {
private final TrafficsImpl serviceClient;
private static final ClientLogger LOGGER = new ClientLogger(TrafficAsyncClient.class);
/**
* Initializes an instance of Traffics client.
*
* @param serviceClient the service client implementation.
*/
TrafficAsyncClient(TrafficsImpl serviceClient) {
this.serviceClient = serviceClient;
}
/**
* __Traffic Flow Tile__
*
*
*
* System.out.println("Get Traffic Flow Tile:");
*
* // options
* asyncClient.getTrafficFlowTile(
* new TrafficFlowTileOptions()
* .setTrafficFlowTileStyle(TrafficFlowTileStyle.RELATIVE_DELAY).setFormat(TileFormat.PNG).setZoom(10));
*
* // complete
* asyncClient.getTrafficFlowTile(
* new TrafficFlowTileOptions()
* .setTrafficFlowTileStyle(TrafficFlowTileStyle.RELATIVE_DELAY).setFormat(TileFormat.PNG).setZoom(10)
* .setTileIndex(new TileIndex().setX(2044).setY(1360)).setThickness(10));
*
*
*
* **Applies to**: S0 and S1 pricing tiers.
*
*
The Azure Flow Tile API serves 256 x 256 pixel tiles showing traffic flow. All tiles use the same grid system.
* Because the traffic tiles use transparent images, they can be layered on top of map tiles to create a compound
* display. The Flow tiles use colors to indicate either the speed of traffic on different road segments, or the
* difference between that speed and the free-flow speed on the road segment in question.
*
* @param options {@link TrafficFlowTileOptions} the options to be used in this search.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ErrorResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getTrafficFlowTile(TrafficFlowTileOptions options) {
Mono responseMono = this.getTrafficFlowTileWithResponse(options, null);
return BinaryData.fromFlux(responseMono.flatMapMany(response -> {
return response.getValue();
}));
}
/**
* __Traffic Flow Tile__
*
*
*
* System.out.println("Get Traffic Flow Tile:");
*
* // options
* asyncClient.getTrafficFlowTile(
* new TrafficFlowTileOptions()
* .setTrafficFlowTileStyle(TrafficFlowTileStyle.RELATIVE_DELAY).setFormat(TileFormat.PNG).setZoom(10));
*
* // complete
* asyncClient.getTrafficFlowTile(
* new TrafficFlowTileOptions()
* .setTrafficFlowTileStyle(TrafficFlowTileStyle.RELATIVE_DELAY).setFormat(TileFormat.PNG).setZoom(10)
* .setTileIndex(new TileIndex().setX(2044).setY(1360)).setThickness(10));
*
*
*
* **Applies to**: S0 and S1 pricing tiers.
*
*
The Azure Flow Tile API serves 256 x 256 pixel tiles showing traffic flow. All tiles use the same grid system.
* Because the traffic tiles use transparent images, they can be layered on top of map tiles to create a compound
* display. The Flow tiles use colors to indicate either the speed of traffic on different road segments, or the
* difference between that speed and the free-flow speed on the road segment in question.
*
* @param options {@link TrafficFlowTileOptions} the options to be used in this search.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ErrorResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getTrafficFlowTileWithResponse(TrafficFlowTileOptions options) {
if (options == null) {
throw LOGGER.logExceptionAsError(new NullPointerException("Options is null"));
}
StreamResponse response = null;
try {
response = this.getTrafficFlowTileWithResponse(options, null).block();
} catch (RuntimeException ex) {
return FluxUtil.monoError(LOGGER, ex);
}
if (response != null) {
return Mono.just(new SimpleResponse(response.getRequest(), response.getStatusCode(), response.getHeaders(), BinaryData.fromFlux(response.getValue()).block()));
} else {
return Mono.error(new NullPointerException("Response is null"));
}
}
/**
* __Traffic Flow Tile__
*
*
*
* System.out.println("Get Traffic Flow Tile:");
*
* // options
* asyncClient.getTrafficFlowTile(
* new TrafficFlowTileOptions()
* .setTrafficFlowTileStyle(TrafficFlowTileStyle.RELATIVE_DELAY).setFormat(TileFormat.PNG).setZoom(10));
*
* // complete
* asyncClient.getTrafficFlowTile(
* new TrafficFlowTileOptions()
* .setTrafficFlowTileStyle(TrafficFlowTileStyle.RELATIVE_DELAY).setFormat(TileFormat.PNG).setZoom(10)
* .setTileIndex(new TileIndex().setX(2044).setY(1360)).setThickness(10));
*
*
*
* **Applies to**: S0 and S1 pricing tiers.
*
*
The Azure Flow Tile API serves 256 x 256 pixel tiles showing traffic flow. All tiles use the same grid system.
* Because the traffic tiles use transparent images, they can be layered on top of map tiles to create a compound
* display. The Flow tiles use colors to indicate either the speed of traffic on different road segments, or the
* difference between that speed and the free-flow speed on the road segment in question.
*
* @param context The context to associate with this operation.
* @param options {@link TrafficFlowTileOptions} the options to be used in this search.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ErrorResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response.
*/
Mono getTrafficFlowTileWithResponse(TrafficFlowTileOptions options, Context context) {
if (options == null) {
throw LOGGER.logExceptionAsError(new NullPointerException("Options is null"));
}
return this.serviceClient.getTrafficFlowTileWithResponseAsync(
options.getFormat(),
options.getTrafficFlowTileStyle(),
options.getZoom(),
options.getTileIndex(),
options.getThickness(),
context).onErrorMap(throwable -> {
if (!(throwable instanceof ErrorResponseException)) {
return throwable;
}
ErrorResponseException exception = (ErrorResponseException) throwable;
return new HttpResponseException(exception.getMessage(), exception.getResponse());
});
}
/**
* __Traffic Flow Segment__
*
*
*
* System.out.println("Get Traffic Flow Segment:");
*
* // options
* asyncClient.getTrafficFlowSegment(
* new TrafficFlowSegmentOptions()
* .setTrafficFlowSegmentStyle(TrafficFlowSegmentStyle.ABSOLUTE).setZoom(10)
* .setCoordinates(new GeoPosition(4.84239, 52.41072)));
*
* // complete
* asyncClient.getTrafficFlowSegment(
* new TrafficFlowSegmentOptions()
* .setTrafficFlowSegmentStyle(TrafficFlowSegmentStyle.ABSOLUTE).setZoom(10)
* .setCoordinates(new GeoPosition(4.84239, 52.41072)).setOpenLr(false)
* .setThickness(2).setUnit(SpeedUnit.MPH));
*
*
*
* **Applies to**: S0 and S1 pricing tiers.
*
*
This service provides information about the speeds and travel times of the road fragment closest to the given
* coordinates. It is designed to work alongside the Flow layer of the Render Service to support clickable flow data
* visualizations. With this API, the client side can connect any place in the map with flow data on the closest
* road and present it to the user.
*
* @param options {@link TrafficFlowSegmentOptions} the options to be used in this search.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws HttpResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return this object is returned from a successful Traffic Flow Segment call.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getTrafficFlowSegment(TrafficFlowSegmentOptions options) {
Mono> result = this.getTrafficFlowSegmentWithResponse(options, null);
return result.flatMap(response -> {
return Mono.just(response.getValue());
});
}
/**
* __Traffic Flow Segment__
*
*
*
* System.out.println("Get Traffic Flow Segment:");
*
* // options
* asyncClient.getTrafficFlowSegment(
* new TrafficFlowSegmentOptions()
* .setTrafficFlowSegmentStyle(TrafficFlowSegmentStyle.ABSOLUTE).setZoom(10)
* .setCoordinates(new GeoPosition(4.84239, 52.41072)));
*
* // complete
* asyncClient.getTrafficFlowSegment(
* new TrafficFlowSegmentOptions()
* .setTrafficFlowSegmentStyle(TrafficFlowSegmentStyle.ABSOLUTE).setZoom(10)
* .setCoordinates(new GeoPosition(4.84239, 52.41072)).setOpenLr(false)
* .setThickness(2).setUnit(SpeedUnit.MPH));
*
*
*
* **Applies to**: S0 and S1 pricing tiers.
*
*
This service provides information about the speeds and travel times of the road fragment closest to the given
* coordinates. It is designed to work alongside the Flow layer of the Render Service to support clickable flow data
* visualizations. With this API, the client side can connect any place in the map with flow data on the closest
* road and present it to the user.
*
* @param options {@link TrafficFlowSegmentOptions} the options to be used in this search.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ErrorResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return this object is returned from a successful Traffic Flow Segment call.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getTrafficFlowSegmentWithResponse(TrafficFlowSegmentOptions options) {
return this.getTrafficFlowSegmentWithResponse(options, null);
}
/**
* __Traffic Flow Segment__
*
*
*
* System.out.println("Get Traffic Flow Segment:");
*
* // options
* asyncClient.getTrafficFlowSegment(
* new TrafficFlowSegmentOptions()
* .setTrafficFlowSegmentStyle(TrafficFlowSegmentStyle.ABSOLUTE).setZoom(10)
* .setCoordinates(new GeoPosition(4.84239, 52.41072)));
*
* // complete
* asyncClient.getTrafficFlowSegment(
* new TrafficFlowSegmentOptions()
* .setTrafficFlowSegmentStyle(TrafficFlowSegmentStyle.ABSOLUTE).setZoom(10)
* .setCoordinates(new GeoPosition(4.84239, 52.41072)).setOpenLr(false)
* .setThickness(2).setUnit(SpeedUnit.MPH));
*
*
*
* **Applies to**: S0 and S1 pricing tiers.
*
*
This service provides information about the speeds and travel times of the road fragment closest to the given
* coordinates. It is designed to work alongside the Flow layer of the Render Service to support clickable flow data
* visualizations. With this API, the client side can connect any place in the map with flow data on the closest
* road and present it to the user.
*
* @param options {@link TrafficFlowSegmentOptions} the options to be used in this search.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ErrorResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return this object is returned from a successful Traffic Flow Segment call.
*/
Mono> getTrafficFlowSegmentWithResponse(TrafficFlowSegmentOptions options, Context context) {
return this.serviceClient.getTrafficFlowSegmentWithResponseAsync(
ResponseFormat.JSON,
options.getTrafficFlowSegmentStyle(),
options.getZoom(),
Utility.toCoordinates(options.getCoordinates()),
options.getUnit(),
options.getThickness(),
options.getOpenLr(),
context).onErrorMap(throwable -> {
if (!(throwable instanceof ErrorResponseException)) {
return throwable;
}
ErrorResponseException exception = (ErrorResponseException) throwable;
return new HttpResponseException(exception.getMessage(), exception.getResponse());
});
}
/**
* __Traffic Incident Tile__
*
*
*
* System.out.println("Get Traffic Incident Tile:");
*
* // options
* client.getTrafficIncidentTile(
* new TrafficIncidentTileOptions()
* .setFormat(TileFormat.PNG).setTrafficIncidentTileStyle(TrafficIncidentTileStyle.S3)
* .setZoom(10));
*
* // complete
* client.getTrafficIncidentTile(
* new TrafficIncidentTileOptions()
* .setFormat(TileFormat.PNG).setTrafficIncidentTileStyle(TrafficIncidentTileStyle.S3)
* .setZoom(10).setTileIndex(new TileIndex().setX(175).setY(408)));
*
*
*
* **Applies to**: S0 and S1 pricing tiers.
*
*
This service serves 256 x 256 pixel tiles showing traffic incidents. All tiles use the same grid system.
* Because the traffic tiles use transparent images, they can be layered on top of map tiles to create a compound
* display. Traffic tiles render graphics to indicate traffic on the roads in the specified area.
*
* @param options {@link TrafficIncidentTileOptions} the options to be used in this search.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ErrorResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getTrafficIncidentTile(TrafficIncidentTileOptions options) {
Mono responseMono = this.getTrafficIncidentTileWithResponse(options, null);
if (options == null) {
throw LOGGER.logExceptionAsError(new NullPointerException("Options is null"));
}
return BinaryData.fromFlux(responseMono.flatMapMany(response -> {
return response.getValue();
}));
}
/**
* __Traffic Incident Tile__
*
*
*
* System.out.println("Get Traffic Incident Tile:");
*
* // options
* client.getTrafficIncidentTile(
* new TrafficIncidentTileOptions()
* .setFormat(TileFormat.PNG).setTrafficIncidentTileStyle(TrafficIncidentTileStyle.S3)
* .setZoom(10));
*
* // complete
* client.getTrafficIncidentTile(
* new TrafficIncidentTileOptions()
* .setFormat(TileFormat.PNG).setTrafficIncidentTileStyle(TrafficIncidentTileStyle.S3)
* .setZoom(10).setTileIndex(new TileIndex().setX(175).setY(408)));
*
*
*
* **Applies to**: S0 and S1 pricing tiers.
*
*
This service serves 256 x 256 pixel tiles showing traffic incidents. All tiles use the same grid system.
* Because the traffic tiles use transparent images, they can be layered on top of map tiles to create a compound
* display. Traffic tiles render graphics to indicate traffic on the roads in the specified area.
*
* @param options {@link TrafficIncidentTileOptions} the options to be used in this search.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ErrorResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getTrafficIncidentTileWithResponse(TrafficIncidentTileOptions options) {
if (options == null) {
throw LOGGER.logExceptionAsError(new NullPointerException("Options is null"));
}
StreamResponse response = this.getTrafficIncidentTileWithResponse(options, null).block();
if (response != null) {
return Mono.just(new SimpleResponse(response.getRequest(), response.getStatusCode(), response.getHeaders(), null));
} else {
return Mono.error(new NullPointerException("Response is null"));
}
}
/**
* __Traffic Incident Tile__
*
*
*
* System.out.println("Get Traffic Incident Tile:");
*
* // options
* client.getTrafficIncidentTile(
* new TrafficIncidentTileOptions()
* .setFormat(TileFormat.PNG).setTrafficIncidentTileStyle(TrafficIncidentTileStyle.S3)
* .setZoom(10));
*
* // complete
* client.getTrafficIncidentTile(
* new TrafficIncidentTileOptions()
* .setFormat(TileFormat.PNG).setTrafficIncidentTileStyle(TrafficIncidentTileStyle.S3)
* .setZoom(10).setTileIndex(new TileIndex().setX(175).setY(408)));
*
*
*
* **Applies to**: S0 and S1 pricing tiers.
*
*
This service serves 256 x 256 pixel tiles showing traffic incidents. All tiles use the same grid system.
* Because the traffic tiles use transparent images, they can be layered on top of map tiles to create a compound
* display. Traffic tiles render graphics to indicate traffic on the roads in the specified area.
*
* @param context The context to associate with this operation.
* @param options {@link TrafficIncidentTileOptions} the options to be used in this search.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ErrorResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return the response.
*/
Mono getTrafficIncidentTileWithResponse(TrafficIncidentTileOptions options, Context context) {
if (options == null) {
throw LOGGER.logExceptionAsError(new NullPointerException("Options is null"));
}
return this.serviceClient.getTrafficIncidentTileWithResponseAsync(
options.getFormat(),
options.getTrafficIncidentTileStyle(),
options.getZoom(),
options.getTileIndex(),
options.getTrafficState(),
context).onErrorMap(throwable -> {
if (!(throwable instanceof ErrorResponseException)) {
return throwable;
}
ErrorResponseException exception = (ErrorResponseException) throwable;
return new HttpResponseException(exception.getMessage(), exception.getResponse());
});
}
/**
* __Traffic Incident Detail__
*
*
*
* System.out.println("Get Traffic Incident Detail:");
*
* // options
* client.getTrafficIncidentDetail(
* new TrafficIncidentDetailOptions()
* .setBoundingBox(new GeoBoundingBox(45, 45, 45, 45)).setBoundingZoom(11)
* .setIncidentDetailStyle(IncidentDetailStyle.S3).setBoundingZoom(11)
* .setTrafficmodelId("1335294634919"));
*
* // complete
* client.getTrafficIncidentDetail(
* new TrafficIncidentDetailOptions()
* .setBoundingBox(new GeoBoundingBox(45, 45, 45, 45)).setBoundingZoom(11)
* .setIncidentDetailStyle(IncidentDetailStyle.S3).setBoundingZoom(11)
* .setTrafficmodelId("1335294634919").setLanguage("en")
* .setProjectionStandard(ProjectionStandard.EPSG900913).setIncidentGeometryType(IncidentGeometryType.ORIGINAL)
* .setExpandCluster(false).setOriginalPosition(false));
*
*
*
* **Applies to**: S0 and S1 pricing tiers.
*
*
This API provides information on traffic incidents inside a given bounding box, based on the current Traffic
* Model ID. The Traffic Model ID is available to grant synchronization of data between calls and API's. The Traffic
* Model ID is a key value for determining the currency of traffic incidents. It is updated every minute, and is
* valid for two minutes before it times out. It is used in rendering [incident
* tiles](https://docs.microsoft.com/rest/api/maps/traffic/gettrafficincidenttile). It can be obtained from
* the [Viewport API](https://docs.microsoft.com/rest/api/maps/traffic/gettrafficincidentviewport).
*
* @param options {@link TrafficIncidentDetailOptions} the options to be used in this search.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ErrorResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return this object is returned from a successful Traffic incident Detail call.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getTrafficIncidentDetail(TrafficIncidentDetailOptions options) {
Mono> result = this.getTrafficIncidentDetailWithResponse(options, null);
return result.flatMap(response -> {
return Mono.just(response.getValue());
});
}
/**
* __Traffic Incident Detail__
*
*
*
* System.out.println("Get Traffic Incident Detail:");
*
* // options
* client.getTrafficIncidentDetail(
* new TrafficIncidentDetailOptions()
* .setBoundingBox(new GeoBoundingBox(45, 45, 45, 45)).setBoundingZoom(11)
* .setIncidentDetailStyle(IncidentDetailStyle.S3).setBoundingZoom(11)
* .setTrafficmodelId("1335294634919"));
*
* // complete
* client.getTrafficIncidentDetail(
* new TrafficIncidentDetailOptions()
* .setBoundingBox(new GeoBoundingBox(45, 45, 45, 45)).setBoundingZoom(11)
* .setIncidentDetailStyle(IncidentDetailStyle.S3).setBoundingZoom(11)
* .setTrafficmodelId("1335294634919").setLanguage("en")
* .setProjectionStandard(ProjectionStandard.EPSG900913).setIncidentGeometryType(IncidentGeometryType.ORIGINAL)
* .setExpandCluster(false).setOriginalPosition(false));
*
*
*
* **Applies to**: S0 and S1 pricing tiers.
*
*
This API provides information on traffic incidents inside a given bounding box, based on the current Traffic
* Model ID. The Traffic Model ID is available to grant synchronization of data between calls and API's. The Traffic
* Model ID is a key value for determining the currency of traffic incidents. It is updated every minute, and is
* valid for two minutes before it times out. It is used in rendering [incident
* tiles](https://docs.microsoft.com/rest/api/maps/traffic/gettrafficincidenttile). It can be obtained from
* the [Viewport API](https://docs.microsoft.com/rest/api/maps/traffic/gettrafficincidentviewport).
*
* @param options {@link TrafficIncidentDetailOptions} the options to be used in this search.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ErrorResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return this object is returned from a successful Traffic incident Detail call.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getTrafficIncidentDetailWithResponse(TrafficIncidentDetailOptions options) {
return this.getTrafficIncidentDetailWithResponse(options, null);
}
/**
* __Traffic Incident Detail__
*
*
*
* System.out.println("Get Traffic Incident Detail:");
*
* // options
* client.getTrafficIncidentDetail(
* new TrafficIncidentDetailOptions()
* .setBoundingBox(new GeoBoundingBox(45, 45, 45, 45)).setBoundingZoom(11)
* .setIncidentDetailStyle(IncidentDetailStyle.S3).setBoundingZoom(11)
* .setTrafficmodelId("1335294634919"));
*
* // complete
* client.getTrafficIncidentDetail(
* new TrafficIncidentDetailOptions()
* .setBoundingBox(new GeoBoundingBox(45, 45, 45, 45)).setBoundingZoom(11)
* .setIncidentDetailStyle(IncidentDetailStyle.S3).setBoundingZoom(11)
* .setTrafficmodelId("1335294634919").setLanguage("en")
* .setProjectionStandard(ProjectionStandard.EPSG900913).setIncidentGeometryType(IncidentGeometryType.ORIGINAL)
* .setExpandCluster(false).setOriginalPosition(false));
*
*
*
* **Applies to**: S0 and S1 pricing tiers.
*
*
This API provides information on traffic incidents inside a given bounding box, based on the current Traffic
* Model ID. The Traffic Model ID is available to grant synchronization of data between calls and API's. The Traffic
* Model ID is a key value for determining the currency of traffic incidents. It is updated every minute, and is
* valid for two minutes before it times out. It is used in rendering [incident
* tiles](https://docs.microsoft.com/rest/api/maps/traffic/gettrafficincidenttile). It can be obtained from
* the [Viewport API](https://docs.microsoft.com/rest/api/maps/traffic/gettrafficincidentviewport).
*
* @param context The context to associate with this operation.
* @param options {@link TrafficIncidentDetailOptions} the options to be used in this search.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ErrorResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return this object is returned from a successful Traffic incident Detail call.
*/
Mono> getTrafficIncidentDetailWithResponse(TrafficIncidentDetailOptions options, Context context) {
return this.serviceClient.getTrafficIncidentDetailWithResponseAsync(
ResponseFormat.JSON,
options.getIncidentDetailStyle(),
Utility.toBoundingBox(options.getBoundingBox()),
options.getBoundingZoom(),
options.getTrafficIncidentDetailTrafficModelId(),
options.getLanguage(),
options.getProjectionStandard(),
options.getIncidentGeometryType(),
options.getExpandCluster(),
options.getOriginalPosition(),
context).onErrorMap(throwable -> {
if (!(throwable instanceof ErrorResponseException)) {
return throwable;
}
ErrorResponseException exception = (ErrorResponseException) throwable;
return new HttpResponseException(exception.getMessage(), exception.getResponse());
});
}
/**
* __Traffic Incident Viewport__
*
*
*
* System.out.println("Get Traffic Incident Tile:");
*
* // options
* client.getTrafficIncidentViewport(
* new TrafficIncidentViewportOptions()
* .setBoundingBox(new GeoBoundingBox(45, 45, 45, 45))
* .setBoundingZoom(2).setOverview(new GeoBoundingBox(45, 45, 45, 45))
* .setOverviewZoom(2));
*
* // complete
* client.getTrafficIncidentViewport(
* new TrafficIncidentViewportOptions()
* .setBoundingBox(new GeoBoundingBox(45, 45, 45, 45))
* .setBoundingZoom(2).setOverview(new GeoBoundingBox(45, 45, 45, 45))
* .setOverviewZoom(2).setCopyright(true));
*
*
*
* **Applies to**: S0 and S1 pricing tiers.
*
*
This API returns legal and technical information for the viewport described in the request. It should be
* called by client applications whenever the viewport changes (for instance, through zooming, panning, going to a
* location, or displaying a route). The request should contain the bounding box and zoom level of the viewport
* whose information is needed. The return will contain map version information, as well as the current Traffic
* Model ID and copyright IDs. The Traffic Model ID returned by the Viewport Description is used by other APIs to
* retrieve last traffic information for further processing.
*
* @param options {@link TrafficIncidentViewportOptions} the options to be used in this search.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ErrorResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return this object is returned from a successful Traffic Incident Viewport call.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono getTrafficIncidentViewport(TrafficIncidentViewportOptions options) {
Mono> result = this.getTrafficIncidentViewportWithResponse(options, null);
return result.flatMap(response -> {
return Mono.just(response.getValue());
});
}
/**
* __Traffic Incident Viewport__
*
*
*
* System.out.println("Get Traffic Incident Tile:");
*
* // options
* client.getTrafficIncidentViewport(
* new TrafficIncidentViewportOptions()
* .setBoundingBox(new GeoBoundingBox(45, 45, 45, 45))
* .setBoundingZoom(2).setOverview(new GeoBoundingBox(45, 45, 45, 45))
* .setOverviewZoom(2));
*
* // complete
* client.getTrafficIncidentViewport(
* new TrafficIncidentViewportOptions()
* .setBoundingBox(new GeoBoundingBox(45, 45, 45, 45))
* .setBoundingZoom(2).setOverview(new GeoBoundingBox(45, 45, 45, 45))
* .setOverviewZoom(2).setCopyright(true));
*
*
*
* **Applies to**: S0 and S1 pricing tiers.
*
*
This API returns legal and technical information for the viewport described in the request. It should be
* called by client applications whenever the viewport changes (for instance, through zooming, panning, going to a
* location, or displaying a route). The request should contain the bounding box and zoom level of the viewport
* whose information is needed. The return will contain map version information, as well as the current Traffic
* Model ID and copyright IDs. The Traffic Model ID returned by the Viewport Description is used by other APIs to
* retrieve last traffic information for further processing.
*
* @param options {@link TrafficIncidentViewportOptions} the options to be used in this search.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ErrorResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return this object is returned from a successful Traffic Incident Viewport call.
*/
@ServiceMethod(returns = ReturnType.SINGLE)
public Mono> getTrafficIncidentViewportWithResponse(TrafficIncidentViewportOptions options) {
return this.getTrafficIncidentViewportWithResponse(options, null);
}
/**
* __Traffic Incident Viewport__
*
*
*
* System.out.println("Get Traffic Incident Tile:");
*
* // options
* client.getTrafficIncidentViewport(
* new TrafficIncidentViewportOptions()
* .setBoundingBox(new GeoBoundingBox(45, 45, 45, 45))
* .setBoundingZoom(2).setOverview(new GeoBoundingBox(45, 45, 45, 45))
* .setOverviewZoom(2));
*
* // complete
* client.getTrafficIncidentViewport(
* new TrafficIncidentViewportOptions()
* .setBoundingBox(new GeoBoundingBox(45, 45, 45, 45))
* .setBoundingZoom(2).setOverview(new GeoBoundingBox(45, 45, 45, 45))
* .setOverviewZoom(2).setCopyright(true));
*
*
*
* **Applies to**: S0 and S1 pricing tiers.
*
*
This API returns legal and technical information for the viewport described in the request. It should be
* called by client applications whenever the viewport changes (for instance, through zooming, panning, going to a
* location, or displaying a route). The request should contain the bounding box and zoom level of the viewport
* whose information is needed. The return will contain map version information, as well as the current Traffic
* Model ID and copyright IDs. The Traffic Model ID returned by the Viewport Description is used by other APIs to
* retrieve last traffic information for further processing.
*
* @param context The context to associate with this operation.
* @param options {@link TrafficIncidentViewportOptions} the options to be used in this search.
* @throws IllegalArgumentException thrown if parameters fail the validation.
* @throws ErrorResponseException thrown if the request is rejected by server.
* @throws RuntimeException all other wrapped checked exceptions if the request fails to be sent.
* @return this object is returned from a successful Traffic Incident Viewport call.
*/
Mono> getTrafficIncidentViewportWithResponse(TrafficIncidentViewportOptions options, Context context) {
return this.serviceClient.getTrafficIncidentViewportWithResponseAsync(
ResponseFormat.JSON,
Utility.toBoundingBox(options.getBoundingBox()),
options.getBoundingZoom(),
Utility.toBoundingBox(options.getOverviewBox()),
options.getOverviewZoom(),
options.getCopyright(),
context).onErrorMap(throwable -> {
if (!(throwable instanceof ErrorResponseException)) {
return throwable;
}
ErrorResponseException exception = (ErrorResponseException) throwable;
return new HttpResponseException(exception.getMessage(), exception.getResponse());
});
}
}